PHP - Optimise code that does a reconciliation between two arrays based on numerous fields
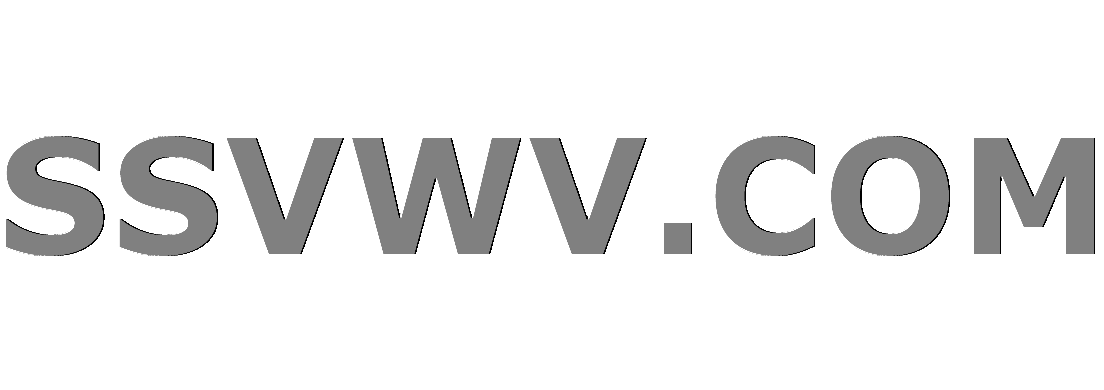
Multi tool use
up vote
0
down vote
favorite
I build two arrays (I can't use SQL for this as I am using ROQL qhich only allows for very basic SELECT statements as shown) from a SQL query.
I then want to see if any row matches in Array B based off X columns between Array A and Array B
So in the simplified example below, it does a "FULL" match if both the forename and surname exist in a row. It returns "SURNAME" match if just the surname, and "FORENAME" match if it is just the forename that exists.
My question is whether I am using the right functions - how could this be optimised? Returning a filtered array seems too much where I just want to see if that combination of values exists across multiple columns
CODE
$a = array();
$b = array();
RNCPHPConnectAPI::getCurrentContext()->ApplicationContext = "Get contacts";
$res = RNCPHPROQL::query("SELECT Contact.Name.First,Contact.Name.Last FROM CONTACT WHERE disabled=0 LIMIT 500")->next();
while($r = $res->next()) {
try {
$a_inner = array();
$a_inner["forename"] = $r['First'];
$a_inner["surname"] = $r['Last'];
$a = $a_inner;
}
catch ( Exception $err ){
echo "<p><b>Exception</b>: line ".__LINE__.": ".$err->getMessage()."</p>";
}
}
$res = RNCPHPROQL::query("SELECT Contact.Name.First,Contact.Name.Last FROM CONTACT WHERE disabled=1 LIMIT 20000")->next();
while($r = $res->next()) {
try {
$b_inner = array();
$b_inner["forename"] = $r['First'];
$b_inner["surname"] = $r['Last'];
$b = $b_inner;
}
catch ( Exception $err ){
echo "<p><b>Exception</b>: line ".__LINE__.": ".$err->getMessage()."</p>";
}
}
foreach ($a as $k => $v) {
$n = $a['forename'];
$e = $a['surname'];
$full_match = array_filter($b, function($val) use($n, $e){
return ($val['forename']==$n AND $val['surname']==$e);
});
echo $v['forename'] . ' ' . $v['surname'] . ' - ';
if (!empty($full_match)) {
echo 'FULL MATCH<br>';
//return false;
continue;
}
$surname_match = array_filter($b, function($val) use($e){
return ($val['surname']==$e);
});
if (!empty($surname_match)) {
echo 'SURNAME MATCH<br>';
//return false;
continue;
}
$forname_match = array_filter($b, function($val) use($n){
return ($val['forename']==$n);
});
if (!empty($forname_match)) {
echo 'FORENAME MATCH<br>';
//return false;
continue;
}
echo '<br>';
}
performance php array
add a comment |
up vote
0
down vote
favorite
I build two arrays (I can't use SQL for this as I am using ROQL qhich only allows for very basic SELECT statements as shown) from a SQL query.
