Unit test for a method that populates a dictionary from an API call
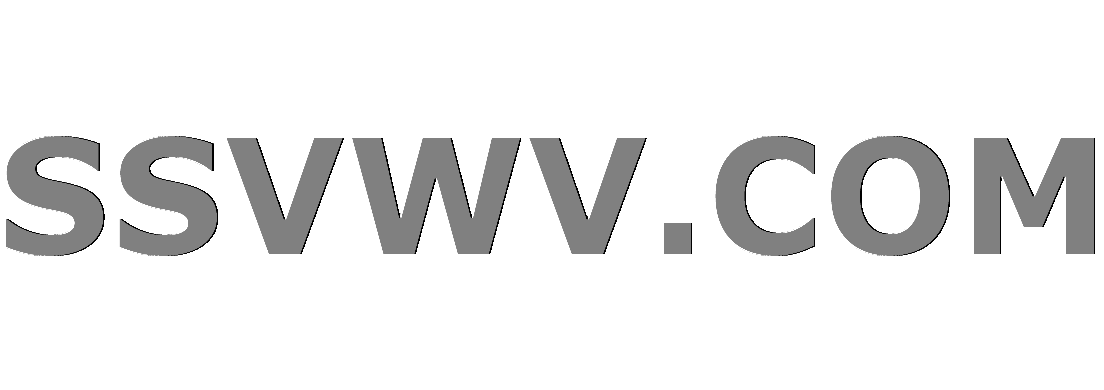
Multi tool use
up vote
2
down vote
favorite
I am building a unit test for a method that populates a dictionary from an API call (responding with JSON):
<TestMethod()> Public Sub GetListOfBadKPI_EmptyResponse_ReturnsEmptyDict()
'Arrange
Const scheduleName As String = "testSchedule"
Const emptyResponseJSON As String = "{""data"":[{""r"":""N""}]}"
Dim fakeResult As Data = JsonConvert.DeserializeObject(Of Data)(emptyResponseJSON)
Dim fakeServer As MockServer = New MockServer With {.ReturnData = fakeResult}
Dim CalculationSchedule As CalculationSchedule = New CalculationSchedule(scheduleName, fakeServer)
'Act
Dim resultDictionary As Dictionary(Of String, Integer) =
CalculationSchedule.GetDictionaryOfBadCalculation()
'Assert
Assert.IsTrue(resultDictionary.Count = 0)
End Sub
The fake server is used to return any data I need for the test, without actually connecting with the API:
Public Class MockServer
Implements IServer
Public Property ReturnData As Data
Public Function ExecuteQuery(query As String, Optional server As String = Nothing) As Data Implements IServer.ExecuteSQLQuery
Return ReturnData
End Function
End Class
This setup allows me to simulate API responses to test different cases.
However, I am not happy with the way it is done, mainly since JSON strings have a lot of quotes "
and dealing with those in VB.NET is daunting, since you have to double them up, it makes copy-pasting difficult, and so on.
Moreover, it does not feel very 'professional' to do it like this.
How could I improve this unit test?
unit-testing vb.net
bumped to the homepage by Community♦ 4 mins ago
This question has answers that may be good or bad; the system has marked it active so that they can be reviewed.
add a comment |
up vote
2
down vote
favorite
I am building a unit test for a method that populates a dictionary from an API call (responding with JSON):
<TestMethod()> Public Sub GetListOfBadKPI_EmptyResponse_ReturnsEmptyDict()
'Arrange
Const scheduleName As String = "testSchedule"
Const emptyResponseJSON As String = "{""data"":[{""r"":""N""}]}"
Dim fakeResult As Data = JsonConvert.DeserializeObject(Of Data)(emptyResponseJSON)
Dim fakeServer As MockServer = New MockServer With {.ReturnData = fakeResult}
Dim CalculationSchedule As CalculationSchedule = New CalculationSchedule(scheduleName, fakeServer)
'Act
Dim resultDictionary As Dictionary(Of String, Integer) =
CalculationSchedule.GetDictionaryOfBadCalculation()
'Assert
Assert.IsTrue(resultDictionary.Count = 0)
End Sub
The fake server is used to return any data I need for the test, without actually connecting with the API:
Public Class MockServer
Implements IServer
Public Property ReturnData As Data
Public Function ExecuteQuery(query As String, Optional server As String = Nothing) As Data Implements IServer.ExecuteSQLQuery
Return ReturnData
End Function
End Class
This setup allows me to simulate API responses to test different cases.
