javascript/react - async/await with for loop POST requests
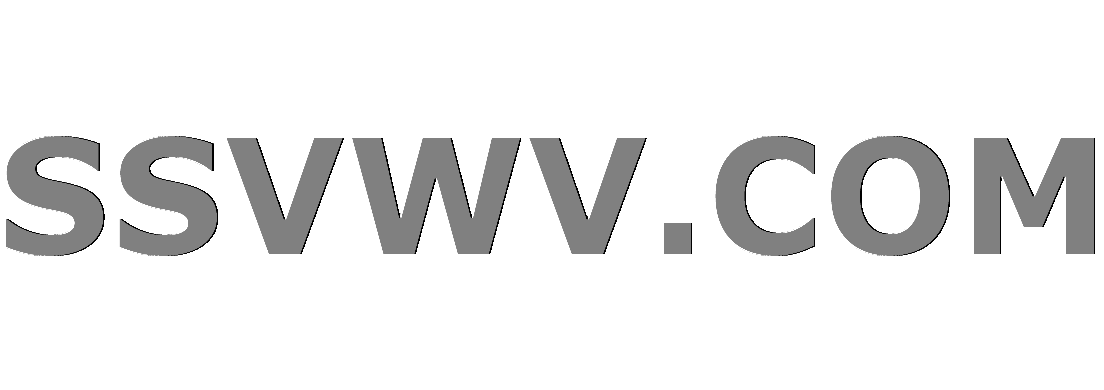
Multi tool use
up vote
2
down vote
favorite
I'm creating a picture converter where my JS code gets the file inputs from the user, sends it to my python back-end where they are converted and saved to a folder. Python then sends a response back to JS (react), which updates the state for each file individually as "converted" and re-renders the necessary components.
I have a for loop that sends individual POST requests for each file. This is fine until I want to create a .zip for the entire directory after all files have been converted. My problem lies there. My zip is always returned empty or with incomplete files.
// function which takes the file inputs from user
uploadBatch = async () => {
const files = this.getFilesFromInput();
const batch = Math.floor(Math.random() * 999999 + 100000);
for (let i = 0; i < files.length; i++) {
// sets the state which will then be updated
await this.setState(
{
files: [
...this.state.files,
{
// file state values
}
]
},
() => {
const formData = new FormData();
// appends stuff to form data to send to python
axios
.post('/api/upload', formData, {
headers: {
'Content-Type': 'multipart/form-data'
},
responsetype: 'json'
})
.then(response => {
// update the state for this particular file
});
}
);
}
return batch;
};
// function which zips the folder after files are converted
handleUpload = async e => {
e.preventDefault();
// shouldn't this next line wait for uploadBatch() to finish before
// proceeding?
const batch = await this.uploadBatch();
// this successfully zips my files, but it seems to run too soon
axios.post('/api/zip', { batch: batch }).then(response => {
console.log(response.data);
});
};
I have used async/await but I don't think I've used them well. I don't quite fundamentally understand this concept so an explanation would be greatly appreciated.
javascript reactjs asynchronous
add a comment |
up vote
2
down vote
favorite
I'm creating a picture converter where my JS code gets the file inputs from the user, sends it to my python back-end where they are converted and saved to a folder. Python then sends a response back to JS (react), which updates the state for each file individually as "converted" and re-renders the necessary components.
I have a for loop that sends individual POST requests for each file. This is fine until I want to create a .zip for the entire directory after all files have been converted. My problem lies there. My zip is always returned empty or with incomplete files.
