Get the highest values of the dictionary with keys until reaching given limit
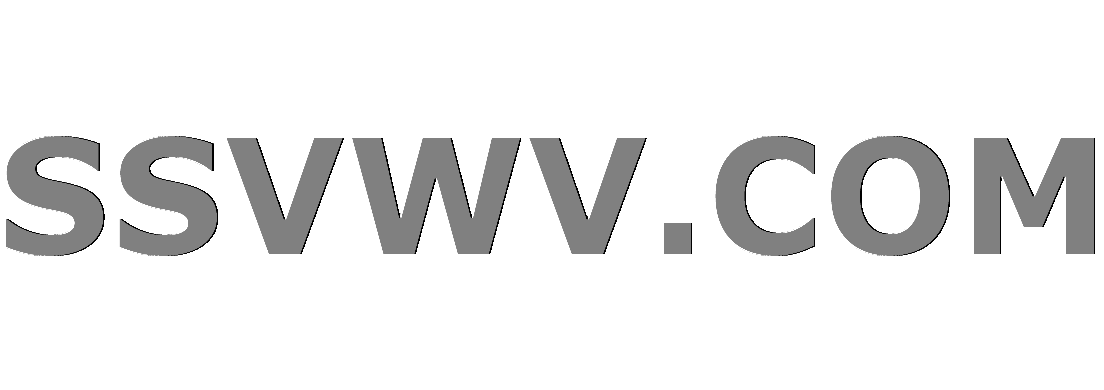
Multi tool use
given dictionary and limit for the number of keys for the new dictionary. In the new dictionary we should have the highest number of values from given dictionary.
the given is:
dict = {'apple':5, 'pears':4, 'orange':3, 'kiwi':3, 'banana':1
I want to get a new dictionary with the highest value with key.
new is
{'apple':5}
because there should be only one
if the limit is 2.
{'apple':5, 'pears':4}
because there are three values but the limit is 2 it we don't take anything. I tried
if the limit is 3. It's still
{'apple':5, 'pears':4}
because I can't add orange. If I add it will be more than the limit.
new = {}
while len(new) < limit:
then I have to append the highest value with key in new until I reach
the limit. I should not add the key if it will more than the limit.
python list dictionary
add a comment |
given dictionary and limit for the number of keys for the new dictionary. In the new dictionary we should have the highest number of values from given dictionary.
the given is:
dict = {'apple':5, 'pears':4, 'orange':3, 'kiwi':3, 'banana':1
I want to get a new dictionary with the highest value with key.
new is
{'apple':5}
because there should be only one
if the limit is 2.
{'apple':5, 'pears':4}
because there are three values but the limit is 2 it we don't take anything. I tried
if the limit is 3. It's still
{'apple':5, 'pears':4}
because I can't add orange. If I add it will be more than the limit.
new = {}
while len(new) < limit:
then I have to append the highest value with key in new until I reach
the limit. I should not add the key if it will more than the limit.
python list dictionary
add a comment |
given dictionary and limit for the number of keys for the new dictionary. In the new dictionary we should have the highest number of values from given dictionary.
the given is:
dict = {'apple':5, 'pears':4, 'orange':3, 'kiwi':3, 'banana':1
I want to get a new dictionary with the highest value with key.
new is
{'apple':5}
because there should be only one
if the limit is 2.
{'apple':5, 'pears':4}
because there are three values but the limit is 2 it we don't take anything. I tried
if the limit is 3. It's still
{'apple':5, 'pears':4}
because I can't add orange. If I add it will be more than the limit.
new = {}
while len(new) < limit:
then I have to append the highest value with key in new until I reach
the limit. I should not add the key if it will more than the limit.
python list dictionary
given dictionary and limit for the number of keys for the new dictionary. In the new dictionary we should have the highest number of values from given dictionary.
the given is:
dict = {'apple':5, 'pears':4, 'orange':3, 'kiwi':3, 'banana':1
I want to get a new dictionary with the highest value with key.
new is
{'apple':5}
because there should be only one
if the limit is 2.
{'apple':5, 'pears':4}
because there are three values but the limit is 2 it we don't take anything. I tried
if the limit is 3. It's still
{'apple':5, 'pears':4}
because I can't add orange. If I add it will be more than the limit.
new = {}
while len(new) < limit:
then I have to append the highest value with key in new until I reach
the limit. I should not add the key if it will more than the limit.
