Order of the elements in the Anchor Pane
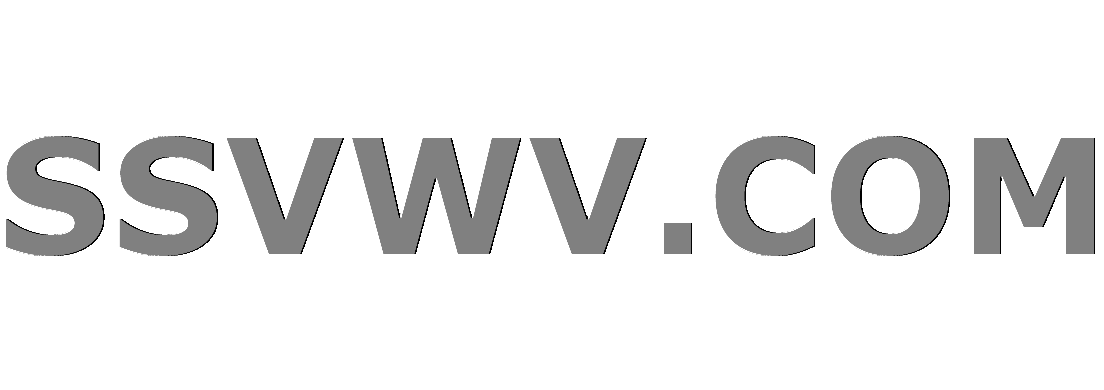
Multi tool use
up vote
0
down vote
favorite
when I try to initialize the Anchor Pane a strange things happens: if I put the elements in this order I cannot click with the mouse, for example, on the elements of the list.
AnchorPane root = new AnchorPane(listLoader.load(), fieldLoader.load(), textareaLoader.load(), buttonLoader.load(), menuLoader.load());
But if I write it on this way I'm able to select the items on the list but not the items in the menubar:
AnchorPane root = new AnchorPane(fieldLoader.load(), textareaLoader.load(), buttonLoader.load(), menuLoader.load(), listLoader.load());
Do you know How should I write the elements to make at least the button, the menu and the list clickable?
This is the full code:
@Override
public void start(Stage stage) throws Exception {
FXMLLoader listLoader = new FXMLLoader(getClass().getResource("lista.fxml"));
FXMLLoader textareaLoader = new FXMLLoader(getClass().getResource("textarea.fxml"));
FXMLLoader fieldLoader = new FXMLLoader(getClass().getResource("textfield.fxml"));
FXMLLoader menuLoader = new FXMLLoader(getClass().getResource("menubar.fxml"));
FXMLLoader buttonLoader = new FXMLLoader(getClass().getResource("button.fxml"));
AnchorPane root = new AnchorPane(listLoader.load(), fieldLoader.load(), textareaLoader.load(), buttonLoader.load(), menuLoader.load());
ListController listController = listLoader.getController();
TextAreaController textareaController = textareaLoader.getController();
TextFieldController fieldController = fieldLoader.getController();
MenuBarController menuController = menuLoader.getController();
ButtonController buttonController = buttonLoader.getController();
DataModel model = new DataModel();
listController.initModel(model);
textareaController.initModel(model);
fieldController.initModel(model);
menuController.initModel(model);
buttonController.initModel(model);
Scene scene = new Scene(root, 603, 403);
stage.setScene(scene);
stage.show();
}
Lista.fxml:
<AnchorPane maxHeight="-Infinity" maxWidth="-Infinity" minHeight="-Infinity" minWidth="-Infinity" prefHeight="400.0" prefWidth="600.0" xmlns="http://javafx.com/javafx/8" xmlns:fx="http://javafx.com/fxml/1" fx:controller="mailbox.ListController">
Button.fxml:
<AnchorPane maxHeight="-Infinity" maxWidth="-Infinity" minHeight="-Infinity" minWidth="-Infinity" prefHeight="400.0" prefWidth="600.0" xmlns="http://javafx.com/javafx/8" xmlns:fx="http://javafx.com/fxml/1" fx:controller="mailbox.ButtonController">
MenuBar.fxml:
<AnchorPane maxHeight="-Infinity" maxWidth="-Infinity" minHeight="-Infinity" minWidth="-Infinity" prefHeight="400.0" prefWidth="600.0" xmlns:fx="http://javafx.com/fxml/1" xmlns="http://javafx.com/javafx/8" fx:controller="mailbox.MenuBarController">
<children>
<MenuBar fx:id="menuBar" layoutX="0.0" layoutY="0.0">
<menus>
<Menu text="File">
<items>
<MenuItem onAction="#elimina" text="Elimina" />
</items>
</Menu>
<Menu text="Cambia Account">
<items>
<MenuItem fx:id="email1" text="filippo@hotmail.it" />
<MenuItem fx:id="email2" text="giancarlo@yahoo.it" />
<MenuItem fx:id="email3" text="alessandro@gmail.it" />
</items>
</Menu>
</menus>
</MenuBar>
</children>
