c# find all filenames in directory that contain a value specified in list/array/datatable etc
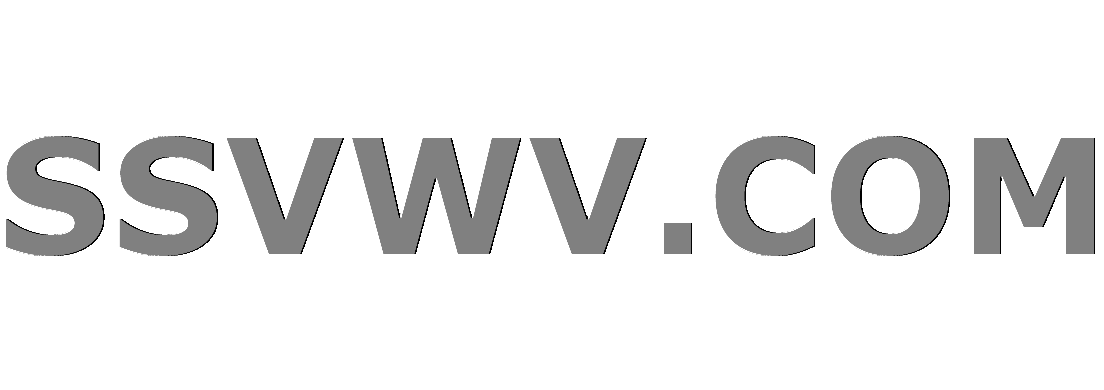
Multi tool use
Using c# .NET 4.6.1 I have a group of strings I want to use to select "valid" files from a given directory. Here's what I mean:
Here is an example of the strings I want to use to get expected filenames from a directory that are stored in an array. I don't care if the values are stored in an array or a list or some other kind of collection. I can put them in whatever kind of collection that would work best for searching for the file names I want.
//"ValidValues" doesn't have to be an array,
//it can be a list/array/datatable or some other kind of collection
string ValidValues = new string[2] {"ab","cd"};
Files in the directory:
file_1_.txt
file_ab_.blah
file_2_.something
file_cd_.blo
etc.
I want to search for the filenames in the directory using the ValidValues
collection that contains the strings I want to use as my search criteria. I've gotten filenames out of a directory in the past using this:
string ValidFiles = Directory.GetFiles(@"C:Drop");
But I don't know how to specify a filter that uses values contained in a collection to be searched for in the filenames in a directory. I know that there are lambda expressions that might be able to do this but I'm not quite sure how to use them. After the code I'm looking for is run I would expect to have
file_ab_.blah
and
file_cd_.blo
in ValidFiles
.
I've seen some posts where the search criteria is hard coded and separated by "||" or others where the collection of search strings is looped through but I want to make this dynamic by using ValidValues
to find filenames in my directory that contain any of the values in ValidValues
. I'm getting the strings contained in ValidValues
from a database.
Hopefully this makes sense, I have a strong feeling that it can be done I just don't know the exact syntax to accomplish this. I don't care how it's done, using LINQ or whatever else, I just want the most elegant and efficient method possible. If possible I'd like to avoid looping through the collection of strings I get out of the database and instead use the collection of strings I get out of the database as search criteria in a single statement, possibly using something like a lambda expression or something of the like. Thanks in advance.
c# search getfiles
add a comment |
Using c# .NET 4.6.1 I have a group of strings I want to use to select "valid" files from a given directory. Here's what I mean:
Here is an example of the strings I want to use to get expected filenames from a directory that are stored in an array. I don't care if the values are stored in an array or a list or some other kind of collection. I can put them in whatever kind of collection that would work best for searching for the file names I want.
//"ValidValues" doesn't have to be an array,
//it can be a list/array/datatable or some other kind of collection
string ValidValues = new string[2] {"ab","cd"};
Files in the directory:
file_1_.txt
file_ab_.blah
file_2_.something
file_cd_.blo
etc.
I want to search for the filenames in the directory using the ValidValues
collection that contains the strings I want to use as my search criteria. I've gotten filenames out of a directory in the past using this:
string ValidFiles = Directory.GetFiles(@"C:Drop");
But I don't know how to specify a filter that uses values contained in a collection to be searched for in the filenames in a directory. I know that there are lambda expressions that might be able to do this but I'm not quite sure how to use them. After the code I'm looking for is run I would expect to have
file_ab_.blah
and
file_cd_.blo
in ValidFiles
.
