How can I return an observable in Angular 7?
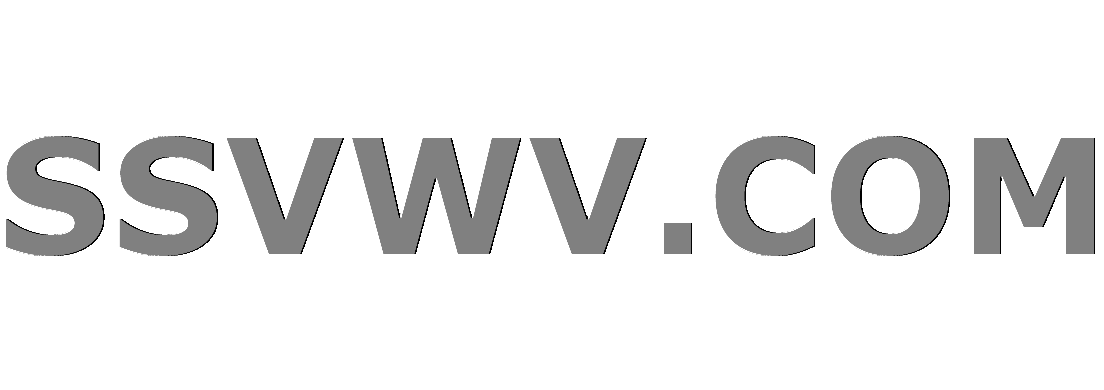
Multi tool use
up vote
0
down vote
favorite
I had a project that was previously on Angular 5.x and I updated it to 7. There is a particular instance where I need to return an httpClient observable but I can't figure out how it changed in Angular 7. It's giving me an error on the "catch" of the callApi function. [ts] Property 'catch' does not exist on type 'Observable'. [2339] is my error
callApi(url: string): Observable<any> {
return this.httpClient
.get(url)
.catch(this.handleError);
}
This is all of the code in my config.service.ts file.
import { Injectable } from '@angular/core';
import { HttpClient, HttpHeaders } from '@angular/common/http';
import { Observable } from 'rxjs/Observable';
import 'rxjs/add/observable/forkJoin';
import 'rxjs/add/operator/catch';
import 'rxjs/add/operator/map';
import 'rxjs/add/observable/throw';
import { environment } from './../environments/environment';
@Injectable()
export class ConfigService {
private configUrl = environment.production ? './assets/config/config.prod.json' : './assets/config/config.dev.json';
private _config: any;
constructor(private httpClient: HttpClient) {
}
load(): Promise<any> {
let promise: Promise<any> = new Promise((resolve: any) => {
this.callApi(this.configUrl)
.subscribe(config => {
this._config = config;
resolve(true)
});
});
return promise;
}
getConfig(key: any) {
return this._config[key];
}
private handleError(error: Response | any) {
let errMsg: string;
if (error instanceof Response) {
let body = {}
try {
body = error.json();
} catch (e) {
}
const err = body['error'] || JSON.stringify(body);
errMsg = `${error.status} - ${error.statusText || ''} ${err}`;
} else {
errMsg = error.message ? error.message : error.toString();
}
console.error(errMsg);
return Observable.throw(errMsg);
}
callApi(url: string): Observable<any> {
return this.httpClient
.get(url)
.catch(this.handleError);
}
}
angular angular7
add a comment |
up vote
0
down vote
favorite
I had a project that was previously on Angular 5.x and I updated it to 7. There is a particular instance where I need to return an httpClient observable but I can't figure out how it changed in Angular 7. It's giving me an error on the "catch" of the callApi function. [ts] Property 'catch' does not exist on type 'Observable'. [2339] is my error
callApi(url: string): Observable<any> {
return this.httpClient
.get(url)
.catch(this.handleError);
}
This is all of the code in my config.service.ts file.
