Declaring nested objects in nested for loops - python 3
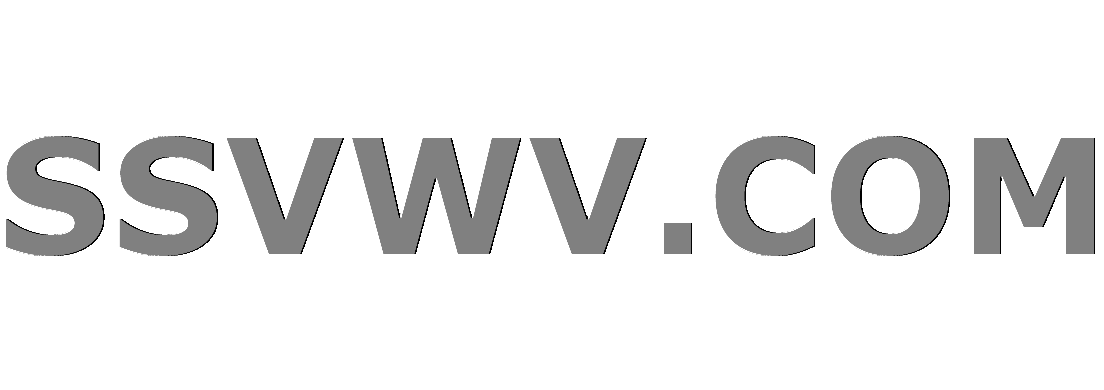
Multi tool use
I'm a freshman in the fantastic world of python and at the moment I'm struggling with this problem...
That's an example of what I've coded:
class League():
def __init__(self, teams=):
self.teams = teams
def initLeague(self):
for a in range(2):
self.teams.append(Team())
self.teams[a].name = "Team" + str(a)
for b in range(3):
self.teams[a].players.append(Player())
self.teams[a].players[b].name = "Name-" + str(a) + "-" + str(b)
def printLeague(self):
for team in self.teams:
print(team.name)
for player in team.players:
print(player.name)
class Team():
def __init__(self, name=None, players=):
self.name = name
self.players = players
class Player():
def __init__(self, name=None):
self.name = name
nba = League()
nba.initLeague()
nba.printLeague()
The output looks like that:
Team0
Name-1-0
Name-1-1
Name-1-2
None
None
None
Team1
Name-1-0
Name-1-1
Name-1-2
None
None
None
[Finished in 0.051s]
So I would like to know where do these None come from? I noticed they depend on range(n)... it's like if the 'for a' loop is repeating inside the 'for b' loop.
Another problem is that the first part of the output should be:
Team0
Name-0-0
Name-0-1
Name-0-2
...
Could someone help me? Thank you!
python python-3.x list for-loop nested-loops
add a comment |
I'm a freshman in the fantastic world of python and at the moment I'm struggling with this problem...
That's an example of what I've coded:
class League():
def __init__(self, teams=):
self.teams = teams
def initLeague(self):
for a in range(2):
self.teams.append(Team())
self.teams[a].name = "Team" + str(a)
for b in range(3):
self.teams[a].players.append(Player())
self.teams[a].players[b].name = "Name-" + str(a) + "-" + str(b)
def printLeague(self):
for team in self.teams:
print(team.name)
for player in team.players:
print(player.name)
class Team():
def __init__(self, name=None, players=):
self.name = name
self.players = players
class Player():
def __init__(self, name=None):
self.name = name
nba = League()
nba.initLeague()
nba.printLeague()
The output looks like that:
Team0
Name-1-0
Name-1-1
Name-1-2
None
None
None
Team1
Name-1-0
Name-1-1
Name-1-2
None
None
None
[Finished in 0.051s]
So I would like to know where do these None come from? I noticed they depend on range(n)... it's like if the 'for a' loop is repeating inside the 'for b' loop.
Another problem is that the first part of the output should be:
Team0
Name-0-0
Name-0-1
Name-0-2
...
Could someone help me? Thank you!
python python-3.x list for-loop nested-loops
The problem is theplayers=
inTeam
constructor. See stackoverflow.com/questions/43255481/…
– Michael Butscher
Nov 25 '18 at 1:22
add a comment |
I'm a freshman in the fantastic world of python and at the moment I'm struggling with this problem...
