Azure DevOps - .NET Core Build Include Web.config file
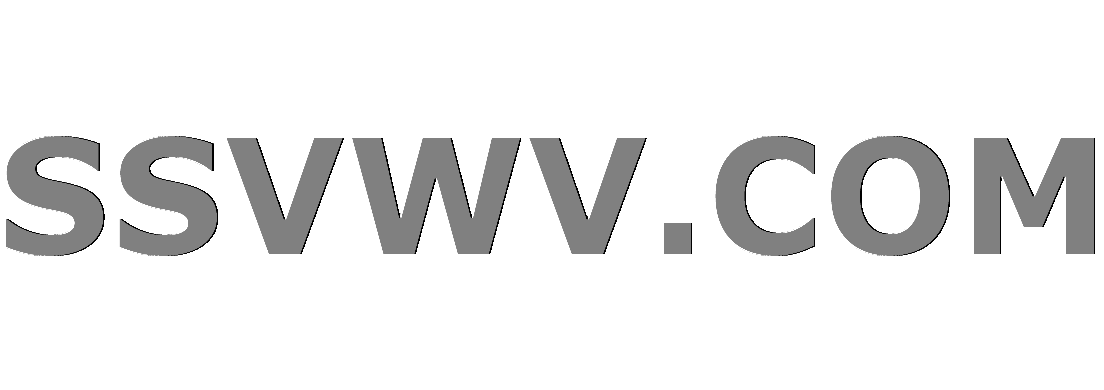
Multi tool use
up vote
1
down vote
favorite
I'm using Azure DevOps for building and releasing a .NET Core MVC web application to a Windows Server 2016 EC2 instance in AWS.
I have different environments, so I've created the following appsettings.json files:
- appsettings.DEV.json
- appsettings.STG.json
- appsettings.PRD.json
After some research, I see that we can set the ASPNETCORE_ENVIRONMENT variable in the web.config file:
<?xml version="1.0" encoding="utf-8"?>
<configuration>
<location path="." inheritInChildApplications="false">
<system.webServer>
<handlers>
<add name="aspNetCore" path="*" verb="*" modules="AspNetCoreModule" resourceType="Unspecified" />
</handlers>
<aspNetCore processPath="dotnet" arguments=".www.MyApp.dll" stdoutLogEnabled="false" stdoutLogFile=".logsstdout">
<environmentVariables>
<environmentVariable name="ASPNETCORE_ENVIRONMENT" value="[ENV]" />
</environmentVariables>
</aspNetCore>
</system.webServer>
</location>
</configuration>
I can then load the respective environment appsetting.json file using the following code in Program.cs:
public class Program
{
public static void Main(string args)
{
CreateWebHostBuilder(args).Build().Run();
}
public static IWebHostBuilder CreateWebHostBuilder(string args) =>
WebHost.CreateDefaultBuilder(args)
.ConfigureAppConfiguration(ConfigConfiguration)
.UseStartup<Startup>();
static void ConfigConfiguration(WebHostBuilderContext ctx, IConfigurationBuilder config)
{
config.SetBasePath(Directory.GetCurrentDirectory())
.AddJsonFile("appsettings.json", optional: false, reloadOnChange: true)
.AddJsonFile($"appsettings.{ctx.HostingEnvironment.EnvironmentName}.json", optional: true, reloadOnChange: true);
}
}
For each deployment, I would like to control the web.config that gets deployed so that I can control the value of ASPNETCORE_ENVIRONMENT. Similar to web.config transform in a traditional ASP.NET environment.
Is there a way to do this in Azure DevOps, or via a setting in Visual Studio? I've read that .NET Core 2.2 will offer a solution for this, but what can be done in the meantime?
c# .net-core

add a comment |
up vote
1
down vote
favorite
I'm using Azure DevOps for building and releasing a .NET Core MVC web application to a Windows Server 2016 EC2 instance in AWS.