import { Injectable } from '@angular/core';
import { HttpClient, HttpHeaders } from '@angular/common/http';
import { Observable } from 'rxjs/Observable';
import 'rxjs/add/observable/forkJoin';
import 'rxjs/add/operator/catch';
import 'rxjs/add/operator/map';
import 'rxjs/add/observable/throw';
import { environment } from './../environments/environment';
@Injectable()
export class ConfigService {
private configUrl = environment.production ? './assets/config/config.prod.json' : './assets/config/config.dev.json';
private _config: any;
constructor(private httpClient: HttpClient) {
}
load(): Promise<any> {
let promise: Promise<any> = new Promise((resolve: any) => {
this.callApi(this.configUrl)
.subscribe(config => {
this._config = config;
resolve(true)
});
});
return promise;
}
getConfig(key: any) {
return this._config[key];
}
private handleError(error: Response | any) {
let errMsg: string;
if (error instanceof Response) {
let body = {}
try {
body = error.json();
} catch (e) {
}
const err = body['error'] || JSON.stringify(body);
errMsg = `${error.status} - ${error.statusText || ''} ${err}`;
} else {
errMsg = error.message ? error.message : error.toString();
}
console.error(errMsg);
return Observable.throw(errMsg);
}
callApi(url: string): Observable<any> {
return this.httpClient
.get(url)
.catch(this.handleError);
}
}
angular angular7
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I had a project that was previously on Angular 5.x and I updated it to 7. There is a particular instance where I need to return an httpClient observable but I can't figure out how it changed in Angular 7. It's giving me an error on the "catch" of the callApi function. [ts] Property 'catch' does not exist on type 'Observable'. [2339] is my error
callApi(url: string): Observable<any> {
return this.httpClient
.get(url)
.catch(this.handleError);
}
This is all of the code in my config.service.ts file.
import { Injectable } from '@angular/core';
import { HttpClient, HttpHeaders } from '@angular/common/http';
import { Observable } from 'rxjs/Observable';
import 'rxjs/add/observable/forkJoin';
import 'rxjs/add/operator/catch';
import 'rxjs/add/operator/map';
import 'rxjs/add/observable/throw';
import { environment } from './../environments/environment';
@Injectable()
export class ConfigService {
private configUrl = environment.production ? './assets/config/config.prod.json' : './assets/config/config.dev.json';
private _config: any;
constructor(private httpClient: HttpClient) {
}
load(): Promise<any> {
let promise: Promise<any> = new Promise((resolve: any) => {
this.callApi(this.configUrl)
.subscribe(config => {
this._config = config;
resolve(true)
});
});
return promise;
}
getConfig(key: any) {
return this._config[key];
}
private handleError(error: Response | any) {
let errMsg: string;
if (error instanceof Response) {
let body = {}
try {
body = error.json();
} catch (e) {
}
const err = body['error'] || JSON.stringify(body);
errMsg = `${error.status} - ${error.statusText || ''} ${err}`;
} else {
errMsg = error.message ? error.message : error.toString();
}
console.error(errMsg);
return Observable.throw(errMsg);
}
callApi(url: string): Observable<any> {
return this.httpClient
.get(url)
.catch(this.handleError);
}
}
angular angular7
I had a project that was previously on Angular 5.x and I updated it to 7. There is a particular instance where I need to return an httpClient observable but I can't figure out how it changed in Angular 7. It's giving me an error on the "catch" of the callApi function. [ts] Property 'catch' does not exist on type 'Observable'. [2339] is my error
callApi(url: string): Observable<any> {
return this.httpClient
.get(url)
.catch(this.handleError);
}
This is all of the code in my config.service.ts file.
