3D Computer Graphic Animation : Back-Face Culling not working properly
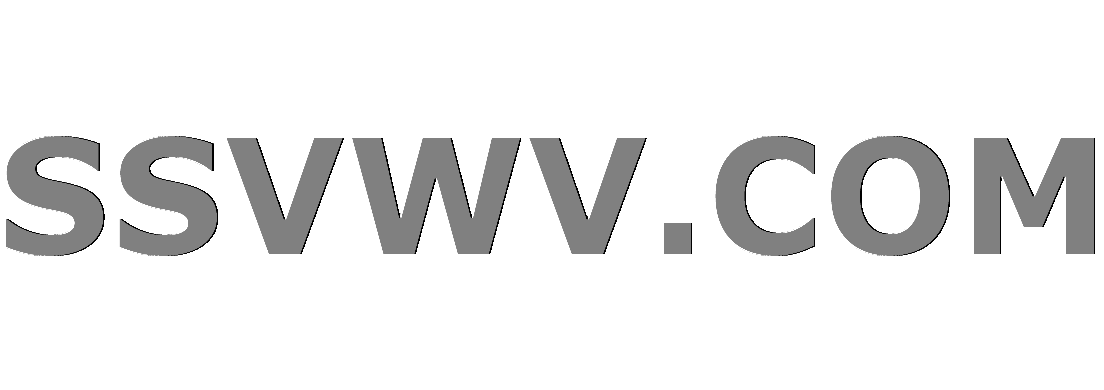
Multi tool use
So I've tried to do a backface culling on the cube with Visual Basic. I'm sure that the code that i wrote is right, but somehow the it's not the back face that i want.
This is the data structure
Structure TLine
Dim p1, p2 As Integer
End Structure
Structure TLine2
Dim p1, p2 As TPoint
End Structure
Structure TPoint
Dim x, y, z, w As Double
End Structure
Structure TPolygon
Dim p1, p2, p3 As Integer
End Structure
This is the function
Sub SetPoint(ByRef V As TPoint, ByVal a As Double, ByVal b As Double, ByVal c As Double, ByVal d As Double)
V.x = a
V.y = b
V.z = c
V.w = 1
End Sub
Sub SetLine(ByVal idx As Integer, ByVal p1 As Integer, ByVal p2 As Integer)
edge(idx).p1 = p1
edge(idx).p2 = p2
End Sub
Sub SetPolygon(ByVal idx As Integer, ByVal p1 As Integer, ByVal p2 As Integer, ByVal p3 As Integer)
polygon(idx).p1 = p1
polygon(idx).p2 = p2
polygon(idx).p3 = p3
End Sub
This is the draw function
Sub draw()
bmp = New Bitmap(400, 400)
Dim grp As Graphics = Graphics.FromImage(bmp)
Dim i As Integer
Dim p As New Pen(Color.Black)
DOP.x = 0
DOP.y = 0
DOP.z = -2
For i = 0 To 11
temp_v1.x = v(polygon(i).p2).x - v(polygon(i).p1).x
temp_v1.y = v(polygon(i).p2).y - v(polygon(i).p1).y
temp_v1.z = v(polygon(i).p2).z - v(polygon(i).p1).z
temp_v2.x = v(polygon(i).p3).x - v(polygon(i).p1).x
temp_v2.y = v(polygon(i).p3).y - v(polygon(i).p1).y
temp_v2.z = v(polygon(i).p3).z - v(polygon(i).p1).z
normal.x = (temp_v1.y * temp_v2.z) - (temp_v2.y * temp_v1.z)
normal.y = (temp_v1.z * temp_v2.x) - (temp_v2.z * temp_v1.x)
normal.z = (temp_v1.x * temp_v2.y) - (temp_v2.x * temp_v1.y)
result = (DOP.x * normal.x) + (DOP.y * normal.y) + (DOP.z * normal.z)
If result < 0 Then
grp.DrawLine(p, CInt(vs(polygon(i).p1).x), CInt(vs(polygon(i).p1).y), CInt(vs(polygon(i).p2).x), CInt(vs(polygon(i).p2).y))
grp.DrawLine(p, CInt(vs(polygon(i).p1).x), CInt(vs(polygon(i).p1).y), CInt(vs(polygon(i).p3).x), CInt(vs(polygon(i).p3).y))
grp.DrawLine(p, CInt(vs(polygon(i).p2).x), CInt(vs(polygon(i).p2).y), CInt(vs(polygon(i).p3).x), CInt(vs(polygon(i).p3).y))
PictureBox1.Image = bmp
End If
Next
'PictureBox1.Image = bmp
End Sub
And this is the set of point and polygon in one cube
SetPoint(v(0), -1, -1, 1, 1)
SetPoint(v(1), 1, -1, 1, 1)
SetPoint(v(2), 1, 1, 1, 1)
SetPoint(v(3), -1, 1, 1, 1)
SetPoint(v(4), -1, -1, -1, 1)
SetPoint(v(5), 1, -1, -1, 1)
SetPoint(v(6), 1, 1, -1, 1)
SetPoint(v(7), -1, 1, -1, 1)
SetPolygon(0, 0, 1, 2)
SetPolygon(1, 0, 3, 2)
SetPolygon(2, 5, 4, 7)
SetPolygon(3, 5, 6, 7)
SetPolygon(4, 3, 2, 6)
SetPolygon(5, 3, 7, 6)
SetPolygon(6, 4, 5, 1)
SetPolygon(7, 4, 0, 1)
SetPolygon(8, 1, 5, 6)
SetPolygon(9, 1, 2, 6)