I have different environments, so I've created the following appsettings.json files:
- appsettings.DEV.json
- appsettings.STG.json
- appsettings.PRD.json
After some research, I see that we can set the ASPNETCORE_ENVIRONMENT variable in the web.config file:
<?xml version="1.0" encoding="utf-8"?>
<configuration>
<location path="." inheritInChildApplications="false">
<system.webServer>
<handlers>
<add name="aspNetCore" path="*" verb="*" modules="AspNetCoreModule" resourceType="Unspecified" />
</handlers>
<aspNetCore processPath="dotnet" arguments=".www.MyApp.dll" stdoutLogEnabled="false" stdoutLogFile=".logsstdout">
<environmentVariables>
<environmentVariable name="ASPNETCORE_ENVIRONMENT" value="[ENV]" />
</environmentVariables>
</aspNetCore>
</system.webServer>
</location>
</configuration>
I can then load the respective environment appsetting.json file using the following code in Program.cs:
public class Program
{
public static void Main(string args)
{
CreateWebHostBuilder(args).Build().Run();
}
public static IWebHostBuilder CreateWebHostBuilder(string args) =>
WebHost.CreateDefaultBuilder(args)
.ConfigureAppConfiguration(ConfigConfiguration)
.UseStartup<Startup>();
static void ConfigConfiguration(WebHostBuilderContext ctx, IConfigurationBuilder config)
{
config.SetBasePath(Directory.GetCurrentDirectory())
.AddJsonFile("appsettings.json", optional: false, reloadOnChange: true)
.AddJsonFile($"appsettings.{ctx.HostingEnvironment.EnvironmentName}.json", optional: true, reloadOnChange: true);
}
}
For each deployment, I would like to control the web.config that gets deployed so that I can control the value of ASPNETCORE_ENVIRONMENT. Similar to web.config transform in a traditional ASP.NET environment.
Is there a way to do this in Azure DevOps, or via a setting in Visual Studio? I've read that .NET Core 2.2 will offer a solution for this, but what can be done in the meantime?
c# .net-core

Any reason for not using just build variables for replacement?
– Camilo Terevinto
Nov 17 at 19:52
Can you not set the Environment Variable directly on the servers, that is the entire point of using Environment Variables to hold that information. Failing that see @CamiloTerevinto's comment as that would much easier.
– Adam Carr
Nov 17 at 21:28
add a comment |
up vote
1
down vote
favorite
up vote
1
down vote
favorite
I'm using Azure DevOps for building and releasing a .NET Core MVC web application to a Windows Server 2016 EC2 instance in AWS.
I have different environments, so I've created the following appsettings.json files:
- appsettings.DEV.json
- appsettings.STG.json
- appsettings.PRD.json
After some research, I see that we can set the ASPNETCORE_ENVIRONMENT variable in the web.config file:
<?xml version="1.0" encoding="utf-8"?>
<configuration>
<location path="." inheritInChildApplications="false">
<system.webServer>
<handlers>
<add name="aspNetCore" path="*" verb="*" modules="AspNetCoreModule" resourceType="Unspecified" />
</handlers>
<aspNetCore processPath="dotnet" arguments=".www.MyApp.dll" stdoutLogEnabled="false" stdoutLogFile=".logsstdout">
<environmentVariables>
<environmentVariable name="ASPNETCORE_ENVIRONMENT" value="[ENV]" />
</environmentVariables>
</aspNetCore>
</system.webServer>
</location>
</configuration>
I can then load the respective environment appsetting.json file using the following code in Program.cs:
public class Program
{
public static void Main(string args)
{
CreateWebHostBuilder(args).Build().Run();
}
public static IWebHostBuilder CreateWebHostBuilder(string args) =>
WebHost.CreateDefaultBuilder(args)
.ConfigureAppConfiguration(ConfigConfiguration)
.UseStartup<Startup>();
static void ConfigConfiguration(WebHostBuilderContext ctx, IConfigurationBuilder config)
{
config.SetBasePath(Directory.GetCurrentDirectory())
.AddJsonFile("appsettings.json", optional: false, reloadOnChange: true)
.AddJsonFile($"appsettings.{ctx.HostingEnvironment.EnvironmentName}.json", optional: true, reloadOnChange: true);
}
}
For each deployment, I would like to control the web.config that gets deployed so that I can control the value of ASPNETCORE_ENVIRONMENT. Similar to web.config transform in a traditional ASP.NET environment.
