How to Use Recursion to Generate Arrays in Python
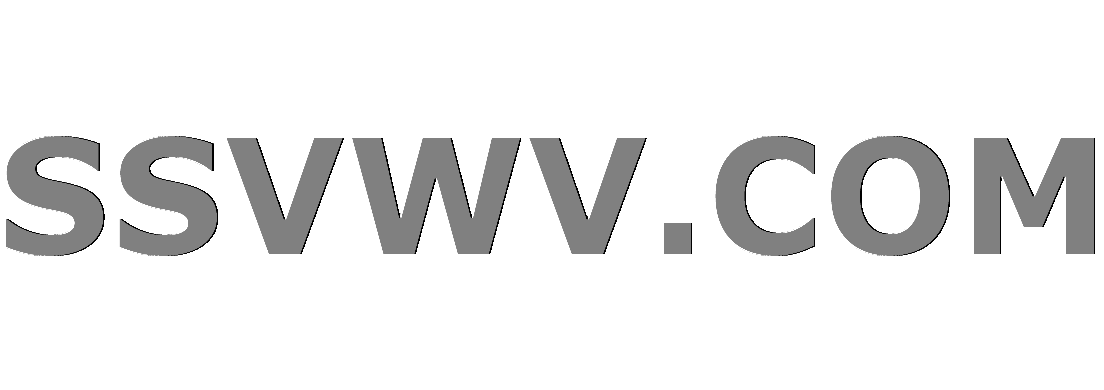
Multi tool use
I am trying to generate all possible arrays with values from 1 - 9 using recursion in python. My code is below:
totalArr =
def recursion(arr, n):
for i in range(9):
if (arr[i] == 0):
arr[i] = n
if (n < 8):
recursion(arr, n + 1)
else:
print(arr)
totalArr.append(arr)
recursion([0, 0, 0, 0, 0, 0, 0, 0, 0], 0)
print(len(totalArr))
When I run this code, all I get is the single array below:
[1, 2, 3, 4, 5, 6, 7, 8, 9]
I am aware that I can use permutations to the arrays, however for my use case of these arrays, I believe that recursion is better in the long run.
python arrays python-3.x list recursion
|
show 3 more comments
I am trying to generate all possible arrays with values from 1 - 9 using recursion in python. My code is below:
totalArr =
def recursion(arr, n):
for i in range(9):
if (arr[i] == 0):
arr[i] = n
if (n < 8):
recursion(arr, n + 1)
else:
print(arr)
totalArr.append(arr)
recursion([0, 0, 0, 0, 0, 0, 0, 0, 0], 0)
print(len(totalArr))
When I run this code, all I get is the single array below:
[1, 2, 3, 4, 5, 6, 7, 8, 9]
I am aware that I can use permutations to the arrays, however for my use case of these arrays, I believe that recursion is better in the long run.
python arrays python-3.x list recursion
2
arr[i] == n
does not assignn
toarr[i]
. You need=
, not equality operator.
– Austin
Nov 25 '18 at 3:38
2
What output are you expecting?
– 0liveradam8
Nov 25 '18 at 3:50
Welcome to SO! "I am aware that I can use permutations to the arrays, however for my use case of these arrays, I believe that recursion is better in the long run." Permutation != recursion. The definition of "all possible arrays with values 1-9" is all permutations of an input array with those values, and you can do this recursively, which is what you appear to be attempting. What is the problem with your code (infinite loop due to==
typo aside)?
– ggorlen
Nov 25 '18 at 3:57
"however for my use case of these arrays, I believe that recursion is better in the long run"... why do you believe that?
– Mateen Ulhaq
Nov 25 '18 at 4:43
Yeah, sorry about that typo. The problem with my code is that all the arrays it creates are the same [1, 2, 3, 4, 5, 6, 7, 8, 9]
– Luke Schultz
Nov 25 '18 at 4:43
|
show 3 more comments
I am trying to generate all possible arrays with values from 1 - 9 using recursion in python. My code is below:
totalArr =
def recursion(arr, n):
for i in range(9):
if (arr[i] == 0):
arr[i] = n
if (n < 8):
recursion(arr, n + 1)
else:
print(arr)
totalArr.append(arr)
recursion([0, 0, 0, 0, 0, 0, 0, 0, 0], 0)
print(len(totalArr))
When I run this code, all I get is the single array below:
[1, 2, 3, 4, 5, 6, 7, 8, 9]
I am aware that I can use permutations to the arrays, however for my use case of these arrays, I believe that recursion is better in the long run.
