Using Puppeteer to get a handle to the new page after “_blank” click?
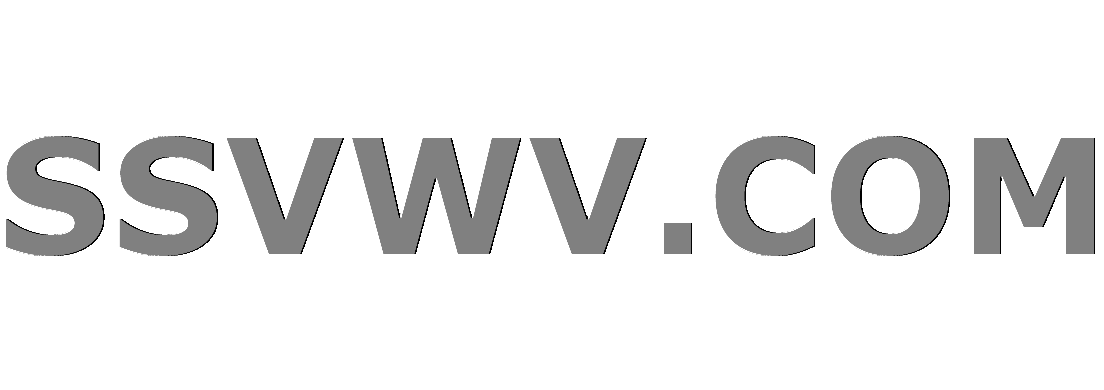
Multi tool use
up vote
1
down vote
favorite
Looking at this simple code :
const browser = await puppeteer.launch({headless: false});
const page: Page = await browser.newPage();
await page.evaluate((a) =>
{
//This will open a new page :
([...document.querySelectorAll("a")][0] as HTMLElement).click();
});
If the clicked A
(anchor) element has target="_blank"
, then the page would be opened as a new page.
It's not the original page object.
Question:
How can I get the new page object after a user has clicked on <a target="_blank"
?
web-scraping puppeteer
add a comment |
up vote
1
down vote
favorite
Looking at this simple code :
const browser = await puppeteer.launch({headless: false});
const page: Page = await browser.newPage();
await page.evaluate((a) =>
{
//This will open a new page :
([...document.querySelectorAll("a")][0] as HTMLElement).click();
});
If the clicked A
(anchor) element has target="_blank"
, then the page would be opened as a new page.
It's not the original page object.
Question:
How can I get the new page object after a user has clicked on <a target="_blank"
?
web-scraping puppeteer
Did you check this ticket?
– Andersson
Nov 19 at 12:30
@Andersson Thanks for response. Before I delete it as a dup , I didn't see in the answers there , How can I get a handle to the new page that was opened ( without scanning pages)
– Royi Namir
Nov 19 at 12:37
Unfortunately I don't know another way to identify last opened tab except handling list of tabs...
– Andersson
Nov 19 at 12:47
You could probably save yourself a headache by using fetch() instead of clicking those if you're only after the page contents.
– pguardiario
Nov 19 at 22:52
add a comment |
up vote
1
down vote
favorite
up vote
1
down vote
favorite
Looking at this simple code :
const browser = await puppeteer.launch({headless: false});
const page: Page = await browser.newPage();
await page.evaluate((a) =>
{
//This will open a new page :
([...document.querySelectorAll("a")][0] as HTMLElement).click();
});
If the clicked A
(anchor) element has target="_blank"
, then the page would be opened as a new page.
It's not the original page object.
Question:
How can I get the new page object after a user has clicked on <a target="_blank"
?
web-scraping puppeteer
Looking at this simple code :
const browser = await puppeteer.launch({headless: false});
const page: Page = await browser.newPage();
await page.evaluate((a) =>
{
//This will open a new page :
([...document.querySelectorAll("a")][0] as HTMLElement).click();
});
If the clicked A
(anchor) element has target="_blank"
, then the page would be opened as a new page.
It's not the original page object.
Question:
How can I get the new page object after a user has clicked on <a target="_blank"
?
web-scraping puppeteer
web-scraping puppeteer
asked Nov 19 at 12:15


Royi Namir
74.1k98328585
74.1k98328585
Did you check this ticket?
– Andersson
Nov 19 at 12:30
@Andersson Thanks for response. Before I delete it as a dup , I didn't see in the answers there , How can I get a handle to the new page that was opened ( without scanning pages)
– Royi Namir
Nov 19 at 12:37
Unfortunately I don't know another way to identify last opened tab except handling list of tabs...