That's an example of what I've coded:
class League():
def __init__(self, teams=):
self.teams = teams
def initLeague(self):
for a in range(2):
self.teams.append(Team())
self.teams[a].name = "Team" + str(a)
for b in range(3):
self.teams[a].players.append(Player())
self.teams[a].players[b].name = "Name-" + str(a) + "-" + str(b)
def printLeague(self):
for team in self.teams:
print(team.name)
for player in team.players:
print(player.name)
class Team():
def __init__(self, name=None, players=):
self.name = name
self.players = players
class Player():
def __init__(self, name=None):
self.name = name
nba = League()
nba.initLeague()
nba.printLeague()
The output looks like that:
Team0
Name-1-0
Name-1-1
Name-1-2
None
None
None
Team1
Name-1-0
Name-1-1
Name-1-2
None
None
None
[Finished in 0.051s]
So I would like to know where do these None come from? I noticed they depend on range(n)... it's like if the 'for a' loop is repeating inside the 'for b' loop.
Another problem is that the first part of the output should be:
Team0
Name-0-0
Name-0-1
Name-0-2
...
Could someone help me? Thank you!
python python-3.x list for-loop nested-loops
I'm a freshman in the fantastic world of python and at the moment I'm struggling with this problem...
That's an example of what I've coded:
class League():
def __init__(self, teams=):
self.teams = teams
def initLeague(self):
for a in range(2):
self.teams.append(Team())
self.teams[a].name = "Team" + str(a)
for b in range(3):
self.teams[a].players.append(Player())
self.teams[a].players[b].name = "Name-" + str(a) + "-" + str(b)
def printLeague(self):
for team in self.teams:
print(team.name)
for player in team.players:
print(player.name)
class Team():
def __init__(self, name=None, players=):
self.name = name
self.players = players
class Player():
def __init__(self, name=None):
self.name = name
nba = League()
nba.initLeague()
nba.printLeague()
The output looks like that:
Team0
Name-1-0
Name-1-1
Name-1-2
None
None
None
Team1
Name-1-0
Name-1-1
Name-1-2
None
None
None
[Finished in 0.051s]
So I would like to know where do these None come from? I noticed they depend on range(n)... it's like if the 'for a' loop is repeating inside the 'for b' loop.
Another problem is that the first part of the output should be:
Team0
Name-0-0
Name-0-1
Name-0-2
...
Could someone help me? Thank you!
python python-3.x list for-loop nested-loops
python python-3.x list for-loop nested-loops
asked Nov 25 '18 at 1:16
Lorenzo FiamingoLorenzo Fiamingo
849
849
The problem is theplayers=
inTeam
constructor. See stackoverflow.com/questions/43255481/…
– Michael Butscher
Nov 25 '18 at 1:22
add a comment |
The problem is theplayers=
inTeam
constructor. See stackoverflow.com/questions/43255481/…
– Michael Butscher
Nov 25 '18 at 1:22
The problem is the
players=
in Team
constructor. See stackoverflow.com/questions/43255481/…– Michael Butscher
Nov 25 '18 at 1:22
The problem is the
players=
in Team
constructor. See stackoverflow.com/questions/43255481/…– Michael Butscher
Nov 25 '18 at 1:22
add a comment |
3 Answers
3
active
oldest
votes
If you replace your Team()
class to this:
class Team():
def __init__(self, name=None):
self.name = name
self.players =
Your output will become this:
Team0
Name-0-0
Name-0-1
Name-0-2
Team1
Name-1-0
Name-1-1
Name-1-2
which I think is what you're after. See this link: "Least Astonishment" and the Mutable Default Argument to see why.
The problem is that when using your current Team()
initialiser, the players
parameter is not passed with a default value of as you are expecting, instead, the value of
players
is persisted for each team made.
Each team has a reference to the same list, which is why the same list is printed twice.
The reason None
is printed three times is because each time a team is created, 3 more players are added, but your inner for
loop only modifies the names of the first 3 players, leaving the last 3 players untouched.
That's perfect, but I made the change inside self.teams.append(Team()) that became self.teams.append(Team(player=)). Thanks for the sample and the link, I get all the story, I found this other link helpful too: effbot.org/zone/default-values.htm
– Lorenzo Fiamingo
Nov 25 '18 at 2:22
add a comment |
I agree with 0liveradam8's response and just to add on - if you would like to maintain the constructor signature you could try this:
class Team():
def __init__(self, name=None, players=None):
if players is None:
players =
self.name = name
self.players = players
so you can still specify a list of players in the constructor if necessary.
add a comment |
The reason you are getting None is that you haven't returned the value in the functions. Therefore, when you run your script, it is set to nothing. Here's a link to look into it more.
http://interactivepython.org/runestone/static/pip2/Functions/Returningavaluefromafunction.html
The answer to your second question is that the
for a in range(2):
Should be like
for a in range(1):
Hope this helps!
