Java - Is a Connection/Stream already closed if an Exception occurs and how to handle it properly?
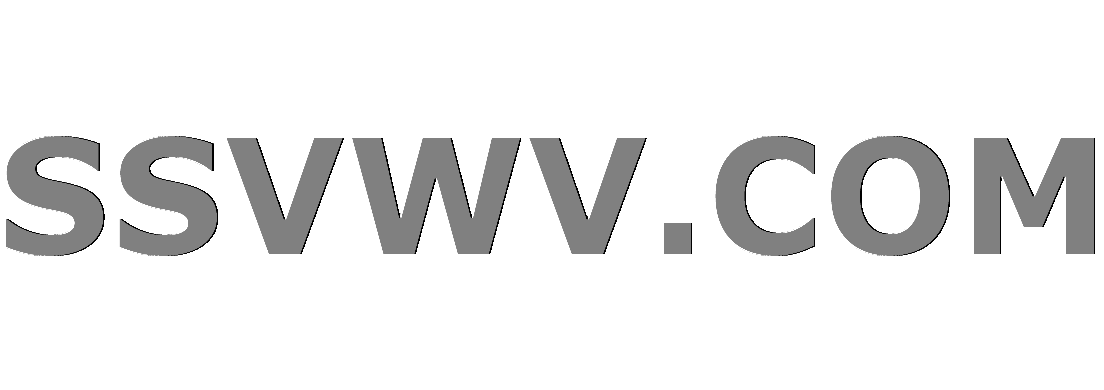
Multi tool use
up vote
1
down vote
favorite
I am wondering, if I have some sort of Connection or Stream in my code that should be closed to release ressources, what does it mean for the connection instance itself?
Code example:
CustomConnection connection;
try{
connection = //some code that opens a connection
//some code using the connection
}catch(IOException){
//Some logging and Handling of IOExceptions
}finally{
//resources cleanup
try{
connection.close();
}catch(IOException){
//Some Logging
//What else should be done here?
//Is my Connection closed at this point and can I just move on?
//Or should there be anything else done here
//to ensure that the connection is actually closed?
}
}
For example if I have an opened TCP-Connection to let's say an SQL-Server and I am unable to close it because the server has crushed or my device can't reach the device anymore. I would obviously get an IO or in this case an SQLException. If so:
- Should I consider the resource or socket to actually have benn
released? - Will the JVM or the OS handle it overtime? (the OS would probablly do that eventually but it's about what is actually a good programming practice in this case)
- Should I handle the issue myself by trying
Edit 1: I am aware of the "try with resources" construct. I only just wonder how to handle a connection closing if the Connection I am using does not implement AutoCloseable.
java exception-handling connection inputstream ioexception
add a comment |
up vote
1
down vote
favorite
I am wondering, if I have some sort of Connection or Stream in my code that should be closed to release ressources, what does it mean for the connection instance itself?
Code example:
CustomConnection connection;
try{
connection = //some code that opens a connection
//some code using the connection
}catch(IOException){
//Some logging and Handling of IOExceptions
}finally{
//resources cleanup
try{
connection.close();
}catch(IOException){
//Some Logging
//What else should be done here?
//Is my Connection closed at this point and can I just move on?
//Or should there be anything else done here
//to ensure that the connection is actually closed?
}
}
For example if I have an opened TCP-Connection to let's say an SQL-Server and I am unable to close it because the server has crushed or my device can't reach the device anymore. I would obviously get an IO or in this case an SQLException. If so:
- Should I consider the resource or socket to actually have benn
released? - Will the JVM or the OS handle it overtime? (the OS would probablly do that eventually but it's about what is actually a good programming practice in this case)
- Should I handle the issue myself by trying
Edit 1: I am aware of the "try with resources" construct. I only just wonder how to handle a connection closing if the Connection I am using does not implement AutoCloseable.
java exception-handling connection inputstream ioexception
Java does have a "try with resources" language implementation, that takes care of your issues directly. See docs.oracle.com/javase/tutorial/essential/exceptions/…
– Dragonthoughts
Nov 19 at 16:33
1
I agree with @Dragonthoughts on this. Use atry with resources
to insure your connection is always closed. You do need afinally
statement then either.
– Dom
Nov 19 at 16:35
add a comment |
up vote
1
down vote
favorite
up vote
1
down vote
favorite
I am wondering, if I have some sort of Connection or Stream in my code that should be closed to release ressources, what does it mean for the connection instance itself?
