How to go from JWK to accessing claims in tokens provided by my IDP in Go?
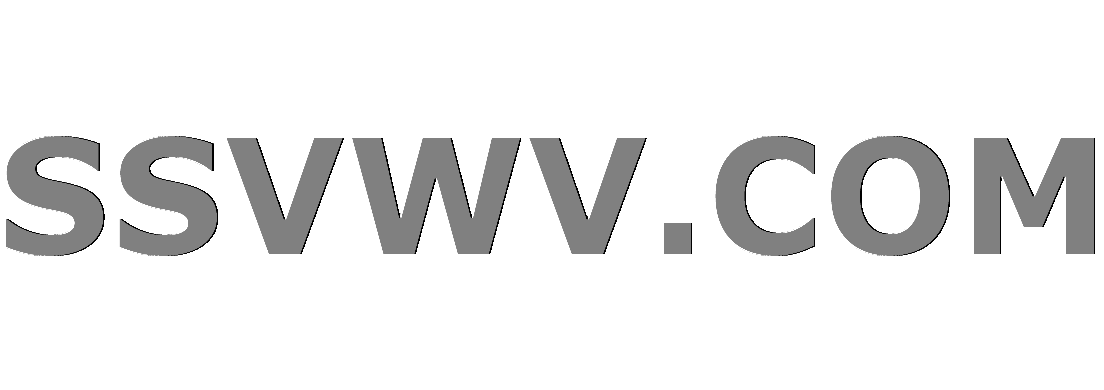
Multi tool use
I'm having a lot of trouble going from a well-known.json url to getting the claims and using them internally.
My biggest issue right now is parsing the JWK from a well-known json string to a public key to verify my tokens with.
import (
"fmt"
"github.com/dgrijalva/jwt-go"
"github.com/lestrrat-go/jwx/jwk"
)
func main() {
// I have a JWK that contains a key encoded using RS256
set, _ := jwk.ParseString(jwkString)
// This doesn't return a public key
// Is this the right way to get a public key back from the string?
publicKey, _ := set.Keys[0].Materialize()
token, _ := jwt.Parse("<token string>", func(*jwt.Token) (interface{}, error) {
return byte(publicKey), nil
})
// Do I have to manually cast the claims to typed variables for use?
email := fmt.Sprint(token.Claims.(jwt.MapClaims)["email"])
fmt.Println("email " + email)
}
go
add a comment |
I'm having a lot of trouble going from a well-known.json url to getting the claims and using them internally.
My biggest issue right now is parsing the JWK from a well-known json string to a public key to verify my tokens with.
import (
"fmt"
"github.com/dgrijalva/jwt-go"
"github.com/lestrrat-go/jwx/jwk"
)
func main() {
// I have a JWK that contains a key encoded using RS256
set, _ := jwk.ParseString(jwkString)
// This doesn't return a public key
// Is this the right way to get a public key back from the string?
publicKey, _ := set.Keys[0].Materialize()
token, _ := jwt.Parse("<token string>", func(*jwt.Token) (interface{}, error) {
return byte(publicKey), nil
})
// Do I have to manually cast the claims to typed variables for use?
email := fmt.Sprint(token.Claims.(jwt.MapClaims)["email"])
fmt.Println("email " + email)
}
go
What libraries arejwk
andjwt
? (Can you addimport
statements to your example?)
– David Maze
Nov 24 '18 at 12:50
@DavidMaze added - though feel free to suggest better alternatives.
– David Alsh
Nov 24 '18 at 12:51
Oh wow, it actually already works! I think I am getting confused about what I am expecting as a return result from.Materialze()
– David Alsh
Nov 24 '18 at 12:53
add a comment |
I'm having a lot of trouble going from a well-known.json url to getting the claims and using them internally.
