JAVA: Method that sums the areas of all rooms on the same floor
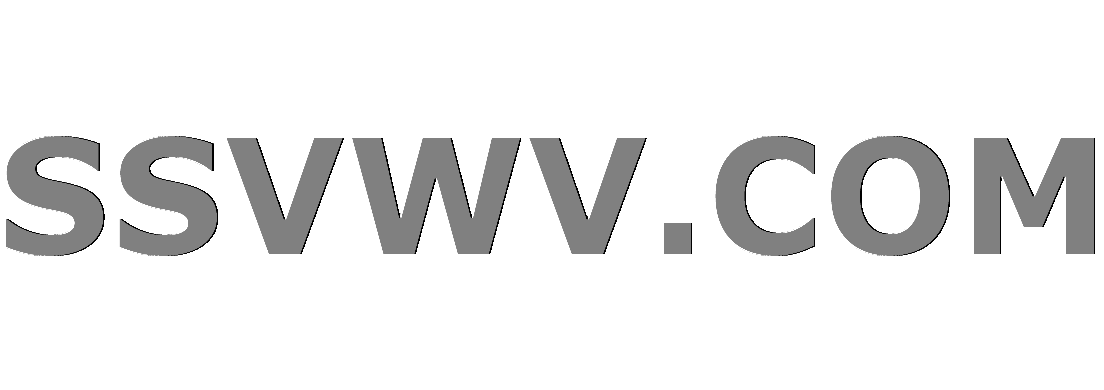
Multi tool use
I have two classes, the first is this:
public class Room {
public String typeOfRoom;
public double area;
public int floor;
public int windowsCount;
public String getTypeOfRoom() {
return typeOfRoom;
}
public double getArea() {
return area;
}
public int getFloor() {
return floor;
}
public int getWindowsCount() {
return windowsCount;
}
public void setTypeOfRoom(String typeOfRoom) {
this.typeOfRoom = typeOfRoom;
}
public void setArea(double area) {
this.area = area;
}
public void setFloor(int floor) {
this.floor = floor;
}
public void setWindowsCount(int windowsCount) {
this.windowsCount = windowsCount;
}
}
And the second is this:
public class House {
public Address addressOfHouse;
public Room bedroom;
public Room childrenroom;
public Room kitchen;
public Room livingroom;
public Room lobby;
public Room workroom;
public House (){
addressOfHouse=new Address();
bedroom=new Room();
childrenroom=new Room();
kitchen=new Room();
livingroom=new Room();
lobby=new Room();
workroom=new Room();
}
public double totalArea (int floor){
double totalArea=1;
return totalArea;
}
public double totalArea (){
//Here I do not know how to keep on...
}
}
My problem is that I have to complete the method "public double totalArea(){...}" and I am not sure about how to do it. This method should add all the areas of the rooms that are on the first floor of a house. I do not know exactly how to express it in the method, I do not know what kind of command I should use, I have thought of "if" or "for", but my biggest trouble is about how to express that the total area is the sum of the areas of the rooms on the first floor, if the area has to be set in the Main class and I do not know how to declare the "sum of the areas" in the method... Could you help me with that? If you could just give me some direction, just tell what steps I should follow, send links or something, I would be very glad. I have already looked for the solution of it for two weeks... I hope you have comprehended me. Thank you very much!
java
add a comment |
I have two classes, the first is this:
public class Room {
public String typeOfRoom;
public double area;
public int floor;
public int windowsCount;
public String getTypeOfRoom() {
return typeOfRoom;
}
public double getArea() {
return area;
}
public int getFloor() {
return floor;
}
public int getWindowsCount() {
return windowsCount;
}
public void setTypeOfRoom(String typeOfRoom) {
this.typeOfRoom = typeOfRoom;
}
public void setArea(double area) {
this.area = area;
}
public void setFloor(int floor) {
this.floor = floor;
}
public void setWindowsCount(int windowsCount) {
this.windowsCount = windowsCount;
}
}
And the second is this:
public class House {
public Address addressOfHouse;
public Room bedroom;
public Room childrenroom;
public Room kitchen;
public Room livingroom;
public Room lobby;
public Room workroom;
public House (){
addressOfHouse=new Address();
bedroom=new Room();
childrenroom=new Room();
kitchen=new Room();
livingroom=new Room();
lobby=new Room();
workroom=new Room();
}
public double totalArea (int floor){
double totalArea=1;
return totalArea;
}
public double totalArea (){
//Here I do not know how to keep on...
}
}
My problem is that I have to complete the method "public double totalArea(){...}" and I am not sure about how to do it. This method should add all the areas of the rooms that are on the first floor of a house. I do not know exactly how to express it in the method, I do not know what kind of command I should use, I have thought of "if" or "for", but my biggest trouble is about how to express that the total area is the sum of the areas of the rooms on the first floor, if the area has to be set in the Main class and I do not know how to declare the "sum of the areas" in the method... Could you help me with that? If you could just give me some direction, just tell what steps I should follow, send links or something, I would be very glad. I have already looked for the solution of it for two weeks... I hope you have comprehended me. Thank you very much!
java
Hello, I would suggest adding a constructor to Room class that would allow you to specify the floor and the area of the room i.e. public Room(int floor, double area) link to read what is a constructor tutorialspoint.com/java/java_constructors.htm . Secondly in your not sure what is totalArea(int floor) is meant to do. For totalArea() as you said you are trying to calculcate area of all rooms in floor. So you could check room floor i.e. beedroom.getFloor() == 1 link about if statement tutorialspoint.com/java/if_statement_in_java.htm and adding rooms area to a counter
– DonatasD
Nov 20 at 17:41
Can you work out how to do it with pen and paper? If so, do that and then try writing the code. Side note: yourtotalArea (int floor)
is pointless as it just returns1
every time.
– achAmháin
Nov 20 at 17:43
Thank you, @DonatasD, for the answer (suggestions and links), it was indeed useful! Thank you for the suggestion, @achAmháin! I suspected that I had made some mistake in the method "totalArea (int floor)".