python list dictionary
python list dictionary
asked Nov 21 '18 at 3:55
Comp
456
456
add a comment |
add a comment |
3 Answers
3
active
oldest
votes
I believe your problem is that you have
while len(new) < limit:
it needs to be while len(new) <= limit:
add a comment |
Whole code being a function:
def f(d,limit):
return dict(sorted(d.items(),key=lambda x: -x[1])[:limit])
And now:
print(f(d,1))
Is:
{'apple': 5}
And:
print(f(d,2))
Is:
{'apple': 5, 'pears': 4}
Note that if the dictionary is always sorted by values like the dictionary you have now, do:
def f(d,limit):
return dict(d.items()[:limit])
add a comment |
You can just use most_common()
from collections.Counter()
:
from collections import Counter
def largest(dct, n):
return dict(Counter(dct).most_common(n))
print(largest(dct={'apple':5, 'pears':4, 'orange':3, 'kiwi':3, 'banana':1}, n=2))
# {'apple': 5, 'pears': 4}
print(largest(dct={'apple':5, 'pears':4, 'orange':3, 'kiwi':3, 'banana':1}, n=3))
# {'apple': 5, 'pears': 4, 'orange': 3}
Or even with heapq.nlargest()
:
from heapq import nlargest
from operator import itemgetter
def largest(dct, n):
return dict(nlargest(n, dct.items(), key=itemgetter(1)))
print(largest(dct={'apple':5, 'pears':4, 'orange':3, 'kiwi':3, 'banana':1}, n=2))
# {'apple': 5, 'pears': 4}
print(largest(dct={'apple':5, 'pears':4, 'orange':3, 'kiwi':3, 'banana':1}, n=3))
# {'apple': 5, 'pears': 4, 'orange': 3}
It shouldn't be {'apple': 5, 'pears': 4, 'orange': 3} when n=3 because you should also include kiwi if you include orange but if you include kiwi then it will exceed the limit so we shouldn't include both of them.
– Comp
Nov 21 '18 at 18:35
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53405044%2fget-the-highest-values-of-the-dictionary-with-keys-until-reaching-given-limit%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
I believe your problem is that you have
while len(new) < limit:
it needs to be while len(new) <= limit:
add a comment |
I believe your problem is that you have
while len(new) < limit:
it needs to be while len(new) <= limit:
add a comment |
I believe your problem is that you have
while len(new) < limit:
it needs to be while len(new) <= limit:
I believe your problem is that you have
while len(new) < limit:
it needs to be while len(new) <= limit:
answered Nov 21 '18 at 3:58
calisurfer
2299
2299
add a comment |
add a comment |
Whole code being a function:
def f(d,limit):
return dict(sorted(d.items(),key=lambda x: -x[1])[:limit])
And now:
print(f(d,1))
Is:
{'apple': 5}
And:
print(f(d,2))
Is:
{'apple': 5, 'pears': 4}
Note that if the dictionary is always sorted by values like the dictionary you have now, do:
def f(d,limit):
return dict(d.items()[:limit])
add a comment |
Whole code being a function:
def f(d,limit):
return dict(sorted(d.items(),key=lambda x: -x[1])[:limit])
And now:
print(f(d,1))
Is:
{'apple': 5}
And:
print(f(d,2))
Is:
{'apple': 5, 'pears': 4}
Note that if the dictionary is always sorted by values like the dictionary you have now, do:
def f(d,limit):
return dict(d.items()[:limit])
add a comment |
Whole code being a function:
def f(d,limit):
return dict(sorted(d.items(),key=lambda x: -x[1])[:limit])
And now:
print(f(d,1))
Is:
{'apple': 5}
And:
print(f(d,2))
Is:
{'apple': 5, 'pears': 4}
Note that if the dictionary is always sorted by values like the dictionary you have now, do:
def f(d,limit):
return dict(d.items()[:limit])
Whole code being a function:
def f(d,limit):
return dict(sorted(d.items(),key=lambda x: -x[1])[:limit])
And now:
print(f(d,1))
Is:
{'apple': 5}
And:
print(f(d,2))
Is:
{'apple': 5, 'pears': 4}
Note that if the dictionary is always sorted by values like the dictionary you have now, do:
def f(d,limit):
return dict(d.items()[:limit])
answered Nov 21 '18 at 3:59


U9-Forward
13.3k21237
13.3k21237
add a comment |
add a comment |
You can just use most_common()
from collections.Counter()
:
from collections import Counter
def largest(dct, n):
return dict(Counter(dct).most_common(n))
print(largest(dct={'apple':5, 'pears':4, 'orange':3, 'kiwi':3, 'banana':1}, n=2))
# {'apple': 5, 'pears': 4}
print(largest(dct={'apple':5, 'pears':4, 'orange':3, 'kiwi':3, 'banana':1}, n=3))
# {'apple': 5, 'pears': 4, 'orange': 3}
Or even with heapq.nlargest()
:
from heapq import nlargest
from operator import itemgetter
def largest(dct, n):
return dict(nlargest(n, dct.items(), key=itemgetter(1)))
print(largest(dct={'apple':5, 'pears':4, 'orange':3, 'kiwi':3, 'banana':1}, n=2))
# {'apple': 5, 'pears': 4}
print(largest(dct={'apple':5, 'pears':4, 'orange':3, 'kiwi':3, 'banana':1}, n=3))
# {'apple': 5, 'pears': 4, 'orange': 3}
It shouldn't be {'apple': 5, 'pears': 4, 'orange': 3} when n=3 because you should also include kiwi if you include orange but if you include kiwi then it will exceed the limit so we shouldn't include both of them.