I've seen some posts where the search criteria is hard coded and separated by "||" or others where the collection of search strings is looped through but I want to make this dynamic by using ValidValues
to find filenames in my directory that contain any of the values in ValidValues
. I'm getting the strings contained in ValidValues
from a database.
Hopefully this makes sense, I have a strong feeling that it can be done I just don't know the exact syntax to accomplish this. I don't care how it's done, using LINQ or whatever else, I just want the most elegant and efficient method possible. If possible I'd like to avoid looping through the collection of strings I get out of the database and instead use the collection of strings I get out of the database as search criteria in a single statement, possibly using something like a lambda expression or something of the like. Thanks in advance.
c# search getfiles
The problem with this question is you haven't really done enough research, i mean there are many solutions to this, and with all the information its still a little unclear what you are actually wanting. What i suggest is you break your problems down in to small components. 1 get data from database, 2 enumerate files. 3 Filters files, 4... then when you have a problem with 1 aspect of this then ask the question with your code, and a detailed debugged description of whats not working, what you expect and why
– Michael Randall
Nov 25 '18 at 4:23
Sorry, I'll try to be more specific. 1. I have a collection (a list or array or whatever) called "ValidValues" that contains the strings "ab" and "cd". 2. I have a directory with files in it. 3. I want to find all of the filenames in the directory that contain any of the strings in "ValidValues", in this example "ab" or "cd". 4. I want to do this with a single statement if possible without looping through the elements in "ValidValues". I don't have any code yet to do this, that's why I'm asking the question. Hope this clarifies it a bit.
– user7789299
Nov 25 '18 at 4:30
Searching and research is not about finding some snippet of code that does exactly what you want. Rather to find out about the concepts you need to formulate your own solution
– Make StackOverflow Good Again
Nov 25 '18 at 7:26
I'm sorry I didn't communicate what I had done up to this point more clearly. I have modified my OP to show the steps I went through that led me to the point of asking this question.If my OP could be reviewed so that I would not be restricted from asking questions in the future based off of this I would appreciate it. Thanks.
– user7789299
Nov 25 '18 at 22:28
add a comment |
Using c# .NET 4.6.1 I have a group of strings I want to use to select "valid" files from a given directory. Here's what I mean:
Here is an example of the strings I want to use to get expected filenames from a directory that are stored in an array. I don't care if the values are stored in an array or a list or some other kind of collection. I can put them in whatever kind of collection that would work best for searching for the file names I want.
//"ValidValues" doesn't have to be an array,
//it can be a list/array/datatable or some other kind of collection
string ValidValues = new string[2] {"ab","cd"};
Files in the directory:
file_1_.txt
file_ab_.blah
file_2_.something
file_cd_.blo
etc.
I want to search for the filenames in the directory using the ValidValues
collection that contains the strings I want to use as my search criteria. I've gotten filenames out of a directory in the past using this:
string ValidFiles = Directory.GetFiles(@"C:Drop");
But I don't know how to specify a filter that uses values contained in a collection to be searched for in the filenames in a directory. I know that there are lambda expressions that might be able to do this but I'm not quite sure how to use them. After the code I'm looking for is run I would expect to have
file_ab_.blah
and
file_cd_.blo
in ValidFiles
.
I've seen some posts where the search criteria is hard coded and separated by "||" or others where the collection of search strings is looped through but I want to make this dynamic by using ValidValues
to find filenames in my directory that contain any of the values in ValidValues
. I'm getting the strings contained in ValidValues
from a database.
Hopefully this makes sense, I have a strong feeling that it can be done I just don't know the exact syntax to accomplish this. I don't care how it's done, using LINQ or whatever else, I just want the most elegant and efficient method possible. If possible I'd like to avoid looping through the collection of strings I get out of the database and instead use the collection of strings I get out of the database as search criteria in a single statement, possibly using something like a lambda expression or something of the like. Thanks in advance.
c# search getfiles
Using c# .NET 4.6.1 I have a group of strings I want to use to select "valid" files from a given directory. Here's what I mean:
Here is an example of the strings I want to use to get expected filenames from a directory that are stored in an array. I don't care if the values are stored in an array or a list or some other kind of collection. I can put them in whatever kind of collection that would work best for searching for the file names I want.
//"ValidValues" doesn't have to be an array,
//it can be a list/array/datatable or some other kind of collection
string ValidValues = new string[2] {"ab","cd"};
Files in the directory:
file_1_.txt
file_ab_.blah
file_2_.something
file_cd_.blo
etc.