SetPolygon(10, 4, 0, 3)
SetPolygon(11, 4, 7, 3)
This is the preview of the cube that i tried to back face culling with a rotation.
3d culling
add a comment |
So I've tried to do a backface culling on the cube with Visual Basic. I'm sure that the code that i wrote is right, but somehow the it's not the back face that i want.
This is the data structure
Structure TLine
Dim p1, p2 As Integer
End Structure
Structure TLine2
Dim p1, p2 As TPoint
End Structure
Structure TPoint
Dim x, y, z, w As Double
End Structure
Structure TPolygon
Dim p1, p2, p3 As Integer
End Structure
This is the function
Sub SetPoint(ByRef V As TPoint, ByVal a As Double, ByVal b As Double, ByVal c As Double, ByVal d As Double)
V.x = a
V.y = b
V.z = c
V.w = 1
End Sub
Sub SetLine(ByVal idx As Integer, ByVal p1 As Integer, ByVal p2 As Integer)
edge(idx).p1 = p1
edge(idx).p2 = p2
End Sub
Sub SetPolygon(ByVal idx As Integer, ByVal p1 As Integer, ByVal p2 As Integer, ByVal p3 As Integer)
polygon(idx).p1 = p1
polygon(idx).p2 = p2
polygon(idx).p3 = p3
End Sub
This is the draw function
Sub draw()
bmp = New Bitmap(400, 400)
Dim grp As Graphics = Graphics.FromImage(bmp)
Dim i As Integer
Dim p As New Pen(Color.Black)
DOP.x = 0
DOP.y = 0
DOP.z = -2
For i = 0 To 11
temp_v1.x = v(polygon(i).p2).x - v(polygon(i).p1).x
temp_v1.y = v(polygon(i).p2).y - v(polygon(i).p1).y
temp_v1.z = v(polygon(i).p2).z - v(polygon(i).p1).z
temp_v2.x = v(polygon(i).p3).x - v(polygon(i).p1).x
temp_v2.y = v(polygon(i).p3).y - v(polygon(i).p1).y
temp_v2.z = v(polygon(i).p3).z - v(polygon(i).p1).z
normal.x = (temp_v1.y * temp_v2.z) - (temp_v2.y * temp_v1.z)
normal.y = (temp_v1.z * temp_v2.x) - (temp_v2.z * temp_v1.x)
normal.z = (temp_v1.x * temp_v2.y) - (temp_v2.x * temp_v1.y)
result = (DOP.x * normal.x) + (DOP.y * normal.y) + (DOP.z * normal.z)
If result < 0 Then
grp.DrawLine(p, CInt(vs(polygon(i).p1).x), CInt(vs(polygon(i).p1).y), CInt(vs(polygon(i).p2).x), CInt(vs(polygon(i).p2).y))
grp.DrawLine(p, CInt(vs(polygon(i).p1).x), CInt(vs(polygon(i).p1).y), CInt(vs(polygon(i).p3).x), CInt(vs(polygon(i).p3).y))
grp.DrawLine(p, CInt(vs(polygon(i).p2).x), CInt(vs(polygon(i).p2).y), CInt(vs(polygon(i).p3).x), CInt(vs(polygon(i).p3).y))
PictureBox1.Image = bmp
End If
Next
'PictureBox1.Image = bmp
End Sub
And this is the set of point and polygon in one cube
SetPoint(v(0), -1, -1, 1, 1)
SetPoint(v(1), 1, -1, 1, 1)
SetPoint(v(2), 1, 1, 1, 1)
SetPoint(v(3), -1, 1, 1, 1)
SetPoint(v(4), -1, -1, -1, 1)
SetPoint(v(5), 1, -1, -1, 1)
SetPoint(v(6), 1, 1, -1, 1)
SetPoint(v(7), -1, 1, -1, 1)
SetPolygon(0, 0, 1, 2)
SetPolygon(1, 0, 3, 2)
SetPolygon(2, 5, 4, 7)
SetPolygon(3, 5, 6, 7)
SetPolygon(4, 3, 2, 6)
SetPolygon(5, 3, 7, 6)
SetPolygon(6, 4, 5, 1)
SetPolygon(7, 4, 0, 1)
SetPolygon(8, 1, 5, 6)
