How to pass multiple DB data into jQuery's Autocomplete (AJAX) in Laravel 5.7
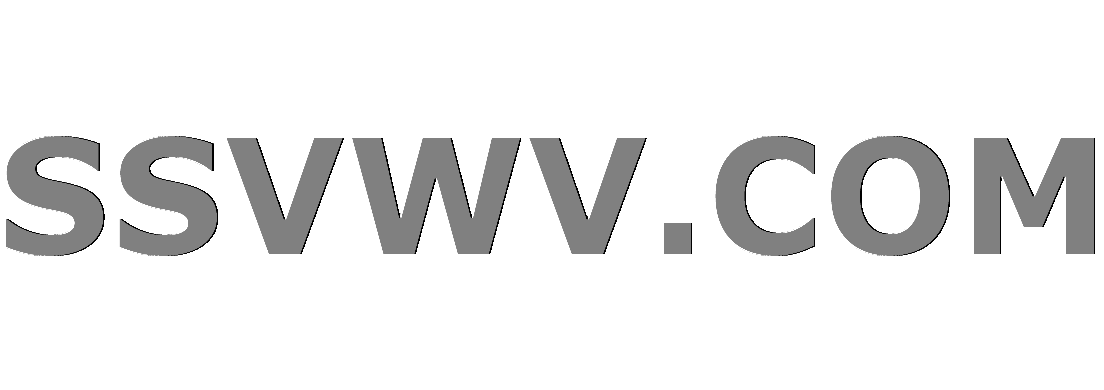
Multi tool use
up vote
0
down vote
favorite
I'm using jQuery's Autocomplete to get data while the user is typing. It works; I get some zipcode that matches the user's input.
The thing is that I want to pass more than the zipcode, like the neighborhood associated with that zipcode. Both are in the same table.
I want to pass the zipcode AND the neighborhood in the autocomplete.
Controller:
<?php
namespace Grupo_VillanuevaHttpControllers;
use IlluminateHttpRequest;
use IlluminateSupportFacadesDB;
use IlluminateSupportFacadesResponse;
class SearchController extends Controller
{
public function buscarCp(Request $request)
{
$term = $request->input('term');
$results = ;
$queries = DB::connection('db_postalcodes')
->table('postal_code')
->where('postal_code', 'LIKE', '%' . $term . '%') //search 'postal_code' column
->take(10)
->get(); // get 5 results
foreach ($queries as $query) {
$results = [
'postal_code' => $query->postal_code,
'value' => $query->postal_code,
'colonia' => $query->neighborhood];
}
return Response::json($results);
}
}
I thought about fetching it with the colonia
inside the associative array, though I'm not an expert.
JS:
@if (Request::is('admin/posts/create'))
{{-- <script src="https://cdnjs.cloudflare.com/ajax/libs/select2/4.0.6-rc.0/js/select2.min.js"></script> --}}
<script src="https://code.jquery.com/jquery-1.12.4.js"></script>
<script src="https://code.jquery.com/ui/1.12.1/jquery-ui.js"></script>
<script>
$('.postalCode').autocomplete({
source: "{{ route('busqueda-cp') }}",
minLength: 3,
select: function (event, ui) {
$('#postalCode').val(ui.item.value);
}
});
</script>
@else
<!-- No se cargó select2-->
@endif
Route:
// Aquí se guarda la función que hace, vía ajax, búsqueda de código postal.
Route::get('/buscarCp', 'SearchController@buscarCp')->name('busqueda-cp');
javascript php jquery laravel autocomplete
add a comment |
up vote
0
down vote
favorite
I'm using jQuery's Autocomplete to get data while the user is typing. It works; I get some zipcode that matches the user's input.
The thing is that I want to pass more than the zipcode, like the neighborhood associated with that zipcode. Both are in the same table.
I want to pass the zipcode AND the neighborhood in the autocomplete.
