C++ for loop nested statements to python
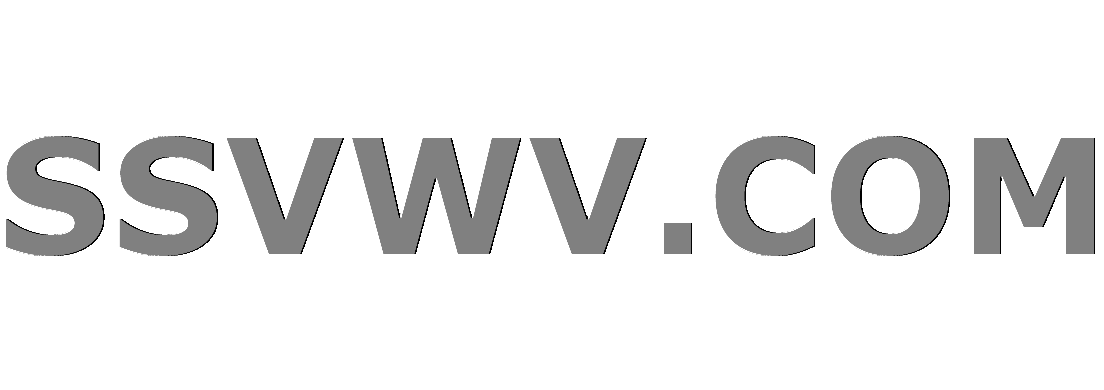
Multi tool use
up vote
2
down vote
favorite
How would I translate the following C++ for loop into a python for loop?
for(i = xSize - 1; i >= 0 && ptr.points[i] > temp; i--){
doSomething;
}
I am having trouble because of the and operator. Without it, I know it would be like
for i in range(xSize - 1, 0, -1)
Is this equivalent?
for i in range(xSize - 1, 0, -1):
if ptr.points[i] > temp:
doSomething
python c++ for-loop
add a comment |
up vote
2
down vote
favorite
How would I translate the following C++ for loop into a python for loop?
for(i = xSize - 1; i >= 0 && ptr.points[i] > temp; i--){
doSomething;
}
I am having trouble because of the and operator. Without it, I know it would be like
for i in range(xSize - 1, 0, -1)
Is this equivalent?
for i in range(xSize - 1, 0, -1):
if ptr.points[i] > temp:
doSomething
python c++ for-loop
Just break out of the loop once the condition becomes false:for i in range(xSize-1, 0, -1): if i < 0 or ptr.points[i] <= temp: break else: doSomething
– pault
Nov 19 at 20:38
thanks, but why use the negation? Does it has advantages?
– paul
Nov 19 at 20:49
They should be equivalent. See the answer I posted (without the negation) for more explanation.
– pault
Nov 19 at 20:53
add a comment |
up vote
2
down vote
favorite
up vote
2
down vote
favorite
How would I translate the following C++ for loop into a python for loop?
for(i = xSize - 1; i >= 0 && ptr.points[i] > temp; i--){
doSomething;
}
I am having trouble because of the and operator. Without it, I know it would be like
for i in range(xSize - 1, 0, -1)
Is this equivalent?
for i in range(xSize - 1, 0, -1):
if ptr.points[i] > temp:
doSomething
python c++ for-loop
How would I translate the following C++ for loop into a python for loop?
for(i = xSize - 1; i >= 0 && ptr.points[i] > temp; i--){
doSomething;
}
I am having trouble because of the and operator. Without it, I know it would be like
for i in range(xSize - 1, 0, -1)
Is this equivalent?
for i in range(xSize - 1, 0, -1):
if ptr.points[i] > temp:
doSomething
python c++ for-loop
python c++ for-loop
asked Nov 19 at 20:35
paul
204
204
Just break out of the loop once the condition becomes false:for i in range(xSize-1, 0, -1): if i < 0 or ptr.points[i] <= temp: break else: doSomething
– pault
Nov 19 at 20:38
thanks, but why use the negation? Does it has advantages?
– paul
Nov 19 at 20:49
They should be equivalent. See the answer I posted (without the negation) for more explanation.
– pault
Nov 19 at 20:53
add a comment |
Just break out of the loop once the condition becomes false:for i in range(xSize-1, 0, -1): if i < 0 or ptr.points[i] <= temp: break else: doSomething
– pault
Nov 19 at 20:38
thanks, but why use the negation? Does it has advantages?
– paul
Nov 19 at 20:49
They should be equivalent. See the answer I posted (without the negation) for more explanation.
