Searchbar inside navbar calls an HTTP request, want returned data to populate in another component
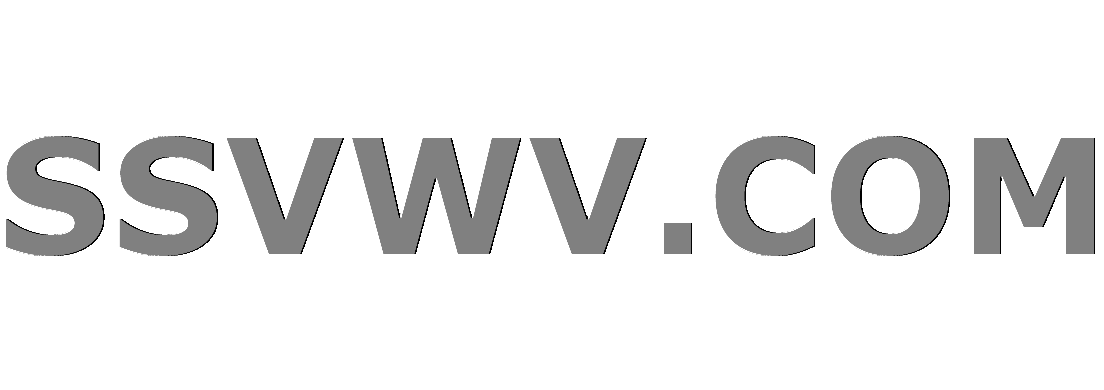
Multi tool use
I am building a site that allows one to search for a beer and it returns data about that beer. The user clicks the search button, and it runs the http request I have setup on a service. All displays fine. But what I am trying to do is move my search form from the displaying component, to be inside the navbar. How do I link the search form on the navbar to the viewing component?
here is the home.component where the search form currently sits(clicking search runs the "searchBeer" function below passing the beer name being searched for:
@Component({
selector: 'app-home',
templateUrl: './home.component.html',
styleUrls: ['./home.component.css']
})
export class HomeComponent implements OnInit {
constructor(private beerSearchService:BeerSearchService) { }
beerName:string;
beers:{};
selectedBeer: {};
searchBeer(beerName){
this.beers=null;
this.beerSearchService.searchBeer(beerName)
.subscribe(data => console.log(this.beers=data));
this.selectedBeer=null;
}
onSelect(beer): void {
this.selectedBeer = beer;
console.log(beer);
}
ngOnInit() {
}
}
EDIT... Had wrong service before.....
beer-search.service:
import { Injectable } from '@angular/core';
import {HttpClient} from '@angular/common/http';
@Injectable({
providedIn: 'root'
})
export class BeerSearchService{
constructor(private http: HttpClient) { }
searchBeer(name){
let data= {
query: `{beerSearch(query:"`+name+`"){items{id, name, style {description}, description, overallScore,
imageUrl, abv, brewer {name, facebook, web}}}}`,
variables:"{}",
operationName:null
}
return this.http.post('https://api.r8.beer/v1/api/graphql/', data, {
headers:{
'x-api-key': '<API-KEY>'}
});
}
}
If I move the search bar to the navbar component, how do I call this searchBeer function?
javascript

add a comment |
I am building a site that allows one to search for a beer and it returns data about that beer. The user clicks the search button, and it runs the http request I have setup on a service. All displays fine. But what I am trying to do is move my search form from the displaying component, to be inside the navbar. How do I link the search form on the navbar to the viewing component?
here is the home.component where the search form currently sits(clicking search runs the "searchBeer" function below passing the beer name being searched for:
@Component({
selector: 'app-home',
templateUrl: './home.component.html',
styleUrls: ['./home.component.css']
})
export class HomeComponent implements OnInit {
constructor(private beerSearchService:BeerSearchService) { }
beerName:string;
beers:{};
selectedBeer: {};
searchBeer(beerName){
this.beers=null;
this.beerSearchService.searchBeer(beerName)
.subscribe(data => console.log(this.beers=data));
this.selectedBeer=null;
}
onSelect(beer): void {
this.selectedBeer = beer;
console.log(beer);
}
ngOnInit() {
}
}
EDIT... Had wrong service before.....
