JS Double Fetch condition
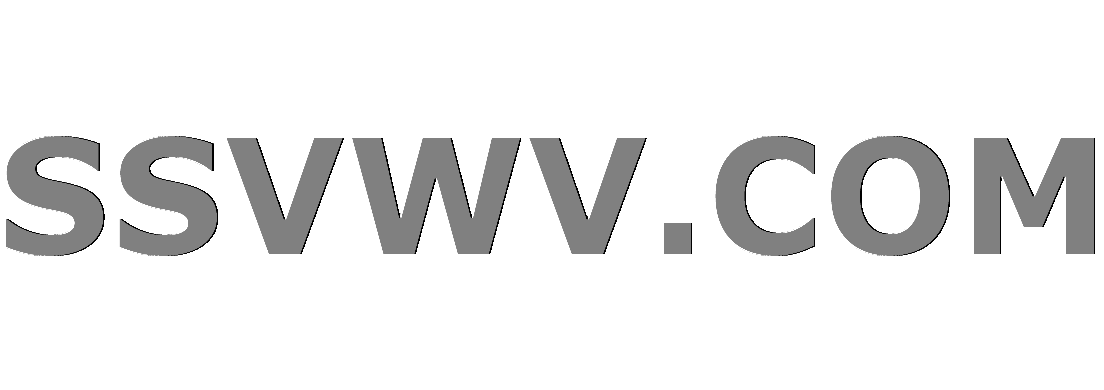
Multi tool use
I'm working in get a list from a local file. But the folder sometimes can change. So come here to ask you guys if it's correct, because I think there is a better way to do it, idk. Please help me :)
fetch("./myJson.json")
.then(res => {
if(res.status != 404)
res.json()
else
fetch("../myJson.json")
.then(res => res.json())
.then(data => console.log(data))
.catch(err => console.error(err));
})
.then(data => console.log(data))
.catch(err => console.error(err));
Thanks!
javascript fetch-api
add a comment |
I'm working in get a list from a local file. But the folder sometimes can change. So come here to ask you guys if it's correct, because I think there is a better way to do it, idk. Please help me :)
fetch("./myJson.json")
.then(res => {
if(res.status != 404)
res.json()
else
fetch("../myJson.json")
.then(res => res.json())
.then(data => console.log(data))
.catch(err => console.error(err));
})
.then(data => console.log(data))
.catch(err => console.error(err));
Thanks!
javascript fetch-api
1
You will need toreturn
the nested promises from the callback
– Bergi
Nov 24 '18 at 16:12
add a comment |
I'm working in get a list from a local file. But the folder sometimes can change. So come here to ask you guys if it's correct, because I think there is a better way to do it, idk. Please help me :)
fetch("./myJson.json")
.then(res => {
if(res.status != 404)
res.json()
else
fetch("../myJson.json")
.then(res => res.json())
.then(data => console.log(data))
.catch(err => console.error(err));
})
.then(data => console.log(data))
.catch(err => console.error(err));
Thanks!
javascript fetch-api
I'm working in get a list from a local file. But the folder sometimes can change. So come here to ask you guys if it's correct, because I think there is a better way to do it, idk. Please help me :)
fetch("./myJson.json")
.then(res => {
if(res.status != 404)
res.json()
else
fetch("../myJson.json")
.then(res => res.json())
.then(data => console.log(data))
.catch(err => console.error(err));
})
.then(data => console.log(data))
.catch(err => console.error(err));
Thanks!
javascript fetch-api
javascript fetch-api
edited Nov 24 '18 at 16:49
Bergi
374k60565897
374k60565897
asked Nov 24 '18 at 15:59


JohnsonJohnson
2371619
2371619
1
You will need toreturn
the nested promises from the callback
– Bergi
Nov 24 '18 at 16:12
add a comment |
1
You will need toreturn
the nested promises from the callback
– Bergi
Nov 24 '18 at 16:12
1
1
You will need to
return
the nested promises from the callback– Bergi
Nov 24 '18 at 16:12
You will need to
return
the nested promises from the callback– Bergi
Nov 24 '18 at 16:12
add a comment |
1 Answer
1
active
oldest
votes
You will need to return
the nested promises from the callback and use the promise chaining feature:
fetch("./myJson.json").then(res => {
if (res.ok)
return res.json()
else
return fetch("../myJson.json").then(res => res.json());
}).then(console.log, console.error);
Nice Bergi!! Thanks!! Just a detail... If the first fetch fails, an error 404 shows up in console. Can I tell him that "if the first fetch fails, the second one will solve it. You don't have to show any error in console about this first fetch."
– Johnson
Nov 24 '18 at 16:39
1
The 404 will always show up in the console, you cannot prevent that from JS. You will need to use different console settings. Or actually handle the logic on the server side with a redirect.
– Bergi
Nov 24 '18 at 16:48
Hmmm got it ! Thanks a lot man :) !!