– IHSV555
Nov 20 at 18:11
add a comment |
I have two classes, the first is this:
public class Room {
public String typeOfRoom;
public double area;
public int floor;
public int windowsCount;
public String getTypeOfRoom() {
return typeOfRoom;
}
public double getArea() {
return area;
}
public int getFloor() {
return floor;
}
public int getWindowsCount() {
return windowsCount;
}
public void setTypeOfRoom(String typeOfRoom) {
this.typeOfRoom = typeOfRoom;
}
public void setArea(double area) {
this.area = area;
}
public void setFloor(int floor) {
this.floor = floor;
}
public void setWindowsCount(int windowsCount) {
this.windowsCount = windowsCount;
}
}
And the second is this:
public class House {
public Address addressOfHouse;
public Room bedroom;
public Room childrenroom;
public Room kitchen;
public Room livingroom;
public Room lobby;
public Room workroom;
public House (){
addressOfHouse=new Address();
bedroom=new Room();
childrenroom=new Room();
kitchen=new Room();
livingroom=new Room();
lobby=new Room();
workroom=new Room();
}
public double totalArea (int floor){
double totalArea=1;
return totalArea;
}
public double totalArea (){
//Here I do not know how to keep on...
}
}
My problem is that I have to complete the method "public double totalArea(){...}" and I am not sure about how to do it. This method should add all the areas of the rooms that are on the first floor of a house. I do not know exactly how to express it in the method, I do not know what kind of command I should use, I have thought of "if" or "for", but my biggest trouble is about how to express that the total area is the sum of the areas of the rooms on the first floor, if the area has to be set in the Main class and I do not know how to declare the "sum of the areas" in the method... Could you help me with that? If you could just give me some direction, just tell what steps I should follow, send links or something, I would be very glad. I have already looked for the solution of it for two weeks... I hope you have comprehended me. Thank you very much!
java
I have two classes, the first is this:
public class Room {
public String typeOfRoom;
public double area;
public int floor;
public int windowsCount;
public String getTypeOfRoom() {
return typeOfRoom;
}
public double getArea() {
return area;
}
public int getFloor() {
return floor;
}
public int getWindowsCount() {
return windowsCount;
}
public void setTypeOfRoom(String typeOfRoom) {
this.typeOfRoom = typeOfRoom;
}
public void setArea(double area) {
this.area = area;
}
public void setFloor(int floor) {
this.floor = floor;
}
public void setWindowsCount(int windowsCount) {
this.windowsCount = windowsCount;
}
}
And the second is this:
public class House {
public Address addressOfHouse;
public Room bedroom;
public Room childrenroom;
public Room kitchen;
public Room livingroom;
public Room lobby;
public Room workroom;
public House (){
addressOfHouse=new Address();
bedroom=new Room();
childrenroom=new Room();
kitchen=new Room();
livingroom=new Room();
lobby=new Room();
workroom=new Room();
}
public double totalArea (int floor){
double totalArea=1;
return totalArea;
}
public double totalArea (){
//Here I do not know how to keep on...
}
}
My problem is that I have to complete the method "public double totalArea(){...}" and I am not sure about how to do it. This method should add all the areas of the rooms that are on the first floor of a house. I do not know exactly how to express it in the method, I do not know what kind of command I should use, I have thought of "if" or "for", but my biggest trouble is about how to express that the total area is the sum of the areas of the rooms on the first floor, if the area has to be set in the Main class and I do not know how to declare the "sum of the areas" in the method... Could you help me with that? If you could just give me some direction, just tell what steps I should follow, send links or something, I would be very glad. I have already looked for the solution of it for two weeks... I hope you have comprehended me. Thank you very much!
java
java
asked Nov 20 at 17:26
IHSV555
154
154
Hello, I would suggest adding a constructor to Room class that would allow you to specify the floor and the area of the room i.e. public Room(int floor, double area) link to read what is a constructor tutorialspoint.com/java/java_constructors.htm . Secondly in your not sure what is totalArea(int floor) is meant to do. For totalArea() as you said you are trying to calculcate area of all rooms in floor. So you could check room floor i.e. beedroom.getFloor() == 1 link about if statement tutorialspoint.com/java/if_statement_in_java.htm and adding rooms area to a counter
– DonatasD
Nov 20 at 17:41
Can you work out how to do it with pen and paper? If so, do that and then try writing the code. Side note: yourtotalArea (int floor)
is pointless as it just returns1
every time.
– achAmháin
Nov 20 at 17:43
Thank you, @DonatasD, for the answer (suggestions and links), it was indeed useful! Thank you for the suggestion, @achAmháin! I suspected that I had made some mistake in the method "totalArea (int floor)".
– IHSV555
Nov 20 at 18:11
add a comment |
Hello, I would suggest adding a constructor to Room class that would allow you to specify the floor and the area of the room i.e. public Room(int floor, double area) link to read what is a constructor tutorialspoint.com/java/java_constructors.htm . Secondly in your not sure what is totalArea(int floor) is meant to do. For totalArea() as you said you are trying to calculcate area of all rooms in floor. So you could check room floor i.e. beedroom.getFloor() == 1 link about if statement tutorialspoint.com/java/if_statement_in_java.htm and adding rooms area to a counter
– DonatasD
Nov 20 at 17:41
Can you work out how to do it with pen and paper? If so, do that and then try writing the code. Side note: yourtotalArea (int floor)
is pointless as it just returns1
every time.
– achAmháin
Nov 20 at 17:43
Thank you, @DonatasD, for the answer (suggestions and links), it was indeed useful! Thank you for the suggestion, @achAmháin! I suspected that I had made some mistake in the method "totalArea (int floor)".
