Numpy array function returns inconsistent shapes
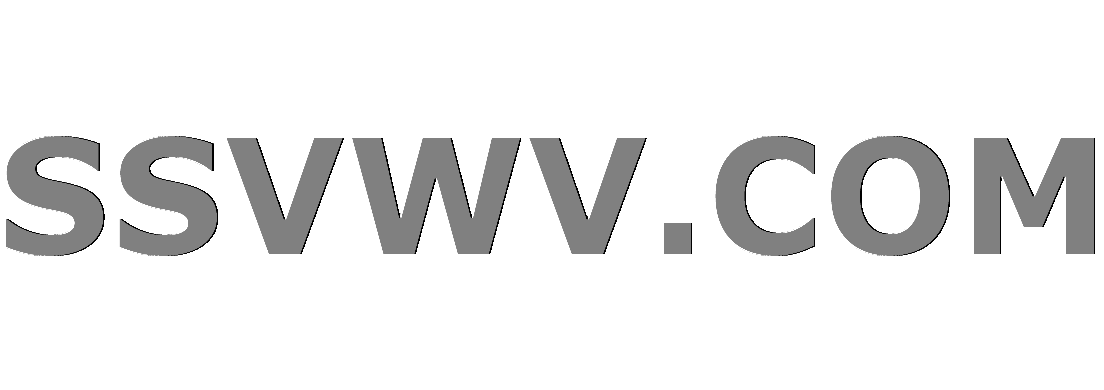
Multi tool use
I'm trying to define a simple function ddf()
that outputs the Hessian matrix of a particular mathematical function, given a 2D vector, x
as the input :
import numpy as np
def ddf(x):
dd11 = 2*x[1]+8
dd12 = 2*x[0]-8*x[1]-8
dd21 = 2*x[0]-8*x[1]-8
dd22 = -8*x[0]+2
return np.array([[dd11, dd12], [dd21, dd22]])
x0 = np.zeros((2,1))
G = ddf(x0)
print(G)
I expect the output to be a 2x2 square array/matrix, however it yields what appears to be a 4x1 column instead.
Stranger still, using
G.shape
yields (2L, 2L, 1L), not (2L,2L) as expected.
My objective is to obtain G in 2x2 form. Can anyone assist? Thanks
python numpy
add a comment |
I'm trying to define a simple function ddf()
that outputs the Hessian matrix of a particular mathematical function, given a 2D vector, x
as the input :
import numpy as np
def ddf(x):
dd11 = 2*x[1]+8
dd12 = 2*x[0]-8*x[1]-8
dd21 = 2*x[0]-8*x[1]-8
dd22 = -8*x[0]+2
return np.array([[dd11, dd12], [dd21, dd22]])
x0 = np.zeros((2,1))
G = ddf(x0)
print(G)
I expect the output to be a 2x2 square array/matrix, however it yields what appears to be a 4x1 column instead.
Stranger still, using
G.shape
yields (2L, 2L, 1L), not (2L,2L) as expected.
My objective is to obtain G in 2x2 form. Can anyone assist? Thanks
python numpy
add a comment |
I'm trying to define a simple function ddf()
that outputs the Hessian matrix of a particular mathematical function, given a 2D vector, x
as the input :
import numpy as np
def ddf(x):
dd11 = 2*x[1]+8
dd12 = 2*x[0]-8*x[1]-8
dd21 = 2*x[0]-8*x[1]-8
dd22 = -8*x[0]+2
return np.array([[dd11, dd12], [dd21, dd22]])
x0 = np.zeros((2,1))
G = ddf(x0)
print(G)
I expect the output to be a 2x2 square array/matrix, however it yields what appears to be a 4x1 column instead.
Stranger still, using
G.shape
yields (2L, 2L, 1L), not (2L,2L) as expected.
My objective is to obtain G in 2x2 form. Can anyone assist? Thanks
python numpy
I'm trying to define a simple function ddf()
that outputs the Hessian matrix of a particular mathematical function, given a 2D vector, x
as the input :
import numpy as np
def ddf(x):
dd11 = 2*x[1]+8
dd12 = 2*x[0]-8*x[1]-8
dd21 = 2*x[0]-8*x[1]-8
dd22 = -8*x[0]+2
return np.array([[dd11, dd12], [dd21, dd22]])
x0 = np.zeros((2,1))
G = ddf(x0)
print(G)
I expect the output to be a 2x2 square array/matrix, however it yields what appears to be a 4x1 column instead.
Stranger still, using
G.shape
yields (2L, 2L, 1L), not (2L,2L) as expected.
My objective is to obtain G in 2x2 form. Can anyone assist? Thanks
python numpy
python numpy
edited Nov 24 '18 at 15:12
Deepak Saini
1,599815
1,599815
asked Nov 24 '18 at 12:50
ruphzruphz
111
111
add a comment |
add a comment |
2 Answers
2
active
oldest
votes
Your input to the function ddf()
is a 2x1 matrix, meaning all of x[0] and x[1] are vectors not scalers(floats or ints). So each element of your output matrix are 1-sized vectors, as all operations in numpy are applied elements wise if arrays are passed to the functions.