SetPolygon(9, 1, 2, 6)
SetPolygon(10, 4, 0, 3)
SetPolygon(11, 4, 7, 3)
This is the preview of the cube that i tried to back face culling with a rotation.
3d culling
add a comment |
So I've tried to do a backface culling on the cube with Visual Basic. I'm sure that the code that i wrote is right, but somehow the it's not the back face that i want.
This is the data structure
Structure TLine
Dim p1, p2 As Integer
End Structure
Structure TLine2
Dim p1, p2 As TPoint
End Structure
Structure TPoint
Dim x, y, z, w As Double
End Structure
Structure TPolygon
Dim p1, p2, p3 As Integer
End Structure
This is the function
Sub SetPoint(ByRef V As TPoint, ByVal a As Double, ByVal b As Double, ByVal c As Double, ByVal d As Double)
V.x = a
V.y = b
V.z = c
V.w = 1
End Sub
Sub SetLine(ByVal idx As Integer, ByVal p1 As Integer, ByVal p2 As Integer)
edge(idx).p1 = p1
edge(idx).p2 = p2
End Sub
Sub SetPolygon(ByVal idx As Integer, ByVal p1 As Integer, ByVal p2 As Integer, ByVal p3 As Integer)
polygon(idx).p1 = p1
polygon(idx).p2 = p2
polygon(idx).p3 = p3
End Sub
This is the draw function
Sub draw()
bmp = New Bitmap(400, 400)
Dim grp As Graphics = Graphics.FromImage(bmp)
Dim i As Integer
Dim p As New Pen(Color.Black)
DOP.x = 0
DOP.y = 0
DOP.z = -2
For i = 0 To 11
temp_v1.x = v(polygon(i).p2).x - v(polygon(i).p1).x
temp_v1.y = v(polygon(i).p2).y - v(polygon(i).p1).y
temp_v1.z = v(polygon(i).p2).z - v(polygon(i).p1).z
temp_v2.x = v(polygon(i).p3).x - v(polygon(i).p1).x
temp_v2.y = v(polygon(i).p3).y - v(polygon(i).p1).y
temp_v2.z = v(polygon(i).p3).z - v(polygon(i).p1).z
normal.x = (temp_v1.y * temp_v2.z) - (temp_v2.y * temp_v1.z)
normal.y = (temp_v1.z * temp_v2.x) - (temp_v2.z * temp_v1.x)
normal.z = (temp_v1.x * temp_v2.y) - (temp_v2.x * temp_v1.y)
result = (DOP.x * normal.x) + (DOP.y * normal.y) + (DOP.z * normal.z)
If result < 0 Then
grp.DrawLine(p, CInt(vs(polygon(i).p1).x), CInt(vs(polygon(i).p1).y), CInt(vs(polygon(i).p2).x), CInt(vs(polygon(i).p2).y))
grp.DrawLine(p, CInt(vs(polygon(i).p1).x), CInt(vs(polygon(i).p1).y), CInt(vs(polygon(i).p3).x), CInt(vs(polygon(i).p3).y))
grp.DrawLine(p, CInt(vs(polygon(i).p2).x), CInt(vs(polygon(i).p2).y), CInt(vs(polygon(i).p3).x), CInt(vs(polygon(i).p3).y))
PictureBox1.Image = bmp
End If
Next
'PictureBox1.Image = bmp
End Sub
And this is the set of point and polygon in one cube
SetPoint(v(0), -1, -1, 1, 1)
SetPoint(v(1), 1, -1, 1, 1)
SetPoint(v(2), 1, 1, 1, 1)
SetPoint(v(3), -1, 1, 1, 1)
SetPoint(v(4), -1, -1, -1, 1)
SetPoint(v(5), 1, -1, -1, 1)
SetPoint(v(6), 1, 1, -1, 1)
SetPoint(v(7), -1, 1, -1, 1)
SetPolygon(0, 0, 1, 2)
SetPolygon(1, 0, 3, 2)
SetPolygon(2, 5, 4, 7)
SetPolygon(3, 5, 6, 7)
SetPolygon(4, 3, 2, 6)
SetPolygon(5, 3, 7, 6)
SetPolygon(6, 4, 5, 1)
SetPolygon(7, 4, 0, 1)