Code example:
CustomConnection connection;
try{
connection = //some code that opens a connection
//some code using the connection
}catch(IOException){
//Some logging and Handling of IOExceptions
}finally{
//resources cleanup
try{
connection.close();
}catch(IOException){
//Some Logging
//What else should be done here?
//Is my Connection closed at this point and can I just move on?
//Or should there be anything else done here
//to ensure that the connection is actually closed?
}
}
For example if I have an opened TCP-Connection to let's say an SQL-Server and I am unable to close it because the server has crushed or my device can't reach the device anymore. I would obviously get an IO or in this case an SQLException. If so:
- Should I consider the resource or socket to actually have benn
released? - Will the JVM or the OS handle it overtime? (the OS would probablly do that eventually but it's about what is actually a good programming practice in this case)
- Should I handle the issue myself by trying
Edit 1: I am aware of the "try with resources" construct. I only just wonder how to handle a connection closing if the Connection I am using does not implement AutoCloseable.
java exception-handling connection inputstream ioexception
I am wondering, if I have some sort of Connection or Stream in my code that should be closed to release ressources, what does it mean for the connection instance itself?
Code example:
CustomConnection connection;
try{
connection = //some code that opens a connection
//some code using the connection
}catch(IOException){
//Some logging and Handling of IOExceptions
}finally{
//resources cleanup
try{
connection.close();
}catch(IOException){
//Some Logging
//What else should be done here?
//Is my Connection closed at this point and can I just move on?
//Or should there be anything else done here
//to ensure that the connection is actually closed?
}
}
For example if I have an opened TCP-Connection to let's say an SQL-Server and I am unable to close it because the server has crushed or my device can't reach the device anymore. I would obviously get an IO or in this case an SQLException. If so:
- Should I consider the resource or socket to actually have benn
released? - Will the JVM or the OS handle it overtime? (the OS would probablly do that eventually but it's about what is actually a good programming practice in this case)
- Should I handle the issue myself by trying
Edit 1: I am aware of the "try with resources" construct. I only just wonder how to handle a connection closing if the Connection I am using does not implement AutoCloseable.
java exception-handling connection inputstream ioexception
java exception-handling connection inputstream ioexception
edited Nov 19 at 17:06
asked Nov 19 at 16:30
patvax
238
238
Java does have a "try with resources" language implementation, that takes care of your issues directly. See docs.oracle.com/javase/tutorial/essential/exceptions/…
– Dragonthoughts
Nov 19 at 16:33
1
I agree with @Dragonthoughts on this. Use atry with resources
to insure your connection is always closed. You do need afinally
statement then either.
– Dom
Nov 19 at 16:35
add a comment |
Java does have a "try with resources" language implementation, that takes care of your issues directly. See docs.oracle.com/javase/tutorial/essential/exceptions/…
– Dragonthoughts
Nov 19 at 16:33
1
I agree with @Dragonthoughts on this. Use atry with resources
to insure your connection is always closed. You do need afinally
statement then either.
– Dom
Nov 19 at 16:35
Java does have a "try with resources" language implementation, that takes care of your issues directly. See docs.oracle.com/javase/tutorial/essential/exceptions/…
– Dragonthoughts
Nov 19 at 16:33
Java does have a "try with resources" language implementation, that takes care of your issues directly. See docs.oracle.com/javase/tutorial/essential/exceptions/…
– Dragonthoughts
Nov 19 at 16:33
1
1
I agree with @Dragonthoughts on this. Use a
try with resources
to insure your connection is always closed. You do need a finally
statement then either.– Dom
Nov 19 at 16:35
I agree with @Dragonthoughts on this. Use a
try with resources
to insure your connection is always closed. You do need a finally
statement then either.– Dom
Nov 19 at 16:35
add a comment |
1 Answer
1
active
oldest
votes
up vote
1
down vote
accepted
I would have CustomConnection
implement the AutoClosable
interface so that it can be used within a try-with-resources statement:
try (CustomConnection connection = ...) {
} catch (IOException e) {
// connection is not in scope here, and it is closed.
}
From Oracle's try-with-resources tutorial, it states:
Note: A try-with-resources statement can have catch and finally blocks just like an ordinary try statement. In a try-with-resources statement, any catch or finally block is run after the resources declared have been closed.
Using this to answer your question, your resources will be closed upon entering either a catch
or finally
block.