Is there a way to do this in Azure DevOps, or via a setting in Visual Studio? I've read that .NET Core 2.2 will offer a solution for this, but what can be done in the meantime?
c# .net-core

I'm using Azure DevOps for building and releasing a .NET Core MVC web application to a Windows Server 2016 EC2 instance in AWS.
I have different environments, so I've created the following appsettings.json files:
- appsettings.DEV.json
- appsettings.STG.json
- appsettings.PRD.json
After some research, I see that we can set the ASPNETCORE_ENVIRONMENT variable in the web.config file:
<?xml version="1.0" encoding="utf-8"?>
<configuration>
<location path="." inheritInChildApplications="false">
<system.webServer>
<handlers>
<add name="aspNetCore" path="*" verb="*" modules="AspNetCoreModule" resourceType="Unspecified" />
</handlers>
<aspNetCore processPath="dotnet" arguments=".www.MyApp.dll" stdoutLogEnabled="false" stdoutLogFile=".logsstdout">
<environmentVariables>
<environmentVariable name="ASPNETCORE_ENVIRONMENT" value="[ENV]" />
</environmentVariables>
</aspNetCore>
</system.webServer>
</location>
</configuration>
I can then load the respective environment appsetting.json file using the following code in Program.cs:
public class Program
{
public static void Main(string args)
{
CreateWebHostBuilder(args).Build().Run();
}
public static IWebHostBuilder CreateWebHostBuilder(string args) =>
WebHost.CreateDefaultBuilder(args)
.ConfigureAppConfiguration(ConfigConfiguration)
.UseStartup<Startup>();
static void ConfigConfiguration(WebHostBuilderContext ctx, IConfigurationBuilder config)
{
config.SetBasePath(Directory.GetCurrentDirectory())
.AddJsonFile("appsettings.json", optional: false, reloadOnChange: true)
.AddJsonFile($"appsettings.{ctx.HostingEnvironment.EnvironmentName}.json", optional: true, reloadOnChange: true);
}
}
For each deployment, I would like to control the web.config that gets deployed so that I can control the value of ASPNETCORE_ENVIRONMENT. Similar to web.config transform in a traditional ASP.NET environment.
Is there a way to do this in Azure DevOps, or via a setting in Visual Studio? I've read that .NET Core 2.2 will offer a solution for this, but what can be done in the meantime?
c# .net-core

c# .net-core

asked Nov 17 at 19:45


Kyle Barnes
771315
771315
Any reason for not using just build variables for replacement?
– Camilo Terevinto
Nov 17 at 19:52
Can you not set the Environment Variable directly on the servers, that is the entire point of using Environment Variables to hold that information. Failing that see @CamiloTerevinto's comment as that would much easier.
– Adam Carr
Nov 17 at 21:28
add a comment |
Any reason for not using just build variables for replacement?
– Camilo Terevinto
Nov 17 at 19:52
Can you not set the Environment Variable directly on the servers, that is the entire point of using Environment Variables to hold that information. Failing that see @CamiloTerevinto's comment as that would much easier.
– Adam Carr
Nov 17 at 21:28
Any reason for not using just build variables for replacement?
– Camilo Terevinto
Nov 17 at 19:52
Any reason for not using just build variables for replacement?
– Camilo Terevinto
Nov 17 at 19:52
Can you not set the Environment Variable directly on the servers, that is the entire point of using Environment Variables to hold that information. Failing that see @CamiloTerevinto's comment as that would much easier.
– Adam Carr
Nov 17 at 21:28
Can you not set the Environment Variable directly on the servers, that is the entire point of using Environment Variables to hold that information. Failing that see @CamiloTerevinto's comment as that would much easier.
– Adam Carr
Nov 17 at 21:28
add a comment |
1 Answer
1
active
oldest
votes
up vote
2
down vote
accepted
I'm using standard web.config transformations (deploy to IIS)
Transformation web.staging.config:
<configuration xmlns:xdt="http://schemas.microsoft.com/XML-Document-Transform">
<location>
<system.webServer>
<aspNetCore>
<environmentVariables>
<environmentVariable xdt:Transform="Replace" xdt:Locator="Match(name)" name="ASPNETCORE_ENVIRONMENT" value="Staging" />
</environmentVariables>
</aspNetCore>
</system.webServer>
</location>
</configuration>
This does not answer the question.Appsettings.**json**
files are not XML.