python arrays python-3.x list recursion
I am trying to generate all possible arrays with values from 1 - 9 using recursion in python. My code is below:
totalArr =
def recursion(arr, n):
for i in range(9):
if (arr[i] == 0):
arr[i] = n
if (n < 8):
recursion(arr, n + 1)
else:
print(arr)
totalArr.append(arr)
recursion([0, 0, 0, 0, 0, 0, 0, 0, 0], 0)
print(len(totalArr))
When I run this code, all I get is the single array below:
[1, 2, 3, 4, 5, 6, 7, 8, 9]
I am aware that I can use permutations to the arrays, however for my use case of these arrays, I believe that recursion is better in the long run.
python arrays python-3.x list recursion
python arrays python-3.x list recursion
edited Nov 25 '18 at 4:40
Luke Schultz
asked Nov 25 '18 at 3:36


Luke SchultzLuke Schultz
464
464
2
arr[i] == n
does not assignn
toarr[i]
. You need=
, not equality operator.
– Austin
Nov 25 '18 at 3:38
2
What output are you expecting?
– 0liveradam8
Nov 25 '18 at 3:50
Welcome to SO! "I am aware that I can use permutations to the arrays, however for my use case of these arrays, I believe that recursion is better in the long run." Permutation != recursion. The definition of "all possible arrays with values 1-9" is all permutations of an input array with those values, and you can do this recursively, which is what you appear to be attempting. What is the problem with your code (infinite loop due to==
typo aside)?
– ggorlen
Nov 25 '18 at 3:57
"however for my use case of these arrays, I believe that recursion is better in the long run"... why do you believe that?
– Mateen Ulhaq
Nov 25 '18 at 4:43
Yeah, sorry about that typo. The problem with my code is that all the arrays it creates are the same [1, 2, 3, 4, 5, 6, 7, 8, 9]
– Luke Schultz
Nov 25 '18 at 4:43
|
show 3 more comments
2
arr[i] == n
does not assignn
toarr[i]
. You need=
, not equality operator.
– Austin
Nov 25 '18 at 3:38
2
What output are you expecting?
– 0liveradam8
Nov 25 '18 at 3:50
Welcome to SO! "I am aware that I can use permutations to the arrays, however for my use case of these arrays, I believe that recursion is better in the long run." Permutation != recursion. The definition of "all possible arrays with values 1-9" is all permutations of an input array with those values, and you can do this recursively, which is what you appear to be attempting. What is the problem with your code (infinite loop due to==
typo aside)?
– ggorlen
Nov 25 '18 at 3:57
"however for my use case of these arrays, I believe that recursion is better in the long run"... why do you believe that?
– Mateen Ulhaq
Nov 25 '18 at 4:43
Yeah, sorry about that typo. The problem with my code is that all the arrays it creates are the same [1, 2, 3, 4, 5, 6, 7, 8, 9]
– Luke Schultz
Nov 25 '18 at 4:43
2
2
arr[i] == n
does not assign n
to arr[i]
. You need =
, not equality operator.– Austin
Nov 25 '18 at 3:38
arr[i] == n
does not assign n
to arr[i]
. You need =
, not equality operator.– Austin
Nov 25 '18 at 3:38
2
2
What output are you expecting?
– 0liveradam8
Nov 25 '18 at 3:50
What output are you expecting?
– 0liveradam8
Nov 25 '18 at 3:50
Welcome to SO! "I am aware that I can use permutations to the arrays, however for my use case of these arrays, I believe that recursion is better in the long run." Permutation != recursion. The definition of "all possible arrays with values 1-9" is all permutations of an input array with those values, and you can do this recursively, which is what you appear to be attempting. What is the problem with your code (infinite loop due to
==
typo aside)?– ggorlen
Nov 25 '18 at 3:57
Welcome to SO! "I am aware that I can use permutations to the arrays, however for my use case of these arrays, I believe that recursion is better in the long run." Permutation != recursion. The definition of "all possible arrays with values 1-9" is all permutations of an input array with those values, and you can do this recursively, which is what you appear to be attempting. What is the problem with your code (infinite loop due to
==
typo aside)?– ggorlen
Nov 25 '18 at 3:57
"however for my use case of these arrays, I believe that recursion is better in the long run"... why do you believe that?