// function which takes the file inputs from user
uploadBatch = async () => {
const files = this.getFilesFromInput();
const batch = Math.floor(Math.random() * 999999 + 100000);
for (let i = 0; i < files.length; i++) {
// sets the state which will then be updated
await this.setState(
{
files: [
...this.state.files,
{
// file state values
}
]
},
() => {
const formData = new FormData();
// appends stuff to form data to send to python
axios
.post('/api/upload', formData, {
headers: {
'Content-Type': 'multipart/form-data'
},
responsetype: 'json'
})
.then(response => {
// update the state for this particular file
});
}
);
}
return batch;
};
// function which zips the folder after files are converted
handleUpload = async e => {
e.preventDefault();
// shouldn't this next line wait for uploadBatch() to finish before
// proceeding?
const batch = await this.uploadBatch();
// this successfully zips my files, but it seems to run too soon
axios.post('/api/zip', { batch: batch }).then(response => {
console.log(response.data);
});
};
I have used async/await but I don't think I've used them well. I don't quite fundamentally understand this concept so an explanation would be greatly appreciated.
javascript reactjs asynchronous
async await documentation - note, I doubtthis.setState
returns a Promise - which is whatawait
awaits
– Bravo
Nov 20 at 2:15
@Bravo no, it just sets the state. I have read multiple documentations but am still not quite how or where exactly I'd include a promise
– dariuscosden
Nov 20 at 2:28
Possible Duplicate of What if you can use async/await to make React's setState synchronous
– Shubham Khatri
Nov 20 at 5:14
add a comment |
up vote
2
down vote
favorite
up vote
2
down vote
favorite
I'm creating a picture converter where my JS code gets the file inputs from the user, sends it to my python back-end where they are converted and saved to a folder. Python then sends a response back to JS (react), which updates the state for each file individually as "converted" and re-renders the necessary components.
I have a for loop that sends individual POST requests for each file. This is fine until I want to create a .zip for the entire directory after all files have been converted. My problem lies there. My zip is always returned empty or with incomplete files.
// function which takes the file inputs from user
uploadBatch = async () => {
const files = this.getFilesFromInput();
const batch = Math.floor(Math.random() * 999999 + 100000);
for (let i = 0; i < files.length; i++) {
// sets the state which will then be updated
await this.setState(
{
files: [
...this.state.files,
{
// file state values
}
]
},
() => {
const formData = new FormData();
// appends stuff to form data to send to python
axios
.post('/api/upload', formData, {
headers: {
'Content-Type': 'multipart/form-data'
},
responsetype: 'json'
})
.then(response => {
// update the state for this particular file
});
}
);
}
return batch;
};
// function which zips the folder after files are converted
handleUpload = async e => {
e.preventDefault();
// shouldn't this next line wait for uploadBatch() to finish before
// proceeding?
const batch = await this.uploadBatch();
// this successfully zips my files, but it seems to run too soon
axios.post('/api/zip', { batch: batch }).then(response => {
console.log(response.data);
});
};
I have used async/await but I don't think I've used them well. I don't quite fundamentally understand this concept so an explanation would be greatly appreciated.
javascript reactjs asynchronous
I'm creating a picture converter where my JS code gets the file inputs from the user, sends it to my python back-end where they are converted and saved to a folder. Python then sends a response back to JS (react), which updates the state for each file individually as "converted" and re-renders the necessary components.
I have a for loop that sends individual POST requests for each file. This is fine until I want to create a .zip for the entire directory after all files have been converted. My problem lies there. My zip is always returned empty or with incomplete files.
// function which takes the file inputs from user
uploadBatch = async () => {
const files = this.getFilesFromInput();
const batch = Math.floor(Math.random() * 999999 + 100000);
for (let i = 0; i < files.length; i++) {
// sets the state which will then be updated
await this.setState(
{
files: [
...this.state.files,
{
// file state values
}
]
},
() => {
const formData = new FormData();
// appends stuff to form data to send to python
axios
.post('/api/upload', formData, {
headers: {
'Content-Type': 'multipart/form-data'
},
responsetype: 'json'
})
.then(response => {
// update the state for this particular file
});
}
);
}
return batch;
};
// function which zips the folder after files are converted
handleUpload = async e => {
e.preventDefault();
// shouldn't this next line wait for uploadBatch() to finish before
// proceeding?
const batch = await this.uploadBatch();
// this successfully zips my files, but it seems to run too soon
axios.post('/api/zip', { batch: batch }).then(response => {
console.log(response.data);
});
};
I have used async/await but I don't think I've used them well. I don't quite fundamentally understand this concept so an explanation would be greatly appreciated.
