What is the difference between React component instance property and state property?
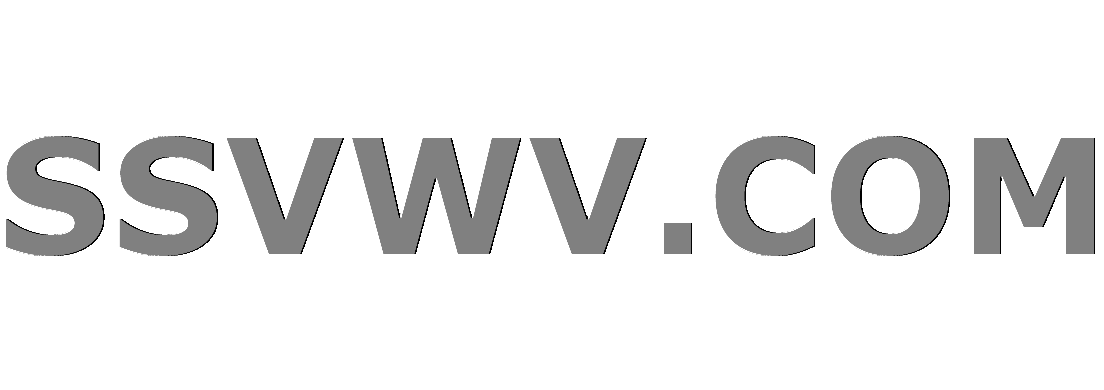
Multi tool use
up vote
5
down vote
favorite
Consider the below example
class MyApp extends Component {
counter = 0;
state = {
counter: 0
};
incrementCounter() {
this.counter = this.counter + 1;
this.setState({
counter: this.state.counter + 1
});
}
render() {
return <div>
<p>{this.counter} and {this.state.counter}</p>
<button onClick={this.incrementCounter}>Increment</button>
</div>
}
}
When I click the button I see both this.counter and this.state.counter are showing the incremented value
My question is why I have to use state? though react is capable of re-rendering all the instance properties
counter = 0;
incrementCounter() {
this.counter = this.counter + 1;
this.setState({});
}
In above snippet, just calling this.setState({}) is doing the trick, then why should I use this.state property for storing my component state?
javascript reactjs
add a comment |
up vote
5
down vote
favorite
Consider the below example
class MyApp extends Component {
counter = 0;
state = {
counter: 0
};
incrementCounter() {
this.counter = this.counter + 1;
this.setState({
counter: this.state.counter + 1
});
}
render() {
return <div>
<p>{this.counter} and {this.state.counter}</p>
<button onClick={this.incrementCounter}>Increment</button>
</div>
}
}
When I click the button I see both this.counter and this.state.counter are showing the incremented value
My question is why I have to use state? though react is capable of re-rendering all the instance properties
counter = 0;
incrementCounter() {
this.counter = this.counter + 1;
this.setState({});
}
In above snippet, just calling this.setState({}) is doing the trick, then why should I use this.state property for storing my component state?
javascript reactjs
add a comment |
up vote
5
down vote
favorite
up vote
5
down vote
favorite
Consider the below example
class MyApp extends Component {
counter = 0;
state = {
counter: 0
};
incrementCounter() {
this.counter = this.counter + 1;
this.setState({
counter: this.state.counter + 1
});
}
render() {
return <div>
<p>{this.counter} and {this.state.counter}</p>
<button onClick={this.incrementCounter}>Increment</button>
</div>
}
}
When I click the button I see both this.counter and this.state.counter are showing the incremented value
My question is why I have to use state? though react is capable of re-rendering all the instance properties
counter = 0;
incrementCounter() {
this.counter = this.counter + 1;
this.setState({});
}
In above snippet, just calling this.setState({}) is doing the trick, then why should I use this.state property for storing my component state?
javascript reactjs
Consider the below example
class MyApp extends Component {
counter = 0;
state = {
counter: 0
};
incrementCounter() {
this.counter = this.counter + 1;
this.setState({
counter: this.state.counter + 1
});
}
render() {
return <div>
<p>{this.counter} and {this.state.counter}</p>
<button onClick={this.incrementCounter}>Increment</button>
</div>
}
}
When I click the button I see both this.counter and this.state.counter are showing the incremented value
My question is why I have to use state? though react is capable of re-rendering all the instance properties
counter = 0;
incrementCounter() {
this.counter = this.counter + 1;
this.setState({});
}
In above snippet, just calling this.setState({}) is doing the trick, then why should I use this.state property for storing my component state?
javascript reactjs
javascript reactjs
asked Nov 19 at 12:45
lsk
383
383
add a comment |
add a comment |
2 Answers
2
active
oldest
votes
up vote
5
down vote
accepted
state
and instance properties
serve different purposes. While calling setState with empty arguments will cause a render and will reflect the updated instance properties, state can be used for many more features like
comparing prevState
and currentState
in shouldComponentUpdate to decide whether you want to render or not, or in lifecycle method like componentDidUpdate where you can take an action based on state change.
state is a special instance property used by react to serve special purposes. Also in setState
, state updates are batched for performance reasons and state updates happen asynchronously unlike class variable updates which happen synchronously. A class variable won't have these features.