Controller:
<?php
namespace Grupo_VillanuevaHttpControllers;
use IlluminateHttpRequest;
use IlluminateSupportFacadesDB;
use IlluminateSupportFacadesResponse;
class SearchController extends Controller
{
public function buscarCp(Request $request)
{
$term = $request->input('term');
$results = ;
$queries = DB::connection('db_postalcodes')
->table('postal_code')
->where('postal_code', 'LIKE', '%' . $term . '%') //search 'postal_code' column
->take(10)
->get(); // get 5 results
foreach ($queries as $query) {
$results = [
'postal_code' => $query->postal_code,
'value' => $query->postal_code,
'colonia' => $query->neighborhood];
}
return Response::json($results);
}
}
I thought about fetching it with the colonia
inside the associative array, though I'm not an expert.
JS:
@if (Request::is('admin/posts/create'))
{{-- <script src="https://cdnjs.cloudflare.com/ajax/libs/select2/4.0.6-rc.0/js/select2.min.js"></script> --}}
<script src="https://code.jquery.com/jquery-1.12.4.js"></script>
<script src="https://code.jquery.com/ui/1.12.1/jquery-ui.js"></script>
<script>
$('.postalCode').autocomplete({
source: "{{ route('busqueda-cp') }}",
minLength: 3,
select: function (event, ui) {
$('#postalCode').val(ui.item.value);
}
});
</script>
@else
<!-- No se cargó select2-->
@endif
Route:
// Aquí se guarda la función que hace, vía ajax, búsqueda de código postal.
Route::get('/buscarCp', 'SearchController@buscarCp')->name('busqueda-cp');
javascript php jquery laravel autocomplete
1
Do you mean you want to append the neighborhood to the zip code? In your$results
you can do this:'value' => $query->postal_code . ' ' . $query->neighborhood,
– newUserName02
Nov 20 at 2:09
@newUserName02 Works. Thanks. Questions: So could I append any number of data that way?. Also, answer the question so I can select you as the winner!
– dawn
Nov 20 at 2:12
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I'm using jQuery's Autocomplete to get data while the user is typing. It works; I get some zipcode that matches the user's input.
The thing is that I want to pass more than the zipcode, like the neighborhood associated with that zipcode. Both are in the same table.
I want to pass the zipcode AND the neighborhood in the autocomplete.
Controller:
<?php
namespace Grupo_VillanuevaHttpControllers;
use IlluminateHttpRequest;
use IlluminateSupportFacadesDB;
use IlluminateSupportFacadesResponse;
class SearchController extends Controller
{
public function buscarCp(Request $request)
{
$term = $request->input('term');
$results = ;
$queries = DB::connection('db_postalcodes')
->table('postal_code')
->where('postal_code', 'LIKE', '%' . $term . '%') //search 'postal_code' column
->take(10)
->get(); // get 5 results
foreach ($queries as $query) {
$results = [
'postal_code' => $query->postal_code,
'value' => $query->postal_code,
'colonia' => $query->neighborhood];
}
return Response::json($results);
}
}
I thought about fetching it with the colonia
inside the associative array, though I'm not an expert.
JS:
@if (Request::is('admin/posts/create'))
{{-- <script src="https://cdnjs.cloudflare.com/ajax/libs/select2/4.0.6-rc.0/js/select2.min.js"></script> --}}
<script src="https://code.jquery.com/jquery-1.12.4.js"></script>
<script src="https://code.jquery.com/ui/1.12.1/jquery-ui.js"></script>
<script>
$('.postalCode').autocomplete({
source: "{{ route('busqueda-cp') }}",
minLength: 3,
select: function (event, ui) {
$('#postalCode').val(ui.item.value);
}
});
</script>
@else
<!-- No se cargó select2-->
@endif
Route:
// Aquí se guarda la función que hace, vía ajax, búsqueda de código postal.
Route::get('/buscarCp', 'SearchController@buscarCp')->name('busqueda-cp');
javascript php jquery laravel autocomplete
I'm using jQuery's Autocomplete to get data while the user is typing. It works; I get some zipcode that matches the user's input.
The thing is that I want to pass more than the zipcode, like the neighborhood associated with that zipcode. Both are in the same table.
I want to pass the zipcode AND the neighborhood in the autocomplete.