javascript reactjs asynchronous
javascript reactjs asynchronous
asked Nov 20 at 2:04
dariuscosden
6618
6618
async await documentation - note, I doubtthis.setState
returns a Promise - which is whatawait
awaits
– Bravo
Nov 20 at 2:15
@Bravo no, it just sets the state. I have read multiple documentations but am still not quite how or where exactly I'd include a promise
– dariuscosden
Nov 20 at 2:28
Possible Duplicate of What if you can use async/await to make React's setState synchronous
– Shubham Khatri
Nov 20 at 5:14
add a comment |
async await documentation - note, I doubtthis.setState
returns a Promise - which is whatawait
awaits
– Bravo
Nov 20 at 2:15
@Bravo no, it just sets the state. I have read multiple documentations but am still not quite how or where exactly I'd include a promise
– dariuscosden
Nov 20 at 2:28
Possible Duplicate of What if you can use async/await to make React's setState synchronous
– Shubham Khatri
Nov 20 at 5:14
async await documentation - note, I doubt
this.setState
returns a Promise - which is what await
awaits– Bravo
Nov 20 at 2:15
async await documentation - note, I doubt
this.setState
returns a Promise - which is what await
awaits– Bravo
Nov 20 at 2:15
@Bravo no, it just sets the state. I have read multiple documentations but am still not quite how or where exactly I'd include a promise
– dariuscosden
Nov 20 at 2:28
@Bravo no, it just sets the state. I have read multiple documentations but am still not quite how or where exactly I'd include a promise
– dariuscosden
Nov 20 at 2:28
Possible Duplicate of What if you can use async/await to make React's setState synchronous
– Shubham Khatri
Nov 20 at 5:14
Possible Duplicate of What if you can use async/await to make React's setState synchronous
– Shubham Khatri
Nov 20 at 5:14
add a comment |
1 Answer
1
active
oldest
votes
up vote
1
down vote
Whenever you call setState()
, the component will re-render. You should ideally complete all your actions and call setState()
at the end.
Something like this should get things working for you
// function which takes the file inputs from user
uploadBatch = async () => {
const files = this.getFilesFromInput();
const batch = Math.floor(Math.random() * 999999 + 100000);
const files = ;
for (let i = 0; i < files.length; i++) {
const formData = new FormData();
// appends stuff to form data to send to python
const res =
await axios
.post('/api/upload', formData, {
headers: {
'Content-Type': 'multipart/form-data'
},
responsetype: 'json'
});
files.push('push data into files arr');
}
return { files, batch };
};
// function which zips the folder after files are converted
handleUpload = async e => {
e.preventDefault();
// get batch and files to be uploaded and updated
const { files, batch } = await this.uploadBatch();
// this successfully zips my files, but it seems to run too soon
await axios.post('/api/zip', { batch: batch }).then(response => {
console.log(response.data);
});
// set the state after all actions are done
this.setState( { files: files });
};
I actually want to re-render each time since I want to display the file status for each file being uploaded (converting... -> converted). As soon as each file is converted, Python sends a response back to which then react updates the state. The problem is that I needhandleUpload
to wait for all the states to be converted before sending the zip request.
– dariuscosden
Nov 20 at 2:26
Then you need to store everything in state, saybatch
,allFilesUploaded
and update the status of each file after the upload. You will need to useallFilesUploaded
state value to trigger the/api/zip
POST call.
– Dinesh Pandiyan
Nov 20 at 2:29
I thought about that, and that would work for sure. But wouldn't using async/await be a better approach to this?
– dariuscosden
Nov 20 at 2:32
setState()
will trigger a re-render every time it's executed and you'll never get to the end of the method even if you useasync-await
. You cannot continue a logic after updating the state by holding off render.
– Dinesh Pandiyan
Nov 20 at 2:44
1
I wasn't holding off any render! I just wanted to wait for the for loop to finish before running another post request. Regardless, I fixed it withasync-await
as I suspected would work. I just had to includepromises
and tell my code to wait for those. I'll answer my own question tomorrow for others who might have this issue! Thanks for the help!