I want to search for the filenames in the directory using the ValidValues
collection that contains the strings I want to use as my search criteria. I've gotten filenames out of a directory in the past using this:
string ValidFiles = Directory.GetFiles(@"C:Drop");
But I don't know how to specify a filter that uses values contained in a collection to be searched for in the filenames in a directory. I know that there are lambda expressions that might be able to do this but I'm not quite sure how to use them. After the code I'm looking for is run I would expect to have
file_ab_.blah
and
file_cd_.blo
in ValidFiles
.
I've seen some posts where the search criteria is hard coded and separated by "||" or others where the collection of search strings is looped through but I want to make this dynamic by using ValidValues
to find filenames in my directory that contain any of the values in ValidValues
. I'm getting the strings contained in ValidValues
from a database.
Hopefully this makes sense, I have a strong feeling that it can be done I just don't know the exact syntax to accomplish this. I don't care how it's done, using LINQ or whatever else, I just want the most elegant and efficient method possible. If possible I'd like to avoid looping through the collection of strings I get out of the database and instead use the collection of strings I get out of the database as search criteria in a single statement, possibly using something like a lambda expression or something of the like. Thanks in advance.
c# search getfiles
c# search getfiles
edited Nov 25 '18 at 22:26
asked Nov 25 '18 at 4:07
user7789299
The problem with this question is you haven't really done enough research, i mean there are many solutions to this, and with all the information its still a little unclear what you are actually wanting. What i suggest is you break your problems down in to small components. 1 get data from database, 2 enumerate files. 3 Filters files, 4... then when you have a problem with 1 aspect of this then ask the question with your code, and a detailed debugged description of whats not working, what you expect and why
– Michael Randall
Nov 25 '18 at 4:23
Sorry, I'll try to be more specific. 1. I have a collection (a list or array or whatever) called "ValidValues" that contains the strings "ab" and "cd". 2. I have a directory with files in it. 3. I want to find all of the filenames in the directory that contain any of the strings in "ValidValues", in this example "ab" or "cd". 4. I want to do this with a single statement if possible without looping through the elements in "ValidValues". I don't have any code yet to do this, that's why I'm asking the question. Hope this clarifies it a bit.
– user7789299
Nov 25 '18 at 4:30
Searching and research is not about finding some snippet of code that does exactly what you want. Rather to find out about the concepts you need to formulate your own solution
– Make StackOverflow Good Again
Nov 25 '18 at 7:26
I'm sorry I didn't communicate what I had done up to this point more clearly. I have modified my OP to show the steps I went through that led me to the point of asking this question.If my OP could be reviewed so that I would not be restricted from asking questions in the future based off of this I would appreciate it. Thanks.
– user7789299
Nov 25 '18 at 22:28
add a comment |
The problem with this question is you haven't really done enough research, i mean there are many solutions to this, and with all the information its still a little unclear what you are actually wanting. What i suggest is you break your problems down in to small components. 1 get data from database, 2 enumerate files. 3 Filters files, 4... then when you have a problem with 1 aspect of this then ask the question with your code, and a detailed debugged description of whats not working, what you expect and why
– Michael Randall
Nov 25 '18 at 4:23
Sorry, I'll try to be more specific. 1. I have a collection (a list or array or whatever) called "ValidValues" that contains the strings "ab" and "cd". 2. I have a directory with files in it. 3. I want to find all of the filenames in the directory that contain any of the strings in "ValidValues", in this example "ab" or "cd". 4. I want to do this with a single statement if possible without looping through the elements in "ValidValues". I don't have any code yet to do this, that's why I'm asking the question. Hope this clarifies it a bit.
– user7789299
Nov 25 '18 at 4:30
Searching and research is not about finding some snippet of code that does exactly what you want. Rather to find out about the concepts you need to formulate your own solution
– Make StackOverflow Good Again
Nov 25 '18 at 7:26
I'm sorry I didn't communicate what I had done up to this point more clearly. I have modified my OP to show the steps I went through that led me to the point of asking this question.If my OP could be reviewed so that I would not be restricted from asking questions in the future based off of this I would appreciate it. Thanks.
