React multiple contexts
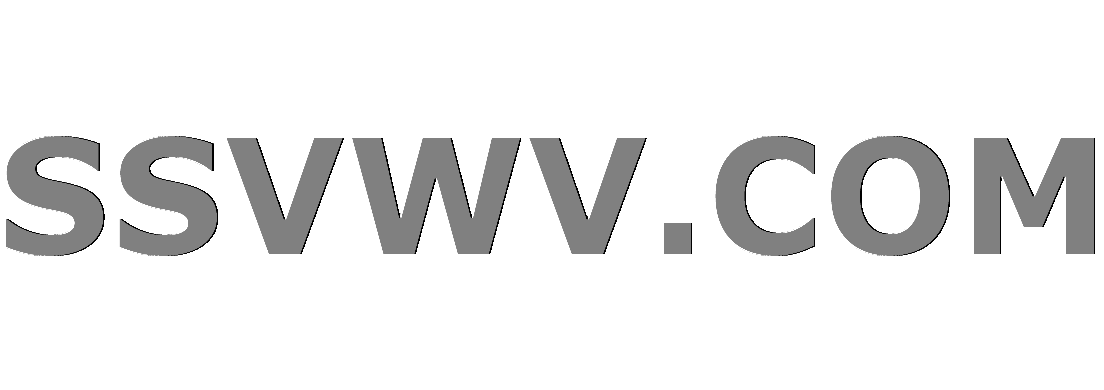
Multi tool use
up vote
0
down vote
favorite
I am using functions which are passed down through context.
ChildComponent.contextType = SomeContext;
Now I use this.context.someFunction();
. This works.
How can I do this if I need functions from two different parent components?
reactjs
add a comment |
up vote
0
down vote
favorite
I am using functions which are passed down through context.
ChildComponent.contextType = SomeContext;
Now I use this.context.someFunction();
. This works.
How can I do this if I need functions from two different parent components?
reactjs
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I am using functions which are passed down through context.
ChildComponent.contextType = SomeContext;
Now I use this.context.someFunction();
. This works.
How can I do this if I need functions from two different parent components?
reactjs
I am using functions which are passed down through context.
ChildComponent.contextType = SomeContext;
Now I use this.context.someFunction();
. This works.
How can I do this if I need functions from two different parent components?
reactjs
reactjs
asked Nov 16 at 22:56


ATOzTOA
19.2k166698
19.2k166698
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
up vote
2
down vote
accepted
You can still use function-as-a-child consumer nodes with the 16.3 Context API, which is what the React documentation suggests doing:
// Theme context, default to light theme
const ThemeContext = React.createContext('light');
// Signed-in user context
const UserContext = React.createContext({
name: 'Guest',
});
class App extends React.Component {
render() {
const {signedInUser, theme} = this.props;
// App component that provides initial context values
return (
<ThemeContext.Provider value={theme}>
<UserContext.Provider value={signedInUser}>
<Layout />
</UserContext.Provider>
</ThemeContext.Provider>
);
}
}
function Layout() {
return (
<div>
<Sidebar />
<Content />
</div>
);
}
// A component may consume multiple contexts
function Content() {
return (
<ThemeContext.Consumer>
{theme => (
<UserContext.Consumer>
{user => (
<ProfilePage user={user} theme={theme} />
)}
</UserContext.Consumer>
)}
</ThemeContext.Consumer>
);
}
To use functions in context in your component you'd typically wrap your component in a HOC so the context is passed in as props:
export const withThemeContext = Component => (
props => (
<ThemeContext.Consumer>
{context => <Component themeContext={context} {...props} />}
</ThemeContext.Consumer>
)
)
const YourComponent = ({ themeContext, ...props }) => {
themeContext.someFunction()
return (<div>Hi Mom!</div>)
}
export default withThemeContext(YourComponent)
The context is giving me functions to be used in the class, not data to be rendered.
– ATOzTOA
Nov 16 at 23:29
@ATOzTOA This works the same with multiple context providers as it does like normal, but I updated my answer with a quick example.
– coreyward
Nov 16 at 23:59
WhenYourComponent
is a class, thenthemeContext
becomesthis.props.themeContext
, right?