However, I am not happy with the way it is done, mainly since JSON strings have a lot of quotes "
and dealing with those in VB.NET is daunting, since you have to double them up, it makes copy-pasting difficult, and so on.
Moreover, it does not feel very 'professional' to do it like this.
How could I improve this unit test?
unit-testing vb.net
bumped to the homepage by Community♦ 4 mins ago
This question has answers that may be good or bad; the system has marked it active so that they can be reviewed.
I'm curious if you have a database you can use for testing where you can store the fake data in a temporary (or testing) table? Perhaps even creating a "dummy" record in the production database with a specific identifier that would normally be ignored in normal operation? Either that or even a simple text file you can have your application read? In my environment, I have a completely separate (PostgreSQL) database running on a different server that is dedicated for testing so that any changes I make during my tests do not affect production data, but I can fully test all aspects of my code.
– G_Hosa_Phat
Jan 30 at 16:03
1
I guess I could. However, I was under the impression that I should avoid unit tests requiring connection to the DB, as that would turn them into integration test, and that's bad. Let's imagine the DB connection breaks. It would make this test break as well, even if the code it's supposed to test is perfectly fine.
– Maxime
Jan 31 at 9:04
I understand that concern. I'm not a QA tester - I'm completely self-taught when it comes to software design and development, as well as database administration - so I'm just working from my own experience. That being said, my first thought on the matter is, if you've fully tested the basic database connectivity and communication from your application, this type of test is merely an extension of that - similar to reading a text file or some other basic operation - and would be preferred to hard-coding test data into the application. (cont.)
– G_Hosa_Phat
Jan 31 at 14:46
(cont.) But I'm not sure if this violates best practices, which is why this is a comment and not an answer. As I understand it, though, your primary purpose for this testing is to ensure the correct behavior when parsing the JSON data. As I mentioned in my first comment, though, you could try simply putting the JSON data into a plain text file stored locally on your computer (or on a network share) and have your test process it that way.
– G_Hosa_Phat
Jan 31 at 14:50
One other thing: I suppose it would also matter to some extent the API's purpose and function. If the API connects to a Web resource, then I don't believe that your connecting to an internal database (the connection to which you've already tested) would qualify as "integration" testing. Of course, if the API's function is to connect to the same database in which you place the test data, then that's a different matter.
– G_Hosa_Phat
Jan 31 at 14:54
add a comment |
up vote
2
down vote
favorite
up vote
2
down vote
favorite
I am building a unit test for a method that populates a dictionary from an API call (responding with JSON):
<TestMethod()> Public Sub GetListOfBadKPI_EmptyResponse_ReturnsEmptyDict()
'Arrange
Const scheduleName As String = "testSchedule"
Const emptyResponseJSON As String = "{""data"":[{""r"":""N""}]}"
Dim fakeResult As Data = JsonConvert.DeserializeObject(Of Data)(emptyResponseJSON)
Dim fakeServer As MockServer = New MockServer With {.ReturnData = fakeResult}
Dim CalculationSchedule As CalculationSchedule = New CalculationSchedule(scheduleName, fakeServer)
'Act
Dim resultDictionary As Dictionary(Of String, Integer) =
CalculationSchedule.GetDictionaryOfBadCalculation()
'Assert
Assert.IsTrue(resultDictionary.Count = 0)
End Sub
The fake server is used to return any data I need for the test, without actually connecting with the API:
Public Class MockServer
Implements IServer
Public Property ReturnData As Data
Public Function ExecuteQuery(query As String, Optional server As String = Nothing) As Data Implements IServer.ExecuteSQLQuery
Return ReturnData
End Function
End Class
This setup allows me to simulate API responses to test different cases.
However, I am not happy with the way it is done, mainly since JSON strings have a lot of quotes "
and dealing with those in VB.NET is daunting, since you have to double them up, it makes copy-pasting difficult, and so on.
Moreover, it does not feel very 'professional' to do it like this.
How could I improve this unit test?
unit-testing vb.net
I am building a unit test for a method that populates a dictionary from an API call (responding with JSON):
<TestMethod()> Public Sub GetListOfBadKPI_EmptyResponse_ReturnsEmptyDict()
'Arrange
Const scheduleName As String = "testSchedule"
Const emptyResponseJSON As String = "{""data"":[{""r"":""N""}]}"
Dim fakeResult As Data = JsonConvert.DeserializeObject(Of Data)(emptyResponseJSON)
Dim fakeServer As MockServer = New MockServer With {.ReturnData = fakeResult}
Dim CalculationSchedule As CalculationSchedule = New CalculationSchedule(scheduleName, fakeServer)
'Act
Dim resultDictionary As Dictionary(Of String, Integer) =
CalculationSchedule.GetDictionaryOfBadCalculation()
'Assert
Assert.IsTrue(resultDictionary.Count = 0)
End Sub
The fake server is used to return any data I need for the test, without actually connecting with the API:
Public Class MockServer
Implements IServer
Public Property ReturnData As Data
Public Function ExecuteQuery(query As String, Optional server As String = Nothing) As Data Implements IServer.ExecuteSQLQuery
Return ReturnData
End Function
End Class
This setup allows me to simulate API responses to test different cases.