Textarea.fxml:
<AnchorPane maxHeight="-Infinity" maxWidth="-Infinity" minHeight="-Infinity" minWidth="-Infinity" prefHeight="400.0" prefWidth="600.0" xmlns:fx="http://javafx.com/fxml/1" xmlns="http://javafx.com/javafx/8" fx:controller="mailbox.TextAreaController">
Textfield.fxml:
<AnchorPane maxHeight="-Infinity" maxWidth="-Infinity" minHeight="-Infinity" minWidth="-Infinity" mouseTransparent="false" prefHeight="400.0" prefWidth="600.0" xmlns="http://javafx.com/javafx/8" xmlns:fx="http://javafx.com/fxml/1" fx:controller="mailbox.TextFieldController">
EDIT:
So should I declare more than one Anchor Pane and attach them to a main Anchor Pane?
AnchorPane root = new AnchorPane();
AnchorPane lista = new AnchorPane(listLoader.load());
AnchorPane textarea = new AnchorPane(textareaLoader.load());
AnchorPane field = new AnchorPane(fieldLoader.load());
AnchorPane menu = new AnchorPane(menuLoader.load());
AnchorPane button = new AnchorPane(buttonLoader.load());
root.getChildren().addAll(lista, textarea, field, menu, button);
EDIT2: This is the output of my program, can I create it with a BorderPane? Because It automatically anchor the elements on the right, left ecc... and for example I cannot put the textfield as you can see in the image
java listview javafx observable menuitem
add a comment |
up vote
0
down vote
favorite
when I try to initialize the Anchor Pane a strange things happens: if I put the elements in this order I cannot click with the mouse, for example, on the elements of the list.
AnchorPane root = new AnchorPane(listLoader.load(), fieldLoader.load(), textareaLoader.load(), buttonLoader.load(), menuLoader.load());
But if I write it on this way I'm able to select the items on the list but not the items in the menubar:
AnchorPane root = new AnchorPane(fieldLoader.load(), textareaLoader.load(), buttonLoader.load(), menuLoader.load(), listLoader.load());
Do you know How should I write the elements to make at least the button, the menu and the list clickable?
This is the full code:
@Override
public void start(Stage stage) throws Exception {
FXMLLoader listLoader = new FXMLLoader(getClass().getResource("lista.fxml"));
FXMLLoader textareaLoader = new FXMLLoader(getClass().getResource("textarea.fxml"));
FXMLLoader fieldLoader = new FXMLLoader(getClass().getResource("textfield.fxml"));
FXMLLoader menuLoader = new FXMLLoader(getClass().getResource("menubar.fxml"));
FXMLLoader buttonLoader = new FXMLLoader(getClass().getResource("button.fxml"));
AnchorPane root = new AnchorPane(listLoader.load(), fieldLoader.load(), textareaLoader.load(), buttonLoader.load(), menuLoader.load());
ListController listController = listLoader.getController();
TextAreaController textareaController = textareaLoader.getController();
TextFieldController fieldController = fieldLoader.getController();
MenuBarController menuController = menuLoader.getController();
ButtonController buttonController = buttonLoader.getController();
DataModel model = new DataModel();
listController.initModel(model);
textareaController.initModel(model);
fieldController.initModel(model);
menuController.initModel(model);
buttonController.initModel(model);
Scene scene = new Scene(root, 603, 403);
stage.setScene(scene);