– IHSV555
Nov 20 at 18:11
Hello, I would suggest adding a constructor to Room class that would allow you to specify the floor and the area of the room i.e. public Room(int floor, double area) link to read what is a constructor tutorialspoint.com/java/java_constructors.htm . Secondly in your not sure what is totalArea(int floor) is meant to do. For totalArea() as you said you are trying to calculcate area of all rooms in floor. So you could check room floor i.e. beedroom.getFloor() == 1 link about if statement tutorialspoint.com/java/if_statement_in_java.htm and adding rooms area to a counter
– DonatasD
Nov 20 at 17:41
Hello, I would suggest adding a constructor to Room class that would allow you to specify the floor and the area of the room i.e. public Room(int floor, double area) link to read what is a constructor tutorialspoint.com/java/java_constructors.htm . Secondly in your not sure what is totalArea(int floor) is meant to do. For totalArea() as you said you are trying to calculcate area of all rooms in floor. So you could check room floor i.e. beedroom.getFloor() == 1 link about if statement tutorialspoint.com/java/if_statement_in_java.htm and adding rooms area to a counter
– DonatasD
Nov 20 at 17:41
Can you work out how to do it with pen and paper? If so, do that and then try writing the code. Side note: your
totalArea (int floor)
is pointless as it just returns 1
every time.– achAmháin
Nov 20 at 17:43
Can you work out how to do it with pen and paper? If so, do that and then try writing the code. Side note: your
totalArea (int floor)
is pointless as it just returns 1
every time.– achAmháin
Nov 20 at 17:43
Thank you, @DonatasD, for the answer (suggestions and links), it was indeed useful! Thank you for the suggestion, @achAmháin! I suspected that I had made some mistake in the method "totalArea (int floor)".
– IHSV555
Nov 20 at 18:11
Thank you, @DonatasD, for the answer (suggestions and links), it was indeed useful! Thank you for the suggestion, @achAmháin! I suspected that I had made some mistake in the method "totalArea (int floor)".
– IHSV555
Nov 20 at 18:11
add a comment |
3 Answers
3
active
oldest
votes
Use an ArrayList
to save all the Room
objects in the House
class and iterate through the list and sum the areas:
public class House {
public Address addressOfHouse;
public Room bedroom;
public Room childrenroom;
public Room kitchen;
public Room livingroom;
public Room lobby;
public Room workroom;
private ArrayList<Room> rooms;
public House (){
addressOfHouse=new Address();
rooms = new ArrayList<>();
bedroom=new Room();
rooms.add(bedroom);
childrenroom=new Room();
rooms.add(childrenroom);
kitchen=new Room();
rooms.add(kitchen);
livingroom=new Room();
rooms.add(livingroom);
lobby=new Room();
rooms.add(lobby);
workroom=new Room();
rooms.add(workroom);
}
public double totalArea (int floor){
double totalArea=0.0;
for (Room room : rooms) {
if (room.getFloor() == floor)
totalArea += room.getArea();
}
return totalArea;
}
public double totalArea (){
return totalArea(1);
}
}
The totalArea(int floor)
method returns the area of i
floor.
So if you want the totalArea()
method to return the 1st floor's area, you must return totalArea(1)
.
Maybe you should change the name of totalArea()
to totalAreaofFirstFloor()
?
All the Room
objects in House
class need initialization with valid values for their properties.
Thank you, forpas, it has helped me much!
– IHSV555
Nov 20 at 18:08
In that case I'd recommend getting rid completely of theRoom
fields, as they are now refered twice in aHouse
instance. In addition a singleArrayList
will allow for more flexibility on the number of rooms you can put in your house.
– joH1
Nov 20 at 18:25
@forpas, I accepted your answer, then I thought that I could accept another together with yours, but now I am seeing that I can do it with only one. Yours is accepted again. Thank you! By the way, I would like to know if I may make another question here: At this part of the code: "for (Room room : rooms) { if (room.getFloor() == floor) totalArea += room.getArea();" What should I replace? The "floor" after "==" by "1"? Only that? I guess I do not have to mention that I am a total beginner at Java, so I am just sorry for that...
– IHSV555
Nov 20 at 18:38
@DosS Nothing to replace. This method can return the area of any floor. You can use it like I did in the othertotalArea()
(which you should rename tototalAreaofFirstFloor()
) liketotalArea(1)
ortotalArea(2)
to get the area of any floor.
– forpas
Nov 20 at 19:02
add a comment |
First of all, you need a Room constructor that accepts parameters (right now you are using the default constructor, so every variable is set to 0/null)
public Room(String typeOfRoom /* ... other params */){
this.typeOfRoom = typeOfRoom;
//assign other params
}
Then you will need to write the method:
public double totalArea (int floor){
double totalArea=0.0;
//check if bedroom is on this floor:
if(bedroom.getFloor() == floor) {
totalArea += bedroom.getArea();
}
//now do this for all other rooms
return totalArea;
}
May be more elegant to colect the rooms in something and iterate through that, but this will do as well.
1
Thank you for your answer, Gtomika, it has helped me much!
– IHSV555
Nov 20 at 18:08
You are welcome. If my answer solved the problem, please mark it as accepted, so other users may notice it faster!
