QLineEdit and QComboBox have unrelated behavior
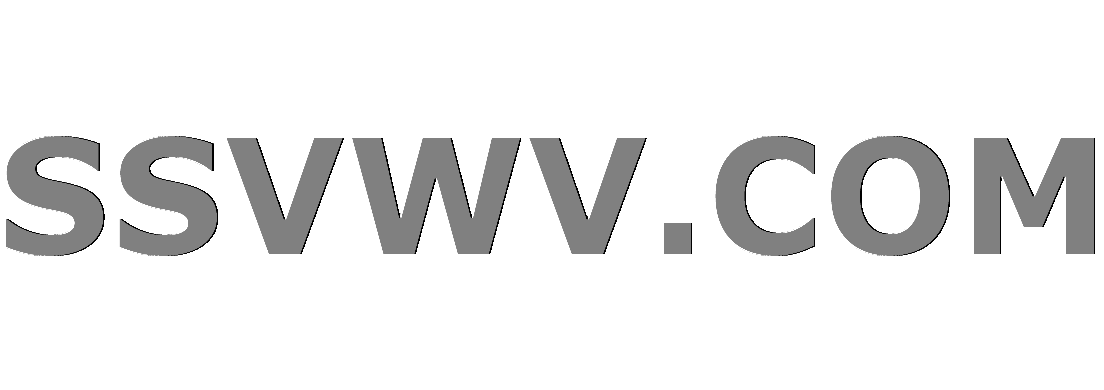
Multi tool use
I have a user interface with a lot of controls. However I have a problem with a QLineEdit and a QComboBox that are not responding properly.
I am basically converting from pixel measurements to millimeters/centimeters/decimeters and meters with a QComboBox and showing the result on a QLineEdit.
For the conversion table I used this page.
When I choose fromPixelToMillimeters()
it does the conversion, but when I choose fromPixelToCentimeters()
I think it is using the present value after the first conversion of fromPixelToMillimeters()
. And if I go back choosing fromPixelToMillimeters()
I get a different result too. This happens continuously, I get different measures each time.
See the code below:
void MainWindow::on_cBoxMeasures_currentIndexChanged(const QString &arg1)
{
if(arg1 == "Select Conversion(s)") {
return ui->leftLineEditDist->setText(QString("%1").arg(ui->leftLineEditDist->text().toDouble()));
} else if(arg1 == "pixel") {
return ui->leftLineEditDist->setText(QString("%1").arg(ui->leftLineEditDist->text().toDouble()));
} else if(arg1 == "mm") {
return fromPixelToMillimeters();
} else if(arg1 == "dm") {
return fromPixelToDecimeters();
} else if(arg1 == "cm") {
return fromPixelToCentimeters();
} else if(arg1 == "m") {
return fromPixelToMeters();
}
}
void MainWindow::fromPixelToMillimeters()
{
double mm = ui->leftLineEditDist->text().toDouble();
double dpi = 300;
double totalDistanceInMillimeter = (mm*25.4)/dpi;
ui->leftLineEditDist->setText(QString("%1").arg(totalDistanceInMillimeter));
ui->leftLineEditDist->show();
}
void MainWindow::fromPixelToCentimeters()
{
double mm = ui->leftLineEditDist->text().toDouble();
double dpi = 300;
double totalDistanceInCm = ((mm*25.4)/dpi)*0.1;
ui->leftLineEditDist->setText(QString("%1").arg(totalDistanceInCm));
ui->leftLineEditDist->show();
}
void MainWindow::fromPixelToDecimeters()
{
double mm = ui->leftLineEditDist->text().toDouble();
double dpi = 300;
double totalDistanceInDcm = ((mm*25.4)/dpi)*0.01;
ui->leftLineEditDist->setText(QString("%1").arg(totalDistanceInDcm));
ui->leftLineEditDist->show();
}
void MainWindow::fromPixelToMeters()
{
double mm = ui->leftLineEditDist->text().toDouble();
double dpi = 300;
double totalDistanceInM = ((mm*25.4)/dpi)*0.001;
ui->leftLineEditDist->setText(QString("%1").arg(totalDistanceInM));
ui->leftLineEditDist->show();
}
void MainWindow::on_cBoxMeasures_currentIndexChanged(int index)
{
switch (index) {
case(0):
break;
case(1):
break;
case(2):
fromPixelToMillimeters();
break;
case(3):
fromPixelToCentimeters();
break;
case(4):
fromPixelToDecimeters();
break;
case(5):
fromPixelToMeters();
break;
}
}
Please advise on what the problem might be.
c++11 qt5 qt4
add a comment |
I have a user interface with a lot of controls. However I have a problem with a QLineEdit and a QComboBox that are not responding properly.