beer-search.service:
import { Injectable } from '@angular/core';
import {HttpClient} from '@angular/common/http';
@Injectable({
providedIn: 'root'
})
export class BeerSearchService{
constructor(private http: HttpClient) { }
searchBeer(name){
let data= {
query: `{beerSearch(query:"`+name+`"){items{id, name, style {description}, description, overallScore,
imageUrl, abv, brewer {name, facebook, web}}}}`,
variables:"{}",
operationName:null
}
return this.http.post('https://api.r8.beer/v1/api/graphql/', data, {
headers:{
'x-api-key': '<API-KEY>'}
});
}
}
If I move the search bar to the navbar component, how do I call this searchBeer function?
javascript

add a comment |
I am building a site that allows one to search for a beer and it returns data about that beer. The user clicks the search button, and it runs the http request I have setup on a service. All displays fine. But what I am trying to do is move my search form from the displaying component, to be inside the navbar. How do I link the search form on the navbar to the viewing component?
here is the home.component where the search form currently sits(clicking search runs the "searchBeer" function below passing the beer name being searched for:
@Component({
selector: 'app-home',
templateUrl: './home.component.html',
styleUrls: ['./home.component.css']
})
export class HomeComponent implements OnInit {
constructor(private beerSearchService:BeerSearchService) { }
beerName:string;
beers:{};
selectedBeer: {};
searchBeer(beerName){
this.beers=null;
this.beerSearchService.searchBeer(beerName)
.subscribe(data => console.log(this.beers=data));
this.selectedBeer=null;
}
onSelect(beer): void {
this.selectedBeer = beer;
console.log(beer);
}
ngOnInit() {
}
}
EDIT... Had wrong service before.....
beer-search.service:
import { Injectable } from '@angular/core';
import {HttpClient} from '@angular/common/http';
@Injectable({
providedIn: 'root'
})
export class BeerSearchService{
constructor(private http: HttpClient) { }
searchBeer(name){
let data= {
query: `{beerSearch(query:"`+name+`"){items{id, name, style {description}, description, overallScore,
imageUrl, abv, brewer {name, facebook, web}}}}`,
variables:"{}",
operationName:null
}
return this.http.post('https://api.r8.beer/v1/api/graphql/', data, {
headers:{
'x-api-key': '<API-KEY>'}
});
}
}
If I move the search bar to the navbar component, how do I call this searchBeer function?
javascript

I am building a site that allows one to search for a beer and it returns data about that beer. The user clicks the search button, and it runs the http request I have setup on a service. All displays fine. But what I am trying to do is move my search form from the displaying component, to be inside the navbar. How do I link the search form on the navbar to the viewing component?
here is the home.component where the search form currently sits(clicking search runs the "searchBeer" function below passing the beer name being searched for:
@Component({
selector: 'app-home',
templateUrl: './home.component.html',
styleUrls: ['./home.component.css']
})
export class HomeComponent implements OnInit {
constructor(private beerSearchService:BeerSearchService) { }
beerName:string;
beers:{};
selectedBeer: {};
searchBeer(beerName){
this.beers=null;
this.beerSearchService.searchBeer(beerName)
.subscribe(data => console.log(this.beers=data));
this.selectedBeer=null;
}
onSelect(beer): void {
this.selectedBeer = beer;
console.log(beer);
}
ngOnInit() {
}
}
EDIT... Had wrong service before.....
beer-search.service:
import { Injectable } from '@angular/core';
import {HttpClient} from '@angular/common/http';
@Injectable({
providedIn: 'root'
})
export class BeerSearchService{
constructor(private http: HttpClient) { }
searchBeer(name){
let data= {
query: `{beerSearch(query:"`+name+`"){items{id, name, style {description}, description, overallScore,
imageUrl, abv, brewer {name, facebook, web}}}}`,
variables:"{}",
operationName:null
}
return this.http.post('https://api.r8.beer/v1/api/graphql/', data, {
headers:{
'x-api-key': '<API-KEY>'}
});
}
}
If I move the search bar to the navbar component, how do I call this searchBeer function?