Couple of things, you can do :
- It seems that you expect x[0], x[1] to be scalars, so change the input to shape (2,) in
x0 = np.zeros((2,))
. - Or reshape the output as
G.reshape((2,2))
to remove the extra dimension.
add a comment |
I'm very new to python, but I think that will work:
...
G = ddf(x0)
G = np.reshape(G, (2,2))
print(G)
It yields a (2,2) as you wanted.
It does indeed, thank you very much. I also found that reshaping the 2x1 vector (x) as a 1x2 vector does the job
– ruphz
Nov 24 '18 at 13:06
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53458326%2fnumpy-array-function-returns-inconsistent-shapes%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
Your input to the function ddf()
is a 2x1 matrix, meaning all of x[0] and x[1] are vectors not scalers(floats or ints). So each element of your output matrix are 1-sized vectors, as all operations in numpy are applied elements wise if arrays are passed to the functions.
Couple of things, you can do :
- It seems that you expect x[0], x[1] to be scalars, so change the input to shape (2,) in
x0 = np.zeros((2,))
. - Or reshape the output as
G.reshape((2,2))
to remove the extra dimension.
add a comment |
Your input to the function ddf()
is a 2x1 matrix, meaning all of x[0] and x[1] are vectors not scalers(floats or ints). So each element of your output matrix are 1-sized vectors, as all operations in numpy are applied elements wise if arrays are passed to the functions.
Couple of things, you can do :
- It seems that you expect x[0], x[1] to be scalars, so change the input to shape (2,) in
x0 = np.zeros((2,))
. - Or reshape the output as
G.reshape((2,2))
to remove the extra dimension.
add a comment |
Your input to the function ddf()
is a 2x1 matrix, meaning all of x[0] and x[1] are vectors not scalers(floats or ints). So each element of your output matrix are 1-sized vectors, as all operations in numpy are applied elements wise if arrays are passed to the functions.
Couple of things, you can do :
- It seems that you expect x[0], x[1] to be scalars, so change the input to shape (2,) in
x0 = np.zeros((2,))
. - Or reshape the output as
G.reshape((2,2))
to remove the extra dimension.
Your input to the function ddf()
is a 2x1 matrix, meaning all of x[0] and x[1] are vectors not scalers(floats or ints). So each element of your output matrix are 1-sized vectors, as all operations in numpy are applied elements wise if arrays are passed to the functions.
Couple of things, you can do :
- It seems that you expect x[0], x[1] to be scalars, so change the input to shape (2,) in
x0 = np.zeros((2,))
. - Or reshape the output as
G.reshape((2,2))
to remove the extra dimension.
answered Nov 24 '18 at 13:08
Deepak SainiDeepak Saini
1,599815
1,599815
add a comment |
add a comment |
I'm very new to python, but I think that will work:
...
G = ddf(x0)
G = np.reshape(G, (2,2))
print(G)
It yields a (2,2) as you wanted.
It does indeed, thank you very much. I also found that reshaping the 2x1 vector (x) as a 1x2 vector does the job
– ruphz
Nov 24 '18 at 13:06
add a comment |
I'm very new to python, but I think that will work:
...
G = ddf(x0)
G = np.reshape(G, (2,2))
print(G)
It yields a (2,2) as you wanted.
It does indeed, thank you very much. I also found that reshaping the 2x1 vector (x) as a 1x2 vector does the job
– ruphz
Nov 24 '18 at 13:06
add a comment |
I'm very new to python, but I think that will work:
...
G = ddf(x0)
G = np.reshape(G, (2,2))
print(G)
It yields a (2,2) as you wanted.
I'm very new to python, but I think that will work:
...
G = ddf(x0)
G = np.reshape(G, (2,2))
print(G)
It yields a (2,2) as you wanted.
edited Nov 24 '18 at 13:10
answered Nov 24 '18 at 12:59


karsteankarstean
1114
1114
It does indeed, thank you very much. I also found that reshaping the 2x1 vector (x) as a 1x2 vector does the job
– ruphz
Nov 24 '18 at 13:06
add a comment |
It does indeed, thank you very much. I also found that reshaping the 2x1 vector (x) as a 1x2 vector does the job
– ruphz
Nov 24 '18 at 13:06
It does indeed, thank you very much. I also found that reshaping the 2x1 vector (x) as a 1x2 vector does the job
– ruphz
Nov 24 '18 at 13:06
It does indeed, thank you very much. I also found that reshaping the 2x1 vector (x) as a 1x2 vector does the job
– ruphz
Nov 24 '18 at 13:06
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53458326%2fnumpy-array-function-returns-inconsistent-shapes%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
LZHqV4AUj8zp0e0,rnslBlkV,mlUowRKvvYJh6aK1p4jmLVk,F