However, I am not happy with the way it is done, mainly since JSON strings have a lot of quotes "
and dealing with those in VB.NET is daunting, since you have to double them up, it makes copy-pasting difficult, and so on.
Moreover, it does not feel very 'professional' to do it like this.
How could I improve this unit test?
unit-testing vb.net
unit-testing vb.net
edited Jun 2 at 4:02


Jamal♦
30.2k11115226
30.2k11115226
asked Jan 3 at 14:10
Maxime
211111
211111
bumped to the homepage by Community♦ 4 mins ago
This question has answers that may be good or bad; the system has marked it active so that they can be reviewed.
bumped to the homepage by Community♦ 4 mins ago
This question has answers that may be good or bad; the system has marked it active so that they can be reviewed.
I'm curious if you have a database you can use for testing where you can store the fake data in a temporary (or testing) table? Perhaps even creating a "dummy" record in the production database with a specific identifier that would normally be ignored in normal operation? Either that or even a simple text file you can have your application read? In my environment, I have a completely separate (PostgreSQL) database running on a different server that is dedicated for testing so that any changes I make during my tests do not affect production data, but I can fully test all aspects of my code.
– G_Hosa_Phat
Jan 30 at 16:03
1
I guess I could. However, I was under the impression that I should avoid unit tests requiring connection to the DB, as that would turn them into integration test, and that's bad. Let's imagine the DB connection breaks. It would make this test break as well, even if the code it's supposed to test is perfectly fine.
– Maxime
Jan 31 at 9:04
I understand that concern. I'm not a QA tester - I'm completely self-taught when it comes to software design and development, as well as database administration - so I'm just working from my own experience. That being said, my first thought on the matter is, if you've fully tested the basic database connectivity and communication from your application, this type of test is merely an extension of that - similar to reading a text file or some other basic operation - and would be preferred to hard-coding test data into the application. (cont.)
– G_Hosa_Phat
Jan 31 at 14:46
(cont.) But I'm not sure if this violates best practices, which is why this is a comment and not an answer. As I understand it, though, your primary purpose for this testing is to ensure the correct behavior when parsing the JSON data. As I mentioned in my first comment, though, you could try simply putting the JSON data into a plain text file stored locally on your computer (or on a network share) and have your test process it that way.
– G_Hosa_Phat
Jan 31 at 14:50
One other thing: I suppose it would also matter to some extent the API's purpose and function. If the API connects to a Web resource, then I don't believe that your connecting to an internal database (the connection to which you've already tested) would qualify as "integration" testing. Of course, if the API's function is to connect to the same database in which you place the test data, then that's a different matter.
– G_Hosa_Phat
Jan 31 at 14:54
add a comment |
I'm curious if you have a database you can use for testing where you can store the fake data in a temporary (or testing) table? Perhaps even creating a "dummy" record in the production database with a specific identifier that would normally be ignored in normal operation? Either that or even a simple text file you can have your application read? In my environment, I have a completely separate (PostgreSQL) database running on a different server that is dedicated for testing so that any changes I make during my tests do not affect production data, but I can fully test all aspects of my code.
– G_Hosa_Phat
Jan 30 at 16:03
1
I guess I could. However, I was under the impression that I should avoid unit tests requiring connection to the DB, as that would turn them into integration test, and that's bad. Let's imagine the DB connection breaks. It would make this test break as well, even if the code it's supposed to test is perfectly fine.
– Maxime
Jan 31 at 9:04
I understand that concern. I'm not a QA tester - I'm completely self-taught when it comes to software design and development, as well as database administration - so I'm just working from my own experience. That being said, my first thought on the matter is, if you've fully tested the basic database connectivity and communication from your application, this type of test is merely an extension of that - similar to reading a text file or some other basic operation - and would be preferred to hard-coding test data into the application. (cont.)