SetPolygon(8, 1, 5, 6)
SetPolygon(9, 1, 2, 6)
SetPolygon(10, 4, 0, 3)
SetPolygon(11, 4, 7, 3)
This is the preview of the cube that i tried to back face culling with a rotation.
3d culling
So I've tried to do a backface culling on the cube with Visual Basic. I'm sure that the code that i wrote is right, but somehow the it's not the back face that i want.
This is the data structure
Structure TLine
Dim p1, p2 As Integer
End Structure
Structure TLine2
Dim p1, p2 As TPoint
End Structure
Structure TPoint
Dim x, y, z, w As Double
End Structure
Structure TPolygon
Dim p1, p2, p3 As Integer
End Structure
This is the function
Sub SetPoint(ByRef V As TPoint, ByVal a As Double, ByVal b As Double, ByVal c As Double, ByVal d As Double)
V.x = a
V.y = b
V.z = c
V.w = 1
End Sub
Sub SetLine(ByVal idx As Integer, ByVal p1 As Integer, ByVal p2 As Integer)
edge(idx).p1 = p1
edge(idx).p2 = p2
End Sub
Sub SetPolygon(ByVal idx As Integer, ByVal p1 As Integer, ByVal p2 As Integer, ByVal p3 As Integer)
polygon(idx).p1 = p1
polygon(idx).p2 = p2
polygon(idx).p3 = p3
End Sub
This is the draw function
Sub draw()
bmp = New Bitmap(400, 400)
Dim grp As Graphics = Graphics.FromImage(bmp)
Dim i As Integer
Dim p As New Pen(Color.Black)
DOP.x = 0
DOP.y = 0
DOP.z = -2
For i = 0 To 11
temp_v1.x = v(polygon(i).p2).x - v(polygon(i).p1).x
temp_v1.y = v(polygon(i).p2).y - v(polygon(i).p1).y
temp_v1.z = v(polygon(i).p2).z - v(polygon(i).p1).z
temp_v2.x = v(polygon(i).p3).x - v(polygon(i).p1).x
temp_v2.y = v(polygon(i).p3).y - v(polygon(i).p1).y
temp_v2.z = v(polygon(i).p3).z - v(polygon(i).p1).z
normal.x = (temp_v1.y * temp_v2.z) - (temp_v2.y * temp_v1.z)
normal.y = (temp_v1.z * temp_v2.x) - (temp_v2.z * temp_v1.x)
normal.z = (temp_v1.x * temp_v2.y) - (temp_v2.x * temp_v1.y)
result = (DOP.x * normal.x) + (DOP.y * normal.y) + (DOP.z * normal.z)
If result < 0 Then
grp.DrawLine(p, CInt(vs(polygon(i).p1).x), CInt(vs(polygon(i).p1).y), CInt(vs(polygon(i).p2).x), CInt(vs(polygon(i).p2).y))
grp.DrawLine(p, CInt(vs(polygon(i).p1).x), CInt(vs(polygon(i).p1).y), CInt(vs(polygon(i).p3).x), CInt(vs(polygon(i).p3).y))
grp.DrawLine(p, CInt(vs(polygon(i).p2).x), CInt(vs(polygon(i).p2).y), CInt(vs(polygon(i).p3).x), CInt(vs(polygon(i).p3).y))
PictureBox1.Image = bmp
End If
Next
'PictureBox1.Image = bmp
End Sub
And this is the set of point and polygon in one cube
SetPoint(v(0), -1, -1, 1, 1)
SetPoint(v(1), 1, -1, 1, 1)
SetPoint(v(2), 1, 1, 1, 1)
SetPoint(v(3), -1, 1, 1, 1)
SetPoint(v(4), -1, -1, -1, 1)
SetPoint(v(5), 1, -1, -1, 1)
SetPoint(v(6), 1, 1, -1, 1)
SetPoint(v(7), -1, 1, -1, 1)
SetPolygon(0, 0, 1, 2)
SetPolygon(1, 0, 3, 2)
SetPolygon(2, 5, 4, 7)
SetPolygon(3, 5, 6, 7)
SetPolygon(4, 3, 2, 6)
SetPolygon(5, 3, 7, 6)
SetPolygon(6, 4, 5, 1)
SetPolygon(7, 4, 0, 1)