If initializing the connection can throw an exception, you'll have to watch out for suppressed exceptions:
An exception can be thrown from the block of code associated with the try-with-resources statement. In the example writeToFileZipFileContents, an exception can be thrown from the try block, and up to two exceptions can be thrown from the try-with-resources statement when it tries to close the ZipFile and BufferedWriter objects. If an exception is thrown from the try block and one or more exceptions are thrown from the try-with-resources statement, then those exceptions thrown from the try-with-resources statement are suppressed, and the exception thrown by the block is the one that is thrown by the writeToFileZipFileContents method. You can retrieve these suppressed exceptions by calling the Throwable.getSuppressed method from the exception thrown by the try block.
Yes I am aware of the try with resources construct. I only just wonder how to handle a connection closing if the Connection I am using does not implement AutoCloseable.
– patvax
Nov 19 at 17:04
1
@patvax in that case, you could create a wrapper class for your connection that implementsAutoClosable
. It's a lot easier than having to handle closing them yourself.
– Jacob G.
Nov 19 at 17:06
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
1
down vote
accepted
I would have CustomConnection
implement the AutoClosable
interface so that it can be used within a try-with-resources statement:
try (CustomConnection connection = ...) {
} catch (IOException e) {
// connection is not in scope here, and it is closed.
}
From Oracle's try-with-resources tutorial, it states:
Note: A try-with-resources statement can have catch and finally blocks just like an ordinary try statement. In a try-with-resources statement, any catch or finally block is run after the resources declared have been closed.
Using this to answer your question, your resources will be closed upon entering either a catch
or finally
block.
If initializing the connection can throw an exception, you'll have to watch out for suppressed exceptions:
An exception can be thrown from the block of code associated with the try-with-resources statement. In the example writeToFileZipFileContents, an exception can be thrown from the try block, and up to two exceptions can be thrown from the try-with-resources statement when it tries to close the ZipFile and BufferedWriter objects. If an exception is thrown from the try block and one or more exceptions are thrown from the try-with-resources statement, then those exceptions thrown from the try-with-resources statement are suppressed, and the exception thrown by the block is the one that is thrown by the writeToFileZipFileContents method. You can retrieve these suppressed exceptions by calling the Throwable.getSuppressed method from the exception thrown by the try block.
Yes I am aware of the try with resources construct. I only just wonder how to handle a connection closing if the Connection I am using does not implement AutoCloseable.
– patvax
Nov 19 at 17:04
1
@patvax in that case, you could create a wrapper class for your connection that implementsAutoClosable
. It's a lot easier than having to handle closing them yourself.
– Jacob G.
Nov 19 at 17:06
add a comment |
up vote
1
down vote
accepted
I would have CustomConnection
implement the AutoClosable
interface so that it can be used within a try-with-resources statement:
try (CustomConnection connection = ...) {
} catch (IOException e) {
// connection is not in scope here, and it is closed.
}
From Oracle's try-with-resources tutorial, it states:
Note: A try-with-resources statement can have catch and finally blocks just like an ordinary try statement. In a try-with-resources statement, any catch or finally block is run after the resources declared have been closed.
Using this to answer your question, your resources will be closed upon entering either a catch
or finally
block.
If initializing the connection can throw an exception, you'll have to watch out for suppressed exceptions:
An exception can be thrown from the block of code associated with the try-with-resources statement. In the example writeToFileZipFileContents, an exception can be thrown from the try block, and up to two exceptions can be thrown from the try-with-resources statement when it tries to close the ZipFile and BufferedWriter objects. If an exception is thrown from the try block and one or more exceptions are thrown from the try-with-resources statement, then those exceptions thrown from the try-with-resources statement are suppressed, and the exception thrown by the block is the one that is thrown by the writeToFileZipFileContents method. You can retrieve these suppressed exceptions by calling the Throwable.getSuppressed method from the exception thrown by the try block.
Yes I am aware of the try with resources construct. I only just wonder how to handle a connection closing if the Connection I am using does not implement AutoCloseable.
– patvax
Nov 19 at 17:04
1
@patvax in that case, you could create a wrapper class for your connection that implementsAutoClosable
. It's a lot easier than having to handle closing them yourself.
– Jacob G.
Nov 19 at 17:06
add a comment |
up vote
1
down vote
accepted
up vote
1
down vote
accepted
I would have CustomConnection
implement the AutoClosable
interface so that it can be used within a try-with-resources statement:
try (CustomConnection connection = ...) {
} catch (IOException e) {
// connection is not in scope here, and it is closed.