stage.show();
}
Lista.fxml:
<AnchorPane maxHeight="-Infinity" maxWidth="-Infinity" minHeight="-Infinity" minWidth="-Infinity" prefHeight="400.0" prefWidth="600.0" xmlns="http://javafx.com/javafx/8" xmlns:fx="http://javafx.com/fxml/1" fx:controller="mailbox.ListController">
Button.fxml:
<AnchorPane maxHeight="-Infinity" maxWidth="-Infinity" minHeight="-Infinity" minWidth="-Infinity" prefHeight="400.0" prefWidth="600.0" xmlns="http://javafx.com/javafx/8" xmlns:fx="http://javafx.com/fxml/1" fx:controller="mailbox.ButtonController">
MenuBar.fxml:
<AnchorPane maxHeight="-Infinity" maxWidth="-Infinity" minHeight="-Infinity" minWidth="-Infinity" prefHeight="400.0" prefWidth="600.0" xmlns:fx="http://javafx.com/fxml/1" xmlns="http://javafx.com/javafx/8" fx:controller="mailbox.MenuBarController">
<children>
<MenuBar fx:id="menuBar" layoutX="0.0" layoutY="0.0">
<menus>
<Menu text="File">
<items>
<MenuItem onAction="#elimina" text="Elimina" />
</items>
</Menu>
<Menu text="Cambia Account">
<items>
<MenuItem fx:id="email1" text="filippo@hotmail.it" />
<MenuItem fx:id="email2" text="giancarlo@yahoo.it" />
<MenuItem fx:id="email3" text="alessandro@gmail.it" />
</items>
</Menu>
</menus>
</MenuBar>
</children>
Textarea.fxml:
<AnchorPane maxHeight="-Infinity" maxWidth="-Infinity" minHeight="-Infinity" minWidth="-Infinity" prefHeight="400.0" prefWidth="600.0" xmlns:fx="http://javafx.com/fxml/1" xmlns="http://javafx.com/javafx/8" fx:controller="mailbox.TextAreaController">
Textfield.fxml:
<AnchorPane maxHeight="-Infinity" maxWidth="-Infinity" minHeight="-Infinity" minWidth="-Infinity" mouseTransparent="false" prefHeight="400.0" prefWidth="600.0" xmlns="http://javafx.com/javafx/8" xmlns:fx="http://javafx.com/fxml/1" fx:controller="mailbox.TextFieldController">
EDIT:
So should I declare more than one Anchor Pane and attach them to a main Anchor Pane?
AnchorPane root = new AnchorPane();
AnchorPane lista = new AnchorPane(listLoader.load());
AnchorPane textarea = new AnchorPane(textareaLoader.load());
AnchorPane field = new AnchorPane(fieldLoader.load());
AnchorPane menu = new AnchorPane(menuLoader.load());
AnchorPane button = new AnchorPane(buttonLoader.load());
root.getChildren().addAll(lista, textarea, field, menu, button);
EDIT2: This is the output of my program, can I create it with a BorderPane? Because It automatically anchor the elements on the right, left ecc... and for example I cannot put the textfield as you can see in the image
java listview javafx observable menuitem
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
when I try to initialize the Anchor Pane a strange things happens: if I put the elements in this order I cannot click with the mouse, for example, on the elements of the list.
AnchorPane root = new AnchorPane(listLoader.load(), fieldLoader.load(), textareaLoader.load(), buttonLoader.load(), menuLoader.load());
But if I write it on this way I'm able to select the items on the list but not the items in the menubar:
AnchorPane root = new AnchorPane(fieldLoader.load(), textareaLoader.load(), buttonLoader.load(), menuLoader.load(), listLoader.load());
Do you know How should I write the elements to make at least the button, the menu and the list clickable?