– user7789299
Nov 25 '18 at 22:28
The problem with this question is you haven't really done enough research, i mean there are many solutions to this, and with all the information its still a little unclear what you are actually wanting. What i suggest is you break your problems down in to small components. 1 get data from database, 2 enumerate files. 3 Filters files, 4... then when you have a problem with 1 aspect of this then ask the question with your code, and a detailed debugged description of whats not working, what you expect and why
– Michael Randall
Nov 25 '18 at 4:23
The problem with this question is you haven't really done enough research, i mean there are many solutions to this, and with all the information its still a little unclear what you are actually wanting. What i suggest is you break your problems down in to small components. 1 get data from database, 2 enumerate files. 3 Filters files, 4... then when you have a problem with 1 aspect of this then ask the question with your code, and a detailed debugged description of whats not working, what you expect and why
– Michael Randall
Nov 25 '18 at 4:23
Sorry, I'll try to be more specific. 1. I have a collection (a list or array or whatever) called "ValidValues" that contains the strings "ab" and "cd". 2. I have a directory with files in it. 3. I want to find all of the filenames in the directory that contain any of the strings in "ValidValues", in this example "ab" or "cd". 4. I want to do this with a single statement if possible without looping through the elements in "ValidValues". I don't have any code yet to do this, that's why I'm asking the question. Hope this clarifies it a bit.
– user7789299
Nov 25 '18 at 4:30
Sorry, I'll try to be more specific. 1. I have a collection (a list or array or whatever) called "ValidValues" that contains the strings "ab" and "cd". 2. I have a directory with files in it. 3. I want to find all of the filenames in the directory that contain any of the strings in "ValidValues", in this example "ab" or "cd". 4. I want to do this with a single statement if possible without looping through the elements in "ValidValues". I don't have any code yet to do this, that's why I'm asking the question. Hope this clarifies it a bit.
– user7789299
Nov 25 '18 at 4:30
Searching and research is not about finding some snippet of code that does exactly what you want. Rather to find out about the concepts you need to formulate your own solution
– Make StackOverflow Good Again
Nov 25 '18 at 7:26
Searching and research is not about finding some snippet of code that does exactly what you want. Rather to find out about the concepts you need to formulate your own solution
– Make StackOverflow Good Again
Nov 25 '18 at 7:26
I'm sorry I didn't communicate what I had done up to this point more clearly. I have modified my OP to show the steps I went through that led me to the point of asking this question.If my OP could be reviewed so that I would not be restricted from asking questions in the future based off of this I would appreciate it. Thanks.
– user7789299
Nov 25 '18 at 22:28
I'm sorry I didn't communicate what I had done up to this point more clearly. I have modified my OP to show the steps I went through that led me to the point of asking this question.If my OP could be reviewed so that I would not be restricted from asking questions in the future based off of this I would appreciate it. Thanks.
– user7789299
Nov 25 '18 at 22:28
add a comment |
1 Answer
1
active
oldest
votes
Considering you already have your filter keywords in a Collection, you could do the following.
var filteredFileList = ValidStrings.SelectMany(filter => Directory.EnumerateFiles(filePath, $"*{filter}*"))
.ToList();
Where ValidStrings is collection of your filter keywords, filePath is your location to search
1
this is along the lines of what I'm looking for. So, say my values from the database are stored in a collection called ValidStrings, how would your suggestion look?
– user7789299
Nov 25 '18 at 4:27
1
I have updated the code with your specified collection name 'ValidStrings'
– Anu Viswan
Nov 25 '18 at 4:29
1
so$"*{filter}*"
should stay as is in the statement?
– user7789299
Nov 25 '18 at 4:32
1
yes it would, "filter" in this context is the parameter name within the Lambda expression. You can rename it to anything, as per your naming conventions, as long as it matches the LHS
– Anu Viswan
Nov 25 '18 at 4:33
1
Just got it set up as you outlined in your answer and it works like a charm. Accepted as answer and tried to up vote but my reputation is too low for it to show. I don't understand why I got a down vote for this question, it seems like I was criticized for not having any code but I didn't have any to show, hence the reason for asking this question. Perhaps someone could do me a solid and up vote this question for me to counter act the down vote?? ; ) Thanks @Anu Viswan
– user7789299
Nov 25 '18 at 5:35
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53464568%2fc-sharp-find-all-filenames-in-directory-that-contain-a-value-specified-in-list-a%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
Considering you already have your filter keywords in a Collection, you could do the following.
var filteredFileList = ValidStrings.SelectMany(filter => Directory.EnumerateFiles(filePath, $"*{filter}*"))
.ToList();
Where ValidStrings is collection of your filter keywords, filePath is your location to search
1
this is along the lines of what I'm looking for. So, say my values from the database are stored in a collection called ValidStrings, how would your suggestion look?