}
From Oracle's try-with-resources tutorial, it states:
Note: A try-with-resources statement can have catch and finally blocks just like an ordinary try statement. In a try-with-resources statement, any catch or finally block is run after the resources declared have been closed.
Using this to answer your question, your resources will be closed upon entering either a catch
or finally
block.
If initializing the connection can throw an exception, you'll have to watch out for suppressed exceptions:
An exception can be thrown from the block of code associated with the try-with-resources statement. In the example writeToFileZipFileContents, an exception can be thrown from the try block, and up to two exceptions can be thrown from the try-with-resources statement when it tries to close the ZipFile and BufferedWriter objects. If an exception is thrown from the try block and one or more exceptions are thrown from the try-with-resources statement, then those exceptions thrown from the try-with-resources statement are suppressed, and the exception thrown by the block is the one that is thrown by the writeToFileZipFileContents method. You can retrieve these suppressed exceptions by calling the Throwable.getSuppressed method from the exception thrown by the try block.
I would have CustomConnection
implement the AutoClosable
interface so that it can be used within a try-with-resources statement:
try (CustomConnection connection = ...) {
} catch (IOException e) {
// connection is not in scope here, and it is closed.
}
From Oracle's try-with-resources tutorial, it states:
Note: A try-with-resources statement can have catch and finally blocks just like an ordinary try statement. In a try-with-resources statement, any catch or finally block is run after the resources declared have been closed.
Using this to answer your question, your resources will be closed upon entering either a catch
or finally
block.
If initializing the connection can throw an exception, you'll have to watch out for suppressed exceptions:
An exception can be thrown from the block of code associated with the try-with-resources statement. In the example writeToFileZipFileContents, an exception can be thrown from the try block, and up to two exceptions can be thrown from the try-with-resources statement when it tries to close the ZipFile and BufferedWriter objects. If an exception is thrown from the try block and one or more exceptions are thrown from the try-with-resources statement, then those exceptions thrown from the try-with-resources statement are suppressed, and the exception thrown by the block is the one that is thrown by the writeToFileZipFileContents method. You can retrieve these suppressed exceptions by calling the Throwable.getSuppressed method from the exception thrown by the try block.
answered Nov 19 at 16:38


Jacob G.
15k51962
15k51962
Yes I am aware of the try with resources construct. I only just wonder how to handle a connection closing if the Connection I am using does not implement AutoCloseable.
– patvax
Nov 19 at 17:04
1
@patvax in that case, you could create a wrapper class for your connection that implementsAutoClosable
. It's a lot easier than having to handle closing them yourself.
– Jacob G.
Nov 19 at 17:06
add a comment |
Yes I am aware of the try with resources construct. I only just wonder how to handle a connection closing if the Connection I am using does not implement AutoCloseable.
– patvax
Nov 19 at 17:04
1
@patvax in that case, you could create a wrapper class for your connection that implementsAutoClosable
. It's a lot easier than having to handle closing them yourself.
– Jacob G.
Nov 19 at 17:06
Yes I am aware of the try with resources construct. I only just wonder how to handle a connection closing if the Connection I am using does not implement AutoCloseable.
– patvax
Nov 19 at 17:04
Yes I am aware of the try with resources construct. I only just wonder how to handle a connection closing if the Connection I am using does not implement AutoCloseable.
– patvax
Nov 19 at 17:04
1
1
@patvax in that case, you could create a wrapper class for your connection that implements
AutoClosable
. It's a lot easier than having to handle closing them yourself.– Jacob G.
Nov 19 at 17:06
@patvax in that case, you could create a wrapper class for your connection that implements
AutoClosable
. It's a lot easier than having to handle closing them yourself.– Jacob G.
Nov 19 at 17:06
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53378962%2fjava-is-a-connection-stream-already-closed-if-an-exception-occurs-and-how-to-h%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
rxyJ46aXMfc,bN 5u3,b9ZUPMfTo7j DQSzPQ aMxdwenGyVW7N9,dQBHx4CyetLNb,h6i,Cqa78w
Java does have a "try with resources" language implementation, that takes care of your issues directly. See docs.oracle.com/javase/tutorial/essential/exceptions/…
– Dragonthoughts
Nov 19 at 16:33
1
I agree with @Dragonthoughts on this. Use a
try with resources
to insure your connection is always closed. You do need afinally
statement then either.– Dom
Nov 19 at 16:35