– dariuscosden
Nov 20 at 3:27
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53385197%2fjavascript-react-async-await-with-for-loop-post-requests%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
1
down vote
Whenever you call setState()
, the component will re-render. You should ideally complete all your actions and call setState()
at the end.
Something like this should get things working for you
// function which takes the file inputs from user
uploadBatch = async () => {
const files = this.getFilesFromInput();
const batch = Math.floor(Math.random() * 999999 + 100000);
const files = ;
for (let i = 0; i < files.length; i++) {
const formData = new FormData();
// appends stuff to form data to send to python
const res =
await axios
.post('/api/upload', formData, {
headers: {
'Content-Type': 'multipart/form-data'
},
responsetype: 'json'
});
files.push('push data into files arr');
}
return { files, batch };
};
// function which zips the folder after files are converted
handleUpload = async e => {
e.preventDefault();
// get batch and files to be uploaded and updated
const { files, batch } = await this.uploadBatch();
// this successfully zips my files, but it seems to run too soon
await axios.post('/api/zip', { batch: batch }).then(response => {
console.log(response.data);
});
// set the state after all actions are done
this.setState( { files: files });
};
I actually want to re-render each time since I want to display the file status for each file being uploaded (converting... -> converted). As soon as each file is converted, Python sends a response back to which then react updates the state. The problem is that I needhandleUpload
to wait for all the states to be converted before sending the zip request.
– dariuscosden
Nov 20 at 2:26
Then you need to store everything in state, saybatch
,allFilesUploaded
and update the status of each file after the upload. You will need to useallFilesUploaded
state value to trigger the/api/zip
POST call.
– Dinesh Pandiyan
Nov 20 at 2:29
I thought about that, and that would work for sure. But wouldn't using async/await be a better approach to this?
– dariuscosden
Nov 20 at 2:32
setState()
will trigger a re-render every time it's executed and you'll never get to the end of the method even if you useasync-await
. You cannot continue a logic after updating the state by holding off render.
– Dinesh Pandiyan
Nov 20 at 2:44
1
I wasn't holding off any render! I just wanted to wait for the for loop to finish before running another post request. Regardless, I fixed it withasync-await
as I suspected would work. I just had to includepromises
and tell my code to wait for those. I'll answer my own question tomorrow for others who might have this issue! Thanks for the help!
– dariuscosden
Nov 20 at 3:27
add a comment |
up vote
1
down vote
Whenever you call setState()
, the component will re-render. You should ideally complete all your actions and call setState()
at the end.
Something like this should get things working for you
// function which takes the file inputs from user
uploadBatch = async () => {
const files = this.getFilesFromInput();
const batch = Math.floor(Math.random() * 999999 + 100000);
const files = ;
for (let i = 0; i < files.length; i++) {
const formData = new FormData();
// appends stuff to form data to send to python
const res =
await axios
.post('/api/upload', formData, {
headers: {
'Content-Type': 'multipart/form-data'
},
responsetype: 'json'
});
files.push('push data into files arr');
}
return { files, batch };
};
// function which zips the folder after files are converted
handleUpload = async e => {
e.preventDefault();
// get batch and files to be uploaded and updated
const { files, batch } = await this.uploadBatch();
// this successfully zips my files, but it seems to run too soon
await axios.post('/api/zip', { batch: batch }).then(response => {
console.log(response.data);
});
// set the state after all actions are done
this.setState( { files: files });
};
I actually want to re-render each time since I want to display the file status for each file being uploaded (converting... -> converted). As soon as each file is converted, Python sends a response back to which then react updates the state. The problem is that I needhandleUpload
to wait for all the states to be converted before sending the zip request.
– dariuscosden
Nov 20 at 2:26
Then you need to store everything in state, saybatch
,allFilesUploaded
and update the status of each file after the upload. You will need to useallFilesUploaded
state value to trigger the/api/zip
POST call.
– Dinesh Pandiyan
Nov 20 at 2:29
I thought about that, and that would work for sure. But wouldn't using async/await be a better approach to this?
– dariuscosden
Nov 20 at 2:32
setState()
will trigger a re-render every time it's executed and you'll never get to the end of the method even if you useasync-await
. You cannot continue a logic after updating the state by holding off render.