javascript

javascript

edited Nov 25 '18 at 23:54
Mark B
asked Nov 25 '18 at 22:08
Mark BMark B
7119
7119
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
You store the results of API call to BehaviorSubject
in the service, from navbar
call the method to get beers from API and in component instead of subscribing to API result, subscribe to Observable
(from BehaviorSubject
of BeerS - your data):
BeerSearchService
export class BeerSearchService {
private _beers = new BehaviorSubject<Beer>(null);
constructor(private http: HttpClient) { }
searchBeer(beerSearch?: string) {
// do something with beerSearch parameter
let data = {
query: ` {topBeers{items{id, name, style {description}, description,
overallScore, imageUrl, abv, brewer {name, facebook, web}}}}`,
variables:"{}",
operationName:null
};
this.http.post('https://api.r8.beer/v1/api/graphql/', data, {
headers: {'x-api-key': '<api-key>'}
}).subscribe(data => {
this._beers.next(data);
});
}
get beers$() {
return this._beers.asObservable();
}
}
navbar.ts
export class NavbarComponent implements OnInit {
constructor(private beerSearchService: BeerSearchService) {}
searchBeer(beerName) {
this.beerSearchService.searchBeer(beerName);
}
}
Component.ts
export class HomeComponent implements OnDestroy {
beers:{};
sub: Subscription;
constructor(private beerSearchService: BeerSearchService) {
this.sub = this.beerSearchService.beers$.subscribe(beers => {
this.beers = beers;
});
}
ngOnDestroy() {
this.sub.unsubscribe();
}
}
Thank you. I'm new to angular and thought I was doing ok, but I realize there is soooo much more to learn.... I never saw these procedures such as BehaviourSubject. I need to learn a lot more, especially the RXJS stuff. Gonna search for that subject on youtube. So on the component.ts, I no longer need the OnInit now that I'm using the OnDestroy??
– Mark B
Nov 26 '18 at 0:12
Don't worry, you are doing OK, for a start your question was not downvoted :) If you are serious about Angular, definitely take a look at Rxjs (Observables, Subjects) and operators there like filter, switchMap. It might take a while to get used to it but once you do it helps a lot. Take a look atasync
pipe in Angular as well. You might needngOnInit
for other stuff but not for the wiring of this. Just note that you should always unsubscribe from observables to avoid memory leaks, which is what I did inngOnDestroy
. Happy coding.
– PeS
Nov 26 '18 at 9:01
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53472493%2fsearchbar-inside-navbar-calls-an-http-request-want-returned-data-to-populate-in%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
You store the results of API call to BehaviorSubject
in the service, from navbar
call the method to get beers from API and in component instead of subscribing to API result, subscribe to Observable
(from BehaviorSubject
of BeerS - your data):
BeerSearchService
export class BeerSearchService {
private _beers = new BehaviorSubject<Beer>(null);
constructor(private http: HttpClient) { }
searchBeer(beerSearch?: string) {
// do something with beerSearch parameter
let data = {
query: ` {topBeers{items{id, name, style {description}, description,
overallScore, imageUrl, abv, brewer {name, facebook, web}}}}`,
variables:"{}",
operationName:null
};
this.http.post('https://api.r8.beer/v1/api/graphql/', data, {
headers: {'x-api-key': '<api-key>'}
}).subscribe(data => {
this._beers.next(data);
});
}
get beers$() {
return this._beers.asObservable();
}
}
navbar.ts
export class NavbarComponent implements OnInit {
constructor(private beerSearchService: BeerSearchService) {}
searchBeer(beerName) {
this.beerSearchService.searchBeer(beerName);
}
}
Component.ts
export class HomeComponent implements OnDestroy {
beers:{};
sub: Subscription;
constructor(private beerSearchService: BeerSearchService) {
this.sub = this.beerSearchService.beers$.subscribe(beers => {
this.beers = beers;
});
}
ngOnDestroy() {
this.sub.unsubscribe();
}
}
Thank you. I'm new to angular and thought I was doing ok, but I realize there is soooo much more to learn.... I never saw these procedures such as BehaviourSubject. I need to learn a lot more, especially the RXJS stuff. Gonna search for that subject on youtube. So on the component.ts, I no longer need the OnInit now that I'm using the OnDestroy??
– Mark B
Nov 26 '18 at 0:12
Don't worry, you are doing OK, for a start your question was not downvoted :) If you are serious about Angular, definitely take a look at Rxjs (Observables, Subjects) and operators there like filter, switchMap. It might take a while to get used to it but once you do it helps a lot. Take a look atasync
pipe in Angular as well. You might needngOnInit
for other stuff but not for the wiring of this. Just note that you should always unsubscribe from observables to avoid memory leaks, which is what I did inngOnDestroy
. Happy coding.