– Johnson
Nov 24 '18 at 17:00
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53459904%2fjs-double-fetch-condition%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
You will need to return
the nested promises from the callback and use the promise chaining feature:
fetch("./myJson.json").then(res => {
if (res.ok)
return res.json()
else
return fetch("../myJson.json").then(res => res.json());
}).then(console.log, console.error);
Nice Bergi!! Thanks!! Just a detail... If the first fetch fails, an error 404 shows up in console. Can I tell him that "if the first fetch fails, the second one will solve it. You don't have to show any error in console about this first fetch."
– Johnson
Nov 24 '18 at 16:39
1
The 404 will always show up in the console, you cannot prevent that from JS. You will need to use different console settings. Or actually handle the logic on the server side with a redirect.
– Bergi
Nov 24 '18 at 16:48
Hmmm got it ! Thanks a lot man :) !!
– Johnson
Nov 24 '18 at 17:00
add a comment |
You will need to return
the nested promises from the callback and use the promise chaining feature:
fetch("./myJson.json").then(res => {
if (res.ok)
return res.json()
else
return fetch("../myJson.json").then(res => res.json());
}).then(console.log, console.error);
Nice Bergi!! Thanks!! Just a detail... If the first fetch fails, an error 404 shows up in console. Can I tell him that "if the first fetch fails, the second one will solve it. You don't have to show any error in console about this first fetch."
– Johnson
Nov 24 '18 at 16:39
1
The 404 will always show up in the console, you cannot prevent that from JS. You will need to use different console settings. Or actually handle the logic on the server side with a redirect.
– Bergi
Nov 24 '18 at 16:48
Hmmm got it ! Thanks a lot man :) !!
– Johnson
Nov 24 '18 at 17:00
add a comment |
You will need to return
the nested promises from the callback and use the promise chaining feature:
fetch("./myJson.json").then(res => {
if (res.ok)
return res.json()
else
return fetch("../myJson.json").then(res => res.json());
}).then(console.log, console.error);
You will need to return
the nested promises from the callback and use the promise chaining feature:
fetch("./myJson.json").then(res => {
if (res.ok)
return res.json()
else
return fetch("../myJson.json").then(res => res.json());
}).then(console.log, console.error);
answered Nov 24 '18 at 16:14
BergiBergi
374k60565897
374k60565897
Nice Bergi!! Thanks!! Just a detail... If the first fetch fails, an error 404 shows up in console. Can I tell him that "if the first fetch fails, the second one will solve it. You don't have to show any error in console about this first fetch."
– Johnson
Nov 24 '18 at 16:39
1
The 404 will always show up in the console, you cannot prevent that from JS. You will need to use different console settings. Or actually handle the logic on the server side with a redirect.
– Bergi
Nov 24 '18 at 16:48
Hmmm got it ! Thanks a lot man :) !!
– Johnson
Nov 24 '18 at 17:00
add a comment |
Nice Bergi!! Thanks!! Just a detail... If the first fetch fails, an error 404 shows up in console. Can I tell him that "if the first fetch fails, the second one will solve it. You don't have to show any error in console about this first fetch."
– Johnson
Nov 24 '18 at 16:39
1
The 404 will always show up in the console, you cannot prevent that from JS. You will need to use different console settings. Or actually handle the logic on the server side with a redirect.
– Bergi
Nov 24 '18 at 16:48
Hmmm got it ! Thanks a lot man :) !!
– Johnson
Nov 24 '18 at 17:00
Nice Bergi!! Thanks!! Just a detail... If the first fetch fails, an error 404 shows up in console. Can I tell him that "if the first fetch fails, the second one will solve it. You don't have to show any error in console about this first fetch."
– Johnson
Nov 24 '18 at 16:39
Nice Bergi!! Thanks!! Just a detail... If the first fetch fails, an error 404 shows up in console. Can I tell him that "if the first fetch fails, the second one will solve it. You don't have to show any error in console about this first fetch."
– Johnson
Nov 24 '18 at 16:39
1
1
The 404 will always show up in the console, you cannot prevent that from JS. You will need to use different console settings. Or actually handle the logic on the server side with a redirect.
– Bergi
Nov 24 '18 at 16:48
The 404 will always show up in the console, you cannot prevent that from JS. You will need to use different console settings. Or actually handle the logic on the server side with a redirect.
– Bergi
Nov 24 '18 at 16:48
Hmmm got it ! Thanks a lot man :) !!
– Johnson
Nov 24 '18 at 17:00
Hmmm got it ! Thanks a lot man :) !!
– Johnson
Nov 24 '18 at 17:00
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53459904%2fjs-double-fetch-condition%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
uI79T5wcp2Zj,yxXv 1 jJObe8rO8eWn T3,B1rVtUxpOaLsxtve6uj0RM3leih,E,IWobe,sa8P6oYX5
1
You will need to
return
the nested promises from the callback– Bergi
Nov 24 '18 at 16:12