– pault
Nov 19 at 20:53
Just break out of the loop once the condition becomes false:
for i in range(xSize-1, 0, -1): if i < 0 or ptr.points[i] <= temp: break else: doSomething
– pault
Nov 19 at 20:38
Just break out of the loop once the condition becomes false:
for i in range(xSize-1, 0, -1): if i < 0 or ptr.points[i] <= temp: break else: doSomething
– pault
Nov 19 at 20:38
thanks, but why use the negation? Does it has advantages?
– paul
Nov 19 at 20:49
thanks, but why use the negation? Does it has advantages?
– paul
Nov 19 at 20:49
They should be equivalent. See the answer I posted (without the negation) for more explanation.
– pault
Nov 19 at 20:53
They should be equivalent. See the answer I posted (without the negation) for more explanation.
– pault
Nov 19 at 20:53
add a comment |
3 Answers
3
active
oldest
votes
up vote
0
down vote
Python itself has no support for this kind of operation.
However, your intuition is right. You can write as you stated, but as @François Andrieux correctly stated, you should also provide a break
clause.
Also, you should take a look at itertools
module provided by standard library. It offers a wide rage of functions to deal with efficient looping.
from itertools import takewhile, count
for i in takewhile(lambda i:i > 0 and i<xSize -1 and ptr.Points[i] > temp, i = i-1): doSth()
1
I'm not an expert in Python, but it looks to me like the python version of the loop will iterate over all of the range and simply skip those that agree with the predicate. The c++ loop ends when the first such value is found, so even if future values don't match it, they will also be "skipped".
– François Andrieux
Nov 19 at 20:42
Thanks for the reply.
– paul
Nov 19 at 20:51
Yes, that's correct. Thank you for the completation, I also completed the answer including your observation
– bogdan tudose
Nov 19 at 20:57
add a comment |
up vote
0
down vote
The flow of a C++ for loop is roughly:
for ( initialize; condition; increment/decrement ) {
doSomething;
}
Once the condition evaluates to false
, execution will break out of the loop. In your example, the condition is i >= 0 && ptr.points[i] > temp
which means that you will break out of the loop if the value of i
goes negative or ptr.points[i] <= temp
.
Thus you can equivalently write:
for(int i = xSize - 1; i >= 0; i--){
if(ptr.points[i] > temp) {
doSomething;
}
else {
break;
}
}
Which, translates quite easily to python
as:
for i in range(xSize-1, 0, -1):
if i >= 0 and ptr.points[i] > temp:
doSomething
else:
break
(Though you don't really need the i >= 0
check)
Thanks for the reply. In the python if statement you used or, I believe that is a typo right?
– paul
Nov 19 at 21:00
@paul indeed it was a typo. fixed it. Also note that this is different than what you posted in your question, because your version will not break out of the loop.
– pault
Nov 19 at 21:04
Yes. I understand that part.
– paul
Nov 19 at 21:22
add a comment |
up vote
0
down vote
I think this should be okay as a while loop and intuitive, too, IMHO
i=xSize-1
while True:
if ptr.points[i] > temp:
doSomething
else:
break
i-=1
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53382249%2fc-for-loop-nested-statements-to-python%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
Python itself has no support for this kind of operation.
However, your intuition is right. You can write as you stated, but as @François Andrieux correctly stated, you should also provide a break
clause.
Also, you should take a look at itertools
module provided by standard library. It offers a wide rage of functions to deal with efficient looping.
from itertools import takewhile, count
for i in takewhile(lambda i:i > 0 and i<xSize -1 and ptr.Points[i] > temp, i = i-1): doSth()
1
I'm not an expert in Python, but it looks to me like the python version of the loop will iterate over all of the range and simply skip those that agree with the predicate. The c++ loop ends when the first such value is found, so even if future values don't match it, they will also be "skipped".
– François Andrieux
Nov 19 at 20:42
Thanks for the reply.
– paul
Nov 19 at 20:51
Yes, that's correct. Thank you for the completation, I also completed the answer including your observation
– bogdan tudose
Nov 19 at 20:57
add a comment |
up vote
0
down vote
Python itself has no support for this kind of operation.
However, your intuition is right. You can write as you stated, but as @François Andrieux correctly stated, you should also provide a break
clause.
Also, you should take a look at itertools
module provided by standard library. It offers a wide rage of functions to deal with efficient looping.
from itertools import takewhile, count
for i in takewhile(lambda i:i > 0 and i<xSize -1 and ptr.Points[i] > temp, i = i-1): doSth()
1
I'm not an expert in Python, but it looks to me like the python version of the loop will iterate over all of the range and simply skip those that agree with the predicate. The c++ loop ends when the first such value is found, so even if future values don't match it, they will also be "skipped".
– François Andrieux
Nov 19 at 20:42
Thanks for the reply.
– paul
Nov 19 at 20:51
Yes, that's correct. Thank you for the completation, I also completed the answer including your observation
– bogdan tudose
Nov 19 at 20:57
add a comment |
up vote
0
down vote
up vote
0
down vote
Python itself has no support for this kind of operation.