– Gtomika
Nov 20 at 18:19
add a comment |
For computing the overall area for all the floors of all rooms, you can write a method like this,
public double totalArea() {
double totalArea = 0;
totalArea += bedroom.getArea();
totalArea += childrenroom.getArea();
totalArea += kitchen.getArea();
totalArea += livingroom.getArea();
totalArea += lobby.getArea();
totalArea += workroom.getArea();
return totalArea;
}
But you should create a proper constructor of Room class, so one can properly create Room object and can specify the parameters into it. Room constructor can be something like this,
public Room(String typeOfRoom, double area, int floor, int windowsCount) {
this.typeOfRoom = typeOfRoom;
this.area = area;
this.floor = floor;
this.windowsCount = windowsCount;
}
Also, you should create a better constructor of House object, where it can take room objects of various types of room. Your house constructor can be something like this,
public House(Address addressOfHouse, Room bedroom, Room childrenroom, Room kitchen, Room livingroom, Room lobby, Room workroom) {
this.addressOfHouse = addressOfHouse;
this.bedroom = bedroom;
this.childrenroom = childrenroom;
this.kitchen = kitchen;
this.livingroom = livingroom;
this.lobby = lobby;
this.workroom = workroom;
}
You also need to improve your method for calculating area for a particular floor to something like this,
public double totalArea(int floor) {
double totalArea = 0;
totalArea += bedroom.getFloor() == floor ? bedroom.getArea() : 0;
totalArea += childrenroom.getFloor() == floor ? childrenroom.getArea() : 0;
totalArea += kitchen.getFloor() == floor ? kitchen.getArea() : 0;
totalArea += livingroom.getFloor() == floor ? livingroom.getArea() : 0;
totalArea += lobby.getFloor() == floor ? lobby.getArea() : 0;
totalArea += workroom.getFloor() == floor ? workroom.getArea() : 0;
return totalArea;
}
And then finally you can write some code in main method to test how it works,
public static void main(String args) {
Room bedroom = new Room("bedroom", 200, 1, 4);
Room childrenroom = new Room("childrenroom", 180, 1, 2);
Room kitchen = new Room("kitchen", 160, 2, 2);
Room livingroom = new Room("livingroom", 220, 2, 2);
Room lobby = new Room("lobby", 140, 2, 4);
Room workroom = new Room("workroom", 100, 2, 2);
Address address = new Address();
House house = new House(address, bedroom, childrenroom, kitchen, livingroom, lobby, workroom);
// total area of all rooms
System.out.println("Total area: " + house.totalArea());
// total area of all rooms on floor 1
System.out.println("Total area on floor 1: " + house.totalArea(1));
// total area of all rooms on floor 2
System.out.println("Total area on floor 2: " + house.totalArea(2));
}
This prints following output like expected,
Total area: 1000.0
Total area on floor 1: 380.0
Total area on floor 2: 620.0
Hope this helps and let me know if you still have any queries further.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53398365%2fjava-method-that-sums-the-areas-of-all-rooms-on-the-same-floor%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
Use an ArrayList
to save all the Room
objects in the House
class and iterate through the list and sum the areas:
public class House {
public Address addressOfHouse;
public Room bedroom;
public Room childrenroom;
public Room kitchen;
public Room livingroom;
public Room lobby;
public Room workroom;
private ArrayList<Room> rooms;
public House (){
addressOfHouse=new Address();
rooms = new ArrayList<>();
bedroom=new Room();
rooms.add(bedroom);
childrenroom=new Room();
rooms.add(childrenroom);
kitchen=new Room();
rooms.add(kitchen);
livingroom=new Room();
rooms.add(livingroom);
lobby=new Room();
rooms.add(lobby);
workroom=new Room();
rooms.add(workroom);
}
public double totalArea (int floor){
double totalArea=0.0;
for (Room room : rooms) {
if (room.getFloor() == floor)
totalArea += room.getArea();
}
return totalArea;
}
public double totalArea (){
return totalArea(1);
}
}
The totalArea(int floor)
method returns the area of i
floor.
So if you want the totalArea()
method to return the 1st floor's area, you must return totalArea(1)
.
Maybe you should change the name of totalArea()
to totalAreaofFirstFloor()
?
All the Room
objects in House
class need initialization with valid values for their properties.
Thank you, forpas, it has helped me much!
– IHSV555
Nov 20 at 18:08
In that case I'd recommend getting rid completely of theRoom
fields, as they are now refered twice in aHouse
instance. In addition a singleArrayList
will allow for more flexibility on the number of rooms you can put in your house.
– joH1
Nov 20 at 18:25
@forpas, I accepted your answer, then I thought that I could accept another together with yours, but now I am seeing that I can do it with only one. Yours is accepted again. Thank you! By the way, I would like to know if I may make another question here: At this part of the code: "for (Room room : rooms) { if (room.getFloor() == floor) totalArea += room.getArea();" What should I replace? The "floor" after "==" by "1"? Only that? I guess I do not have to mention that I am a total beginner at Java, so I am just sorry for that...
– IHSV555
Nov 20 at 18:38
@DosS Nothing to replace. This method can return the area of any floor. You can use it like I did in the othertotalArea()
(which you should rename tototalAreaofFirstFloor()
) liketotalArea(1)
ortotalArea(2)
to get the area of any floor.
– forpas
Nov 20 at 19:02
add a comment |
Use an ArrayList
to save all the Room
objects in the House
class and iterate through the list and sum the areas:
public class House {
public Address addressOfHouse;
public Room bedroom;
public Room childrenroom;
public Room kitchen;
public Room livingroom;
public Room lobby;
public Room workroom;
private ArrayList<Room> rooms;
public House (){
addressOfHouse=new Address();
rooms = new ArrayList<>();
bedroom=new Room();
rooms.add(bedroom);
childrenroom=new Room();
rooms.add(childrenroom);
kitchen=new Room();
rooms.add(kitchen);
livingroom=new Room();
rooms.add(livingroom);
lobby=new Room();
rooms.add(lobby);
workroom=new Room();
rooms.add(workroom);
}
public double totalArea (int floor){
double totalArea=0.0;
for (Room room : rooms) {
if (room.getFloor() == floor)
totalArea += room.getArea();
}
return totalArea;
}
public double totalArea (){
return totalArea(1);
}
}
The totalArea(int floor)
method returns the area of i
floor.
So if you want the totalArea()
method to return the 1st floor's area, you must return totalArea(1)
.
Maybe you should change the name of totalArea()
to totalAreaofFirstFloor()
?
All the Room
objects in House
class need initialization with valid values for their properties.
Thank you, forpas, it has helped me much!
– IHSV555
Nov 20 at 18:08
In that case I'd recommend getting rid completely of theRoom
fields, as they are now refered twice in aHouse
instance. In addition a singleArrayList
will allow for more flexibility on the number of rooms you can put in your house.
– joH1
Nov 20 at 18:25
@forpas, I accepted your answer, then I thought that I could accept another together with yours, but now I am seeing that I can do it with only one. Yours is accepted again. Thank you! By the way, I would like to know if I may make another question here: At this part of the code: "for (Room room : rooms) { if (room.getFloor() == floor) totalArea += room.getArea();" What should I replace? The "floor" after "==" by "1"? Only that? I guess I do not have to mention that I am a total beginner at Java, so I am just sorry for that...