Controller:
<?php
namespace Grupo_VillanuevaHttpControllers;
use IlluminateHttpRequest;
use IlluminateSupportFacadesDB;
use IlluminateSupportFacadesResponse;
class SearchController extends Controller
{
public function buscarCp(Request $request)
{
$term = $request->input('term');
$results = ;
$queries = DB::connection('db_postalcodes')
->table('postal_code')
->where('postal_code', 'LIKE', '%' . $term . '%') //search 'postal_code' column
->take(10)
->get(); // get 5 results
foreach ($queries as $query) {
$results = [
'postal_code' => $query->postal_code,
'value' => $query->postal_code,
'colonia' => $query->neighborhood];
}
return Response::json($results);
}
}
I thought about fetching it with the colonia
inside the associative array, though I'm not an expert.
JS:
@if (Request::is('admin/posts/create'))
{{-- <script src="https://cdnjs.cloudflare.com/ajax/libs/select2/4.0.6-rc.0/js/select2.min.js"></script> --}}
<script src="https://code.jquery.com/jquery-1.12.4.js"></script>
<script src="https://code.jquery.com/ui/1.12.1/jquery-ui.js"></script>
<script>
$('.postalCode').autocomplete({
source: "{{ route('busqueda-cp') }}",
minLength: 3,
select: function (event, ui) {
$('#postalCode').val(ui.item.value);
}
});
</script>
@else
<!-- No se cargó select2-->
@endif
Route:
// Aquí se guarda la función que hace, vía ajax, búsqueda de código postal.
Route::get('/buscarCp', 'SearchController@buscarCp')->name('busqueda-cp');
javascript php jquery laravel autocomplete
javascript php jquery laravel autocomplete
asked Nov 20 at 1:59


dawn
3191317
3191317
1
Do you mean you want to append the neighborhood to the zip code? In your$results
you can do this:'value' => $query->postal_code . ' ' . $query->neighborhood,
– newUserName02
Nov 20 at 2:09
@newUserName02 Works. Thanks. Questions: So could I append any number of data that way?. Also, answer the question so I can select you as the winner!
– dawn
Nov 20 at 2:12
add a comment |
1
Do you mean you want to append the neighborhood to the zip code? In your$results
you can do this:'value' => $query->postal_code . ' ' . $query->neighborhood,
– newUserName02
Nov 20 at 2:09
@newUserName02 Works. Thanks. Questions: So could I append any number of data that way?. Also, answer the question so I can select you as the winner!
– dawn
Nov 20 at 2:12
1
1
Do you mean you want to append the neighborhood to the zip code? In your
$results
you can do this: 'value' => $query->postal_code . ' ' . $query->neighborhood,
– newUserName02
Nov 20 at 2:09
Do you mean you want to append the neighborhood to the zip code? In your
$results
you can do this: 'value' => $query->postal_code . ' ' . $query->neighborhood,
– newUserName02
Nov 20 at 2:09
@newUserName02 Works. Thanks. Questions: So could I append any number of data that way?. Also, answer the question so I can select you as the winner!
– dawn
Nov 20 at 2:12
@newUserName02 Works. Thanks. Questions: So could I append any number of data that way?. Also, answer the question so I can select you as the winner!
– dawn
Nov 20 at 2:12
add a comment |
2 Answers
2
active
oldest
votes
up vote
1
down vote
accepted
You can concatenate strings together using .
between strings. In your $results
variable:
'value' => $query->postal_code . ' ' . $query->neighborhood,
You can concatenate any number of strings together this way:
$query->postal_code . ' ' . $query->neighborhood . ' (' . $query->foo . ') ' . $query->bar
add a comment |
up vote
1
down vote
If you wanted to keep the array value from php you could use autocomplete's _renderItem
method as seen here.
So your code would look like this:
$('.postalCode').autocomplete({
source: "{{ route('busqueda-cp') }}",
minLength: 3,
select: function (event, ui) {
$('#postalCode').val(ui.item.value);
}
}).autocomplete( "instance" )._renderItem = function( ul, item ) {
return $( "<li>" )
.append( item.postal_code + " " + item.colonia )
.appendTo( ul );
};
Then you could use the value and just change the way the select items look.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53385170%2fhow-to-pass-multiple-db-data-into-jquerys-autocomplete-ajax-in-laravel-5-7%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
1
down vote
accepted
You can concatenate strings together using .