Also when you supply a class variable as prop to the component, a change in this class variable can't be differentiated in the lifecycle methods of the child component unless you are creating a new instance of the variable yourself. React does it with state
property for you already
1
This answer does a good job at explaining the uses and bwnefits of state, but it makes it sound like instance variables should never be used. Might be worth including a note about how instance variously are set synchronously while state variables are set asychronously. In some situations, it can be very useful to be able to set a property sychronously. One example that comes to mind is animation interrupts. Or, if you are tracking the cursor/touch point position, etc...
– Jared Goguen
Nov 19 at 13:28
Thank you, I got the point now
– lsk
Nov 19 at 18:06
add a comment |
up vote
2
down vote
Both have different purpose. Rule of thumb is:
- Use
state
to store data if it is involved in rendering or data flow (i.e. if its used directly or indirectly in render method) - Use
other instance fields
to store data if value is NOT involved in rendering or data flow (to prevent rendering on change of data) e.g. to store a timer ID that is not used in render method. See TimerID example in official docs to understand this valid case.
If some value isn’t used for rendering or data flow (for example, a timer ID), you don’t have
to put it in the state. Such values can be defined as fields on the
component instance
Reference: https://reactjs.org/docs/react-component.html#state
add a comment |
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
5
down vote
accepted
state
and instance properties
serve different purposes. While calling setState with empty arguments will cause a render and will reflect the updated instance properties, state can be used for many more features like
comparing prevState
and currentState
in shouldComponentUpdate to decide whether you want to render or not, or in lifecycle method like componentDidUpdate where you can take an action based on state change.
state is a special instance property used by react to serve special purposes. Also in setState
, state updates are batched for performance reasons and state updates happen asynchronously unlike class variable updates which happen synchronously. A class variable won't have these features.
Also when you supply a class variable as prop to the component, a change in this class variable can't be differentiated in the lifecycle methods of the child component unless you are creating a new instance of the variable yourself. React does it with state
property for you already
1
This answer does a good job at explaining the uses and bwnefits of state, but it makes it sound like instance variables should never be used. Might be worth including a note about how instance variously are set synchronously while state variables are set asychronously. In some situations, it can be very useful to be able to set a property sychronously. One example that comes to mind is animation interrupts. Or, if you are tracking the cursor/touch point position, etc...
– Jared Goguen
Nov 19 at 13:28
Thank you, I got the point now
– lsk
Nov 19 at 18:06
add a comment |
up vote
5
down vote
accepted
state
and instance properties
serve different purposes. While calling setState with empty arguments will cause a render and will reflect the updated instance properties, state can be used for many more features like
comparing prevState
and currentState
in shouldComponentUpdate to decide whether you want to render or not, or in lifecycle method like componentDidUpdate where you can take an action based on state change.
state is a special instance property used by react to serve special purposes. Also in setState
, state updates are batched for performance reasons and state updates happen asynchronously unlike class variable updates which happen synchronously. A class variable won't have these features.
Also when you supply a class variable as prop to the component, a change in this class variable can't be differentiated in the lifecycle methods of the child component unless you are creating a new instance of the variable yourself. React does it with state
property for you already
1
This answer does a good job at explaining the uses and bwnefits of state, but it makes it sound like instance variables should never be used. Might be worth including a note about how instance variously are set synchronously while state variables are set asychronously. In some situations, it can be very useful to be able to set a property sychronously. One example that comes to mind is animation interrupts. Or, if you are tracking the cursor/touch point position, etc...
– Jared Goguen
Nov 19 at 13:28
Thank you, I got the point now
– lsk
Nov 19 at 18:06
add a comment |
up vote
5
down vote
accepted
up vote
5
down vote
accepted
state
and instance properties
serve different purposes. While calling setState with empty arguments will cause a render and will reflect the updated instance properties, state can be used for many more features like
comparing prevState
and currentState
in shouldComponentUpdate to decide whether you want to render or not, or in lifecycle method like componentDidUpdate where you can take an action based on state change.
state is a special instance property used by react to serve special purposes. Also in setState
, state updates are batched for performance reasons and state updates happen asynchronously unlike class variable updates which happen synchronously. A class variable won't have these features.
Also when you supply a class variable as prop to the component, a change in this class variable can't be differentiated in the lifecycle methods of the child component unless you are creating a new instance of the variable yourself. React does it with state
property for you already
state
and instance properties
serve different purposes. While calling setState with empty arguments will cause a render and will reflect the updated instance properties, state can be used for many more features like
comparing prevState
and currentState
in shouldComponentUpdate to decide whether you want to render or not, or in lifecycle method like componentDidUpdate where you can take an action based on state change.
state is a special instance property used by react to serve special purposes. Also in setState
, state updates are batched for performance reasons and state updates happen asynchronously unlike class variable updates which happen synchronously. A class variable won't have these features.