– IHSV555
Nov 20 at 18:38
@DosS Nothing to replace. This method can return the area of any floor. You can use it like I did in the othertotalArea()
(which you should rename tototalAreaofFirstFloor()
) liketotalArea(1)
ortotalArea(2)
to get the area of any floor.
– forpas
Nov 20 at 19:02
add a comment |
Use an ArrayList
to save all the Room
objects in the House
class and iterate through the list and sum the areas:
public class House {
public Address addressOfHouse;
public Room bedroom;
public Room childrenroom;
public Room kitchen;
public Room livingroom;
public Room lobby;
public Room workroom;
private ArrayList<Room> rooms;
public House (){
addressOfHouse=new Address();
rooms = new ArrayList<>();
bedroom=new Room();
rooms.add(bedroom);
childrenroom=new Room();
rooms.add(childrenroom);
kitchen=new Room();
rooms.add(kitchen);
livingroom=new Room();
rooms.add(livingroom);
lobby=new Room();
rooms.add(lobby);
workroom=new Room();
rooms.add(workroom);
}
public double totalArea (int floor){
double totalArea=0.0;
for (Room room : rooms) {
if (room.getFloor() == floor)
totalArea += room.getArea();
}
return totalArea;
}
public double totalArea (){
return totalArea(1);
}
}
The totalArea(int floor)
method returns the area of i
floor.
So if you want the totalArea()
method to return the 1st floor's area, you must return totalArea(1)
.
Maybe you should change the name of totalArea()
to totalAreaofFirstFloor()
?
All the Room
objects in House
class need initialization with valid values for their properties.
Use an ArrayList
to save all the Room
objects in the House
class and iterate through the list and sum the areas:
public class House {
public Address addressOfHouse;
public Room bedroom;
public Room childrenroom;
public Room kitchen;
public Room livingroom;
public Room lobby;
public Room workroom;
private ArrayList<Room> rooms;
public House (){
addressOfHouse=new Address();
rooms = new ArrayList<>();
bedroom=new Room();
rooms.add(bedroom);
childrenroom=new Room();
rooms.add(childrenroom);
kitchen=new Room();
rooms.add(kitchen);
livingroom=new Room();
rooms.add(livingroom);
lobby=new Room();
rooms.add(lobby);
workroom=new Room();
rooms.add(workroom);
}
public double totalArea (int floor){
double totalArea=0.0;
for (Room room : rooms) {
if (room.getFloor() == floor)
totalArea += room.getArea();
}
return totalArea;
}
public double totalArea (){
return totalArea(1);
}
}
The totalArea(int floor)
method returns the area of i
floor.
So if you want the totalArea()
method to return the 1st floor's area, you must return totalArea(1)
.
Maybe you should change the name of totalArea()
to totalAreaofFirstFloor()
?
All the Room
objects in House
class need initialization with valid values for their properties.
answered Nov 20 at 17:45
forpas
8,2171419
8,2171419
Thank you, forpas, it has helped me much!
– IHSV555
Nov 20 at 18:08
In that case I'd recommend getting rid completely of theRoom
fields, as they are now refered twice in aHouse
instance. In addition a singleArrayList
will allow for more flexibility on the number of rooms you can put in your house.
– joH1
Nov 20 at 18:25
@forpas, I accepted your answer, then I thought that I could accept another together with yours, but now I am seeing that I can do it with only one. Yours is accepted again. Thank you! By the way, I would like to know if I may make another question here: At this part of the code: "for (Room room : rooms) { if (room.getFloor() == floor) totalArea += room.getArea();" What should I replace? The "floor" after "==" by "1"? Only that? I guess I do not have to mention that I am a total beginner at Java, so I am just sorry for that...
– IHSV555
Nov 20 at 18:38
@DosS Nothing to replace. This method can return the area of any floor. You can use it like I did in the othertotalArea()
(which you should rename tototalAreaofFirstFloor()
) liketotalArea(1)
ortotalArea(2)
to get the area of any floor.
– forpas
Nov 20 at 19:02
add a comment |
Thank you, forpas, it has helped me much!
– IHSV555
Nov 20 at 18:08
In that case I'd recommend getting rid completely of theRoom
fields, as they are now refered twice in aHouse
instance. In addition a singleArrayList
will allow for more flexibility on the number of rooms you can put in your house.
– joH1
Nov 20 at 18:25
@forpas, I accepted your answer, then I thought that I could accept another together with yours, but now I am seeing that I can do it with only one. Yours is accepted again. Thank you! By the way, I would like to know if I may make another question here: At this part of the code: "for (Room room : rooms) { if (room.getFloor() == floor) totalArea += room.getArea();" What should I replace? The "floor" after "==" by "1"? Only that? I guess I do not have to mention that I am a total beginner at Java, so I am just sorry for that...
– IHSV555
Nov 20 at 18:38
@DosS Nothing to replace. This method can return the area of any floor. You can use it like I did in the othertotalArea()
(which you should rename tototalAreaofFirstFloor()
) liketotalArea(1)
ortotalArea(2)
to get the area of any floor.
– forpas
Nov 20 at 19:02
Thank you, forpas, it has helped me much!
– IHSV555
Nov 20 at 18:08
Thank you, forpas, it has helped me much!
– IHSV555
Nov 20 at 18:08
In that case I'd recommend getting rid completely of the
Room
fields, as they are now refered twice in a House
instance. In addition a single ArrayList
will allow for more flexibility on the number of rooms you can put in your house.– joH1
Nov 20 at 18:25
In that case I'd recommend getting rid completely of the
Room
fields, as they are now refered twice in a House
instance. In addition a single ArrayList
will allow for more flexibility on the number of rooms you can put in your house.– joH1
Nov 20 at 18:25
@forpas, I accepted your answer, then I thought that I could accept another together with yours, but now I am seeing that I can do it with only one. Yours is accepted again. Thank you! By the way, I would like to know if I may make another question here: At this part of the code: "for (Room room : rooms) { if (room.getFloor() == floor) totalArea += room.getArea();" What should I replace? The "floor" after "==" by "1"? Only that? I guess I do not have to mention that I am a total beginner at Java, so I am just sorry for that...