I am basically converting from pixel measurements to millimeters/centimeters/decimeters and meters with a QComboBox and showing the result on a QLineEdit.
For the conversion table I used this page.
When I choose fromPixelToMillimeters()
it does the conversion, but when I choose fromPixelToCentimeters()
I think it is using the present value after the first conversion of fromPixelToMillimeters()
. And if I go back choosing fromPixelToMillimeters()
I get a different result too. This happens continuously, I get different measures each time.
See the code below:
void MainWindow::on_cBoxMeasures_currentIndexChanged(const QString &arg1)
{
if(arg1 == "Select Conversion(s)") {
return ui->leftLineEditDist->setText(QString("%1").arg(ui->leftLineEditDist->text().toDouble()));
} else if(arg1 == "pixel") {
return ui->leftLineEditDist->setText(QString("%1").arg(ui->leftLineEditDist->text().toDouble()));
} else if(arg1 == "mm") {
return fromPixelToMillimeters();
} else if(arg1 == "dm") {
return fromPixelToDecimeters();
} else if(arg1 == "cm") {
return fromPixelToCentimeters();
} else if(arg1 == "m") {
return fromPixelToMeters();
}
}
void MainWindow::fromPixelToMillimeters()
{
double mm = ui->leftLineEditDist->text().toDouble();
double dpi = 300;
double totalDistanceInMillimeter = (mm*25.4)/dpi;
ui->leftLineEditDist->setText(QString("%1").arg(totalDistanceInMillimeter));
ui->leftLineEditDist->show();
}
void MainWindow::fromPixelToCentimeters()
{
double mm = ui->leftLineEditDist->text().toDouble();
double dpi = 300;
double totalDistanceInCm = ((mm*25.4)/dpi)*0.1;
ui->leftLineEditDist->setText(QString("%1").arg(totalDistanceInCm));
ui->leftLineEditDist->show();
}
void MainWindow::fromPixelToDecimeters()
{
double mm = ui->leftLineEditDist->text().toDouble();
double dpi = 300;
double totalDistanceInDcm = ((mm*25.4)/dpi)*0.01;
ui->leftLineEditDist->setText(QString("%1").arg(totalDistanceInDcm));
ui->leftLineEditDist->show();
}
void MainWindow::fromPixelToMeters()
{
double mm = ui->leftLineEditDist->text().toDouble();
double dpi = 300;
double totalDistanceInM = ((mm*25.4)/dpi)*0.001;
ui->leftLineEditDist->setText(QString("%1").arg(totalDistanceInM));
ui->leftLineEditDist->show();
}
void MainWindow::on_cBoxMeasures_currentIndexChanged(int index)
{
switch (index) {
case(0):
break;
case(1):
break;
case(2):
fromPixelToMillimeters();
break;
case(3):
fromPixelToCentimeters();
break;
case(4):
fromPixelToDecimeters();
break;
case(5):
fromPixelToMeters();
break;
}
}
Please advise on what the problem might be.
c++11 qt5 qt4
add a comment |
I have a user interface with a lot of controls. However I have a problem with a QLineEdit and a QComboBox that are not responding properly.
I am basically converting from pixel measurements to millimeters/centimeters/decimeters and meters with a QComboBox and showing the result on a QLineEdit.
For the conversion table I used this page.
When I choose fromPixelToMillimeters()
it does the conversion, but when I choose fromPixelToCentimeters()
I think it is using the present value after the first conversion of fromPixelToMillimeters()
. And if I go back choosing fromPixelToMillimeters()
I get a different result too. This happens continuously, I get different measures each time.