My biggest issue right now is parsing the JWK from a well-known json string to a public key to verify my tokens with.
import (
"fmt"
"github.com/dgrijalva/jwt-go"
"github.com/lestrrat-go/jwx/jwk"
)
func main() {
// I have a JWK that contains a key encoded using RS256
set, _ := jwk.ParseString(jwkString)
// This doesn't return a public key
// Is this the right way to get a public key back from the string?
publicKey, _ := set.Keys[0].Materialize()
token, _ := jwt.Parse("<token string>", func(*jwt.Token) (interface{}, error) {
return byte(publicKey), nil
})
// Do I have to manually cast the claims to typed variables for use?
email := fmt.Sprint(token.Claims.(jwt.MapClaims)["email"])
fmt.Println("email " + email)
}
go
I'm having a lot of trouble going from a well-known.json url to getting the claims and using them internally.
My biggest issue right now is parsing the JWK from a well-known json string to a public key to verify my tokens with.
import (
"fmt"
"github.com/dgrijalva/jwt-go"
"github.com/lestrrat-go/jwx/jwk"
)
func main() {
// I have a JWK that contains a key encoded using RS256
set, _ := jwk.ParseString(jwkString)
// This doesn't return a public key
// Is this the right way to get a public key back from the string?
publicKey, _ := set.Keys[0].Materialize()
token, _ := jwt.Parse("<token string>", func(*jwt.Token) (interface{}, error) {
return byte(publicKey), nil
})
// Do I have to manually cast the claims to typed variables for use?
email := fmt.Sprint(token.Claims.(jwt.MapClaims)["email"])
fmt.Println("email " + email)
}
go
go
edited Nov 24 '18 at 12:50
David Alsh
asked Nov 24 '18 at 12:44
David AlshDavid Alsh
9211918
9211918
What libraries arejwk
andjwt
? (Can you addimport
statements to your example?)
– David Maze
Nov 24 '18 at 12:50
@DavidMaze added - though feel free to suggest better alternatives.
– David Alsh
Nov 24 '18 at 12:51
Oh wow, it actually already works! I think I am getting confused about what I am expecting as a return result from.Materialze()
– David Alsh
Nov 24 '18 at 12:53
add a comment |
What libraries arejwk
andjwt
? (Can you addimport
statements to your example?)
– David Maze
Nov 24 '18 at 12:50
@DavidMaze added - though feel free to suggest better alternatives.
– David Alsh
Nov 24 '18 at 12:51
Oh wow, it actually already works! I think I am getting confused about what I am expecting as a return result from.Materialze()
– David Alsh
Nov 24 '18 at 12:53
What libraries are
jwk
and jwt
? (Can you add import
statements to your example?)– David Maze
Nov 24 '18 at 12:50
What libraries are
jwk
and jwt
? (Can you add import
statements to your example?)– David Maze
Nov 24 '18 at 12:50
@DavidMaze added - though feel free to suggest better alternatives.
– David Alsh
Nov 24 '18 at 12:51
@DavidMaze added - though feel free to suggest better alternatives.
– David Alsh
Nov 24 '18 at 12:51
Oh wow, it actually already works! I think I am getting confused about what I am expecting as a return result from
.Materialze()
– David Alsh
Nov 24 '18 at 12:53
Oh wow, it actually already works! I think I am getting confused about what I am expecting as a return result from
.Materialze()
– David Alsh
Nov 24 '18 at 12:53
add a comment |
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53458280%2fhow-to-go-from-jwk-to-accessing-claims-in-tokens-provided-by-my-idp-in-go%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53458280%2fhow-to-go-from-jwk-to-accessing-claims-in-tokens-provided-by-my-idp-in-go%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
3,LU sF6HhyNOwEAbD6qiyuN7w5SpUMW7,xC7DXb zBJvyV1ix5W
What libraries are
jwk
andjwt
? (Can you addimport
statements to your example?)– David Maze
Nov 24 '18 at 12:50
@DavidMaze added - though feel free to suggest better alternatives.
– David Alsh
Nov 24 '18 at 12:51
Oh wow, it actually already works! I think I am getting confused about what I am expecting as a return result from
.Materialze()
– David Alsh
Nov 24 '18 at 12:53