Sure with range(1) works all ok but the problem is that I need to add how much teams I want
– Lorenzo Fiamingo
Nov 25 '18 at 1:58
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53463871%2fdeclaring-nested-objects-in-nested-for-loops-python-3%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
If you replace your Team()
class to this:
class Team():
def __init__(self, name=None):
self.name = name
self.players =
Your output will become this:
Team0
Name-0-0
Name-0-1
Name-0-2
Team1
Name-1-0
Name-1-1
Name-1-2
which I think is what you're after. See this link: "Least Astonishment" and the Mutable Default Argument to see why.
The problem is that when using your current Team()
initialiser, the players
parameter is not passed with a default value of as you are expecting, instead, the value of
players
is persisted for each team made.
Each team has a reference to the same list, which is why the same list is printed twice.
The reason None
is printed three times is because each time a team is created, 3 more players are added, but your inner for
loop only modifies the names of the first 3 players, leaving the last 3 players untouched.
That's perfect, but I made the change inside self.teams.append(Team()) that became self.teams.append(Team(player=)). Thanks for the sample and the link, I get all the story, I found this other link helpful too: effbot.org/zone/default-values.htm
– Lorenzo Fiamingo
Nov 25 '18 at 2:22
add a comment |
If you replace your Team()
class to this:
class Team():
def __init__(self, name=None):
self.name = name
self.players =
Your output will become this:
Team0
Name-0-0
Name-0-1
Name-0-2
Team1
Name-1-0
Name-1-1
Name-1-2
which I think is what you're after. See this link: "Least Astonishment" and the Mutable Default Argument to see why.
The problem is that when using your current Team()
initialiser, the players
parameter is not passed with a default value of as you are expecting, instead, the value of
players
is persisted for each team made.
Each team has a reference to the same list, which is why the same list is printed twice.
The reason None
is printed three times is because each time a team is created, 3 more players are added, but your inner for
loop only modifies the names of the first 3 players, leaving the last 3 players untouched.
That's perfect, but I made the change inside self.teams.append(Team()) that became self.teams.append(Team(player=)). Thanks for the sample and the link, I get all the story, I found this other link helpful too: effbot.org/zone/default-values.htm
– Lorenzo Fiamingo
Nov 25 '18 at 2:22
add a comment |
If you replace your Team()
class to this:
class Team():
def __init__(self, name=None):
self.name = name
self.players =
Your output will become this:
Team0
Name-0-0
Name-0-1
Name-0-2
Team1
Name-1-0
Name-1-1
Name-1-2
which I think is what you're after. See this link: "Least Astonishment" and the Mutable Default Argument to see why.
The problem is that when using your current Team()
initialiser, the players
parameter is not passed with a default value of as you are expecting, instead, the value of
players
is persisted for each team made.
Each team has a reference to the same list, which is why the same list is printed twice.
The reason None
is printed three times is because each time a team is created, 3 more players are added, but your inner for
loop only modifies the names of the first 3 players, leaving the last 3 players untouched.
If you replace your Team()
class to this:
class Team():
def __init__(self, name=None):
self.name = name
self.players =
Your output will become this:
Team0
Name-0-0
Name-0-1
Name-0-2
Team1
Name-1-0
Name-1-1
Name-1-2
which I think is what you're after. See this link: "Least Astonishment" and the Mutable Default Argument to see why.
The problem is that when using your current Team()
initialiser, the players
parameter is not passed with a default value of as you are expecting, instead, the value of
players
is persisted for each team made.
Each team has a reference to the same list, which is why the same list is printed twice.
The reason None
is printed three times is because each time a team is created, 3 more players are added, but your inner for
loop only modifies the names of the first 3 players, leaving the last 3 players untouched.