– Dinesh Pandiyan
Nov 20 at 2:44
1
I wasn't holding off any render! I just wanted to wait for the for loop to finish before running another post request. Regardless, I fixed it withasync-await
as I suspected would work. I just had to includepromises
and tell my code to wait for those. I'll answer my own question tomorrow for others who might have this issue! Thanks for the help!
– dariuscosden
Nov 20 at 3:27
add a comment |
up vote
1
down vote
up vote
1
down vote
Whenever you call setState()
, the component will re-render. You should ideally complete all your actions and call setState()
at the end.
Something like this should get things working for you
// function which takes the file inputs from user
uploadBatch = async () => {
const files = this.getFilesFromInput();
const batch = Math.floor(Math.random() * 999999 + 100000);
const files = ;
for (let i = 0; i < files.length; i++) {
const formData = new FormData();
// appends stuff to form data to send to python
const res =
await axios
.post('/api/upload', formData, {
headers: {
'Content-Type': 'multipart/form-data'
},
responsetype: 'json'
});
files.push('push data into files arr');
}
return { files, batch };
};
// function which zips the folder after files are converted
handleUpload = async e => {
e.preventDefault();
// get batch and files to be uploaded and updated
const { files, batch } = await this.uploadBatch();
// this successfully zips my files, but it seems to run too soon
await axios.post('/api/zip', { batch: batch }).then(response => {
console.log(response.data);
});
// set the state after all actions are done
this.setState( { files: files });
};
Whenever you call setState()
, the component will re-render. You should ideally complete all your actions and call setState()
at the end.
Something like this should get things working for you
// function which takes the file inputs from user
uploadBatch = async () => {
const files = this.getFilesFromInput();
const batch = Math.floor(Math.random() * 999999 + 100000);
const files = ;
for (let i = 0; i < files.length; i++) {
const formData = new FormData();
// appends stuff to form data to send to python
const res =
await axios
.post('/api/upload', formData, {
headers: {
'Content-Type': 'multipart/form-data'
},
responsetype: 'json'
});
files.push('push data into files arr');
}
return { files, batch };
};
// function which zips the folder after files are converted
handleUpload = async e => {
e.preventDefault();
// get batch and files to be uploaded and updated
const { files, batch } = await this.uploadBatch();
// this successfully zips my files, but it seems to run too soon
await axios.post('/api/zip', { batch: batch }).then(response => {
console.log(response.data);
});
// set the state after all actions are done
this.setState( { files: files });
};
answered Nov 20 at 2:19


Dinesh Pandiyan
2,118824
2,118824
I actually want to re-render each time since I want to display the file status for each file being uploaded (converting... -> converted). As soon as each file is converted, Python sends a response back to which then react updates the state. The problem is that I needhandleUpload
to wait for all the states to be converted before sending the zip request.
– dariuscosden
Nov 20 at 2:26
Then you need to store everything in state, saybatch
,allFilesUploaded
and update the status of each file after the upload. You will need to useallFilesUploaded
state value to trigger the/api/zip
POST call.
– Dinesh Pandiyan
Nov 20 at 2:29
I thought about that, and that would work for sure. But wouldn't using async/await be a better approach to this?
– dariuscosden
Nov 20 at 2:32
setState()
will trigger a re-render every time it's executed and you'll never get to the end of the method even if you useasync-await
. You cannot continue a logic after updating the state by holding off render.
– Dinesh Pandiyan
Nov 20 at 2:44
1
I wasn't holding off any render! I just wanted to wait for the for loop to finish before running another post request. Regardless, I fixed it withasync-await
as I suspected would work. I just had to includepromises
and tell my code to wait for those. I'll answer my own question tomorrow for others who might have this issue! Thanks for the help!
– dariuscosden
Nov 20 at 3:27
add a comment |
I actually want to re-render each time since I want to display the file status for each file being uploaded (converting... -> converted). As soon as each file is converted, Python sends a response back to which then react updates the state. The problem is that I needhandleUpload
to wait for all the states to be converted before sending the zip request.