However, your intuition is right. You can write as you stated, but as @François Andrieux correctly stated, you should also provide a break
clause.
Also, you should take a look at itertools
module provided by standard library. It offers a wide rage of functions to deal with efficient looping.
from itertools import takewhile, count
for i in takewhile(lambda i:i > 0 and i<xSize -1 and ptr.Points[i] > temp, i = i-1): doSth()
Python itself has no support for this kind of operation.
However, your intuition is right. You can write as you stated, but as @François Andrieux correctly stated, you should also provide a break
clause.
Also, you should take a look at itertools
module provided by standard library. It offers a wide rage of functions to deal with efficient looping.
from itertools import takewhile, count
for i in takewhile(lambda i:i > 0 and i<xSize -1 and ptr.Points[i] > temp, i = i-1): doSth()
edited Nov 19 at 20:58
answered Nov 19 at 20:38
bogdan tudose
867
867
1
I'm not an expert in Python, but it looks to me like the python version of the loop will iterate over all of the range and simply skip those that agree with the predicate. The c++ loop ends when the first such value is found, so even if future values don't match it, they will also be "skipped".
– François Andrieux
Nov 19 at 20:42
Thanks for the reply.
– paul
Nov 19 at 20:51
Yes, that's correct. Thank you for the completation, I also completed the answer including your observation
– bogdan tudose
Nov 19 at 20:57
add a comment |
1
I'm not an expert in Python, but it looks to me like the python version of the loop will iterate over all of the range and simply skip those that agree with the predicate. The c++ loop ends when the first such value is found, so even if future values don't match it, they will also be "skipped".
– François Andrieux
Nov 19 at 20:42
Thanks for the reply.
– paul
Nov 19 at 20:51
Yes, that's correct. Thank you for the completation, I also completed the answer including your observation
– bogdan tudose
Nov 19 at 20:57
1
1
I'm not an expert in Python, but it looks to me like the python version of the loop will iterate over all of the range and simply skip those that agree with the predicate. The c++ loop ends when the first such value is found, so even if future values don't match it, they will also be "skipped".
– François Andrieux
Nov 19 at 20:42
I'm not an expert in Python, but it looks to me like the python version of the loop will iterate over all of the range and simply skip those that agree with the predicate. The c++ loop ends when the first such value is found, so even if future values don't match it, they will also be "skipped".
– François Andrieux
Nov 19 at 20:42
Thanks for the reply.
– paul
Nov 19 at 20:51
Thanks for the reply.
– paul
Nov 19 at 20:51
Yes, that's correct. Thank you for the completation, I also completed the answer including your observation
– bogdan tudose
Nov 19 at 20:57
Yes, that's correct. Thank you for the completation, I also completed the answer including your observation
– bogdan tudose
Nov 19 at 20:57
add a comment |
up vote
0
down vote
The flow of a C++ for loop is roughly:
for ( initialize; condition; increment/decrement ) {
doSomething;
}
Once the condition evaluates to false
, execution will break out of the loop. In your example, the condition is i >= 0 && ptr.points[i] > temp
which means that you will break out of the loop if the value of i
goes negative or ptr.points[i] <= temp
.
Thus you can equivalently write:
for(int i = xSize - 1; i >= 0; i--){
if(ptr.points[i] > temp) {
doSomething;
}
else {
break;
}
}
Which, translates quite easily to python
as:
for i in range(xSize-1, 0, -1):
if i >= 0 and ptr.points[i] > temp:
doSomething
else:
break
(Though you don't really need the i >= 0
check)
Thanks for the reply. In the python if statement you used or, I believe that is a typo right?
– paul
Nov 19 at 21:00
@paul indeed it was a typo. fixed it. Also note that this is different than what you posted in your question, because your version will not break out of the loop.
– pault
Nov 19 at 21:04
Yes. I understand that part.
– paul
Nov 19 at 21:22
add a comment |
up vote
0
down vote
The flow of a C++ for loop is roughly:
for ( initialize; condition; increment/decrement ) {
doSomething;
}
Once the condition evaluates to false
, execution will break out of the loop. In your example, the condition is i >= 0 && ptr.points[i] > temp
which means that you will break out of the loop if the value of i
goes negative or ptr.points[i] <= temp
.
Thus you can equivalently write:
for(int i = xSize - 1; i >= 0; i--){
if(ptr.points[i] > temp) {
doSomething;
}
else {
break;
}
}
Which, translates quite easily to python
as:
for i in range(xSize-1, 0, -1):
if i >= 0 and ptr.points[i] > temp:
doSomething
else:
break
(Though you don't really need the i >= 0
check)
Thanks for the reply. In the python if statement you used or, I believe that is a typo right?