edited Nov 25 '18 at 2:07
answered Nov 25 '18 at 1:47
0liveradam80liveradam8
606115
606115
That's perfect, but I made the change inside self.teams.append(Team()) that became self.teams.append(Team(player=)). Thanks for the sample and the link, I get all the story, I found this other link helpful too: effbot.org/zone/default-values.htm
– Lorenzo Fiamingo
Nov 25 '18 at 2:22
add a comment |
That's perfect, but I made the change inside self.teams.append(Team()) that became self.teams.append(Team(player=)). Thanks for the sample and the link, I get all the story, I found this other link helpful too: effbot.org/zone/default-values.htm
– Lorenzo Fiamingo
Nov 25 '18 at 2:22
That's perfect, but I made the change inside self.teams.append(Team()) that became self.teams.append(Team(player=)). Thanks for the sample and the link, I get all the story, I found this other link helpful too: effbot.org/zone/default-values.htm
– Lorenzo Fiamingo
Nov 25 '18 at 2:22
That's perfect, but I made the change inside self.teams.append(Team()) that became self.teams.append(Team(player=)). Thanks for the sample and the link, I get all the story, I found this other link helpful too: effbot.org/zone/default-values.htm
– Lorenzo Fiamingo
Nov 25 '18 at 2:22
add a comment |
I agree with 0liveradam8's response and just to add on - if you would like to maintain the constructor signature you could try this:
class Team():
def __init__(self, name=None, players=None):
if players is None:
players =
self.name = name
self.players = players
so you can still specify a list of players in the constructor if necessary.
add a comment |
I agree with 0liveradam8's response and just to add on - if you would like to maintain the constructor signature you could try this:
class Team():
def __init__(self, name=None, players=None):
if players is None:
players =
self.name = name
self.players = players
so you can still specify a list of players in the constructor if necessary.
add a comment |
I agree with 0liveradam8's response and just to add on - if you would like to maintain the constructor signature you could try this:
class Team():
def __init__(self, name=None, players=None):
if players is None:
players =
self.name = name
self.players = players
so you can still specify a list of players in the constructor if necessary.
I agree with 0liveradam8's response and just to add on - if you would like to maintain the constructor signature you could try this:
class Team():
def __init__(self, name=None, players=None):
if players is None:
players =
self.name = name
self.players = players
so you can still specify a list of players in the constructor if necessary.
answered Nov 25 '18 at 5:31
greenBoxgreenBox
191
191
add a comment |
add a comment |
The reason you are getting None is that you haven't returned the value in the functions. Therefore, when you run your script, it is set to nothing. Here's a link to look into it more.
http://interactivepython.org/runestone/static/pip2/Functions/Returningavaluefromafunction.html
The answer to your second question is that the
for a in range(2):
Should be like
for a in range(1):
Hope this helps!
Sure with range(1) works all ok but the problem is that I need to add how much teams I want
– Lorenzo Fiamingo
Nov 25 '18 at 1:58
add a comment |
The reason you are getting None is that you haven't returned the value in the functions. Therefore, when you run your script, it is set to nothing. Here's a link to look into it more.
http://interactivepython.org/runestone/static/pip2/Functions/Returningavaluefromafunction.html
The answer to your second question is that the
for a in range(2):
Should be like
for a in range(1):
Hope this helps!
Sure with range(1) works all ok but the problem is that I need to add how much teams I want
– Lorenzo Fiamingo
Nov 25 '18 at 1:58
add a comment |
The reason you are getting None is that you haven't returned the value in the functions. Therefore, when you run your script, it is set to nothing. Here's a link to look into it more.
http://interactivepython.org/runestone/static/pip2/Functions/Returningavaluefromafunction.html
The answer to your second question is that the
for a in range(2):
Should be like
for a in range(1):
Hope this helps!
The reason you are getting None is that you haven't returned the value in the functions. Therefore, when you run your script, it is set to nothing. Here's a link to look into it more.
http://interactivepython.org/runestone/static/pip2/Functions/Returningavaluefromafunction.html
The answer to your second question is that the
for a in range(2):
Should be like
for a in range(1):
Hope this helps!
edited Nov 25 '18 at 1:52


TeeKea
3,22851832
3,22851832
answered Nov 25 '18 at 1:39


exeexe
13514
13514
Sure with range(1) works all ok but the problem is that I need to add how much teams I want
– Lorenzo Fiamingo
Nov 25 '18 at 1:58
add a comment |
Sure with range(1) works all ok but the problem is that I need to add how much teams I want
– Lorenzo Fiamingo
Nov 25 '18 at 1:58
Sure with range(1) works all ok but the problem is that I need to add how much teams I want
– Lorenzo Fiamingo
Nov 25 '18 at 1:58
Sure with range(1) works all ok but the problem is that I need to add how much teams I want
– Lorenzo Fiamingo
Nov 25 '18 at 1:58
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53463871%2fdeclaring-nested-objects-in-nested-for-loops-python-3%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
eonsuoqhI,9oPu1P8JET
The problem is the
players=
inTeam
constructor. See stackoverflow.com/questions/43255481/…– Michael Butscher
Nov 25 '18 at 1:22