– Daniel Mann
Nov 17 at 21:38
This might be useful. So do you add a web.config file to your .NET Core Project? Then do you include different environments as needed? If so this seems this might work for my case. Where do you set the EnvironmentName in Azure DevOps?
– Kyle Barnes
Nov 17 at 21:45
Yes, i have web.config, web.*.config and appsettings.json, appsettings.*.json included. Name of environment is "Stage name" in task..
– Štěpán Zechner
Nov 17 at 21:48
Not an ideal scenario, but this approach enabled me to deploy for separate environments. Hopefully, they have a better approach for .NET Core 2.2.
– Kyle Barnes
Nov 17 at 23:59
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
2
down vote
accepted
I'm using standard web.config transformations (deploy to IIS)
Transformation web.staging.config:
<configuration xmlns:xdt="http://schemas.microsoft.com/XML-Document-Transform">
<location>
<system.webServer>
<aspNetCore>
<environmentVariables>
<environmentVariable xdt:Transform="Replace" xdt:Locator="Match(name)" name="ASPNETCORE_ENVIRONMENT" value="Staging" />
</environmentVariables>
</aspNetCore>
</system.webServer>
</location>
</configuration>
This does not answer the question.Appsettings.**json**
files are not XML.
– Daniel Mann
Nov 17 at 21:38
This might be useful. So do you add a web.config file to your .NET Core Project? Then do you include different environments as needed? If so this seems this might work for my case. Where do you set the EnvironmentName in Azure DevOps?
– Kyle Barnes
Nov 17 at 21:45
Yes, i have web.config, web.*.config and appsettings.json, appsettings.*.json included. Name of environment is "Stage name" in task..
– Štěpán Zechner
Nov 17 at 21:48
Not an ideal scenario, but this approach enabled me to deploy for separate environments. Hopefully, they have a better approach for .NET Core 2.2.
– Kyle Barnes
Nov 17 at 23:59
add a comment |
up vote
2
down vote
accepted
I'm using standard web.config transformations (deploy to IIS)
Transformation web.staging.config:
<configuration xmlns:xdt="http://schemas.microsoft.com/XML-Document-Transform">
<location>
<system.webServer>
<aspNetCore>
<environmentVariables>
<environmentVariable xdt:Transform="Replace" xdt:Locator="Match(name)" name="ASPNETCORE_ENVIRONMENT" value="Staging" />
</environmentVariables>
</aspNetCore>
</system.webServer>
</location>
</configuration>
This does not answer the question.Appsettings.**json**
files are not XML.
– Daniel Mann
Nov 17 at 21:38
This might be useful. So do you add a web.config file to your .NET Core Project? Then do you include different environments as needed? If so this seems this might work for my case. Where do you set the EnvironmentName in Azure DevOps?
– Kyle Barnes
Nov 17 at 21:45
Yes, i have web.config, web.*.config and appsettings.json, appsettings.*.json included. Name of environment is "Stage name" in task..
– Štěpán Zechner
Nov 17 at 21:48
Not an ideal scenario, but this approach enabled me to deploy for separate environments. Hopefully, they have a better approach for .NET Core 2.2.
– Kyle Barnes
Nov 17 at 23:59
add a comment |
up vote
2
down vote
accepted
up vote
2
down vote
accepted
I'm using standard web.config transformations (deploy to IIS)
Transformation web.staging.config:
<configuration xmlns:xdt="http://schemas.microsoft.com/XML-Document-Transform">
<location>
<system.webServer>
<aspNetCore>
<environmentVariables>
<environmentVariable xdt:Transform="Replace" xdt:Locator="Match(name)" name="ASPNETCORE_ENVIRONMENT" value="Staging" />
</environmentVariables>
</aspNetCore>
</system.webServer>
</location>
</configuration>
I'm using standard web.config transformations (deploy to IIS)
Transformation web.staging.config:
<configuration xmlns:xdt="http://schemas.microsoft.com/XML-Document-Transform">
<location>
<system.webServer>
<aspNetCore>
<environmentVariables>
<environmentVariable xdt:Transform="Replace" xdt:Locator="Match(name)" name="ASPNETCORE_ENVIRONMENT" value="Staging" />
</environmentVariables>
</aspNetCore>
</system.webServer>
</location>
</configuration>
answered Nov 17 at 21:13
Štěpán Zechner
941
941
This does not answer the question.Appsettings.**json**
files are not XML.