This is the full code:
@Override
public void start(Stage stage) throws Exception {
FXMLLoader listLoader = new FXMLLoader(getClass().getResource("lista.fxml"));
FXMLLoader textareaLoader = new FXMLLoader(getClass().getResource("textarea.fxml"));
FXMLLoader fieldLoader = new FXMLLoader(getClass().getResource("textfield.fxml"));
FXMLLoader menuLoader = new FXMLLoader(getClass().getResource("menubar.fxml"));
FXMLLoader buttonLoader = new FXMLLoader(getClass().getResource("button.fxml"));
AnchorPane root = new AnchorPane(listLoader.load(), fieldLoader.load(), textareaLoader.load(), buttonLoader.load(), menuLoader.load());
ListController listController = listLoader.getController();
TextAreaController textareaController = textareaLoader.getController();
TextFieldController fieldController = fieldLoader.getController();
MenuBarController menuController = menuLoader.getController();
ButtonController buttonController = buttonLoader.getController();
DataModel model = new DataModel();
listController.initModel(model);
textareaController.initModel(model);
fieldController.initModel(model);
menuController.initModel(model);
buttonController.initModel(model);
Scene scene = new Scene(root, 603, 403);
stage.setScene(scene);
stage.show();
}
Lista.fxml:
<AnchorPane maxHeight="-Infinity" maxWidth="-Infinity" minHeight="-Infinity" minWidth="-Infinity" prefHeight="400.0" prefWidth="600.0" xmlns="http://javafx.com/javafx/8" xmlns:fx="http://javafx.com/fxml/1" fx:controller="mailbox.ListController">
Button.fxml:
<AnchorPane maxHeight="-Infinity" maxWidth="-Infinity" minHeight="-Infinity" minWidth="-Infinity" prefHeight="400.0" prefWidth="600.0" xmlns="http://javafx.com/javafx/8" xmlns:fx="http://javafx.com/fxml/1" fx:controller="mailbox.ButtonController">
MenuBar.fxml:
<AnchorPane maxHeight="-Infinity" maxWidth="-Infinity" minHeight="-Infinity" minWidth="-Infinity" prefHeight="400.0" prefWidth="600.0" xmlns:fx="http://javafx.com/fxml/1" xmlns="http://javafx.com/javafx/8" fx:controller="mailbox.MenuBarController">
<children>
<MenuBar fx:id="menuBar" layoutX="0.0" layoutY="0.0">
<menus>
<Menu text="File">
<items>
<MenuItem onAction="#elimina" text="Elimina" />
</items>
</Menu>
<Menu text="Cambia Account">
<items>
<MenuItem fx:id="email1" text="filippo@hotmail.it" />
<MenuItem fx:id="email2" text="giancarlo@yahoo.it" />
<MenuItem fx:id="email3" text="alessandro@gmail.it" />
</items>
</Menu>
</menus>
</MenuBar>
</children>
Textarea.fxml:
<AnchorPane maxHeight="-Infinity" maxWidth="-Infinity" minHeight="-Infinity" minWidth="-Infinity" prefHeight="400.0" prefWidth="600.0" xmlns:fx="http://javafx.com/fxml/1" xmlns="http://javafx.com/javafx/8" fx:controller="mailbox.TextAreaController">
Textfield.fxml:
<AnchorPane maxHeight="-Infinity" maxWidth="-Infinity" minHeight="-Infinity" minWidth="-Infinity" mouseTransparent="false" prefHeight="400.0" prefWidth="600.0" xmlns="http://javafx.com/javafx/8" xmlns:fx="http://javafx.com/fxml/1" fx:controller="mailbox.TextFieldController">
EDIT:
So should I declare more than one Anchor Pane and attach them to a main Anchor Pane?
AnchorPane root = new AnchorPane();
AnchorPane lista = new AnchorPane(listLoader.load());
AnchorPane textarea = new AnchorPane(textareaLoader.load());
AnchorPane field = new AnchorPane(fieldLoader.load());
AnchorPane menu = new AnchorPane(menuLoader.load());
AnchorPane button = new AnchorPane(buttonLoader.load());
root.getChildren().addAll(lista, textarea, field, menu, button);
EDIT2: This is the output of my program, can I create it with a BorderPane? Because It automatically anchor the elements on the right, left ecc... and for example I cannot put the textfield as you can see in the image
java listview javafx observable menuitem
when I try to initialize the Anchor Pane a strange things happens: if I put the elements in this order I cannot click with the mouse, for example, on the elements of the list.
AnchorPane root = new AnchorPane(listLoader.load(), fieldLoader.load(), textareaLoader.load(), buttonLoader.load(), menuLoader.load());
But if I write it on this way I'm able to select the items on the list but not the items in the menubar:
AnchorPane root = new AnchorPane(fieldLoader.load(), textareaLoader.load(), buttonLoader.load(), menuLoader.load(), listLoader.load());
Do you know How should I write the elements to make at least the button, the menu and the list clickable?
