Python 3.6.6 Popen, using sys.exit in subprocess, how to suppress the traceback/exceptions
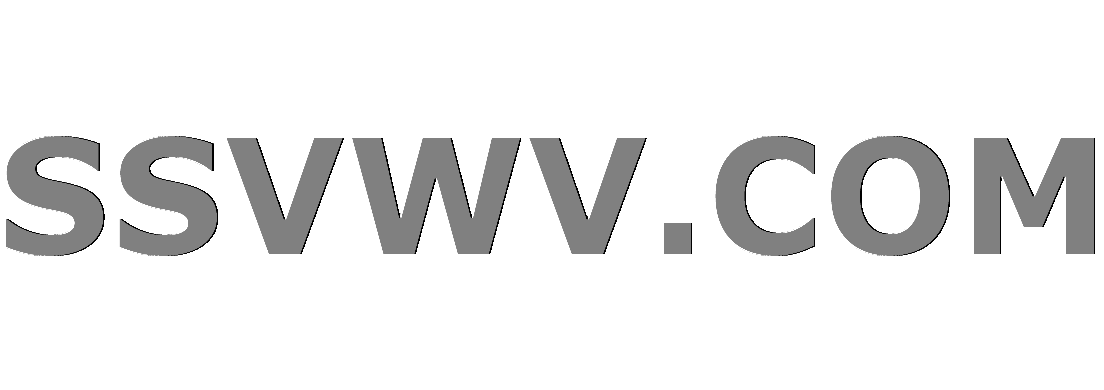
Multi tool use
up vote
1
down vote
favorite
I have a class with some functions that basically do output checks on data, the functions of this class are called using a subprocess.
Now if the output check fails the subprocess has a sys.exit call with a different code depending on which check it failed.
In the main code I have this:
try:
exitCode = 0
#import module for current test
teststr = os.path.splitext(test)[0]
os.chdir(fs.scriptFolder)
test = __import__(teststr)
#delete old output folder and create a new one
if os.path.isdir(fs.outputFolder):
rmtree(fs.outputFolder)
os.mkdir(fs.outputFolder)
# run the test passed into the function as a new subprocess
os.chdir(fs.pythonFolder)
myEnv=os.environ.copy()
myEnv["x"] = "ON"
testSubprocess = Popen(['python', test.testInfo.network + '.py', teststr], env=myEnv)
testSubprocess.wait()
result = addFields(test)
# poke the data into the postgresql database if the network ran successfully
if testSubprocess.returncode == 0:
uploadToPerfDatabase(result)
elif testSubprocess.returncode == 1:
raise Exception("Incorrect total number of rows on output, expected: " + str(test.testInfo.outputValidationProps['TotalRowCount']))
exitCode = 1
elif testSubprocess.returncode == 2:
raise Exception("Incorrect number of columns on output, expected: " + str(test.testInfo.outputValidationProps['ColumnCount']))
exitCode = 1
except Exception as e:
log.out(teststr + " failed", True)
log.out(str(e))
log.out(traceback.format_exc())
exitCode = 1
return exitCode
Now the output from this shows all traceback and python exceptions for the sys.exit calls in the subprocess.
Im actually logging all errors so I dont want anything being displayed in the command prompt unless ive printed it manually.
I'm not quite sure how to go about this.
python subprocess
add a comment |
up vote
1
down vote
favorite
I have a class with some functions that basically do output checks on data, the functions of this class are called using a subprocess.
Now if the output check fails the subprocess has a sys.exit call with a different code depending on which check it failed.
In the main code I have this:
try:
exitCode = 0
#import module for current test
teststr = os.path.splitext(test)[0]
os.chdir(fs.scriptFolder)
test = __import__(teststr)
#delete old output folder and create a new one
if os.path.isdir(fs.outputFolder):
rmtree(fs.outputFolder)
os.mkdir(fs.outputFolder)
# run the test passed into the function as a new subprocess
os.chdir(fs.pythonFolder)
myEnv=os.environ.copy()
myEnv["x"] = "ON"
testSubprocess = Popen(['python', test.testInfo.network + '.py', teststr], env=myEnv)
testSubprocess.wait()
result = addFields(test)
# poke the data into the postgresql database if the network ran successfully
if testSubprocess.returncode == 0:
uploadToPerfDatabase(result)
elif testSubprocess.returncode == 1:
raise Exception("Incorrect total number of rows on output, expected: " + str(test.testInfo.outputValidationProps['TotalRowCount']))
exitCode = 1
elif testSubprocess.returncode == 2:
raise Exception("Incorrect number of columns on output, expected: " + str(test.testInfo.outputValidationProps['ColumnCount']))
exitCode = 1
except Exception as e:
log.out(teststr + " failed", True)
log.out(str(e))
log.out(traceback.format_exc())
exitCode = 1
return exitCode
Now the output from this shows all traceback and python exceptions for the sys.exit calls in the subprocess.