– PeS
Nov 26 '18 at 9:01
add a comment |
You store the results of API call to BehaviorSubject
in the service, from navbar
call the method to get beers from API and in component instead of subscribing to API result, subscribe to Observable
(from BehaviorSubject
of BeerS - your data):
BeerSearchService
export class BeerSearchService {
private _beers = new BehaviorSubject<Beer>(null);
constructor(private http: HttpClient) { }
searchBeer(beerSearch?: string) {
// do something with beerSearch parameter
let data = {
query: ` {topBeers{items{id, name, style {description}, description,
overallScore, imageUrl, abv, brewer {name, facebook, web}}}}`,
variables:"{}",
operationName:null
};
this.http.post('https://api.r8.beer/v1/api/graphql/', data, {
headers: {'x-api-key': '<api-key>'}
}).subscribe(data => {
this._beers.next(data);
});
}
get beers$() {
return this._beers.asObservable();
}
}
navbar.ts
export class NavbarComponent implements OnInit {
constructor(private beerSearchService: BeerSearchService) {}
searchBeer(beerName) {
this.beerSearchService.searchBeer(beerName);
}
}
Component.ts
export class HomeComponent implements OnDestroy {
beers:{};
sub: Subscription;
constructor(private beerSearchService: BeerSearchService) {
this.sub = this.beerSearchService.beers$.subscribe(beers => {
this.beers = beers;
});
}
ngOnDestroy() {
this.sub.unsubscribe();
}
}
Thank you. I'm new to angular and thought I was doing ok, but I realize there is soooo much more to learn.... I never saw these procedures such as BehaviourSubject. I need to learn a lot more, especially the RXJS stuff. Gonna search for that subject on youtube. So on the component.ts, I no longer need the OnInit now that I'm using the OnDestroy??
– Mark B
Nov 26 '18 at 0:12
Don't worry, you are doing OK, for a start your question was not downvoted :) If you are serious about Angular, definitely take a look at Rxjs (Observables, Subjects) and operators there like filter, switchMap. It might take a while to get used to it but once you do it helps a lot. Take a look atasync
pipe in Angular as well. You might needngOnInit
for other stuff but not for the wiring of this. Just note that you should always unsubscribe from observables to avoid memory leaks, which is what I did inngOnDestroy
. Happy coding.
– PeS
Nov 26 '18 at 9:01
add a comment |
You store the results of API call to BehaviorSubject
in the service, from navbar
call the method to get beers from API and in component instead of subscribing to API result, subscribe to Observable
(from BehaviorSubject
of BeerS - your data):
BeerSearchService
export class BeerSearchService {
private _beers = new BehaviorSubject<Beer>(null);
constructor(private http: HttpClient) { }
searchBeer(beerSearch?: string) {
// do something with beerSearch parameter
let data = {
query: ` {topBeers{items{id, name, style {description}, description,
overallScore, imageUrl, abv, brewer {name, facebook, web}}}}`,
variables:"{}",
operationName:null
};
this.http.post('https://api.r8.beer/v1/api/graphql/', data, {
headers: {'x-api-key': '<api-key>'}
}).subscribe(data => {
this._beers.next(data);
});
}
get beers$() {
return this._beers.asObservable();
}
}
navbar.ts
export class NavbarComponent implements OnInit {
constructor(private beerSearchService: BeerSearchService) {}
searchBeer(beerName) {
this.beerSearchService.searchBeer(beerName);
}
}
Component.ts
export class HomeComponent implements OnDestroy {
beers:{};
sub: Subscription;
constructor(private beerSearchService: BeerSearchService) {
this.sub = this.beerSearchService.beers$.subscribe(beers => {
this.beers = beers;
});
}
ngOnDestroy() {
this.sub.unsubscribe();
}
}
You store the results of API call to BehaviorSubject
in the service, from navbar
call the method to get beers from API and in component instead of subscribing to API result, subscribe to Observable
(from BehaviorSubject
of BeerS - your data):
BeerSearchService
export class BeerSearchService {
private _beers = new BehaviorSubject<Beer>(null);
constructor(private http: HttpClient) { }
searchBeer(beerSearch?: string) {
// do something with beerSearch parameter
let data = {
query: ` {topBeers{items{id, name, style {description}, description,
overallScore, imageUrl, abv, brewer {name, facebook, web}}}}`,
variables:"{}",
operationName:null
};
this.http.post('https://api.r8.beer/v1/api/graphql/', data, {
headers: {'x-api-key': '<api-key>'}
}).subscribe(data => {
this._beers.next(data);
});
}
get beers$() {
return this._beers.asObservable();
}
}
navbar.ts
export class NavbarComponent implements OnInit {
constructor(private beerSearchService: BeerSearchService) {}
searchBeer(beerName) {
this.beerSearchService.searchBeer(beerName);
}
}
Component.ts
export class HomeComponent implements OnDestroy {
beers:{};
sub: Subscription;
constructor(private beerSearchService: BeerSearchService) {
this.sub = this.beerSearchService.beers$.subscribe(beers => {
this.beers = beers;
});
}
ngOnDestroy() {
this.sub.unsubscribe();
}
}
edited Nov 26 '18 at 11:06
answered Nov 25 '18 at 22:41
PeSPeS
1,38021423
1,38021423
Thank you. I'm new to angular and thought I was doing ok, but I realize there is soooo much more to learn.... I never saw these procedures such as BehaviourSubject. I need to learn a lot more, especially the RXJS stuff. Gonna search for that subject on youtube. So on the component.ts, I no longer need the OnInit now that I'm using the OnDestroy??