This is the full code:
@Override
public void start(Stage stage) throws Exception {
FXMLLoader listLoader = new FXMLLoader(getClass().getResource("lista.fxml"));
FXMLLoader textareaLoader = new FXMLLoader(getClass().getResource("textarea.fxml"));
FXMLLoader fieldLoader = new FXMLLoader(getClass().getResource("textfield.fxml"));
FXMLLoader menuLoader = new FXMLLoader(getClass().getResource("menubar.fxml"));
FXMLLoader buttonLoader = new FXMLLoader(getClass().getResource("button.fxml"));
AnchorPane root = new AnchorPane(listLoader.load(), fieldLoader.load(), textareaLoader.load(), buttonLoader.load(), menuLoader.load());
ListController listController = listLoader.getController();
TextAreaController textareaController = textareaLoader.getController();
TextFieldController fieldController = fieldLoader.getController();
MenuBarController menuController = menuLoader.getController();
ButtonController buttonController = buttonLoader.getController();
DataModel model = new DataModel();
listController.initModel(model);
textareaController.initModel(model);
fieldController.initModel(model);
menuController.initModel(model);
buttonController.initModel(model);
Scene scene = new Scene(root, 603, 403);
stage.setScene(scene);
stage.show();
}
Lista.fxml:
<AnchorPane maxHeight="-Infinity" maxWidth="-Infinity" minHeight="-Infinity" minWidth="-Infinity" prefHeight="400.0" prefWidth="600.0" xmlns="http://javafx.com/javafx/8" xmlns:fx="http://javafx.com/fxml/1" fx:controller="mailbox.ListController">
Button.fxml:
<AnchorPane maxHeight="-Infinity" maxWidth="-Infinity" minHeight="-Infinity" minWidth="-Infinity" prefHeight="400.0" prefWidth="600.0" xmlns="http://javafx.com/javafx/8" xmlns:fx="http://javafx.com/fxml/1" fx:controller="mailbox.ButtonController">
MenuBar.fxml:
<AnchorPane maxHeight="-Infinity" maxWidth="-Infinity" minHeight="-Infinity" minWidth="-Infinity" prefHeight="400.0" prefWidth="600.0" xmlns:fx="http://javafx.com/fxml/1" xmlns="http://javafx.com/javafx/8" fx:controller="mailbox.MenuBarController">
<children>
<MenuBar fx:id="menuBar" layoutX="0.0" layoutY="0.0">
<menus>
<Menu text="File">
<items>
<MenuItem onAction="#elimina" text="Elimina" />
</items>
</Menu>
<Menu text="Cambia Account">
<items>
<MenuItem fx:id="email1" text="filippo@hotmail.it" />
<MenuItem fx:id="email2" text="giancarlo@yahoo.it" />
<MenuItem fx:id="email3" text="alessandro@gmail.it" />
</items>
</Menu>
</menus>
</MenuBar>
</children>
Textarea.fxml:
<AnchorPane maxHeight="-Infinity" maxWidth="-Infinity" minHeight="-Infinity" minWidth="-Infinity" prefHeight="400.0" prefWidth="600.0" xmlns:fx="http://javafx.com/fxml/1" xmlns="http://javafx.com/javafx/8" fx:controller="mailbox.TextAreaController">
Textfield.fxml:
<AnchorPane maxHeight="-Infinity" maxWidth="-Infinity" minHeight="-Infinity" minWidth="-Infinity" mouseTransparent="false" prefHeight="400.0" prefWidth="600.0" xmlns="http://javafx.com/javafx/8" xmlns:fx="http://javafx.com/fxml/1" fx:controller="mailbox.TextFieldController">
EDIT:
So should I declare more than one Anchor Pane and attach them to a main Anchor Pane?
AnchorPane root = new AnchorPane();
AnchorPane lista = new AnchorPane(listLoader.load());
AnchorPane textarea = new AnchorPane(textareaLoader.load());
AnchorPane field = new AnchorPane(fieldLoader.load());
AnchorPane menu = new AnchorPane(menuLoader.load());
AnchorPane button = new AnchorPane(buttonLoader.load());
root.getChildren().addAll(lista, textarea, field, menu, button);
EDIT2: This is the output of my program, can I create it with a BorderPane? Because It automatically anchor the elements on the right, left ecc... and for example I cannot put the textfield as you can see in the image
java listview javafx observable menuitem
java listview javafx observable menuitem
edited Nov 19 at 14:31
asked Nov 19 at 12:42


Samoa Joe
206
206
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
up vote
0
down vote
accepted
Probably consecutively loaded panes have the same sizes. After loading them you get a stack of panes with the same sizes. In that case only the top most pane (added as last one) is responsive for mouse events. I would suggest to provide correct sizes and anchors for every pane.
If my thesis is incorrect please provide more code.
EDIT: if child1 and child2 had the same sizes only the blue one would be visible but still the red one would be present but underneath and all covered by blue one. It's the same situation with your app. Btw you are misusing AnchorPane. AnchorPane is designated to anchor children.