Im actually logging all errors so I dont want anything being displayed in the command prompt unless ive printed it manually.
I'm not quite sure how to go about this.
python subprocess
add a comment |
up vote
1
down vote
favorite
up vote
1
down vote
favorite
I have a class with some functions that basically do output checks on data, the functions of this class are called using a subprocess.
Now if the output check fails the subprocess has a sys.exit call with a different code depending on which check it failed.
In the main code I have this:
try:
exitCode = 0
#import module for current test
teststr = os.path.splitext(test)[0]
os.chdir(fs.scriptFolder)
test = __import__(teststr)
#delete old output folder and create a new one
if os.path.isdir(fs.outputFolder):
rmtree(fs.outputFolder)
os.mkdir(fs.outputFolder)
# run the test passed into the function as a new subprocess
os.chdir(fs.pythonFolder)
myEnv=os.environ.copy()
myEnv["x"] = "ON"
testSubprocess = Popen(['python', test.testInfo.network + '.py', teststr], env=myEnv)
testSubprocess.wait()
result = addFields(test)
# poke the data into the postgresql database if the network ran successfully
if testSubprocess.returncode == 0:
uploadToPerfDatabase(result)
elif testSubprocess.returncode == 1:
raise Exception("Incorrect total number of rows on output, expected: " + str(test.testInfo.outputValidationProps['TotalRowCount']))
exitCode = 1
elif testSubprocess.returncode == 2:
raise Exception("Incorrect number of columns on output, expected: " + str(test.testInfo.outputValidationProps['ColumnCount']))
exitCode = 1
except Exception as e:
log.out(teststr + " failed", True)
log.out(str(e))
log.out(traceback.format_exc())
exitCode = 1
return exitCode
Now the output from this shows all traceback and python exceptions for the sys.exit calls in the subprocess.
Im actually logging all errors so I dont want anything being displayed in the command prompt unless ive printed it manually.
I'm not quite sure how to go about this.
python subprocess
I have a class with some functions that basically do output checks on data, the functions of this class are called using a subprocess.
Now if the output check fails the subprocess has a sys.exit call with a different code depending on which check it failed.
In the main code I have this:
try:
exitCode = 0
#import module for current test
teststr = os.path.splitext(test)[0]
os.chdir(fs.scriptFolder)
test = __import__(teststr)
#delete old output folder and create a new one
if os.path.isdir(fs.outputFolder):
rmtree(fs.outputFolder)
os.mkdir(fs.outputFolder)
# run the test passed into the function as a new subprocess
os.chdir(fs.pythonFolder)
myEnv=os.environ.copy()
myEnv["x"] = "ON"
testSubprocess = Popen(['python', test.testInfo.network + '.py', teststr], env=myEnv)
testSubprocess.wait()
result = addFields(test)
# poke the data into the postgresql database if the network ran successfully
if testSubprocess.returncode == 0:
uploadToPerfDatabase(result)
elif testSubprocess.returncode == 1:
raise Exception("Incorrect total number of rows on output, expected: " + str(test.testInfo.outputValidationProps['TotalRowCount']))
exitCode = 1
elif testSubprocess.returncode == 2:
raise Exception("Incorrect number of columns on output, expected: " + str(test.testInfo.outputValidationProps['ColumnCount']))
exitCode = 1
except Exception as e:
log.out(teststr + " failed", True)
log.out(str(e))
log.out(traceback.format_exc())
exitCode = 1
return exitCode
Now the output from this shows all traceback and python exceptions for the sys.exit calls in the subprocess.
Im actually logging all errors so I dont want anything being displayed in the command prompt unless ive printed it manually.
I'm not quite sure how to go about this.
python subprocess
python subprocess
asked Nov 19 at 12:45
M. Atkinson
103
103
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
up vote
0
down vote
accepted
You can specify stderr
to write to os.devnull
with the subprocess.DEVNULL
flag:
p = Popen(['python', '-c', 'print(1/0)'], stderr=subprocess.DEVNULL)
subprocess.DEVNULL
Special value that can be used as the stdin, stdout or stderr argument to Popen and indicates that the special file os.devnull will be used.