– G_Hosa_Phat
Jan 31 at 14:46
(cont.) But I'm not sure if this violates best practices, which is why this is a comment and not an answer. As I understand it, though, your primary purpose for this testing is to ensure the correct behavior when parsing the JSON data. As I mentioned in my first comment, though, you could try simply putting the JSON data into a plain text file stored locally on your computer (or on a network share) and have your test process it that way.
– G_Hosa_Phat
Jan 31 at 14:50
One other thing: I suppose it would also matter to some extent the API's purpose and function. If the API connects to a Web resource, then I don't believe that your connecting to an internal database (the connection to which you've already tested) would qualify as "integration" testing. Of course, if the API's function is to connect to the same database in which you place the test data, then that's a different matter.
– G_Hosa_Phat
Jan 31 at 14:54
I'm curious if you have a database you can use for testing where you can store the fake data in a temporary (or testing) table? Perhaps even creating a "dummy" record in the production database with a specific identifier that would normally be ignored in normal operation? Either that or even a simple text file you can have your application read? In my environment, I have a completely separate (PostgreSQL) database running on a different server that is dedicated for testing so that any changes I make during my tests do not affect production data, but I can fully test all aspects of my code.
– G_Hosa_Phat
Jan 30 at 16:03
I'm curious if you have a database you can use for testing where you can store the fake data in a temporary (or testing) table? Perhaps even creating a "dummy" record in the production database with a specific identifier that would normally be ignored in normal operation? Either that or even a simple text file you can have your application read? In my environment, I have a completely separate (PostgreSQL) database running on a different server that is dedicated for testing so that any changes I make during my tests do not affect production data, but I can fully test all aspects of my code.
– G_Hosa_Phat
Jan 30 at 16:03
1
1
I guess I could. However, I was under the impression that I should avoid unit tests requiring connection to the DB, as that would turn them into integration test, and that's bad. Let's imagine the DB connection breaks. It would make this test break as well, even if the code it's supposed to test is perfectly fine.
– Maxime
Jan 31 at 9:04
I guess I could. However, I was under the impression that I should avoid unit tests requiring connection to the DB, as that would turn them into integration test, and that's bad. Let's imagine the DB connection breaks. It would make this test break as well, even if the code it's supposed to test is perfectly fine.
– Maxime
Jan 31 at 9:04
I understand that concern. I'm not a QA tester - I'm completely self-taught when it comes to software design and development, as well as database administration - so I'm just working from my own experience. That being said, my first thought on the matter is, if you've fully tested the basic database connectivity and communication from your application, this type of test is merely an extension of that - similar to reading a text file or some other basic operation - and would be preferred to hard-coding test data into the application. (cont.)
– G_Hosa_Phat
Jan 31 at 14:46
I understand that concern. I'm not a QA tester - I'm completely self-taught when it comes to software design and development, as well as database administration - so I'm just working from my own experience. That being said, my first thought on the matter is, if you've fully tested the basic database connectivity and communication from your application, this type of test is merely an extension of that - similar to reading a text file or some other basic operation - and would be preferred to hard-coding test data into the application. (cont.)
– G_Hosa_Phat
Jan 31 at 14:46
(cont.) But I'm not sure if this violates best practices, which is why this is a comment and not an answer. As I understand it, though, your primary purpose for this testing is to ensure the correct behavior when parsing the JSON data. As I mentioned in my first comment, though, you could try simply putting the JSON data into a plain text file stored locally on your computer (or on a network share) and have your test process it that way.
– G_Hosa_Phat
Jan 31 at 14:50
(cont.) But I'm not sure if this violates best practices, which is why this is a comment and not an answer. As I understand it, though, your primary purpose for this testing is to ensure the correct behavior when parsing the JSON data. As I mentioned in my first comment, though, you could try simply putting the JSON data into a plain text file stored locally on your computer (or on a network share) and have your test process it that way.
– G_Hosa_Phat
Jan 31 at 14:50
One other thing: I suppose it would also matter to some extent the API's purpose and function. If the API connects to a Web resource, then I don't believe that your connecting to an internal database (the connection to which you've already tested) would qualify as "integration" testing. Of course, if the API's function is to connect to the same database in which you place the test data, then that's a different matter.
– G_Hosa_Phat
Jan 31 at 14:54
One other thing: I suppose it would also matter to some extent the API's purpose and function. If the API connects to a Web resource, then I don't believe that your connecting to an internal database (the connection to which you've already tested) would qualify as "integration" testing. Of course, if the API's function is to connect to the same database in which you place the test data, then that's a different matter.