– dariuscosden
Nov 20 at 2:26
Then you need to store everything in state, saybatch
,allFilesUploaded
and update the status of each file after the upload. You will need to useallFilesUploaded
state value to trigger the/api/zip
POST call.
– Dinesh Pandiyan
Nov 20 at 2:29
I thought about that, and that would work for sure. But wouldn't using async/await be a better approach to this?
– dariuscosden
Nov 20 at 2:32
setState()
will trigger a re-render every time it's executed and you'll never get to the end of the method even if you useasync-await
. You cannot continue a logic after updating the state by holding off render.
– Dinesh Pandiyan
Nov 20 at 2:44
1
I wasn't holding off any render! I just wanted to wait for the for loop to finish before running another post request. Regardless, I fixed it withasync-await
as I suspected would work. I just had to includepromises
and tell my code to wait for those. I'll answer my own question tomorrow for others who might have this issue! Thanks for the help!
– dariuscosden
Nov 20 at 3:27
I actually want to re-render each time since I want to display the file status for each file being uploaded (converting... -> converted). As soon as each file is converted, Python sends a response back to which then react updates the state. The problem is that I need
handleUpload
to wait for all the states to be converted before sending the zip request.– dariuscosden
Nov 20 at 2:26
I actually want to re-render each time since I want to display the file status for each file being uploaded (converting... -> converted). As soon as each file is converted, Python sends a response back to which then react updates the state. The problem is that I need
handleUpload
to wait for all the states to be converted before sending the zip request.– dariuscosden
Nov 20 at 2:26
Then you need to store everything in state, say
batch
, allFilesUploaded
and update the status of each file after the upload. You will need to use allFilesUploaded
state value to trigger the /api/zip
POST call.– Dinesh Pandiyan
Nov 20 at 2:29
Then you need to store everything in state, say
batch
, allFilesUploaded
and update the status of each file after the upload. You will need to use allFilesUploaded
state value to trigger the /api/zip
POST call.– Dinesh Pandiyan
Nov 20 at 2:29
I thought about that, and that would work for sure. But wouldn't using async/await be a better approach to this?
– dariuscosden
Nov 20 at 2:32
I thought about that, and that would work for sure. But wouldn't using async/await be a better approach to this?
– dariuscosden
Nov 20 at 2:32
setState()
will trigger a re-render every time it's executed and you'll never get to the end of the method even if you use async-await
. You cannot continue a logic after updating the state by holding off render.– Dinesh Pandiyan
Nov 20 at 2:44
setState()
will trigger a re-render every time it's executed and you'll never get to the end of the method even if you use async-await
. You cannot continue a logic after updating the state by holding off render.– Dinesh Pandiyan
Nov 20 at 2:44
1
1
I wasn't holding off any render! I just wanted to wait for the for loop to finish before running another post request. Regardless, I fixed it with
async-await
as I suspected would work. I just had to include promises
and tell my code to wait for those. I'll answer my own question tomorrow for others who might have this issue! Thanks for the help!– dariuscosden
Nov 20 at 3:27
I wasn't holding off any render! I just wanted to wait for the for loop to finish before running another post request. Regardless, I fixed it with
async-await
as I suspected would work. I just had to include promises
and tell my code to wait for those. I'll answer my own question tomorrow for others who might have this issue! Thanks for the help!– dariuscosden
Nov 20 at 3:27
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53385197%2fjavascript-react-async-await-with-for-loop-post-requests%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
77j3M3AXbFhZ nVLyS 23rzlMNgNdEUPNYVRPLaxmHk1 F,o78,y Vn3bXvoFNjnOzlVDE41 w0YGILJcDIMhDIi
async await documentation - note, I doubt
this.setState
returns a Promise - which is whatawait
awaits– Bravo
Nov 20 at 2:15
@Bravo no, it just sets the state. I have read multiple documentations but am still not quite how or where exactly I'd include a promise
– dariuscosden
Nov 20 at 2:28
Possible Duplicate of What if you can use async/await to make React's setState synchronous
– Shubham Khatri
Nov 20 at 5:14