New in version 3.3. docs
Thanks i mustve skimmed over that flag in the docs worked like a charm on most of the output. I just get which I expected:Caused by: Python exception: 2 File "C:xperformance_branchpythonoutputValidation.py", Line 31, in onEnd sys.exit(2)
– M. Atkinson
Nov 19 at 14:01
@Methodicle That's all you get? No mention of what exception exactly and what at all is causedby: Python exception...
?
– Darkonaut
Nov 19 at 14:10
No in my logging I get the full detail that is was caused by fact output wasn't correct so the run terminated, but in the prompt it just shows that now which ill probably try and suppress too so that it's all contained in the .log file.
– M. Atkinson
Nov 19 at 14:15
@Methodicle Oh sorry, I overlooked the "which I expected" and thought you have an ongoing problem :)
– Darkonaut
Nov 19 at 14:17
Don't worry! Thanks so much for the help and quick response fairly new to python so theirs a few bits to get my head around :D
– M. Atkinson
Nov 19 at 14:18
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
accepted
You can specify stderr
to write to os.devnull
with the subprocess.DEVNULL
flag:
p = Popen(['python', '-c', 'print(1/0)'], stderr=subprocess.DEVNULL)
subprocess.DEVNULL
Special value that can be used as the stdin, stdout or stderr argument to Popen and indicates that the special file os.devnull will be used.
New in version 3.3. docs
Thanks i mustve skimmed over that flag in the docs worked like a charm on most of the output. I just get which I expected:Caused by: Python exception: 2 File "C:xperformance_branchpythonoutputValidation.py", Line 31, in onEnd sys.exit(2)
– M. Atkinson
Nov 19 at 14:01
@Methodicle That's all you get? No mention of what exception exactly and what at all is causedby: Python exception...
?
– Darkonaut
Nov 19 at 14:10
No in my logging I get the full detail that is was caused by fact output wasn't correct so the run terminated, but in the prompt it just shows that now which ill probably try and suppress too so that it's all contained in the .log file.
– M. Atkinson
Nov 19 at 14:15
@Methodicle Oh sorry, I overlooked the "which I expected" and thought you have an ongoing problem :)
– Darkonaut
Nov 19 at 14:17
Don't worry! Thanks so much for the help and quick response fairly new to python so theirs a few bits to get my head around :D
– M. Atkinson
Nov 19 at 14:18
add a comment |
up vote
0
down vote
accepted
You can specify stderr
to write to os.devnull
with the subprocess.DEVNULL
flag:
p = Popen(['python', '-c', 'print(1/0)'], stderr=subprocess.DEVNULL)
subprocess.DEVNULL
Special value that can be used as the stdin, stdout or stderr argument to Popen and indicates that the special file os.devnull will be used.
New in version 3.3. docs
Thanks i mustve skimmed over that flag in the docs worked like a charm on most of the output. I just get which I expected:Caused by: Python exception: 2 File "C:xperformance_branchpythonoutputValidation.py", Line 31, in onEnd sys.exit(2)
– M. Atkinson
Nov 19 at 14:01
@Methodicle That's all you get? No mention of what exception exactly and what at all is causedby: Python exception...
?
– Darkonaut
Nov 19 at 14:10
No in my logging I get the full detail that is was caused by fact output wasn't correct so the run terminated, but in the prompt it just shows that now which ill probably try and suppress too so that it's all contained in the .log file.
– M. Atkinson
Nov 19 at 14:15
@Methodicle Oh sorry, I overlooked the "which I expected" and thought you have an ongoing problem :)
– Darkonaut
Nov 19 at 14:17
Don't worry! Thanks so much for the help and quick response fairly new to python so theirs a few bits to get my head around :D
– M. Atkinson
Nov 19 at 14:18
add a comment |
up vote
0
down vote
accepted
up vote
0
down vote
accepted
You can specify stderr
to write to os.devnull
with the subprocess.DEVNULL
flag:
p = Popen(['python', '-c', 'print(1/0)'], stderr=subprocess.DEVNULL)
subprocess.DEVNULL
Special value that can be used as the stdin, stdout or stderr argument to Popen and indicates that the special file os.devnull will be used.