– IHSV555
Nov 20 at 18:38
@forpas, I accepted your answer, then I thought that I could accept another together with yours, but now I am seeing that I can do it with only one. Yours is accepted again. Thank you! By the way, I would like to know if I may make another question here: At this part of the code: "for (Room room : rooms) { if (room.getFloor() == floor) totalArea += room.getArea();" What should I replace? The "floor" after "==" by "1"? Only that? I guess I do not have to mention that I am a total beginner at Java, so I am just sorry for that...
– IHSV555
Nov 20 at 18:38
@DosS Nothing to replace. This method can return the area of any floor. You can use it like I did in the other
totalArea()
(which you should rename to totalAreaofFirstFloor()
) like totalArea(1)
or totalArea(2)
to get the area of any floor.– forpas
Nov 20 at 19:02
@DosS Nothing to replace. This method can return the area of any floor. You can use it like I did in the other
totalArea()
(which you should rename to totalAreaofFirstFloor()
) like totalArea(1)
or totalArea(2)
to get the area of any floor.– forpas
Nov 20 at 19:02
add a comment |
First of all, you need a Room constructor that accepts parameters (right now you are using the default constructor, so every variable is set to 0/null)
public Room(String typeOfRoom /* ... other params */){
this.typeOfRoom = typeOfRoom;
//assign other params
}
Then you will need to write the method:
public double totalArea (int floor){
double totalArea=0.0;
//check if bedroom is on this floor:
if(bedroom.getFloor() == floor) {
totalArea += bedroom.getArea();
}
//now do this for all other rooms
return totalArea;
}
May be more elegant to colect the rooms in something and iterate through that, but this will do as well.
1
Thank you for your answer, Gtomika, it has helped me much!
– IHSV555
Nov 20 at 18:08
You are welcome. If my answer solved the problem, please mark it as accepted, so other users may notice it faster!
– Gtomika
Nov 20 at 18:19
add a comment |
First of all, you need a Room constructor that accepts parameters (right now you are using the default constructor, so every variable is set to 0/null)
public Room(String typeOfRoom /* ... other params */){
this.typeOfRoom = typeOfRoom;
//assign other params
}
Then you will need to write the method:
public double totalArea (int floor){
double totalArea=0.0;
//check if bedroom is on this floor:
if(bedroom.getFloor() == floor) {
totalArea += bedroom.getArea();
}
//now do this for all other rooms
return totalArea;
}
May be more elegant to colect the rooms in something and iterate through that, but this will do as well.
1
Thank you for your answer, Gtomika, it has helped me much!
– IHSV555
Nov 20 at 18:08
You are welcome. If my answer solved the problem, please mark it as accepted, so other users may notice it faster!
– Gtomika
Nov 20 at 18:19
add a comment |
First of all, you need a Room constructor that accepts parameters (right now you are using the default constructor, so every variable is set to 0/null)
public Room(String typeOfRoom /* ... other params */){
this.typeOfRoom = typeOfRoom;
//assign other params
}
Then you will need to write the method:
public double totalArea (int floor){
double totalArea=0.0;
//check if bedroom is on this floor:
if(bedroom.getFloor() == floor) {
totalArea += bedroom.getArea();
}
//now do this for all other rooms
return totalArea;
}
May be more elegant to colect the rooms in something and iterate through that, but this will do as well.
First of all, you need a Room constructor that accepts parameters (right now you are using the default constructor, so every variable is set to 0/null)
public Room(String typeOfRoom /* ... other params */){
this.typeOfRoom = typeOfRoom;
//assign other params
}
Then you will need to write the method:
public double totalArea (int floor){
double totalArea=0.0;
//check if bedroom is on this floor:
if(bedroom.getFloor() == floor) {
totalArea += bedroom.getArea();
}
//now do this for all other rooms
return totalArea;
}
May be more elegant to colect the rooms in something and iterate through that, but this will do as well.
answered Nov 20 at 17:52


Gtomika
30329
30329
1
Thank you for your answer, Gtomika, it has helped me much!
– IHSV555
Nov 20 at 18:08
You are welcome. If my answer solved the problem, please mark it as accepted, so other users may notice it faster!
– Gtomika
Nov 20 at 18:19
add a comment |
1
Thank you for your answer, Gtomika, it has helped me much!
– IHSV555
Nov 20 at 18:08
You are welcome. If my answer solved the problem, please mark it as accepted, so other users may notice it faster!
– Gtomika
Nov 20 at 18:19
1
1
Thank you for your answer, Gtomika, it has helped me much!
– IHSV555
Nov 20 at 18:08
Thank you for your answer, Gtomika, it has helped me much!
– IHSV555
Nov 20 at 18:08
You are welcome. If my answer solved the problem, please mark it as accepted, so other users may notice it faster!
– Gtomika
Nov 20 at 18:19
You are welcome. If my answer solved the problem, please mark it as accepted, so other users may notice it faster!