– G_Hosa_Phat
Jan 31 at 14:54
add a comment |
1 Answer
1
active
oldest
votes
up vote
0
down vote
There is a better way than using a string, there is a Namespace that you can import called Json or System.Json
after you have imported this you have access to JsonValue Arrays which allows you to nest JavaScript Objects creating a JSON Document.
it actually looks like you are already using the Namespace to deserialize the string into an object, not sure why you aren't just creating the JSON Objects themselves. they are just collections of KeyValuePair
s of Of String, JsonValue
(Dictionaries, Collections, or Enumerables)
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
There is a better way than using a string, there is a Namespace that you can import called Json or System.Json
after you have imported this you have access to JsonValue Arrays which allows you to nest JavaScript Objects creating a JSON Document.
it actually looks like you are already using the Namespace to deserialize the string into an object, not sure why you aren't just creating the JSON Objects themselves. they are just collections of KeyValuePair
s of Of String, JsonValue
(Dictionaries, Collections, or Enumerables)
add a comment |
up vote
0
down vote
There is a better way than using a string, there is a Namespace that you can import called Json or System.Json
after you have imported this you have access to JsonValue Arrays which allows you to nest JavaScript Objects creating a JSON Document.
it actually looks like you are already using the Namespace to deserialize the string into an object, not sure why you aren't just creating the JSON Objects themselves. they are just collections of KeyValuePair
s of Of String, JsonValue
(Dictionaries, Collections, or Enumerables)
add a comment |
up vote
0
down vote
up vote
0
down vote
There is a better way than using a string, there is a Namespace that you can import called Json or System.Json
after you have imported this you have access to JsonValue Arrays which allows you to nest JavaScript Objects creating a JSON Document.
it actually looks like you are already using the Namespace to deserialize the string into an object, not sure why you aren't just creating the JSON Objects themselves. they are just collections of KeyValuePair
s of Of String, JsonValue
(Dictionaries, Collections, or Enumerables)
There is a better way than using a string, there is a Namespace that you can import called Json or System.Json
after you have imported this you have access to JsonValue Arrays which allows you to nest JavaScript Objects creating a JSON Document.
it actually looks like you are already using the Namespace to deserialize the string into an object, not sure why you aren't just creating the JSON Objects themselves. they are just collections of KeyValuePair
s of Of String, JsonValue
(Dictionaries, Collections, or Enumerables)
answered Apr 2 at 17:17


Malachi♦
25.5k772175
25.5k772175
add a comment |
add a comment |
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodereview.stackexchange.com%2fquestions%2f184174%2funit-test-for-a-method-that-populates-a-dictionary-from-an-api-call%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
oTihi40j48yVV2b,grP0Z9 RYoFfPsz0r1TPCrDBlDBW7wXAcav
I'm curious if you have a database you can use for testing where you can store the fake data in a temporary (or testing) table? Perhaps even creating a "dummy" record in the production database with a specific identifier that would normally be ignored in normal operation? Either that or even a simple text file you can have your application read? In my environment, I have a completely separate (PostgreSQL) database running on a different server that is dedicated for testing so that any changes I make during my tests do not affect production data, but I can fully test all aspects of my code.
– G_Hosa_Phat
Jan 30 at 16:03
1
I guess I could. However, I was under the impression that I should avoid unit tests requiring connection to the DB, as that would turn them into integration test, and that's bad. Let's imagine the DB connection breaks. It would make this test break as well, even if the code it's supposed to test is perfectly fine.
– Maxime
Jan 31 at 9:04
I understand that concern. I'm not a QA tester - I'm completely self-taught when it comes to software design and development, as well as database administration - so I'm just working from my own experience. That being said, my first thought on the matter is, if you've fully tested the basic database connectivity and communication from your application, this type of test is merely an extension of that - similar to reading a text file or some other basic operation - and would be preferred to hard-coding test data into the application. (cont.)
– G_Hosa_Phat
Jan 31 at 14:46
(cont.) But I'm not sure if this violates best practices, which is why this is a comment and not an answer. As I understand it, though, your primary purpose for this testing is to ensure the correct behavior when parsing the JSON data. As I mentioned in my first comment, though, you could try simply putting the JSON data into a plain text file stored locally on your computer (or on a network share) and have your test process it that way.
– G_Hosa_Phat
Jan 31 at 14:50
One other thing: I suppose it would also matter to some extent the API's purpose and function. If the API connects to a Web resource, then I don't believe that your connecting to an internal database (the connection to which you've already tested) would qualify as "integration" testing. Of course, if the API's function is to connect to the same database in which you place the test data, then that's a different matter.
– G_Hosa_Phat
Jan 31 at 14:54