– Daniel Mann
Nov 17 at 21:38
This might be useful. So do you add a web.config file to your .NET Core Project? Then do you include different environments as needed? If so this seems this might work for my case. Where do you set the EnvironmentName in Azure DevOps?
– Kyle Barnes
Nov 17 at 21:45
Yes, i have web.config, web.*.config and appsettings.json, appsettings.*.json included. Name of environment is "Stage name" in task..
– Štěpán Zechner
Nov 17 at 21:48
Not an ideal scenario, but this approach enabled me to deploy for separate environments. Hopefully, they have a better approach for .NET Core 2.2.
– Kyle Barnes
Nov 17 at 23:59
add a comment |
This does not answer the question.Appsettings.**json**
files are not XML.
– Daniel Mann
Nov 17 at 21:38
This might be useful. So do you add a web.config file to your .NET Core Project? Then do you include different environments as needed? If so this seems this might work for my case. Where do you set the EnvironmentName in Azure DevOps?
– Kyle Barnes
Nov 17 at 21:45
Yes, i have web.config, web.*.config and appsettings.json, appsettings.*.json included. Name of environment is "Stage name" in task..
– Štěpán Zechner
Nov 17 at 21:48
Not an ideal scenario, but this approach enabled me to deploy for separate environments. Hopefully, they have a better approach for .NET Core 2.2.
– Kyle Barnes
Nov 17 at 23:59
This does not answer the question.
Appsettings.**json**
files are not XML.– Daniel Mann
Nov 17 at 21:38
This does not answer the question.
Appsettings.**json**
files are not XML.– Daniel Mann
Nov 17 at 21:38
This might be useful. So do you add a web.config file to your .NET Core Project? Then do you include different environments as needed? If so this seems this might work for my case. Where do you set the EnvironmentName in Azure DevOps?
– Kyle Barnes
Nov 17 at 21:45
This might be useful. So do you add a web.config file to your .NET Core Project? Then do you include different environments as needed? If so this seems this might work for my case. Where do you set the EnvironmentName in Azure DevOps?
– Kyle Barnes
Nov 17 at 21:45
Yes, i have web.config, web.*.config and appsettings.json, appsettings.*.json included. Name of environment is "Stage name" in task..
– Štěpán Zechner
Nov 17 at 21:48
Yes, i have web.config, web.*.config and appsettings.json, appsettings.*.json included. Name of environment is "Stage name" in task..
– Štěpán Zechner
Nov 17 at 21:48
Not an ideal scenario, but this approach enabled me to deploy for separate environments. Hopefully, they have a better approach for .NET Core 2.2.
– Kyle Barnes
Nov 17 at 23:59
Not an ideal scenario, but this approach enabled me to deploy for separate environments. Hopefully, they have a better approach for .NET Core 2.2.
– Kyle Barnes
Nov 17 at 23:59
add a comment |
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53354920%2fazure-devops-net-core-build-include-web-config-file%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
JTL2M,RPn3yWK cX,LnjGzRR0fANGk2FKZBwS4mGr44 jd6dkw4Z66,PoG,1GO5NZJyu9JD9SnPXySqs8,wWq1Nzm3bAs,UM,0Y0,ie
Any reason for not using just build variables for replacement?
– Camilo Terevinto
Nov 17 at 19:52
Can you not set the Environment Variable directly on the servers, that is the entire point of using Environment Variables to hold that information. Failing that see @CamiloTerevinto's comment as that would much easier.
– Adam Carr
Nov 17 at 21:28