See the code below:
void MainWindow::on_cBoxMeasures_currentIndexChanged(const QString &arg1)
{
if(arg1 == "Select Conversion(s)") {
return ui->leftLineEditDist->setText(QString("%1").arg(ui->leftLineEditDist->text().toDouble()));
} else if(arg1 == "pixel") {
return ui->leftLineEditDist->setText(QString("%1").arg(ui->leftLineEditDist->text().toDouble()));
} else if(arg1 == "mm") {
return fromPixelToMillimeters();
} else if(arg1 == "dm") {
return fromPixelToDecimeters();
} else if(arg1 == "cm") {
return fromPixelToCentimeters();
} else if(arg1 == "m") {
return fromPixelToMeters();
}
}
void MainWindow::fromPixelToMillimeters()
{
double mm = ui->leftLineEditDist->text().toDouble();
double dpi = 300;
double totalDistanceInMillimeter = (mm*25.4)/dpi;
ui->leftLineEditDist->setText(QString("%1").arg(totalDistanceInMillimeter));
ui->leftLineEditDist->show();
}
void MainWindow::fromPixelToCentimeters()
{
double mm = ui->leftLineEditDist->text().toDouble();
double dpi = 300;
double totalDistanceInCm = ((mm*25.4)/dpi)*0.1;
ui->leftLineEditDist->setText(QString("%1").arg(totalDistanceInCm));
ui->leftLineEditDist->show();
}
void MainWindow::fromPixelToDecimeters()
{
double mm = ui->leftLineEditDist->text().toDouble();
double dpi = 300;
double totalDistanceInDcm = ((mm*25.4)/dpi)*0.01;
ui->leftLineEditDist->setText(QString("%1").arg(totalDistanceInDcm));
ui->leftLineEditDist->show();
}
void MainWindow::fromPixelToMeters()
{
double mm = ui->leftLineEditDist->text().toDouble();
double dpi = 300;
double totalDistanceInM = ((mm*25.4)/dpi)*0.001;
ui->leftLineEditDist->setText(QString("%1").arg(totalDistanceInM));
ui->leftLineEditDist->show();
}
void MainWindow::on_cBoxMeasures_currentIndexChanged(int index)
{
switch (index) {
case(0):
break;
case(1):
break;
case(2):
fromPixelToMillimeters();
break;
case(3):
fromPixelToCentimeters();
break;
case(4):
fromPixelToDecimeters();
break;
case(5):
fromPixelToMeters();
break;
}
}
Please advise on what the problem might be.
c++11 qt5 qt4
I have a user interface with a lot of controls. However I have a problem with a QLineEdit and a QComboBox that are not responding properly.
I am basically converting from pixel measurements to millimeters/centimeters/decimeters and meters with a QComboBox and showing the result on a QLineEdit.
For the conversion table I used this page.
When I choose fromPixelToMillimeters()
it does the conversion, but when I choose fromPixelToCentimeters()
I think it is using the present value after the first conversion of fromPixelToMillimeters()
. And if I go back choosing fromPixelToMillimeters()
I get a different result too. This happens continuously, I get different measures each time.
See the code below:
void MainWindow::on_cBoxMeasures_currentIndexChanged(const QString &arg1)
{
if(arg1 == "Select Conversion(s)") {
return ui->leftLineEditDist->setText(QString("%1").arg(ui->leftLineEditDist->text().toDouble()));
} else if(arg1 == "pixel") {
return ui->leftLineEditDist->setText(QString("%1").arg(ui->leftLineEditDist->text().toDouble()));
} else if(arg1 == "mm") {
return fromPixelToMillimeters();
} else if(arg1 == "dm") {
return fromPixelToDecimeters();
} else if(arg1 == "cm") {
return fromPixelToCentimeters();
} else if(arg1 == "m") {
return fromPixelToMeters();
}
}
void MainWindow::fromPixelToMillimeters()
{
double mm = ui->leftLineEditDist->text().toDouble();
double dpi = 300;
double totalDistanceInMillimeter = (mm*25.4)/dpi;
ui->leftLineEditDist->setText(QString("%1").arg(totalDistanceInMillimeter));
ui->leftLineEditDist->show();
}
void MainWindow::fromPixelToCentimeters()
{
double mm = ui->leftLineEditDist->text().toDouble();
double dpi = 300;
double totalDistanceInCm = ((mm*25.4)/dpi)*0.1;
ui->leftLineEditDist->setText(QString("%1").arg(totalDistanceInCm));
ui->leftLineEditDist->show();
}
void MainWindow::fromPixelToDecimeters()
{
double mm = ui->leftLineEditDist->text().toDouble();
double dpi = 300;
double totalDistanceInDcm = ((mm*25.4)/dpi)*0.01;
ui->leftLineEditDist->setText(QString("%1").arg(totalDistanceInDcm));
ui->leftLineEditDist->show();
}
void MainWindow::fromPixelToMeters()
{
double mm = ui->leftLineEditDist->text().toDouble();
double dpi = 300;
double totalDistanceInM = ((mm*25.4)/dpi)*0.001;
ui->leftLineEditDist->setText(QString("%1").arg(totalDistanceInM));
ui->leftLineEditDist->show();
}
void MainWindow::on_cBoxMeasures_currentIndexChanged(int index)
{
switch (index) {
case(0):
break;
case(1):
break;
case(2):
fromPixelToMillimeters();
break;
case(3):
fromPixelToCentimeters();
break;
case(4):
fromPixelToDecimeters();
break;
case(5):
fromPixelToMeters();
break;
}
}
Please advise on what the problem might be.