Also when you supply a class variable as prop to the component, a change in this class variable can't be differentiated in the lifecycle methods of the child component unless you are creating a new instance of the variable yourself. React does it with state
property for you already
edited Nov 19 at 13:32
answered Nov 19 at 12:54


Shubham Khatri
75.8k1385125
75.8k1385125
1
This answer does a good job at explaining the uses and bwnefits of state, but it makes it sound like instance variables should never be used. Might be worth including a note about how instance variously are set synchronously while state variables are set asychronously. In some situations, it can be very useful to be able to set a property sychronously. One example that comes to mind is animation interrupts. Or, if you are tracking the cursor/touch point position, etc...
– Jared Goguen
Nov 19 at 13:28
Thank you, I got the point now
– lsk
Nov 19 at 18:06
add a comment |
1
This answer does a good job at explaining the uses and bwnefits of state, but it makes it sound like instance variables should never be used. Might be worth including a note about how instance variously are set synchronously while state variables are set asychronously. In some situations, it can be very useful to be able to set a property sychronously. One example that comes to mind is animation interrupts. Or, if you are tracking the cursor/touch point position, etc...
– Jared Goguen
Nov 19 at 13:28
Thank you, I got the point now
– lsk
Nov 19 at 18:06
1
1
This answer does a good job at explaining the uses and bwnefits of state, but it makes it sound like instance variables should never be used. Might be worth including a note about how instance variously are set synchronously while state variables are set asychronously. In some situations, it can be very useful to be able to set a property sychronously. One example that comes to mind is animation interrupts. Or, if you are tracking the cursor/touch point position, etc...
– Jared Goguen
Nov 19 at 13:28
This answer does a good job at explaining the uses and bwnefits of state, but it makes it sound like instance variables should never be used. Might be worth including a note about how instance variously are set synchronously while state variables are set asychronously. In some situations, it can be very useful to be able to set a property sychronously. One example that comes to mind is animation interrupts. Or, if you are tracking the cursor/touch point position, etc...
– Jared Goguen
Nov 19 at 13:28
Thank you, I got the point now
– lsk
Nov 19 at 18:06
Thank you, I got the point now
– lsk
Nov 19 at 18:06
add a comment |
up vote
2
down vote
Both have different purpose. Rule of thumb is:
- Use
state
to store data if it is involved in rendering or data flow (i.e. if its used directly or indirectly in render method) - Use
other instance fields
to store data if value is NOT involved in rendering or data flow (to prevent rendering on change of data) e.g. to store a timer ID that is not used in render method. See TimerID example in official docs to understand this valid case.
If some value isn’t used for rendering or data flow (for example, a timer ID), you don’t have
to put it in the state. Such values can be defined as fields on the
component instance
Reference: https://reactjs.org/docs/react-component.html#state
add a comment |
up vote
2
down vote
Both have different purpose. Rule of thumb is:
- Use
state
to store data if it is involved in rendering or data flow (i.e. if its used directly or indirectly in render method) - Use
other instance fields
to store data if value is NOT involved in rendering or data flow (to prevent rendering on change of data) e.g. to store a timer ID that is not used in render method. See TimerID example in official docs to understand this valid case.
If some value isn’t used for rendering or data flow (for example, a timer ID), you don’t have
to put it in the state. Such values can be defined as fields on the
component instance
Reference: https://reactjs.org/docs/react-component.html#state
add a comment |
up vote
2
down vote
up vote
2
down vote
Both have different purpose. Rule of thumb is:
- Use
state
to store data if it is involved in rendering or data flow (i.e. if its used directly or indirectly in render method) - Use
other instance fields
to store data if value is NOT involved in rendering or data flow (to prevent rendering on change of data) e.g. to store a timer ID that is not used in render method. See TimerID example in official docs to understand this valid case.
If some value isn’t used for rendering or data flow (for example, a timer ID), you don’t have
to put it in the state. Such values can be defined as fields on the
component instance
Reference: https://reactjs.org/docs/react-component.html#state
Both have different purpose. Rule of thumb is:
- Use
state
to store data if it is involved in rendering or data flow (i.e. if its used directly or indirectly in render method) - Use
other instance fields
to store data if value is NOT involved in rendering or data flow (to prevent rendering on change of data) e.g. to store a timer ID that is not used in render method. See TimerID example in official docs to understand this valid case.
If some value isn’t used for rendering or data flow (for example, a timer ID), you don’t have
to put it in the state. Such values can be defined as fields on the
component instance
Reference: https://reactjs.org/docs/react-component.html#state
edited Nov 19 at 17:41
answered Nov 19 at 13:20


Abdul Rauf
2,09832144
2,09832144
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53374946%2fwhat-is-the-difference-between-react-component-instance-property-and-state-prope%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
A7knieNDxOrwzAK0M2E6P,gLZMR sK,1tz9jgsWaOwGH,P2Oe