– Mateen Ulhaq
Nov 25 '18 at 4:43
"however for my use case of these arrays, I believe that recursion is better in the long run"... why do you believe that?
– Mateen Ulhaq
Nov 25 '18 at 4:43
Yeah, sorry about that typo. The problem with my code is that all the arrays it creates are the same [1, 2, 3, 4, 5, 6, 7, 8, 9]
– Luke Schultz
Nov 25 '18 at 4:43
Yeah, sorry about that typo. The problem with my code is that all the arrays it creates are the same [1, 2, 3, 4, 5, 6, 7, 8, 9]
– Luke Schultz
Nov 25 '18 at 4:43
|
show 3 more comments
2 Answers
2
active
oldest
votes
# Python program to print all permutations with
# duplicates allowed
# Function to print permutations of my_array
# This function takes three parameters:
# 1. my_array
# 2. Starting index of the my_array
# 3. Ending index of the my_array.
def permute(a, l, r):
if l==r:
print a
else:
for i in xrange(l,r+1):
a[l], a[i] = a[i], a[l]
permute(a, l+1, r)
a[l], a[i] = a[i], a[l] # backtrack
# Driver program to test the above function
my_array = [0,1,2,3,4,5,6,7,8,9]
n = len(my_array)
a = list(my_array)
permute(a, 0, n-1)
We are doing permutation by using recursion with backtracking :
More info on https://www.geeksforgeeks.org/write-a-c-program-to-print-all-permutations-of-a-given-string/
add a comment |
totalArr=
def permute(lst,n):
''' O(n!), optimal'''
if n==1:totalArr.append(lst.copy())
else:
for i in range(n):
lst[i],lst[n-1] = lst[n-1],lst[i]
permute(lst,n-1)
lst[i],lst[n-1] = lst[n-1],lst[i]
lst = [i for i in range(1,9)] # any other lst with unique elements is ok
permute(lst,len(lst))
print(totalArr)
This method can generate all permutations using divide-and-conquer algorithm
When I run your code, all it generates is arrays filled with zeros [0, 0, 0, 0, 0, 0, 0, 0, 0]
– Luke Schultz
Nov 25 '18 at 4:42
@LukeSchultz, there's a line missing in this code where it actually callspermute()
. My guess is something likepermute([1, 2, 3, 4, 5, 6, 7, 8, 9], 9)
before theprint()
statement.
– cdlane
Nov 25 '18 at 6:37
Yes. You should firstly apply functionpermute
in a lst with its length, and then get the result intotalArr
– mbinary
Nov 25 '18 at 12:29
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53464438%2fhow-to-use-recursion-to-generate-arrays-in-python%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
# Python program to print all permutations with
# duplicates allowed
# Function to print permutations of my_array
# This function takes three parameters:
# 1. my_array
# 2. Starting index of the my_array
# 3. Ending index of the my_array.
def permute(a, l, r):
if l==r:
print a
else:
for i in xrange(l,r+1):
a[l], a[i] = a[i], a[l]
permute(a, l+1, r)
a[l], a[i] = a[i], a[l] # backtrack
# Driver program to test the above function
my_array = [0,1,2,3,4,5,6,7,8,9]
n = len(my_array)
a = list(my_array)
permute(a, 0, n-1)
We are doing permutation by using recursion with backtracking :
More info on https://www.geeksforgeeks.org/write-a-c-program-to-print-all-permutations-of-a-given-string/
add a comment |
# Python program to print all permutations with
# duplicates allowed
# Function to print permutations of my_array
# This function takes three parameters:
# 1. my_array
# 2. Starting index of the my_array
# 3. Ending index of the my_array.
def permute(a, l, r):
if l==r:
print a
else:
for i in xrange(l,r+1):
a[l], a[i] = a[i], a[l]
permute(a, l+1, r)
a[l], a[i] = a[i], a[l] # backtrack
# Driver program to test the above function
my_array = [0,1,2,3,4,5,6,7,8,9]
n = len(my_array)
a = list(my_array)
permute(a, 0, n-1)
We are doing permutation by using recursion with backtracking :
More info on https://www.geeksforgeeks.org/write-a-c-program-to-print-all-permutations-of-a-given-string/