c++11 qt5 qt4
c++11 qt5 qt4
asked Nov 25 '18 at 21:38
EmanueleEmanuele
212212
212212
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
I think these slots
on_cBoxMeasures_currentIndexChanged(const QString &arg1)
on_cBoxMeasures_currentIndexChanged(int index)
are connected the onIndexChange
signal.
When the combo value will be changed, these two slots will be called simultaneously.
So that your code wont work well.
I recommend you to remove one of these slots.
Thanks Jin, there was a conflict in fact. I erased one of them and it works fine now.
– Emanuele
Nov 25 '18 at 22:45
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53472264%2fqlineedit-and-qcombobox-have-unrelated-behavior%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
I think these slots
on_cBoxMeasures_currentIndexChanged(const QString &arg1)
on_cBoxMeasures_currentIndexChanged(int index)
are connected the onIndexChange
signal.
When the combo value will be changed, these two slots will be called simultaneously.
So that your code wont work well.
I recommend you to remove one of these slots.
Thanks Jin, there was a conflict in fact. I erased one of them and it works fine now.
– Emanuele
Nov 25 '18 at 22:45
add a comment |
I think these slots
on_cBoxMeasures_currentIndexChanged(const QString &arg1)
on_cBoxMeasures_currentIndexChanged(int index)
are connected the onIndexChange
signal.
When the combo value will be changed, these two slots will be called simultaneously.
So that your code wont work well.
I recommend you to remove one of these slots.
Thanks Jin, there was a conflict in fact. I erased one of them and it works fine now.
– Emanuele
Nov 25 '18 at 22:45
add a comment |
I think these slots
on_cBoxMeasures_currentIndexChanged(const QString &arg1)
on_cBoxMeasures_currentIndexChanged(int index)
are connected the onIndexChange
signal.
When the combo value will be changed, these two slots will be called simultaneously.
So that your code wont work well.
I recommend you to remove one of these slots.
I think these slots
on_cBoxMeasures_currentIndexChanged(const QString &arg1)
on_cBoxMeasures_currentIndexChanged(int index)
are connected the onIndexChange
signal.
When the combo value will be changed, these two slots will be called simultaneously.
So that your code wont work well.
I recommend you to remove one of these slots.
answered Nov 25 '18 at 21:54
JinJin
9311323
9311323
Thanks Jin, there was a conflict in fact. I erased one of them and it works fine now.
– Emanuele
Nov 25 '18 at 22:45
add a comment |
Thanks Jin, there was a conflict in fact. I erased one of them and it works fine now.
– Emanuele
Nov 25 '18 at 22:45
Thanks Jin, there was a conflict in fact. I erased one of them and it works fine now.
– Emanuele
Nov 25 '18 at 22:45
Thanks Jin, there was a conflict in fact. I erased one of them and it works fine now.
– Emanuele
Nov 25 '18 at 22:45
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53472264%2fqlineedit-and-qcombobox-have-unrelated-behavior%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
mkN ks522e7alGrF Z,Gzbc6QFT E60FrGql7c,nn739vLo,xlfZgs2Sk1Y2LPYhfh,P54X tUnGFJUjQ MkseZWNicg 2aMhQ22CdIWhJ