– user7789299
Nov 25 '18 at 4:27
1
I have updated the code with your specified collection name 'ValidStrings'
– Anu Viswan
Nov 25 '18 at 4:29
1
so$"*{filter}*"
should stay as is in the statement?
– user7789299
Nov 25 '18 at 4:32
1
yes it would, "filter" in this context is the parameter name within the Lambda expression. You can rename it to anything, as per your naming conventions, as long as it matches the LHS
– Anu Viswan
Nov 25 '18 at 4:33
1
Just got it set up as you outlined in your answer and it works like a charm. Accepted as answer and tried to up vote but my reputation is too low for it to show. I don't understand why I got a down vote for this question, it seems like I was criticized for not having any code but I didn't have any to show, hence the reason for asking this question. Perhaps someone could do me a solid and up vote this question for me to counter act the down vote?? ; ) Thanks @Anu Viswan
– user7789299
Nov 25 '18 at 5:35
add a comment |
Considering you already have your filter keywords in a Collection, you could do the following.
var filteredFileList = ValidStrings.SelectMany(filter => Directory.EnumerateFiles(filePath, $"*{filter}*"))
.ToList();
Where ValidStrings is collection of your filter keywords, filePath is your location to search
1
this is along the lines of what I'm looking for. So, say my values from the database are stored in a collection called ValidStrings, how would your suggestion look?
– user7789299
Nov 25 '18 at 4:27
1
I have updated the code with your specified collection name 'ValidStrings'
– Anu Viswan
Nov 25 '18 at 4:29
1
so$"*{filter}*"
should stay as is in the statement?
– user7789299
Nov 25 '18 at 4:32
1
yes it would, "filter" in this context is the parameter name within the Lambda expression. You can rename it to anything, as per your naming conventions, as long as it matches the LHS
– Anu Viswan
Nov 25 '18 at 4:33
1
Just got it set up as you outlined in your answer and it works like a charm. Accepted as answer and tried to up vote but my reputation is too low for it to show. I don't understand why I got a down vote for this question, it seems like I was criticized for not having any code but I didn't have any to show, hence the reason for asking this question. Perhaps someone could do me a solid and up vote this question for me to counter act the down vote?? ; ) Thanks @Anu Viswan
– user7789299
Nov 25 '18 at 5:35
add a comment |
Considering you already have your filter keywords in a Collection, you could do the following.
var filteredFileList = ValidStrings.SelectMany(filter => Directory.EnumerateFiles(filePath, $"*{filter}*"))
.ToList();
Where ValidStrings is collection of your filter keywords, filePath is your location to search
Considering you already have your filter keywords in a Collection, you could do the following.
var filteredFileList = ValidStrings.SelectMany(filter => Directory.EnumerateFiles(filePath, $"*{filter}*"))
.ToList();
Where ValidStrings is collection of your filter keywords, filePath is your location to search
edited Nov 25 '18 at 4:28
answered Nov 25 '18 at 4:25


Anu ViswanAnu Viswan
5,6802526
5,6802526
1
this is along the lines of what I'm looking for. So, say my values from the database are stored in a collection called ValidStrings, how would your suggestion look?
– user7789299
Nov 25 '18 at 4:27
1
I have updated the code with your specified collection name 'ValidStrings'
– Anu Viswan
Nov 25 '18 at 4:29
1
so$"*{filter}*"
should stay as is in the statement?
– user7789299
Nov 25 '18 at 4:32
1
yes it would, "filter" in this context is the parameter name within the Lambda expression. You can rename it to anything, as per your naming conventions, as long as it matches the LHS
– Anu Viswan
Nov 25 '18 at 4:33
1
Just got it set up as you outlined in your answer and it works like a charm. Accepted as answer and tried to up vote but my reputation is too low for it to show. I don't understand why I got a down vote for this question, it seems like I was criticized for not having any code but I didn't have any to show, hence the reason for asking this question. Perhaps someone could do me a solid and up vote this question for me to counter act the down vote?? ; ) Thanks @Anu Viswan
– user7789299
Nov 25 '18 at 5:35
add a comment |
1
this is along the lines of what I'm looking for. So, say my values from the database are stored in a collection called ValidStrings, how would your suggestion look?
– user7789299
Nov 25 '18 at 4:27
1
I have updated the code with your specified collection name 'ValidStrings'
– Anu Viswan
Nov 25 '18 at 4:29
1
so$"*{filter}*"
should stay as is in the statement?