add a comment |
# Python program to print all permutations with
# duplicates allowed
# Function to print permutations of my_array
# This function takes three parameters:
# 1. my_array
# 2. Starting index of the my_array
# 3. Ending index of the my_array.
def permute(a, l, r):
if l==r:
print a
else:
for i in xrange(l,r+1):
a[l], a[i] = a[i], a[l]
permute(a, l+1, r)
a[l], a[i] = a[i], a[l] # backtrack
# Driver program to test the above function
my_array = [0,1,2,3,4,5,6,7,8,9]
n = len(my_array)
a = list(my_array)
permute(a, 0, n-1)
We are doing permutation by using recursion with backtracking :
More info on https://www.geeksforgeeks.org/write-a-c-program-to-print-all-permutations-of-a-given-string/
# Python program to print all permutations with
# duplicates allowed
# Function to print permutations of my_array
# This function takes three parameters:
# 1. my_array
# 2. Starting index of the my_array
# 3. Ending index of the my_array.
def permute(a, l, r):
if l==r:
print a
else:
for i in xrange(l,r+1):
a[l], a[i] = a[i], a[l]
permute(a, l+1, r)
a[l], a[i] = a[i], a[l] # backtrack
# Driver program to test the above function
my_array = [0,1,2,3,4,5,6,7,8,9]
n = len(my_array)
a = list(my_array)
permute(a, 0, n-1)
We are doing permutation by using recursion with backtracking :
More info on https://www.geeksforgeeks.org/write-a-c-program-to-print-all-permutations-of-a-given-string/
answered Nov 25 '18 at 5:29


NiksVijNiksVij
676
676
add a comment |
add a comment |
totalArr=
def permute(lst,n):
''' O(n!), optimal'''
if n==1:totalArr.append(lst.copy())
else:
for i in range(n):
lst[i],lst[n-1] = lst[n-1],lst[i]
permute(lst,n-1)
lst[i],lst[n-1] = lst[n-1],lst[i]
lst = [i for i in range(1,9)] # any other lst with unique elements is ok
permute(lst,len(lst))
print(totalArr)
This method can generate all permutations using divide-and-conquer algorithm
When I run your code, all it generates is arrays filled with zeros [0, 0, 0, 0, 0, 0, 0, 0, 0]
– Luke Schultz
Nov 25 '18 at 4:42
@LukeSchultz, there's a line missing in this code where it actually callspermute()
. My guess is something likepermute([1, 2, 3, 4, 5, 6, 7, 8, 9], 9)
before theprint()
statement.
– cdlane
Nov 25 '18 at 6:37
Yes. You should firstly apply functionpermute
in a lst with its length, and then get the result intotalArr
– mbinary
Nov 25 '18 at 12:29
add a comment |
totalArr=
def permute(lst,n):
''' O(n!), optimal'''
if n==1:totalArr.append(lst.copy())
else:
for i in range(n):
lst[i],lst[n-1] = lst[n-1],lst[i]
permute(lst,n-1)
lst[i],lst[n-1] = lst[n-1],lst[i]
lst = [i for i in range(1,9)] # any other lst with unique elements is ok
permute(lst,len(lst))
print(totalArr)
This method can generate all permutations using divide-and-conquer algorithm
When I run your code, all it generates is arrays filled with zeros [0, 0, 0, 0, 0, 0, 0, 0, 0]
– Luke Schultz
Nov 25 '18 at 4:42
@LukeSchultz, there's a line missing in this code where it actually callspermute()
. My guess is something likepermute([1, 2, 3, 4, 5, 6, 7, 8, 9], 9)
before theprint()
statement.