import { Injectable } from '@angular/core';
import { HttpClient, HttpHeaders } from '@angular/common/http';
import { Observable } from 'rxjs/Observable';
import 'rxjs/add/observable/forkJoin';
import 'rxjs/add/operator/catch';
import 'rxjs/add/operator/map';
import 'rxjs/add/observable/throw';
import { environment } from './../environments/environment';
@Injectable()
export class ConfigService {
private configUrl = environment.production ? './assets/config/config.prod.json' : './assets/config/config.dev.json';
private _config: any;
constructor(private httpClient: HttpClient) {
}
load(): Promise<any> {
let promise: Promise<any> = new Promise((resolve: any) => {
this.callApi(this.configUrl)
.subscribe(config => {
this._config = config;
resolve(true)
});
});
return promise;
}
getConfig(key: any) {
return this._config[key];
}
private handleError(error: Response | any) {
let errMsg: string;
if (error instanceof Response) {
let body = {}
try {
body = error.json();
} catch (e) {
}
const err = body['error'] || JSON.stringify(body);
errMsg = `${error.status} - ${error.statusText || ''} ${err}`;
} else {
errMsg = error.message ? error.message : error.toString();
}
console.error(errMsg);
return Observable.throw(errMsg);
}
callApi(url: string): Observable<any> {
return this.httpClient
.get(url)
.catch(this.handleError);
}
}
angular angular7
angular angular7
asked Nov 20 at 1:38
Keithers
5411
5411
add a comment |
add a comment |
3 Answers
3
active
oldest
votes
up vote
2
down vote
accepted
Update your rxjs
library to higher version and change the following code - You need use pipe
operators from rxjs
that might solve your catchError()
issue - change your catch
as catchError
Try something like this
callApi(url: string): Observable<any> {
return this.httpClient
.get(url).pipe(catchError(this.handleError));
}
Hope this helps - Happy coding :)
add a comment |
up vote
1
down vote
In addition to using catchError
rather than catch
, because HttpClient returns an Observable, consider that a subscription to an Observable has 3 callback parameters corresponding to the success events, the error events, and then a completion event when the connection is finished what it was doing (really you can ignore this one most of the time). So, your code could look like this:
config.service.ts
callApi(url: string): Observable<any> {
return this.httpClient
.get(url)
.pipe(catchError(this.handleError)); // adjust handleError function, as necessary
}
some.component.ts
this.configService.callApi('https://google.com')
.subscribe(
goodResult => console.log(goodResult),
error => console.log(error),
() => console.log('the api call is done!')
)
add a comment |
up vote
1
down vote
Angular 6 went from RxJs 5 to RxJs 6 and there are a few breaking changes.
catch has been renamed to catchError
https://www.academind.com/learn/javascript/rxjs-6-what-changed/
There is a compatibility library you can install for when you upgrade from RxJs 5 to 6 but it is better to modify your code to be RxJs 6 compatible.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53385034%2fhow-can-i-return-an-observable-in-angular-7%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
2
down vote
accepted
Update your rxjs
library to higher version and change the following code - You need use pipe
operators from rxjs
that might solve your catchError()
issue - change your catch
as catchError
Try something like this
callApi(url: string): Observable<any> {
return this.httpClient
.get(url).pipe(catchError(this.handleError));
}
Hope this helps - Happy coding :)
add a comment |
up vote
2
down vote
accepted
Update your rxjs
library to higher version and change the following code - You need use pipe
operators from rxjs
that might solve your catchError()
issue - change your catch
as catchError
Try something like this
callApi(url: string): Observable<any> {
return this.httpClient
.get(url).pipe(catchError(this.handleError));
}
Hope this helps - Happy coding :)
add a comment |
up vote
2
down vote
accepted
up vote
2
down vote
accepted
Update your rxjs
library to higher version and change the following code - You need use pipe
operators from rxjs
that might solve your catchError()
issue - change your catch
as catchError
Try something like this
callApi(url: string): Observable<any> {
return this.httpClient
.get(url).pipe(catchError(this.handleError));
}
Hope this helps - Happy coding :)
Update your rxjs
library to higher version and change the following code - You need use pipe
operators from rxjs
that might solve your catchError()
issue - change your catch
as catchError
Try something like this
callApi(url: string): Observable<any> {
return this.httpClient
.get(url).pipe(catchError(this.handleError));
}
Hope this helps - Happy coding :)
answered Nov 20 at 1:50


Rahul
9631315
9631315
add a comment |
add a comment |
up vote
1
down vote
In addition to using catchError
rather than catch
, because HttpClient returns an Observable, consider that a subscription to an Observable has 3 callback parameters corresponding to the success events, the error events, and then a completion event when the connection is finished what it was doing (really you can ignore this one most of the time). So, your code could look like this:
config.service.ts
callApi(url: string): Observable<any> {
return this.httpClient
.get(url)
.pipe(catchError(this.handleError)); // adjust handleError function, as necessary
}
some.component.ts
this.configService.callApi('https://google.com')
.subscribe(
goodResult => console.log(goodResult),
error => console.log(error),
() => console.log('the api call is done!')