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.layout.AnchorPane;
import javafx.stage.Stage;
public class AnchorPaneTest extends Application {
public static void main(String args) {
launch(args);
}
@Override
public void start(Stage stage) throws Exception {
AnchorPane anchorPane = new AnchorPane();
AnchorPane child1 = new AnchorPane();
child1.setPrefSize(400., 600.);
child1.setStyle("-fx-background-color: red;");
AnchorPane child2 = new AnchorPane();
child2.setPrefSize(400., 300.);
child2.setStyle("-fx-background-color: blue;");
anchorPane.getChildren().addAll(child1, child2);
stage.setScene(new Scene(anchorPane));
stage.show();
}
}
I added all the fxml files. I made the design with Scene Builder and when I execute the code the output is as I desire, with none of the objects is anchored on another one.
– Samoa Joe
Nov 19 at 13:33
I've edited my answer to give you better picture.
– Przemek Krysztofiak
Nov 19 at 13:46
I've edited the question.
– Samoa Joe
Nov 19 at 13:59
1
Remove 'maxHeight="-Infinity" maxWidth="-Infinity" minHeight="-Infinity" minWidth="-Infinity" prefHeight="400.0" prefWidth="600.0"' from all fxmls you listed and run the app. This will give you a clue what your are doing wrong. Next try to use BorderPane instead of your main AnchorPane (make a desing in scene builder). Use methods BorderPane.setLeft(), setRigth(), setCenter(), etc...
– Przemek Krysztofiak
Nov 19 at 14:07
@SamoaJoe I will add you should spend a few time to understand the goal of the differentslayout
.AnchorPane
could be useful in some specific cases, but I don't see anything in your code that justify this choice.
– Pagbo
Nov 19 at 14:11
|
show 1 more comment
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
accepted
Probably consecutively loaded panes have the same sizes. After loading them you get a stack of panes with the same sizes. In that case only the top most pane (added as last one) is responsive for mouse events. I would suggest to provide correct sizes and anchors for every pane.
If my thesis is incorrect please provide more code.
EDIT: if child1 and child2 had the same sizes only the blue one would be visible but still the red one would be present but underneath and all covered by blue one. It's the same situation with your app. Btw you are misusing AnchorPane. AnchorPane is designated to anchor children.
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.layout.AnchorPane;
import javafx.stage.Stage;
public class AnchorPaneTest extends Application {
public static void main(String args) {
launch(args);
}
@Override
public void start(Stage stage) throws Exception {
AnchorPane anchorPane = new AnchorPane();
AnchorPane child1 = new AnchorPane();
child1.setPrefSize(400., 600.);
child1.setStyle("-fx-background-color: red;");
AnchorPane child2 = new AnchorPane();
child2.setPrefSize(400., 300.);
child2.setStyle("-fx-background-color: blue;");
anchorPane.getChildren().addAll(child1, child2);
stage.setScene(new Scene(anchorPane));
stage.show();
}
}
I added all the fxml files. I made the design with Scene Builder and when I execute the code the output is as I desire, with none of the objects is anchored on another one.
– Samoa Joe
Nov 19 at 13:33
I've edited my answer to give you better picture.
– Przemek Krysztofiak
Nov 19 at 13:46
I've edited the question.
– Samoa Joe
Nov 19 at 13:59
1
Remove 'maxHeight="-Infinity" maxWidth="-Infinity" minHeight="-Infinity" minWidth="-Infinity" prefHeight="400.0" prefWidth="600.0"' from all fxmls you listed and run the app. This will give you a clue what your are doing wrong. Next try to use BorderPane instead of your main AnchorPane (make a desing in scene builder). Use methods BorderPane.setLeft(), setRigth(), setCenter(), etc...
– Przemek Krysztofiak
Nov 19 at 14:07
@SamoaJoe I will add you should spend a few time to understand the goal of the differentslayout
.AnchorPane
could be useful in some specific cases, but I don't see anything in your code that justify this choice.
– Pagbo
Nov 19 at 14:11
|
show 1 more comment
up vote
0
down vote
accepted
Probably consecutively loaded panes have the same sizes. After loading them you get a stack of panes with the same sizes. In that case only the top most pane (added as last one) is responsive for mouse events. I would suggest to provide correct sizes and anchors for every pane.
If my thesis is incorrect please provide more code.