– paul
Nov 19 at 21:00
@paul indeed it was a typo. fixed it. Also note that this is different than what you posted in your question, because your version will not break out of the loop.
– pault
Nov 19 at 21:04
Yes. I understand that part.
– paul
Nov 19 at 21:22
add a comment |
up vote
0
down vote
up vote
0
down vote
The flow of a C++ for loop is roughly:
for ( initialize; condition; increment/decrement ) {
doSomething;
}
Once the condition evaluates to false
, execution will break out of the loop. In your example, the condition is i >= 0 && ptr.points[i] > temp
which means that you will break out of the loop if the value of i
goes negative or ptr.points[i] <= temp
.
Thus you can equivalently write:
for(int i = xSize - 1; i >= 0; i--){
if(ptr.points[i] > temp) {
doSomething;
}
else {
break;
}
}
Which, translates quite easily to python
as:
for i in range(xSize-1, 0, -1):
if i >= 0 and ptr.points[i] > temp:
doSomething
else:
break
(Though you don't really need the i >= 0
check)
The flow of a C++ for loop is roughly:
for ( initialize; condition; increment/decrement ) {
doSomething;
}
Once the condition evaluates to false
, execution will break out of the loop. In your example, the condition is i >= 0 && ptr.points[i] > temp
which means that you will break out of the loop if the value of i
goes negative or ptr.points[i] <= temp
.
Thus you can equivalently write:
for(int i = xSize - 1; i >= 0; i--){
if(ptr.points[i] > temp) {
doSomething;
}
else {
break;
}
}
Which, translates quite easily to python
as:
for i in range(xSize-1, 0, -1):
if i >= 0 and ptr.points[i] > temp:
doSomething
else:
break
(Though you don't really need the i >= 0
check)
edited Nov 19 at 21:04
answered Nov 19 at 20:52


pault
13.7k31744
13.7k31744
Thanks for the reply. In the python if statement you used or, I believe that is a typo right?
– paul
Nov 19 at 21:00
@paul indeed it was a typo. fixed it. Also note that this is different than what you posted in your question, because your version will not break out of the loop.
– pault
Nov 19 at 21:04
Yes. I understand that part.
– paul
Nov 19 at 21:22
add a comment |
Thanks for the reply. In the python if statement you used or, I believe that is a typo right?
– paul
Nov 19 at 21:00
@paul indeed it was a typo. fixed it. Also note that this is different than what you posted in your question, because your version will not break out of the loop.
– pault
Nov 19 at 21:04
Yes. I understand that part.
– paul
Nov 19 at 21:22
Thanks for the reply. In the python if statement you used or, I believe that is a typo right?
– paul
Nov 19 at 21:00
Thanks for the reply. In the python if statement you used or, I believe that is a typo right?
– paul
Nov 19 at 21:00
@paul indeed it was a typo. fixed it. Also note that this is different than what you posted in your question, because your version will not break out of the loop.
– pault
Nov 19 at 21:04
@paul indeed it was a typo. fixed it. Also note that this is different than what you posted in your question, because your version will not break out of the loop.
– pault
Nov 19 at 21:04
Yes. I understand that part.
– paul
Nov 19 at 21:22
Yes. I understand that part.
– paul
Nov 19 at 21:22
add a comment |
up vote
0
down vote
I think this should be okay as a while loop and intuitive, too, IMHO
i=xSize-1
while True:
if ptr.points[i] > temp:
doSomething
else:
break
i-=1
add a comment |
up vote
0
down vote
I think this should be okay as a while loop and intuitive, too, IMHO
i=xSize-1
while True:
if ptr.points[i] > temp:
doSomething
else:
break
i-=1
add a comment |
up vote
0
down vote
up vote
0
down vote
I think this should be okay as a while loop and intuitive, too, IMHO
i=xSize-1
while True:
if ptr.points[i] > temp:
doSomething
else:
break
i-=1
I think this should be okay as a while loop and intuitive, too, IMHO
i=xSize-1
while True:
if ptr.points[i] > temp:
doSomething
else:
break
i-=1
answered Nov 19 at 21:08


Matt Cremeens
3,19632049
3,19632049
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53382249%2fc-for-loop-nested-statements-to-python%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
sI,VaGI 4gOityECrwCTy43GMI84yckT,r0ZWuvZinIXjA
Just break out of the loop once the condition becomes false:
for i in range(xSize-1, 0, -1): if i < 0 or ptr.points[i] <= temp: break else: doSomething
– pault
Nov 19 at 20:38
thanks, but why use the negation? Does it has advantages?
– paul
Nov 19 at 20:49
They should be equivalent. See the answer I posted (without the negation) for more explanation.
– pault
Nov 19 at 20:53