Java 8 - Optimize multiple nested for loop [on hold]
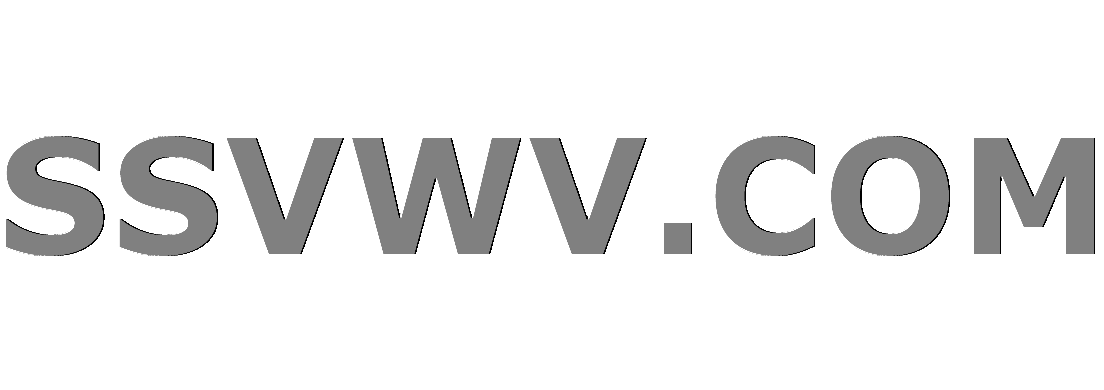
Multi tool use
up vote
0
down vote
favorite
I have a question regarding validate data in nested loop.
public class Object1{
private String obj1Name;
private String obj1Desc;
private List<Object2> object2List;
//Setters and getters
}
public class Object2{
private String obj2Name;
private String obj2Desc;
private List<Object3> object3List;
//Setters and getters
}
public class Object3{
private String obj3Name;
private String obj3Desc;
//Setters and getters
}
I wish to validate both name
and desc
in all object, instead of using nested loop like following:
List<Object1> object1List = getObject1List();
for(Object1 object1 : object1List ){
if(object1.getObj1Name() == null){
//throw error
}
if(object1.getObj1Desc() == null){
//throw error
}
for(Object2 object2 : object1.getObject2List()){
if(object2.getObj2Name() == null){
//throw error
}
if(object2.getObj2Desc() == null){
//throw error
}
//loop Object 3 ...
}
}
How can i improve it in java 8, is it possible to use flatmap in this case?
java
put on hold as off-topic by Jamal♦ 13 mins ago
This question appears to be off-topic. The users who voted to close gave this specific reason:
- "Lacks concrete context: Code Review requires concrete code from a project, with sufficient context for reviewers to understand how that code is used. Pseudocode, stub code, hypothetical code, obfuscated code, and generic best practices are outside the scope of this site." – Jamal
If this question can be reworded to fit the rules in the help center, please edit the question.
add a comment |
up vote
0
down vote
favorite
I have a question regarding validate data in nested loop.
public class Object1{
private String obj1Name;
private String obj1Desc;
private List<Object2> object2List;
//Setters and getters
}
public class Object2{
private String obj2Name;
private String obj2Desc;
private List<Object3> object3List;
//Setters and getters
}
public class Object3{
private String obj3Name;
private String obj3Desc;
//Setters and getters
}
I wish to validate both name
and desc
in all object, instead of using nested loop like following:
List<Object1> object1List = getObject1List();
for(Object1 object1 : object1List ){
if(object1.getObj1Name() == null){
//throw error
}
if(object1.getObj1Desc() == null){
//throw error
}
for(Object2 object2 : object1.getObject2List()){
if(object2.getObj2Name() == null){
//throw error
}
if(object2.getObj2Desc() == null){
//throw error
}
//loop Object 3 ...
}
}
How can i improve it in java 8, is it possible to use flatmap in this case?
java
put on hold as off-topic by Jamal♦ 13 mins ago
This question appears to be off-topic. The users who voted to close gave this specific reason:
- "Lacks concrete context: Code Review requires concrete code from a project, with sufficient context for reviewers to understand how that code is used. Pseudocode, stub code, hypothetical code, obfuscated code, and generic best practices are outside the scope of this site." – Jamal
If this question can be reworded to fit the rules in the help center, please edit the question.
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I have a question regarding validate data in nested loop.
public class Object1{
private String obj1Name;
private String obj1Desc;
private List<Object2> object2List;
//Setters and getters
}
public class Object2{
private String obj2Name;
private String obj2Desc;
private List<Object3> object3List;
//Setters and getters
}
public class Object3{
private String obj3Name;
private String obj3Desc;
//Setters and getters
}
I wish to validate both name
and desc
in all object, instead of using nested loop like following:
List<Object1> object1List = getObject1List();
for(Object1 object1 : object1List ){
if(object1.getObj1Name() == null){
//throw error
}
if(object1.getObj1Desc() == null){
//throw error
}
for(Object2 object2 : object1.getObject2List()){
if(object2.getObj2Name() == null){
//throw error
}
if(object2.getObj2Desc() == null){
//throw error
}
//loop Object 3 ...
}
}
How can i improve it in java 8, is it possible to use flatmap in this case?
java
I have a question regarding validate data in nested loop.
public class Object1{
private String obj1Name;
private String obj1Desc;
private List<Object2> object2List;
//Setters and getters
}
public class Object2{
private String obj2Name;
private String obj2Desc;
private List<Object3> object3List;
//Setters and getters
}
public class Object3{
private String obj3Name;
private String obj3Desc;
//Setters and getters
}
I wish to validate both name
and desc
in all object, instead of using nested loop like following:
List<Object1> object1List = getObject1List();
for(Object1 object1 : object1List ){
if(object1.getObj1Name() == null){
//throw error
}
if(object1.getObj1Desc() == null){
//throw error
}
for(Object2 object2 : object1.getObject2List()){
if(object2.getObj2Name() == null){
//throw error
}
if(object2.getObj2Desc() == null){
//throw error
}
//loop Object 3 ...
}
}
How can i improve it in java 8, is it possible to use flatmap in this case?
java
java
edited 8 mins ago
asked 14 mins ago
hades
18418
18418
put on hold as off-topic by Jamal♦ 13 mins ago
This question appears to be off-topic. The users who voted to close gave this specific reason:
- "Lacks concrete context: Code Review requires concrete code from a project, with sufficient context for reviewers to understand how that code is used. Pseudocode, stub code, hypothetical code, obfuscated code, and generic best practices are outside the scope of this site." – Jamal
If this question can be reworded to fit the rules in the help center, please edit the question.
put on hold as off-topic by Jamal♦ 13 mins ago
This question appears to be off-topic. The users who voted to close gave this specific reason:
- "Lacks concrete context: Code Review requires concrete code from a project, with sufficient context for reviewers to understand how that code is used. Pseudocode, stub code, hypothetical code, obfuscated code, and generic best practices are outside the scope of this site." – Jamal
If this question can be reworded to fit the rules in the help center, please edit the question.
add a comment |
add a comment |
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
94pkmAMia2E,jXDiV,lC45aEG NHlYy95,wGdaSPC viU3KjuWqtkIwV bI1BDSk1UROuREmTZ78mSTRsIZDz3VMYCpl4 y,kaOTp