– user7789299
Nov 25 '18 at 4:32
1
yes it would, "filter" in this context is the parameter name within the Lambda expression. You can rename it to anything, as per your naming conventions, as long as it matches the LHS
– Anu Viswan
Nov 25 '18 at 4:33
1
Just got it set up as you outlined in your answer and it works like a charm. Accepted as answer and tried to up vote but my reputation is too low for it to show. I don't understand why I got a down vote for this question, it seems like I was criticized for not having any code but I didn't have any to show, hence the reason for asking this question. Perhaps someone could do me a solid and up vote this question for me to counter act the down vote?? ; ) Thanks @Anu Viswan
– user7789299
Nov 25 '18 at 5:35
1
1
this is along the lines of what I'm looking for. So, say my values from the database are stored in a collection called ValidStrings, how would your suggestion look?
– user7789299
Nov 25 '18 at 4:27
this is along the lines of what I'm looking for. So, say my values from the database are stored in a collection called ValidStrings, how would your suggestion look?
– user7789299
Nov 25 '18 at 4:27
1
1
I have updated the code with your specified collection name 'ValidStrings'
– Anu Viswan
Nov 25 '18 at 4:29
I have updated the code with your specified collection name 'ValidStrings'
– Anu Viswan
Nov 25 '18 at 4:29
1
1
so
$"*{filter}*"
should stay as is in the statement?– user7789299
Nov 25 '18 at 4:32
so
$"*{filter}*"
should stay as is in the statement?– user7789299
Nov 25 '18 at 4:32
1
1
yes it would, "filter" in this context is the parameter name within the Lambda expression. You can rename it to anything, as per your naming conventions, as long as it matches the LHS
– Anu Viswan
Nov 25 '18 at 4:33
yes it would, "filter" in this context is the parameter name within the Lambda expression. You can rename it to anything, as per your naming conventions, as long as it matches the LHS
– Anu Viswan
Nov 25 '18 at 4:33
1
1
Just got it set up as you outlined in your answer and it works like a charm. Accepted as answer and tried to up vote but my reputation is too low for it to show. I don't understand why I got a down vote for this question, it seems like I was criticized for not having any code but I didn't have any to show, hence the reason for asking this question. Perhaps someone could do me a solid and up vote this question for me to counter act the down vote?? ; ) Thanks @Anu Viswan
– user7789299
Nov 25 '18 at 5:35
Just got it set up as you outlined in your answer and it works like a charm. Accepted as answer and tried to up vote but my reputation is too low for it to show. I don't understand why I got a down vote for this question, it seems like I was criticized for not having any code but I didn't have any to show, hence the reason for asking this question. Perhaps someone could do me a solid and up vote this question for me to counter act the down vote?? ; ) Thanks @Anu Viswan
– user7789299
Nov 25 '18 at 5:35
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53464568%2fc-sharp-find-all-filenames-in-directory-that-contain-a-value-specified-in-list-a%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
AGU4i ircqji,ON5xKRv13Qkyr1fjeTquGHIKjRJ xGZz 4iT
The problem with this question is you haven't really done enough research, i mean there are many solutions to this, and with all the information its still a little unclear what you are actually wanting. What i suggest is you break your problems down in to small components. 1 get data from database, 2 enumerate files. 3 Filters files, 4... then when you have a problem with 1 aspect of this then ask the question with your code, and a detailed debugged description of whats not working, what you expect and why
– Michael Randall
Nov 25 '18 at 4:23
Sorry, I'll try to be more specific. 1. I have a collection (a list or array or whatever) called "ValidValues" that contains the strings "ab" and "cd". 2. I have a directory with files in it. 3. I want to find all of the filenames in the directory that contain any of the strings in "ValidValues", in this example "ab" or "cd". 4. I want to do this with a single statement if possible without looping through the elements in "ValidValues". I don't have any code yet to do this, that's why I'm asking the question. Hope this clarifies it a bit.
– user7789299
Nov 25 '18 at 4:30
Searching and research is not about finding some snippet of code that does exactly what you want. Rather to find out about the concepts you need to formulate your own solution
– Make StackOverflow Good Again
Nov 25 '18 at 7:26
I'm sorry I didn't communicate what I had done up to this point more clearly. I have modified my OP to show the steps I went through that led me to the point of asking this question.If my OP could be reviewed so that I would not be restricted from asking questions in the future based off of this I would appreciate it. Thanks.
– user7789299
Nov 25 '18 at 22:28