– Andersson
Nov 19 at 12:47
You could probably save yourself a headache by using fetch() instead of clicking those if you're only after the page contents.
– pguardiario
Nov 19 at 22:52
add a comment |
Did you check this ticket?
– Andersson
Nov 19 at 12:30
@Andersson Thanks for response. Before I delete it as a dup , I didn't see in the answers there , How can I get a handle to the new page that was opened ( without scanning pages)
– Royi Namir
Nov 19 at 12:37
Unfortunately I don't know another way to identify last opened tab except handling list of tabs...
– Andersson
Nov 19 at 12:47
You could probably save yourself a headache by using fetch() instead of clicking those if you're only after the page contents.
– pguardiario
Nov 19 at 22:52
Did you check this ticket?
– Andersson
Nov 19 at 12:30
Did you check this ticket?
– Andersson
Nov 19 at 12:30
@Andersson Thanks for response. Before I delete it as a dup , I didn't see in the answers there , How can I get a handle to the new page that was opened ( without scanning pages)
– Royi Namir
Nov 19 at 12:37
@Andersson Thanks for response. Before I delete it as a dup , I didn't see in the answers there , How can I get a handle to the new page that was opened ( without scanning pages)
– Royi Namir
Nov 19 at 12:37
Unfortunately I don't know another way to identify last opened tab except handling list of tabs...
– Andersson
Nov 19 at 12:47
Unfortunately I don't know another way to identify last opened tab except handling list of tabs...
– Andersson
Nov 19 at 12:47
You could probably save yourself a headache by using fetch() instead of clicking those if you're only after the page contents.
– pguardiario
Nov 19 at 22:52
You could probably save yourself a headache by using fetch() instead of clicking those if you're only after the page contents.
– pguardiario
Nov 19 at 22:52
add a comment |
1 Answer
1
active
oldest
votes
up vote
1
down vote
accepted
This can be done like this:
const [newTarget] = await Promise.all([
// Await new target to be created with the proper opener
new Promise(x => browser.on('targetcreated', target => {
if (target.opener() !== page.target())
return;
browser.removeListener('targetcreated', arguments.callee);
x();
})),
page.click('link'),
])
// Attach to the newly opened page.
const newPage = await newTarget.page();
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
1
down vote
accepted
This can be done like this:
const [newTarget] = await Promise.all([
// Await new target to be created with the proper opener
new Promise(x => browser.on('targetcreated', target => {
if (target.opener() !== page.target())
return;
browser.removeListener('targetcreated', arguments.callee);
x();
})),
page.click('link'),
])
// Attach to the newly opened page.
const newPage = await newTarget.page();
add a comment |
up vote
1
down vote
accepted
This can be done like this:
const [newTarget] = await Promise.all([
// Await new target to be created with the proper opener
new Promise(x => browser.on('targetcreated', target => {
if (target.opener() !== page.target())
return;
browser.removeListener('targetcreated', arguments.callee);
x();
})),
page.click('link'),
])
// Attach to the newly opened page.
const newPage = await newTarget.page();
add a comment |
up vote
1
down vote
accepted
up vote
1
down vote
accepted
This can be done like this:
const [newTarget] = await Promise.all([
// Await new target to be created with the proper opener
new Promise(x => browser.on('targetcreated', target => {
if (target.opener() !== page.target())
return;
browser.removeListener('targetcreated', arguments.callee);
x();
})),
page.click('link'),
])
// Attach to the newly opened page.
const newPage = await newTarget.page();
This can be done like this:
const [newTarget] = await Promise.all([
// Await new target to be created with the proper opener
new Promise(x => browser.on('targetcreated', target => {
if (target.opener() !== page.target())
return;
browser.removeListener('targetcreated', arguments.callee);
x();
})),
page.click('link'),
])
// Attach to the newly opened page.
const newPage = await newTarget.page();
answered Nov 20 at 2:42
Andrey Lushnikov
1,76621618
1,76621618
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53374443%2fusing-puppeteer-to-get-a-handle-to-the-new-page-after-blank-click%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
yy5bcOBo
Did you check this ticket?
– Andersson
Nov 19 at 12:30
@Andersson Thanks for response. Before I delete it as a dup , I didn't see in the answers there , How can I get a handle to the new page that was opened ( without scanning pages)
– Royi Namir
Nov 19 at 12:37
Unfortunately I don't know another way to identify last opened tab except handling list of tabs...
– Andersson
Nov 19 at 12:47
You could probably save yourself a headache by using fetch() instead of clicking those if you're only after the page contents.
– pguardiario
Nov 19 at 22:52