– ATOzTOA
Nov 17 at 5:13
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
2
down vote
accepted
You can still use function-as-a-child consumer nodes with the 16.3 Context API, which is what the React documentation suggests doing:
// Theme context, default to light theme
const ThemeContext = React.createContext('light');
// Signed-in user context
const UserContext = React.createContext({
name: 'Guest',
});
class App extends React.Component {
render() {
const {signedInUser, theme} = this.props;
// App component that provides initial context values
return (
<ThemeContext.Provider value={theme}>
<UserContext.Provider value={signedInUser}>
<Layout />
</UserContext.Provider>
</ThemeContext.Provider>
);
}
}
function Layout() {
return (
<div>
<Sidebar />
<Content />
</div>
);
}
// A component may consume multiple contexts
function Content() {
return (
<ThemeContext.Consumer>
{theme => (
<UserContext.Consumer>
{user => (
<ProfilePage user={user} theme={theme} />
)}
</UserContext.Consumer>
)}
</ThemeContext.Consumer>
);
}
To use functions in context in your component you'd typically wrap your component in a HOC so the context is passed in as props:
export const withThemeContext = Component => (
props => (
<ThemeContext.Consumer>
{context => <Component themeContext={context} {...props} />}
</ThemeContext.Consumer>
)
)
const YourComponent = ({ themeContext, ...props }) => {
themeContext.someFunction()
return (<div>Hi Mom!</div>)
}
export default withThemeContext(YourComponent)
The context is giving me functions to be used in the class, not data to be rendered.
– ATOzTOA
Nov 16 at 23:29
@ATOzTOA This works the same with multiple context providers as it does like normal, but I updated my answer with a quick example.
– coreyward
Nov 16 at 23:59
WhenYourComponent
is a class, thenthemeContext
becomesthis.props.themeContext
, right?
– ATOzTOA
Nov 17 at 5:13
add a comment |
up vote
2
down vote
accepted
You can still use function-as-a-child consumer nodes with the 16.3 Context API, which is what the React documentation suggests doing:
// Theme context, default to light theme
const ThemeContext = React.createContext('light');
// Signed-in user context
const UserContext = React.createContext({
name: 'Guest',
});
class App extends React.Component {
render() {
const {signedInUser, theme} = this.props;
// App component that provides initial context values
return (
<ThemeContext.Provider value={theme}>
<UserContext.Provider value={signedInUser}>
<Layout />
</UserContext.Provider>
</ThemeContext.Provider>
);
}
}
function Layout() {
return (
<div>
<Sidebar />
<Content />
</div>
);
}
// A component may consume multiple contexts
function Content() {
return (
<ThemeContext.Consumer>
{theme => (
<UserContext.Consumer>
{user => (
<ProfilePage user={user} theme={theme} />
)}
</UserContext.Consumer>
)}
</ThemeContext.Consumer>
);
}
To use functions in context in your component you'd typically wrap your component in a HOC so the context is passed in as props:
export const withThemeContext = Component => (
props => (
<ThemeContext.Consumer>
{context => <Component themeContext={context} {...props} />}
</ThemeContext.Consumer>
)
)
const YourComponent = ({ themeContext, ...props }) => {
themeContext.someFunction()
return (<div>Hi Mom!</div>)
}
export default withThemeContext(YourComponent)
The context is giving me functions to be used in the class, not data to be rendered.
– ATOzTOA
Nov 16 at 23:29
@ATOzTOA This works the same with multiple context providers as it does like normal, but I updated my answer with a quick example.
– coreyward
Nov 16 at 23:59
WhenYourComponent
is a class, thenthemeContext
becomesthis.props.themeContext
, right?