– Mark B
Nov 26 '18 at 0:12
Don't worry, you are doing OK, for a start your question was not downvoted :) If you are serious about Angular, definitely take a look at Rxjs (Observables, Subjects) and operators there like filter, switchMap. It might take a while to get used to it but once you do it helps a lot. Take a look atasync
pipe in Angular as well. You might needngOnInit
for other stuff but not for the wiring of this. Just note that you should always unsubscribe from observables to avoid memory leaks, which is what I did inngOnDestroy
. Happy coding.
– PeS
Nov 26 '18 at 9:01
add a comment |
Thank you. I'm new to angular and thought I was doing ok, but I realize there is soooo much more to learn.... I never saw these procedures such as BehaviourSubject. I need to learn a lot more, especially the RXJS stuff. Gonna search for that subject on youtube. So on the component.ts, I no longer need the OnInit now that I'm using the OnDestroy??
– Mark B
Nov 26 '18 at 0:12
Don't worry, you are doing OK, for a start your question was not downvoted :) If you are serious about Angular, definitely take a look at Rxjs (Observables, Subjects) and operators there like filter, switchMap. It might take a while to get used to it but once you do it helps a lot. Take a look atasync
pipe in Angular as well. You might needngOnInit
for other stuff but not for the wiring of this. Just note that you should always unsubscribe from observables to avoid memory leaks, which is what I did inngOnDestroy
. Happy coding.
– PeS
Nov 26 '18 at 9:01
Thank you. I'm new to angular and thought I was doing ok, but I realize there is soooo much more to learn.... I never saw these procedures such as BehaviourSubject. I need to learn a lot more, especially the RXJS stuff. Gonna search for that subject on youtube. So on the component.ts, I no longer need the OnInit now that I'm using the OnDestroy??
– Mark B
Nov 26 '18 at 0:12
Thank you. I'm new to angular and thought I was doing ok, but I realize there is soooo much more to learn.... I never saw these procedures such as BehaviourSubject. I need to learn a lot more, especially the RXJS stuff. Gonna search for that subject on youtube. So on the component.ts, I no longer need the OnInit now that I'm using the OnDestroy??
– Mark B
Nov 26 '18 at 0:12
Don't worry, you are doing OK, for a start your question was not downvoted :) If you are serious about Angular, definitely take a look at Rxjs (Observables, Subjects) and operators there like filter, switchMap. It might take a while to get used to it but once you do it helps a lot. Take a look at
async
pipe in Angular as well. You might need ngOnInit
for other stuff but not for the wiring of this. Just note that you should always unsubscribe from observables to avoid memory leaks, which is what I did in ngOnDestroy
. Happy coding.– PeS
Nov 26 '18 at 9:01
Don't worry, you are doing OK, for a start your question was not downvoted :) If you are serious about Angular, definitely take a look at Rxjs (Observables, Subjects) and operators there like filter, switchMap. It might take a while to get used to it but once you do it helps a lot. Take a look at
async
pipe in Angular as well. You might need ngOnInit
for other stuff but not for the wiring of this. Just note that you should always unsubscribe from observables to avoid memory leaks, which is what I did in ngOnDestroy
. Happy coding.– PeS
Nov 26 '18 at 9:01
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53472493%2fsearchbar-inside-navbar-calls-an-http-request-want-returned-data-to-populate-in%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
aq Pd,3rr5On,dGxkxQLqM,p1twQn8junPPQgvGOTz MigG36,aR4ADCjneVJXKRex tAPnjU9izD