between strings. In your $results
variable:
'value' => $query->postal_code . ' ' . $query->neighborhood,
You can concatenate any number of strings together this way:
$query->postal_code . ' ' . $query->neighborhood . ' (' . $query->foo . ') ' . $query->bar
add a comment |
up vote
1
down vote
accepted
You can concatenate strings together using .
between strings. In your $results
variable:
'value' => $query->postal_code . ' ' . $query->neighborhood,
You can concatenate any number of strings together this way:
$query->postal_code . ' ' . $query->neighborhood . ' (' . $query->foo . ') ' . $query->bar
add a comment |
up vote
1
down vote
accepted
up vote
1
down vote
accepted
You can concatenate strings together using .
between strings. In your $results
variable:
'value' => $query->postal_code . ' ' . $query->neighborhood,
You can concatenate any number of strings together this way:
$query->postal_code . ' ' . $query->neighborhood . ' (' . $query->foo . ') ' . $query->bar
You can concatenate strings together using .
between strings. In your $results
variable:
'value' => $query->postal_code . ' ' . $query->neighborhood,
You can concatenate any number of strings together this way:
$query->postal_code . ' ' . $query->neighborhood . ' (' . $query->foo . ') ' . $query->bar
answered Nov 20 at 2:18
newUserName02
54849
54849
add a comment |
add a comment |
up vote
1
down vote
If you wanted to keep the array value from php you could use autocomplete's _renderItem
method as seen here.
So your code would look like this:
$('.postalCode').autocomplete({
source: "{{ route('busqueda-cp') }}",
minLength: 3,
select: function (event, ui) {
$('#postalCode').val(ui.item.value);
}
}).autocomplete( "instance" )._renderItem = function( ul, item ) {
return $( "<li>" )
.append( item.postal_code + " " + item.colonia )
.appendTo( ul );
};
Then you could use the value and just change the way the select items look.
add a comment |
up vote
1
down vote
If you wanted to keep the array value from php you could use autocomplete's _renderItem
method as seen here.
So your code would look like this:
$('.postalCode').autocomplete({
source: "{{ route('busqueda-cp') }}",
minLength: 3,
select: function (event, ui) {
$('#postalCode').val(ui.item.value);
}
}).autocomplete( "instance" )._renderItem = function( ul, item ) {
return $( "<li>" )
.append( item.postal_code + " " + item.colonia )
.appendTo( ul );
};
Then you could use the value and just change the way the select items look.
add a comment |
up vote
1
down vote
up vote
1
down vote
If you wanted to keep the array value from php you could use autocomplete's _renderItem
method as seen here.
So your code would look like this:
$('.postalCode').autocomplete({
source: "{{ route('busqueda-cp') }}",
minLength: 3,
select: function (event, ui) {
$('#postalCode').val(ui.item.value);
}
}).autocomplete( "instance" )._renderItem = function( ul, item ) {
return $( "<li>" )
.append( item.postal_code + " " + item.colonia )
.appendTo( ul );
};
Then you could use the value and just change the way the select items look.
If you wanted to keep the array value from php you could use autocomplete's _renderItem
method as seen here.
So your code would look like this:
$('.postalCode').autocomplete({
source: "{{ route('busqueda-cp') }}",
minLength: 3,
select: function (event, ui) {
$('#postalCode').val(ui.item.value);
}
}).autocomplete( "instance" )._renderItem = function( ul, item ) {
return $( "<li>" )
.append( item.postal_code + " " + item.colonia )
.appendTo( ul );
};
Then you could use the value and just change the way the select items look.
answered Nov 20 at 2:29
CUGreen
1,8701511
1,8701511
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53385170%2fhow-to-pass-multiple-db-data-into-jquerys-autocomplete-ajax-in-laravel-5-7%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
8yUOJSMi1 UVP44H4OwE9p,B6ckkscPJR,MVddxO,doR
1
Do you mean you want to append the neighborhood to the zip code? In your
$results
you can do this:'value' => $query->postal_code . ' ' . $query->neighborhood,
– newUserName02
Nov 20 at 2:09
@newUserName02 Works. Thanks. Questions: So could I append any number of data that way?. Also, answer the question so I can select you as the winner!
– dawn
Nov 20 at 2:12