EDIT: if child1 and child2 had the same sizes only the blue one would be visible but still the red one would be present but underneath and all covered by blue one. It's the same situation with your app. Btw you are misusing AnchorPane. AnchorPane is designated to anchor children.
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.layout.AnchorPane;
import javafx.stage.Stage;
public class AnchorPaneTest extends Application {
public static void main(String args) {
launch(args);
}
@Override
public void start(Stage stage) throws Exception {
AnchorPane anchorPane = new AnchorPane();
AnchorPane child1 = new AnchorPane();
child1.setPrefSize(400., 600.);
child1.setStyle("-fx-background-color: red;");
AnchorPane child2 = new AnchorPane();
child2.setPrefSize(400., 300.);
child2.setStyle("-fx-background-color: blue;");
anchorPane.getChildren().addAll(child1, child2);
stage.setScene(new Scene(anchorPane));
stage.show();
}
}
I added all the fxml files. I made the design with Scene Builder and when I execute the code the output is as I desire, with none of the objects is anchored on another one.
– Samoa Joe
Nov 19 at 13:33
I've edited my answer to give you better picture.
– Przemek Krysztofiak
Nov 19 at 13:46
I've edited the question.
– Samoa Joe
Nov 19 at 13:59
1
Remove 'maxHeight="-Infinity" maxWidth="-Infinity" minHeight="-Infinity" minWidth="-Infinity" prefHeight="400.0" prefWidth="600.0"' from all fxmls you listed and run the app. This will give you a clue what your are doing wrong. Next try to use BorderPane instead of your main AnchorPane (make a desing in scene builder). Use methods BorderPane.setLeft(), setRigth(), setCenter(), etc...
– Przemek Krysztofiak
Nov 19 at 14:07
@SamoaJoe I will add you should spend a few time to understand the goal of the differentslayout
.AnchorPane
could be useful in some specific cases, but I don't see anything in your code that justify this choice.
– Pagbo
Nov 19 at 14:11
|
show 1 more comment
up vote
0
down vote
accepted
up vote
0
down vote
accepted
Probably consecutively loaded panes have the same sizes. After loading them you get a stack of panes with the same sizes. In that case only the top most pane (added as last one) is responsive for mouse events. I would suggest to provide correct sizes and anchors for every pane.
If my thesis is incorrect please provide more code.
EDIT: if child1 and child2 had the same sizes only the blue one would be visible but still the red one would be present but underneath and all covered by blue one. It's the same situation with your app. Btw you are misusing AnchorPane. AnchorPane is designated to anchor children.
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.layout.AnchorPane;
import javafx.stage.Stage;
public class AnchorPaneTest extends Application {
public static void main(String args) {
launch(args);
}
@Override
public void start(Stage stage) throws Exception {
AnchorPane anchorPane = new AnchorPane();
AnchorPane child1 = new AnchorPane();
child1.setPrefSize(400., 600.);
child1.setStyle("-fx-background-color: red;");
AnchorPane child2 = new AnchorPane();
child2.setPrefSize(400., 300.);
child2.setStyle("-fx-background-color: blue;");
anchorPane.getChildren().addAll(child1, child2);
stage.setScene(new Scene(anchorPane));
stage.show();
}
}
Probably consecutively loaded panes have the same sizes. After loading them you get a stack of panes with the same sizes. In that case only the top most pane (added as last one) is responsive for mouse events. I would suggest to provide correct sizes and anchors for every pane.
If my thesis is incorrect please provide more code.
EDIT: if child1 and child2 had the same sizes only the blue one would be visible but still the red one would be present but underneath and all covered by blue one. It's the same situation with your app. Btw you are misusing AnchorPane. AnchorPane is designated to anchor children.
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.layout.AnchorPane;
import javafx.stage.Stage;
public class AnchorPaneTest extends Application {
public static void main(String args) {
launch(args);
}
@Override
public void start(Stage stage) throws Exception {
AnchorPane anchorPane = new AnchorPane();
AnchorPane child1 = new AnchorPane();
child1.setPrefSize(400., 600.);
child1.setStyle("-fx-background-color: red;");
AnchorPane child2 = new AnchorPane();
child2.setPrefSize(400., 300.);
child2.setStyle("-fx-background-color: blue;");
anchorPane.getChildren().addAll(child1, child2);
stage.setScene(new Scene(anchorPane));
stage.show();
}
}
edited Nov 19 at 13:45
answered Nov 19 at 13:16
Przemek Krysztofiak
743
743
I added all the fxml files. I made the design with Scene Builder and when I execute the code the output is as I desire, with none of the objects is anchored on another one.