New in version 3.3. docs
You can specify stderr
to write to os.devnull
with the subprocess.DEVNULL
flag:
p = Popen(['python', '-c', 'print(1/0)'], stderr=subprocess.DEVNULL)
subprocess.DEVNULL
Special value that can be used as the stdin, stdout or stderr argument to Popen and indicates that the special file os.devnull will be used.
New in version 3.3. docs
answered Nov 19 at 13:40


Darkonaut
2,3582616
2,3582616
Thanks i mustve skimmed over that flag in the docs worked like a charm on most of the output. I just get which I expected:Caused by: Python exception: 2 File "C:xperformance_branchpythonoutputValidation.py", Line 31, in onEnd sys.exit(2)
– M. Atkinson
Nov 19 at 14:01
@Methodicle That's all you get? No mention of what exception exactly and what at all is causedby: Python exception...
?
– Darkonaut
Nov 19 at 14:10
No in my logging I get the full detail that is was caused by fact output wasn't correct so the run terminated, but in the prompt it just shows that now which ill probably try and suppress too so that it's all contained in the .log file.
– M. Atkinson
Nov 19 at 14:15
@Methodicle Oh sorry, I overlooked the "which I expected" and thought you have an ongoing problem :)
– Darkonaut
Nov 19 at 14:17
Don't worry! Thanks so much for the help and quick response fairly new to python so theirs a few bits to get my head around :D
– M. Atkinson
Nov 19 at 14:18
add a comment |
Thanks i mustve skimmed over that flag in the docs worked like a charm on most of the output. I just get which I expected:Caused by: Python exception: 2 File "C:xperformance_branchpythonoutputValidation.py", Line 31, in onEnd sys.exit(2)
– M. Atkinson
Nov 19 at 14:01
@Methodicle That's all you get? No mention of what exception exactly and what at all is causedby: Python exception...
?
– Darkonaut
Nov 19 at 14:10
No in my logging I get the full detail that is was caused by fact output wasn't correct so the run terminated, but in the prompt it just shows that now which ill probably try and suppress too so that it's all contained in the .log file.
– M. Atkinson
Nov 19 at 14:15
@Methodicle Oh sorry, I overlooked the "which I expected" and thought you have an ongoing problem :)
– Darkonaut
Nov 19 at 14:17
Don't worry! Thanks so much for the help and quick response fairly new to python so theirs a few bits to get my head around :D
– M. Atkinson
Nov 19 at 14:18
Thanks i mustve skimmed over that flag in the docs worked like a charm on most of the output. I just get which I expected:
Caused by: Python exception: 2 File "C:xperformance_branchpythonoutputValidation.py", Line 31, in onEnd sys.exit(2)
– M. Atkinson
Nov 19 at 14:01
Thanks i mustve skimmed over that flag in the docs worked like a charm on most of the output. I just get which I expected:
Caused by: Python exception: 2 File "C:xperformance_branchpythonoutputValidation.py", Line 31, in onEnd sys.exit(2)
– M. Atkinson
Nov 19 at 14:01
@Methodicle That's all you get? No mention of what exception exactly and what at all is caused
by: Python exception...
?– Darkonaut
Nov 19 at 14:10
@Methodicle That's all you get? No mention of what exception exactly and what at all is caused
by: Python exception...
?– Darkonaut
Nov 19 at 14:10
No in my logging I get the full detail that is was caused by fact output wasn't correct so the run terminated, but in the prompt it just shows that now which ill probably try and suppress too so that it's all contained in the .log file.
– M. Atkinson
Nov 19 at 14:15
No in my logging I get the full detail that is was caused by fact output wasn't correct so the run terminated, but in the prompt it just shows that now which ill probably try and suppress too so that it's all contained in the .log file.
– M. Atkinson
Nov 19 at 14:15
@Methodicle Oh sorry, I overlooked the "which I expected" and thought you have an ongoing problem :)
– Darkonaut
Nov 19 at 14:17
@Methodicle Oh sorry, I overlooked the "which I expected" and thought you have an ongoing problem :)
– Darkonaut
Nov 19 at 14:17
Don't worry! Thanks so much for the help and quick response fairly new to python so theirs a few bits to get my head around :D
– M. Atkinson
Nov 19 at 14:18
Don't worry! Thanks so much for the help and quick response fairly new to python so theirs a few bits to get my head around :D
– M. Atkinson
Nov 19 at 14:18
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53374941%2fpython-3-6-6-popen-using-sys-exit-in-subprocess-how-to-suppress-the-traceback%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
7qGzTVg6cWVnu4rg 0pF1IAh