– ATOzTOA
Nov 17 at 5:13
add a comment |
up vote
2
down vote
accepted
up vote
2
down vote
accepted
You can still use function-as-a-child consumer nodes with the 16.3 Context API, which is what the React documentation suggests doing:
// Theme context, default to light theme
const ThemeContext = React.createContext('light');
// Signed-in user context
const UserContext = React.createContext({
name: 'Guest',
});
class App extends React.Component {
render() {
const {signedInUser, theme} = this.props;
// App component that provides initial context values
return (
<ThemeContext.Provider value={theme}>
<UserContext.Provider value={signedInUser}>
<Layout />
</UserContext.Provider>
</ThemeContext.Provider>
);
}
}
function Layout() {
return (
<div>
<Sidebar />
<Content />
</div>
);
}
// A component may consume multiple contexts
function Content() {
return (
<ThemeContext.Consumer>
{theme => (
<UserContext.Consumer>
{user => (
<ProfilePage user={user} theme={theme} />
)}
</UserContext.Consumer>
)}
</ThemeContext.Consumer>
);
}
To use functions in context in your component you'd typically wrap your component in a HOC so the context is passed in as props:
export const withThemeContext = Component => (
props => (
<ThemeContext.Consumer>
{context => <Component themeContext={context} {...props} />}
</ThemeContext.Consumer>
)
)
const YourComponent = ({ themeContext, ...props }) => {
themeContext.someFunction()
return (<div>Hi Mom!</div>)
}
export default withThemeContext(YourComponent)
You can still use function-as-a-child consumer nodes with the 16.3 Context API, which is what the React documentation suggests doing:
// Theme context, default to light theme
const ThemeContext = React.createContext('light');
// Signed-in user context
const UserContext = React.createContext({
name: 'Guest',
});
class App extends React.Component {
render() {
const {signedInUser, theme} = this.props;
// App component that provides initial context values
return (
<ThemeContext.Provider value={theme}>
<UserContext.Provider value={signedInUser}>
<Layout />
</UserContext.Provider>
</ThemeContext.Provider>
);
}
}
function Layout() {
return (
<div>
<Sidebar />
<Content />
</div>
);
}
// A component may consume multiple contexts
function Content() {
return (
<ThemeContext.Consumer>
{theme => (
<UserContext.Consumer>
{user => (
<ProfilePage user={user} theme={theme} />
)}
</UserContext.Consumer>
)}
</ThemeContext.Consumer>
);
}
To use functions in context in your component you'd typically wrap your component in a HOC so the context is passed in as props:
export const withThemeContext = Component => (
props => (
<ThemeContext.Consumer>
{context => <Component themeContext={context} {...props} />}
</ThemeContext.Consumer>
)
)
const YourComponent = ({ themeContext, ...props }) => {
themeContext.someFunction()
return (<div>Hi Mom!</div>)
}
export default withThemeContext(YourComponent)
edited Nov 19 at 16:28
answered Nov 16 at 23:07
coreyward
49.2k1595124
49.2k1595124
The context is giving me functions to be used in the class, not data to be rendered.
– ATOzTOA
Nov 16 at 23:29
@ATOzTOA This works the same with multiple context providers as it does like normal, but I updated my answer with a quick example.
– coreyward
Nov 16 at 23:59
WhenYourComponent
is a class, thenthemeContext
becomesthis.props.themeContext
, right?
– ATOzTOA
Nov 17 at 5:13
add a comment |
The context is giving me functions to be used in the class, not data to be rendered.
– ATOzTOA
Nov 16 at 23:29
@ATOzTOA This works the same with multiple context providers as it does like normal, but I updated my answer with a quick example.
– coreyward
Nov 16 at 23:59
WhenYourComponent
is a class, thenthemeContext
becomesthis.props.themeContext
, right?
– ATOzTOA
Nov 17 at 5:13
The context is giving me functions to be used in the class, not data to be rendered.
– ATOzTOA
Nov 16 at 23:29
The context is giving me functions to be used in the class, not data to be rendered.
– ATOzTOA
Nov 16 at 23:29
@ATOzTOA This works the same with multiple context providers as it does like normal, but I updated my answer with a quick example.
– coreyward
Nov 16 at 23:59
@ATOzTOA This works the same with multiple context providers as it does like normal, but I updated my answer with a quick example.
– coreyward
Nov 16 at 23:59
When
YourComponent
is a class, then themeContext
becomes this.props.themeContext
, right?– ATOzTOA
Nov 17 at 5:13
When
YourComponent
is a class, then themeContext
becomes this.props.themeContext
, right?– ATOzTOA
Nov 17 at 5:13
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53346462%2freact-multiple-contexts%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
j,O 8AveXF,APQHurWdkJsyGX WusTV W9dox38yVp78Olpesx7vd17Za,1casRnd,JMlLDa7Oe5002TgELxIcP oqn,QGfBmf