– Samoa Joe
Nov 19 at 13:33
I've edited my answer to give you better picture.
– Przemek Krysztofiak
Nov 19 at 13:46
I've edited the question.
– Samoa Joe
Nov 19 at 13:59
1
Remove 'maxHeight="-Infinity" maxWidth="-Infinity" minHeight="-Infinity" minWidth="-Infinity" prefHeight="400.0" prefWidth="600.0"' from all fxmls you listed and run the app. This will give you a clue what your are doing wrong. Next try to use BorderPane instead of your main AnchorPane (make a desing in scene builder). Use methods BorderPane.setLeft(), setRigth(), setCenter(), etc...
– Przemek Krysztofiak
Nov 19 at 14:07
@SamoaJoe I will add you should spend a few time to understand the goal of the differentslayout
.AnchorPane
could be useful in some specific cases, but I don't see anything in your code that justify this choice.
– Pagbo
Nov 19 at 14:11
|
show 1 more comment
I added all the fxml files. I made the design with Scene Builder and when I execute the code the output is as I desire, with none of the objects is anchored on another one.
– Samoa Joe
Nov 19 at 13:33
I've edited my answer to give you better picture.
– Przemek Krysztofiak
Nov 19 at 13:46
I've edited the question.
– Samoa Joe
Nov 19 at 13:59
1
Remove 'maxHeight="-Infinity" maxWidth="-Infinity" minHeight="-Infinity" minWidth="-Infinity" prefHeight="400.0" prefWidth="600.0"' from all fxmls you listed and run the app. This will give you a clue what your are doing wrong. Next try to use BorderPane instead of your main AnchorPane (make a desing in scene builder). Use methods BorderPane.setLeft(), setRigth(), setCenter(), etc...
– Przemek Krysztofiak
Nov 19 at 14:07
@SamoaJoe I will add you should spend a few time to understand the goal of the differentslayout
.AnchorPane
could be useful in some specific cases, but I don't see anything in your code that justify this choice.
– Pagbo
Nov 19 at 14:11
I added all the fxml files. I made the design with Scene Builder and when I execute the code the output is as I desire, with none of the objects is anchored on another one.
– Samoa Joe
Nov 19 at 13:33
I added all the fxml files. I made the design with Scene Builder and when I execute the code the output is as I desire, with none of the objects is anchored on another one.
– Samoa Joe
Nov 19 at 13:33
I've edited my answer to give you better picture.
– Przemek Krysztofiak
Nov 19 at 13:46
I've edited my answer to give you better picture.
– Przemek Krysztofiak
Nov 19 at 13:46
I've edited the question.
– Samoa Joe
Nov 19 at 13:59
I've edited the question.
– Samoa Joe
Nov 19 at 13:59
1
1
Remove 'maxHeight="-Infinity" maxWidth="-Infinity" minHeight="-Infinity" minWidth="-Infinity" prefHeight="400.0" prefWidth="600.0"' from all fxmls you listed and run the app. This will give you a clue what your are doing wrong. Next try to use BorderPane instead of your main AnchorPane (make a desing in scene builder). Use methods BorderPane.setLeft(), setRigth(), setCenter(), etc...
– Przemek Krysztofiak
Nov 19 at 14:07
Remove 'maxHeight="-Infinity" maxWidth="-Infinity" minHeight="-Infinity" minWidth="-Infinity" prefHeight="400.0" prefWidth="600.0"' from all fxmls you listed and run the app. This will give you a clue what your are doing wrong. Next try to use BorderPane instead of your main AnchorPane (make a desing in scene builder). Use methods BorderPane.setLeft(), setRigth(), setCenter(), etc...
– Przemek Krysztofiak
Nov 19 at 14:07
@SamoaJoe I will add you should spend a few time to understand the goal of the differents
layout
. AnchorPane
could be useful in some specific cases, but I don't see anything in your code that justify this choice.– Pagbo
Nov 19 at 14:11
@SamoaJoe I will add you should spend a few time to understand the goal of the differents
layout
. AnchorPane
could be useful in some specific cases, but I don't see anything in your code that justify this choice.– Pagbo
Nov 19 at 14:11
|
show 1 more comment
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53374893%2forder-of-the-elements-in-the-anchor-pane%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
WX,FuUO yCnc3sVTs6,W5Jo z6q 6Di y,U