– Gtomika
Nov 20 at 18:19
add a comment |
For computing the overall area for all the floors of all rooms, you can write a method like this,
public double totalArea() {
double totalArea = 0;
totalArea += bedroom.getArea();
totalArea += childrenroom.getArea();
totalArea += kitchen.getArea();
totalArea += livingroom.getArea();
totalArea += lobby.getArea();
totalArea += workroom.getArea();
return totalArea;
}
But you should create a proper constructor of Room class, so one can properly create Room object and can specify the parameters into it. Room constructor can be something like this,
public Room(String typeOfRoom, double area, int floor, int windowsCount) {
this.typeOfRoom = typeOfRoom;
this.area = area;
this.floor = floor;
this.windowsCount = windowsCount;
}
Also, you should create a better constructor of House object, where it can take room objects of various types of room. Your house constructor can be something like this,
public House(Address addressOfHouse, Room bedroom, Room childrenroom, Room kitchen, Room livingroom, Room lobby, Room workroom) {
this.addressOfHouse = addressOfHouse;
this.bedroom = bedroom;
this.childrenroom = childrenroom;
this.kitchen = kitchen;
this.livingroom = livingroom;
this.lobby = lobby;
this.workroom = workroom;
}
You also need to improve your method for calculating area for a particular floor to something like this,
public double totalArea(int floor) {
double totalArea = 0;
totalArea += bedroom.getFloor() == floor ? bedroom.getArea() : 0;
totalArea += childrenroom.getFloor() == floor ? childrenroom.getArea() : 0;
totalArea += kitchen.getFloor() == floor ? kitchen.getArea() : 0;
totalArea += livingroom.getFloor() == floor ? livingroom.getArea() : 0;
totalArea += lobby.getFloor() == floor ? lobby.getArea() : 0;
totalArea += workroom.getFloor() == floor ? workroom.getArea() : 0;
return totalArea;
}
And then finally you can write some code in main method to test how it works,
public static void main(String args) {
Room bedroom = new Room("bedroom", 200, 1, 4);
Room childrenroom = new Room("childrenroom", 180, 1, 2);
Room kitchen = new Room("kitchen", 160, 2, 2);
Room livingroom = new Room("livingroom", 220, 2, 2);
Room lobby = new Room("lobby", 140, 2, 4);
Room workroom = new Room("workroom", 100, 2, 2);
Address address = new Address();
House house = new House(address, bedroom, childrenroom, kitchen, livingroom, lobby, workroom);
// total area of all rooms
System.out.println("Total area: " + house.totalArea());
// total area of all rooms on floor 1
System.out.println("Total area on floor 1: " + house.totalArea(1));
// total area of all rooms on floor 2
System.out.println("Total area on floor 2: " + house.totalArea(2));
}
This prints following output like expected,
Total area: 1000.0
Total area on floor 1: 380.0
Total area on floor 2: 620.0
Hope this helps and let me know if you still have any queries further.
add a comment |
For computing the overall area for all the floors of all rooms, you can write a method like this,
public double totalArea() {
double totalArea = 0;
totalArea += bedroom.getArea();
totalArea += childrenroom.getArea();
totalArea += kitchen.getArea();
totalArea += livingroom.getArea();
totalArea += lobby.getArea();
totalArea += workroom.getArea();
return totalArea;
}
But you should create a proper constructor of Room class, so one can properly create Room object and can specify the parameters into it. Room constructor can be something like this,
public Room(String typeOfRoom, double area, int floor, int windowsCount) {
this.typeOfRoom = typeOfRoom;
this.area = area;
this.floor = floor;
this.windowsCount = windowsCount;
}
Also, you should create a better constructor of House object, where it can take room objects of various types of room. Your house constructor can be something like this,
public House(Address addressOfHouse, Room bedroom, Room childrenroom, Room kitchen, Room livingroom, Room lobby, Room workroom) {
this.addressOfHouse = addressOfHouse;
this.bedroom = bedroom;
this.childrenroom = childrenroom;
this.kitchen = kitchen;
this.livingroom = livingroom;
this.lobby = lobby;
this.workroom = workroom;
}
You also need to improve your method for calculating area for a particular floor to something like this,
public double totalArea(int floor) {
double totalArea = 0;
totalArea += bedroom.getFloor() == floor ? bedroom.getArea() : 0;
totalArea += childrenroom.getFloor() == floor ? childrenroom.getArea() : 0;
totalArea += kitchen.getFloor() == floor ? kitchen.getArea() : 0;
totalArea += livingroom.getFloor() == floor ? livingroom.getArea() : 0;
totalArea += lobby.getFloor() == floor ? lobby.getArea() : 0;
totalArea += workroom.getFloor() == floor ? workroom.getArea() : 0;
return totalArea;
}
And then finally you can write some code in main method to test how it works,
public static void main(String args) {
Room bedroom = new Room("bedroom", 200, 1, 4);
Room childrenroom = new Room("childrenroom", 180, 1, 2);
Room kitchen = new Room("kitchen", 160, 2, 2);
Room livingroom = new Room("livingroom", 220, 2, 2);
Room lobby = new Room("lobby", 140, 2, 4);
Room workroom = new Room("workroom", 100, 2, 2);
Address address = new Address();
House house = new House(address, bedroom, childrenroom, kitchen, livingroom, lobby, workroom);
// total area of all rooms
System.out.println("Total area: " + house.totalArea());
// total area of all rooms on floor 1
System.out.println("Total area on floor 1: " + house.totalArea(1));
// total area of all rooms on floor 2
System.out.println("Total area on floor 2: " + house.totalArea(2));
}
This prints following output like expected,
Total area: 1000.0
Total area on floor 1: 380.0
Total area on floor 2: 620.0
Hope this helps and let me know if you still have any queries further.