– Comp
Nov 21 '18 at 18:35
add a comment |
You can just use most_common()
from collections.Counter()
:
from collections import Counter
def largest(dct, n):
return dict(Counter(dct).most_common(n))
print(largest(dct={'apple':5, 'pears':4, 'orange':3, 'kiwi':3, 'banana':1}, n=2))
# {'apple': 5, 'pears': 4}
print(largest(dct={'apple':5, 'pears':4, 'orange':3, 'kiwi':3, 'banana':1}, n=3))
# {'apple': 5, 'pears': 4, 'orange': 3}
Or even with heapq.nlargest()
:
from heapq import nlargest
from operator import itemgetter
def largest(dct, n):
return dict(nlargest(n, dct.items(), key=itemgetter(1)))
print(largest(dct={'apple':5, 'pears':4, 'orange':3, 'kiwi':3, 'banana':1}, n=2))
# {'apple': 5, 'pears': 4}
print(largest(dct={'apple':5, 'pears':4, 'orange':3, 'kiwi':3, 'banana':1}, n=3))
# {'apple': 5, 'pears': 4, 'orange': 3}
It shouldn't be {'apple': 5, 'pears': 4, 'orange': 3} when n=3 because you should also include kiwi if you include orange but if you include kiwi then it will exceed the limit so we shouldn't include both of them.
– Comp
Nov 21 '18 at 18:35
add a comment |
You can just use most_common()
from collections.Counter()
:
from collections import Counter
def largest(dct, n):
return dict(Counter(dct).most_common(n))
print(largest(dct={'apple':5, 'pears':4, 'orange':3, 'kiwi':3, 'banana':1}, n=2))
# {'apple': 5, 'pears': 4}
print(largest(dct={'apple':5, 'pears':4, 'orange':3, 'kiwi':3, 'banana':1}, n=3))
# {'apple': 5, 'pears': 4, 'orange': 3}
Or even with heapq.nlargest()
:
from heapq import nlargest
from operator import itemgetter
def largest(dct, n):
return dict(nlargest(n, dct.items(), key=itemgetter(1)))
print(largest(dct={'apple':5, 'pears':4, 'orange':3, 'kiwi':3, 'banana':1}, n=2))
# {'apple': 5, 'pears': 4}
print(largest(dct={'apple':5, 'pears':4, 'orange':3, 'kiwi':3, 'banana':1}, n=3))
# {'apple': 5, 'pears': 4, 'orange': 3}
You can just use most_common()
from collections.Counter()
:
from collections import Counter
def largest(dct, n):
return dict(Counter(dct).most_common(n))
print(largest(dct={'apple':5, 'pears':4, 'orange':3, 'kiwi':3, 'banana':1}, n=2))
# {'apple': 5, 'pears': 4}
print(largest(dct={'apple':5, 'pears':4, 'orange':3, 'kiwi':3, 'banana':1}, n=3))
# {'apple': 5, 'pears': 4, 'orange': 3}
Or even with heapq.nlargest()
:
from heapq import nlargest
from operator import itemgetter
def largest(dct, n):
return dict(nlargest(n, dct.items(), key=itemgetter(1)))
print(largest(dct={'apple':5, 'pears':4, 'orange':3, 'kiwi':3, 'banana':1}, n=2))
# {'apple': 5, 'pears': 4}
print(largest(dct={'apple':5, 'pears':4, 'orange':3, 'kiwi':3, 'banana':1}, n=3))
# {'apple': 5, 'pears': 4, 'orange': 3}
edited Nov 21 '18 at 4:30
answered Nov 21 '18 at 4:23


RoadRunner
10.7k31340
10.7k31340
It shouldn't be {'apple': 5, 'pears': 4, 'orange': 3} when n=3 because you should also include kiwi if you include orange but if you include kiwi then it will exceed the limit so we shouldn't include both of them.
– Comp
Nov 21 '18 at 18:35
add a comment |
It shouldn't be {'apple': 5, 'pears': 4, 'orange': 3} when n=3 because you should also include kiwi if you include orange but if you include kiwi then it will exceed the limit so we shouldn't include both of them.
– Comp
Nov 21 '18 at 18:35
It shouldn't be {'apple': 5, 'pears': 4, 'orange': 3} when n=3 because you should also include kiwi if you include orange but if you include kiwi then it will exceed the limit so we shouldn't include both of them.
– Comp
Nov 21 '18 at 18:35
It shouldn't be {'apple': 5, 'pears': 4, 'orange': 3} when n=3 because you should also include kiwi if you include orange but if you include kiwi then it will exceed the limit so we shouldn't include both of them.
– Comp
Nov 21 '18 at 18:35
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53405044%2fget-the-highest-values-of-the-dictionary-with-keys-until-reaching-given-limit%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
R,1pavHf81jQ0,IiZOTxWo,uSh Jp6dNi9,KE 1Szmj1iaXqs7hM nB3,asUw 5pmBxsPEeeZ,A7M,E 48Y8Kq