I then want to see if any row matches in Array B based off X columns between Array A and Array B
So in the simplified example below, it does a "FULL" match if both the forename and surname exist in a row. It returns "SURNAME" match if just the surname, and "FORENAME" match if it is just the forename that exists.
My question is whether I am using the right functions - how could this be optimised? Returning a filtered array seems too much where I just want to see if that combination of values exists across multiple columns
CODE
$a = array();
$b = array();
RNCPHPConnectAPI::getCurrentContext()->ApplicationContext = "Get contacts";
$res = RNCPHPROQL::query("SELECT Contact.Name.First,Contact.Name.Last FROM CONTACT WHERE disabled=0 LIMIT 500")->next();
while($r = $res->next()) {
try {
$a_inner = array();
$a_inner["forename"] = $r['First'];
$a_inner["surname"] = $r['Last'];
$a = $a_inner;
}
catch ( Exception $err ){
echo "<p><b>Exception</b>: line ".__LINE__.": ".$err->getMessage()."</p>";
}
}
$res = RNCPHPROQL::query("SELECT Contact.Name.First,Contact.Name.Last FROM CONTACT WHERE disabled=1 LIMIT 20000")->next();
while($r = $res->next()) {
try {
$b_inner = array();
$b_inner["forename"] = $r['First'];
$b_inner["surname"] = $r['Last'];
$b = $b_inner;
}
catch ( Exception $err ){
echo "<p><b>Exception</b>: line ".__LINE__.": ".$err->getMessage()."</p>";
}
}
foreach ($a as $k => $v) {
$n = $a['forename'];
$e = $a['surname'];
$full_match = array_filter($b, function($val) use($n, $e){
return ($val['forename']==$n AND $val['surname']==$e);
});
echo $v['forename'] . ' ' . $v['surname'] . ' - ';
if (!empty($full_match)) {
echo 'FULL MATCH<br>';
//return false;
continue;
}
$surname_match = array_filter($b, function($val) use($e){
return ($val['surname']==$e);
});
if (!empty($surname_match)) {
echo 'SURNAME MATCH<br>';
//return false;
continue;
}
$forname_match = array_filter($b, function($val) use($n){
return ($val['forename']==$n);
});
if (!empty($forname_match)) {
echo 'FORENAME MATCH<br>';
//return false;
continue;
}
echo '<br>';
}
performance php array
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I build two arrays (I can't use SQL for this as I am using ROQL qhich only allows for very basic SELECT statements as shown) from a SQL query.
I then want to see if any row matches in Array B based off X columns between Array A and Array B
So in the simplified example below, it does a "FULL" match if both the forename and surname exist in a row. It returns "SURNAME" match if just the surname, and "FORENAME" match if it is just the forename that exists.