)
add a comment |
up vote
1
down vote
In addition to using catchError
rather than catch
, because HttpClient returns an Observable, consider that a subscription to an Observable has 3 callback parameters corresponding to the success events, the error events, and then a completion event when the connection is finished what it was doing (really you can ignore this one most of the time). So, your code could look like this:
config.service.ts
callApi(url: string): Observable<any> {
return this.httpClient
.get(url)
.pipe(catchError(this.handleError)); // adjust handleError function, as necessary
}
some.component.ts
this.configService.callApi('https://google.com')
.subscribe(
goodResult => console.log(goodResult),
error => console.log(error),
() => console.log('the api call is done!')
)
add a comment |
up vote
1
down vote
up vote
1
down vote
In addition to using catchError
rather than catch
, because HttpClient returns an Observable, consider that a subscription to an Observable has 3 callback parameters corresponding to the success events, the error events, and then a completion event when the connection is finished what it was doing (really you can ignore this one most of the time). So, your code could look like this:
config.service.ts
callApi(url: string): Observable<any> {
return this.httpClient
.get(url)
.pipe(catchError(this.handleError)); // adjust handleError function, as necessary
}
some.component.ts
this.configService.callApi('https://google.com')
.subscribe(
goodResult => console.log(goodResult),
error => console.log(error),
() => console.log('the api call is done!')
)
In addition to using catchError
rather than catch
, because HttpClient returns an Observable, consider that a subscription to an Observable has 3 callback parameters corresponding to the success events, the error events, and then a completion event when the connection is finished what it was doing (really you can ignore this one most of the time). So, your code could look like this:
config.service.ts
callApi(url: string): Observable<any> {
return this.httpClient
.get(url)
.pipe(catchError(this.handleError)); // adjust handleError function, as necessary
}
some.component.ts
this.configService.callApi('https://google.com')
.subscribe(
goodResult => console.log(goodResult),
error => console.log(error),
() => console.log('the api call is done!')
)
answered Nov 20 at 1:53


Ben Steward
905313
905313
add a comment |
add a comment |
up vote
1
down vote
Angular 6 went from RxJs 5 to RxJs 6 and there are a few breaking changes.
catch has been renamed to catchError
https://www.academind.com/learn/javascript/rxjs-6-what-changed/
There is a compatibility library you can install for when you upgrade from RxJs 5 to 6 but it is better to modify your code to be RxJs 6 compatible.
add a comment |
up vote
1
down vote
Angular 6 went from RxJs 5 to RxJs 6 and there are a few breaking changes.
catch has been renamed to catchError
https://www.academind.com/learn/javascript/rxjs-6-what-changed/
There is a compatibility library you can install for when you upgrade from RxJs 5 to 6 but it is better to modify your code to be RxJs 6 compatible.
add a comment |
up vote
1
down vote
up vote
1
down vote
Angular 6 went from RxJs 5 to RxJs 6 and there are a few breaking changes.
catch has been renamed to catchError
https://www.academind.com/learn/javascript/rxjs-6-what-changed/
There is a compatibility library you can install for when you upgrade from RxJs 5 to 6 but it is better to modify your code to be RxJs 6 compatible.
Angular 6 went from RxJs 5 to RxJs 6 and there are a few breaking changes.
catch has been renamed to catchError
https://www.academind.com/learn/javascript/rxjs-6-what-changed/
There is a compatibility library you can install for when you upgrade from RxJs 5 to 6 but it is better to modify your code to be RxJs 6 compatible.
edited Nov 20 at 3:11
answered Nov 20 at 1:46
Adrian Brand
2,87511018
2,87511018
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53385034%2fhow-can-i-return-an-observable-in-angular-7%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
zEk 3nFeiWp8XRUYwwWrZf