SetPolygon(8, 1, 5, 6)
SetPolygon(9, 1, 2, 6)
SetPolygon(10, 4, 0, 3)
SetPolygon(11, 4, 7, 3)
This is the preview of the cube that i tried to back face culling with a rotation.
3d culling
3d culling
edited Nov 27 '18 at 10:35


Kromster
4,76564987
4,76564987
asked Nov 26 '18 at 3:34


KanzouGKanzouG
1
1
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
Back-Face culling depends on the order of your vertices on the screen. You assume that either clockwise or counterclockwise are "front" and you draw only those.
In your preview I see that you lack some of the front faces and you draw some back faces that you shouldn't. You just need to find those few broken triangles and revert their direction by swapping 2nd and 3rd vertex.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53474469%2f3d-computer-graphic-animation-back-face-culling-not-working-properly%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
Back-Face culling depends on the order of your vertices on the screen. You assume that either clockwise or counterclockwise are "front" and you draw only those.
In your preview I see that you lack some of the front faces and you draw some back faces that you shouldn't. You just need to find those few broken triangles and revert their direction by swapping 2nd and 3rd vertex.
add a comment |
Back-Face culling depends on the order of your vertices on the screen. You assume that either clockwise or counterclockwise are "front" and you draw only those.
In your preview I see that you lack some of the front faces and you draw some back faces that you shouldn't. You just need to find those few broken triangles and revert their direction by swapping 2nd and 3rd vertex.
add a comment |
Back-Face culling depends on the order of your vertices on the screen. You assume that either clockwise or counterclockwise are "front" and you draw only those.
In your preview I see that you lack some of the front faces and you draw some back faces that you shouldn't. You just need to find those few broken triangles and revert their direction by swapping 2nd and 3rd vertex.
Back-Face culling depends on the order of your vertices on the screen. You assume that either clockwise or counterclockwise are "front" and you draw only those.
In your preview I see that you lack some of the front faces and you draw some back faces that you shouldn't. You just need to find those few broken triangles and revert their direction by swapping 2nd and 3rd vertex.
answered Nov 26 '18 at 13:52
kolendakolenda
2,30121223
2,30121223
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53474469%2f3d-computer-graphic-animation-back-face-culling-not-working-properly%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
B6 qDaTeOsSvTt0