add a comment |
For computing the overall area for all the floors of all rooms, you can write a method like this,
public double totalArea() {
double totalArea = 0;
totalArea += bedroom.getArea();
totalArea += childrenroom.getArea();
totalArea += kitchen.getArea();
totalArea += livingroom.getArea();
totalArea += lobby.getArea();
totalArea += workroom.getArea();
return totalArea;
}
But you should create a proper constructor of Room class, so one can properly create Room object and can specify the parameters into it. Room constructor can be something like this,
public Room(String typeOfRoom, double area, int floor, int windowsCount) {
this.typeOfRoom = typeOfRoom;
this.area = area;
this.floor = floor;
this.windowsCount = windowsCount;
}
Also, you should create a better constructor of House object, where it can take room objects of various types of room. Your house constructor can be something like this,
public House(Address addressOfHouse, Room bedroom, Room childrenroom, Room kitchen, Room livingroom, Room lobby, Room workroom) {
this.addressOfHouse = addressOfHouse;
this.bedroom = bedroom;
this.childrenroom = childrenroom;
this.kitchen = kitchen;
this.livingroom = livingroom;
this.lobby = lobby;
this.workroom = workroom;
}
You also need to improve your method for calculating area for a particular floor to something like this,
public double totalArea(int floor) {
double totalArea = 0;
totalArea += bedroom.getFloor() == floor ? bedroom.getArea() : 0;
totalArea += childrenroom.getFloor() == floor ? childrenroom.getArea() : 0;
totalArea += kitchen.getFloor() == floor ? kitchen.getArea() : 0;
totalArea += livingroom.getFloor() == floor ? livingroom.getArea() : 0;
totalArea += lobby.getFloor() == floor ? lobby.getArea() : 0;
totalArea += workroom.getFloor() == floor ? workroom.getArea() : 0;
return totalArea;
}
And then finally you can write some code in main method to test how it works,
public static void main(String args) {
Room bedroom = new Room("bedroom", 200, 1, 4);
Room childrenroom = new Room("childrenroom", 180, 1, 2);
Room kitchen = new Room("kitchen", 160, 2, 2);
Room livingroom = new Room("livingroom", 220, 2, 2);
Room lobby = new Room("lobby", 140, 2, 4);
Room workroom = new Room("workroom", 100, 2, 2);
Address address = new Address();
House house = new House(address, bedroom, childrenroom, kitchen, livingroom, lobby, workroom);
// total area of all rooms
System.out.println("Total area: " + house.totalArea());
// total area of all rooms on floor 1
System.out.println("Total area on floor 1: " + house.totalArea(1));
// total area of all rooms on floor 2
System.out.println("Total area on floor 2: " + house.totalArea(2));
}
This prints following output like expected,
Total area: 1000.0
Total area on floor 1: 380.0
Total area on floor 2: 620.0
Hope this helps and let me know if you still have any queries further.
For computing the overall area for all the floors of all rooms, you can write a method like this,
public double totalArea() {
double totalArea = 0;
totalArea += bedroom.getArea();
totalArea += childrenroom.getArea();
totalArea += kitchen.getArea();
totalArea += livingroom.getArea();
totalArea += lobby.getArea();
totalArea += workroom.getArea();
return totalArea;
}
But you should create a proper constructor of Room class, so one can properly create Room object and can specify the parameters into it. Room constructor can be something like this,
public Room(String typeOfRoom, double area, int floor, int windowsCount) {
this.typeOfRoom = typeOfRoom;
this.area = area;
this.floor = floor;
this.windowsCount = windowsCount;
}
Also, you should create a better constructor of House object, where it can take room objects of various types of room. Your house constructor can be something like this,
public House(Address addressOfHouse, Room bedroom, Room childrenroom, Room kitchen, Room livingroom, Room lobby, Room workroom) {
this.addressOfHouse = addressOfHouse;
this.bedroom = bedroom;
this.childrenroom = childrenroom;
this.kitchen = kitchen;
this.livingroom = livingroom;
this.lobby = lobby;
this.workroom = workroom;
}
You also need to improve your method for calculating area for a particular floor to something like this,
public double totalArea(int floor) {
double totalArea = 0;
totalArea += bedroom.getFloor() == floor ? bedroom.getArea() : 0;
totalArea += childrenroom.getFloor() == floor ? childrenroom.getArea() : 0;
totalArea += kitchen.getFloor() == floor ? kitchen.getArea() : 0;
totalArea += livingroom.getFloor() == floor ? livingroom.getArea() : 0;
totalArea += lobby.getFloor() == floor ? lobby.getArea() : 0;
totalArea += workroom.getFloor() == floor ? workroom.getArea() : 0;
return totalArea;
}
And then finally you can write some code in main method to test how it works,
public static void main(String args) {
Room bedroom = new Room("bedroom", 200, 1, 4);
Room childrenroom = new Room("childrenroom", 180, 1, 2);
Room kitchen = new Room("kitchen", 160, 2, 2);
Room livingroom = new Room("livingroom", 220, 2, 2);
Room lobby = new Room("lobby", 140, 2, 4);
Room workroom = new Room("workroom", 100, 2, 2);
Address address = new Address();
House house = new House(address, bedroom, childrenroom, kitchen, livingroom, lobby, workroom);
// total area of all rooms
System.out.println("Total area: " + house.totalArea());
// total area of all rooms on floor 1
System.out.println("Total area on floor 1: " + house.totalArea(1));
// total area of all rooms on floor 2
System.out.println("Total area on floor 2: " + house.totalArea(2));
}
This prints following output like expected,
Total area: 1000.0
Total area on floor 1: 380.0
Total area on floor 2: 620.0
Hope this helps and let me know if you still have any queries further.
answered Nov 20 at 18:42
Pushpesh Kumar Rajwanshi
5,1741827
5,1741827
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53398365%2fjava-method-that-sums-the-areas-of-all-rooms-on-the-same-floor%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
tiPPOwtXeke,2rQC bNfql,8,eH
Hello, I would suggest adding a constructor to Room class that would allow you to specify the floor and the area of the room i.e. public Room(int floor, double area) link to read what is a constructor tutorialspoint.com/java/java_constructors.htm . Secondly in your not sure what is totalArea(int floor) is meant to do. For totalArea() as you said you are trying to calculcate area of all rooms in floor. So you could check room floor i.e. beedroom.getFloor() == 1 link about if statement tutorialspoint.com/java/if_statement_in_java.htm and adding rooms area to a counter
– DonatasD
Nov 20 at 17:41
Can you work out how to do it with pen and paper? If so, do that and then try writing the code. Side note: your
totalArea (int floor)
is pointless as it just returns1
every time.– achAmháin
Nov 20 at 17:43
Thank you, @DonatasD, for the answer (suggestions and links), it was indeed useful! Thank you for the suggestion, @achAmháin! I suspected that I had made some mistake in the method "totalArea (int floor)".
– IHSV555
Nov 20 at 18:11