My question is whether I am using the right functions - how could this be optimised? Returning a filtered array seems too much where I just want to see if that combination of values exists across multiple columns
CODE
$a = array();
$b = array();
RNCPHPConnectAPI::getCurrentContext()->ApplicationContext = "Get contacts";
$res = RNCPHPROQL::query("SELECT Contact.Name.First,Contact.Name.Last FROM CONTACT WHERE disabled=0 LIMIT 500")->next();
while($r = $res->next()) {
try {
$a_inner = array();
$a_inner["forename"] = $r['First'];
$a_inner["surname"] = $r['Last'];
$a = $a_inner;
}
catch ( Exception $err ){
echo "<p><b>Exception</b>: line ".__LINE__.": ".$err->getMessage()."</p>";
}
}
$res = RNCPHPROQL::query("SELECT Contact.Name.First,Contact.Name.Last FROM CONTACT WHERE disabled=1 LIMIT 20000")->next();
while($r = $res->next()) {
try {
$b_inner = array();
$b_inner["forename"] = $r['First'];
$b_inner["surname"] = $r['Last'];
$b = $b_inner;
}
catch ( Exception $err ){
echo "<p><b>Exception</b>: line ".__LINE__.": ".$err->getMessage()."</p>";
}
}
foreach ($a as $k => $v) {
$n = $a['forename'];
$e = $a['surname'];
$full_match = array_filter($b, function($val) use($n, $e){
return ($val['forename']==$n AND $val['surname']==$e);
});
echo $v['forename'] . ' ' . $v['surname'] . ' - ';
if (!empty($full_match)) {
echo 'FULL MATCH<br>';
//return false;
continue;
}
$surname_match = array_filter($b, function($val) use($e){
return ($val['surname']==$e);
});
if (!empty($surname_match)) {
echo 'SURNAME MATCH<br>';
//return false;
continue;
}
$forname_match = array_filter($b, function($val) use($n){
return ($val['forename']==$n);
});
if (!empty($forname_match)) {
echo 'FORENAME MATCH<br>';
//return false;
continue;
}
echo '<br>';
}
performance php array
I build two arrays (I can't use SQL for this as I am using ROQL qhich only allows for very basic SELECT statements as shown) from a SQL query.
I then want to see if any row matches in Array B based off X columns between Array A and Array B
So in the simplified example below, it does a "FULL" match if both the forename and surname exist in a row. It returns "SURNAME" match if just the surname, and "FORENAME" match if it is just the forename that exists.
My question is whether I am using the right functions - how could this be optimised? Returning a filtered array seems too much where I just want to see if that combination of values exists across multiple columns
CODE
$a = array();
$b = array();
RNCPHPConnectAPI::getCurrentContext()->ApplicationContext = "Get contacts";
$res = RNCPHPROQL::query("SELECT Contact.Name.First,Contact.Name.Last FROM CONTACT WHERE disabled=0 LIMIT 500")->next();
while($r = $res->next()) {
try {
$a_inner = array();
$a_inner["forename"] = $r['First'];
$a_inner["surname"] = $r['Last'];
$a = $a_inner;
}
catch ( Exception $err ){
echo "<p><b>Exception</b>: line ".__LINE__.": ".$err->getMessage()."</p>";
}
}
$res = RNCPHPROQL::query("SELECT Contact.Name.First,Contact.Name.Last FROM CONTACT WHERE disabled=1 LIMIT 20000")->next();
while($r = $res->next()) {
try {
$b_inner = array();
$b_inner["forename"] = $r['First'];
$b_inner["surname"] = $r['Last'];
$b = $b_inner;
}
catch ( Exception $err ){
echo "<p><b>Exception</b>: line ".__LINE__.": ".$err->getMessage()."</p>";
}
}
foreach ($a as $k => $v) {
$n = $a['forename'];
$e = $a['surname'];
$full_match = array_filter($b, function($val) use($n, $e){
return ($val['forename']==$n AND $val['surname']==$e);
});
echo $v['forename'] . ' ' . $v['surname'] . ' - ';
if (!empty($full_match)) {
echo 'FULL MATCH<br>';
//return false;
continue;
}
$surname_match = array_filter($b, function($val) use($e){
return ($val['surname']==$e);
});
if (!empty($surname_match)) {
echo 'SURNAME MATCH<br>';
//return false;
continue;
}
$forname_match = array_filter($b, function($val) use($n){
return ($val['forename']==$n);
});
if (!empty($forname_match)) {
echo 'FORENAME MATCH<br>';
//return false;
continue;
}
echo '<br>';
}
performance php array
performance php array
asked 21 hours ago
pee2pee
19810
19810
add a comment |
add a comment |
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodereview.stackexchange.com%2fquestions%2f208118%2fphp-optimise-code-that-does-a-reconciliation-between-two-arrays-based-on-numer%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
s7IXmOtWxY 6Fq