– cdlane
Nov 25 '18 at 6:37
Yes. You should firstly apply functionpermute
in a lst with its length, and then get the result intotalArr
– mbinary
Nov 25 '18 at 12:29
add a comment |
totalArr=
def permute(lst,n):
''' O(n!), optimal'''
if n==1:totalArr.append(lst.copy())
else:
for i in range(n):
lst[i],lst[n-1] = lst[n-1],lst[i]
permute(lst,n-1)
lst[i],lst[n-1] = lst[n-1],lst[i]
lst = [i for i in range(1,9)] # any other lst with unique elements is ok
permute(lst,len(lst))
print(totalArr)
This method can generate all permutations using divide-and-conquer algorithm
totalArr=
def permute(lst,n):
''' O(n!), optimal'''
if n==1:totalArr.append(lst.copy())
else:
for i in range(n):
lst[i],lst[n-1] = lst[n-1],lst[i]
permute(lst,n-1)
lst[i],lst[n-1] = lst[n-1],lst[i]
lst = [i for i in range(1,9)] # any other lst with unique elements is ok
permute(lst,len(lst))
print(totalArr)
This method can generate all permutations using divide-and-conquer algorithm
edited Nov 25 '18 at 12:31
answered Nov 25 '18 at 4:25


mbinarymbinary
11
11
When I run your code, all it generates is arrays filled with zeros [0, 0, 0, 0, 0, 0, 0, 0, 0]
– Luke Schultz
Nov 25 '18 at 4:42
@LukeSchultz, there's a line missing in this code where it actually callspermute()
. My guess is something likepermute([1, 2, 3, 4, 5, 6, 7, 8, 9], 9)
before theprint()
statement.
– cdlane
Nov 25 '18 at 6:37
Yes. You should firstly apply functionpermute
in a lst with its length, and then get the result intotalArr
– mbinary
Nov 25 '18 at 12:29
add a comment |
When I run your code, all it generates is arrays filled with zeros [0, 0, 0, 0, 0, 0, 0, 0, 0]
– Luke Schultz
Nov 25 '18 at 4:42
@LukeSchultz, there's a line missing in this code where it actually callspermute()
. My guess is something likepermute([1, 2, 3, 4, 5, 6, 7, 8, 9], 9)
before theprint()
statement.
– cdlane
Nov 25 '18 at 6:37
Yes. You should firstly apply functionpermute
in a lst with its length, and then get the result intotalArr
– mbinary
Nov 25 '18 at 12:29
When I run your code, all it generates is arrays filled with zeros [0, 0, 0, 0, 0, 0, 0, 0, 0]
– Luke Schultz
Nov 25 '18 at 4:42
When I run your code, all it generates is arrays filled with zeros [0, 0, 0, 0, 0, 0, 0, 0, 0]
– Luke Schultz
Nov 25 '18 at 4:42
@LukeSchultz, there's a line missing in this code where it actually calls
permute()
. My guess is something like permute([1, 2, 3, 4, 5, 6, 7, 8, 9], 9)
before the print()
statement.– cdlane
Nov 25 '18 at 6:37
@LukeSchultz, there's a line missing in this code where it actually calls
permute()
. My guess is something like permute([1, 2, 3, 4, 5, 6, 7, 8, 9], 9)
before the print()
statement.– cdlane
Nov 25 '18 at 6:37
Yes. You should firstly apply function
permute
in a lst with its length, and then get the result in totalArr
– mbinary
Nov 25 '18 at 12:29
Yes. You should firstly apply function
permute
in a lst with its length, and then get the result in totalArr
– mbinary
Nov 25 '18 at 12:29
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53464438%2fhow-to-use-recursion-to-generate-arrays-in-python%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
kPsW3yzKoG2tm,loMrN99f20fFY4dJgNaz,cibZjV2ct,4nFEMbJe P5P2P Hd hTQ9eXp1Cdjz6s5Rf
2
arr[i] == n
does not assignn
toarr[i]
. You need=
, not equality operator.– Austin
Nov 25 '18 at 3:38
2
What output are you expecting?
– 0liveradam8
Nov 25 '18 at 3:50
Welcome to SO! "I am aware that I can use permutations to the arrays, however for my use case of these arrays, I believe that recursion is better in the long run." Permutation != recursion. The definition of "all possible arrays with values 1-9" is all permutations of an input array with those values, and you can do this recursively, which is what you appear to be attempting. What is the problem with your code (infinite loop due to
==
typo aside)?– ggorlen
Nov 25 '18 at 3:57
"however for my use case of these arrays, I believe that recursion is better in the long run"... why do you believe that?
– Mateen Ulhaq
Nov 25 '18 at 4:43
Yeah, sorry about that typo. The problem with my code is that all the arrays it creates are the same [1, 2, 3, 4, 5, 6, 7, 8, 9]
– Luke Schultz
Nov 25 '18 at 4:43