How to set an arbitrary variable as global in Python?
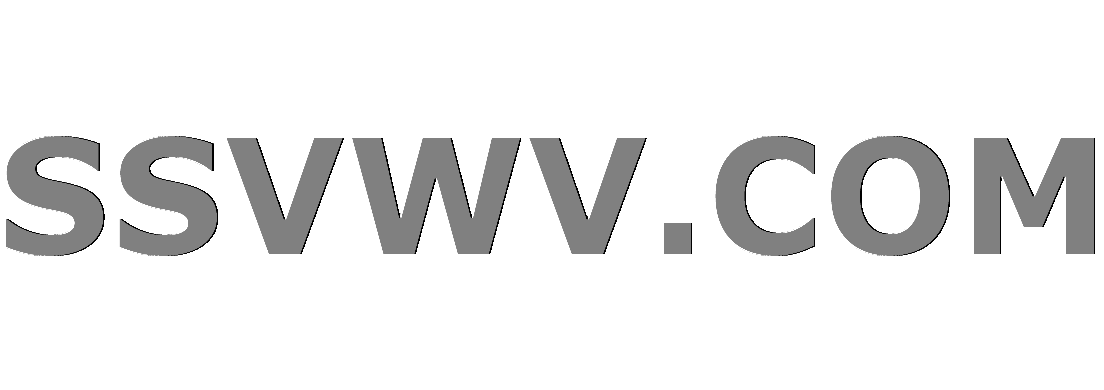
Multi tool use
How do you dynamically set variables as global in Python 3 functions?
Something like this:
def func1(i):
global i
#Some operation on i
How would I get the global variable to set whatever pre-existing variable is passed into the function? Such that:
func1(foo)
Would preform the operation on the variable foo
globally rather than creating a new global variable called i
?
python python-3.x function global-variables
add a comment |
How do you dynamically set variables as global in Python 3 functions?
Something like this:
def func1(i):
global i
#Some operation on i
How would I get the global variable to set whatever pre-existing variable is passed into the function? Such that:
func1(foo)
Would preform the operation on the variable foo
globally rather than creating a new global variable called i
?
python python-3.x function global-variables
2
What are you trying to do with this?foo = func1(foo)
is a much better solution most (all?) cases. There are some horrible hacks with stack frames that I think can do this, but I've made my living for the past 15 years writing Python, and I've never needed them.
– cco
Nov 25 '18 at 1:28
2
When you call a function defined asfunc1(i)
, you are NOT passing a variable to it - you are passing a value. Perhaps that value came from a variable, but there is absolutely no connection from the value to any variable(s) that might have held it.
– jasonharper
Nov 25 '18 at 1:53
Okay, the input being a value instead of the variable itself is exactly what I was hoping against. I essentially want to have: func(#Some Memory Address or variable) to create the output and directly push it to whatever the variable used as input was.
– BenJam_Brady
Nov 28 '18 at 0:30
add a comment |
How do you dynamically set variables as global in Python 3 functions?
Something like this:
def func1(i):
global i
#Some operation on i
How would I get the global variable to set whatever pre-existing variable is passed into the function? Such that:
func1(foo)
Would preform the operation on the variable foo
globally rather than creating a new global variable called i
?
python python-3.x function global-variables
How do you dynamically set variables as global in Python 3 functions?
Something like this:
def func1(i):
global i
#Some operation on i
How would I get the global variable to set whatever pre-existing variable is passed into the function? Such that:
func1(foo)
Would preform the operation on the variable foo
globally rather than creating a new global variable called i
?
python python-3.x function global-variables
python python-3.x function global-variables
edited Nov 28 '18 at 0:34
BenJam_Brady
asked Nov 25 '18 at 1:15
BenJam_BradyBenJam_Brady
11
11
2
What are you trying to do with this?foo = func1(foo)
is a much better solution most (all?) cases. There are some horrible hacks with stack frames that I think can do this, but I've made my living for the past 15 years writing Python, and I've never needed them.
– cco
Nov 25 '18 at 1:28
2
When you call a function defined asfunc1(i)
, you are NOT passing a variable to it - you are passing a value. Perhaps that value came from a variable, but there is absolutely no connection from the value to any variable(s) that might have held it.
– jasonharper
Nov 25 '18 at 1:53
Okay, the input being a value instead of the variable itself is exactly what I was hoping against. I essentially want to have: func(#Some Memory Address or variable) to create the output and directly push it to whatever the variable used as input was.
– BenJam_Brady
Nov 28 '18 at 0:30
add a comment |
2
What are you trying to do with this?foo = func1(foo)
is a much better solution most (all?) cases. There are some horrible hacks with stack frames that I think can do this, but I've made my living for the past 15 years writing Python, and I've never needed them.
– cco
Nov 25 '18 at 1:28
2
When you call a function defined asfunc1(i)
, you are NOT passing a variable to it - you are passing a value. Perhaps that value came from a variable, but there is absolutely no connection from the value to any variable(s) that might have held it.
– jasonharper
Nov 25 '18 at 1:53
Okay, the input being a value instead of the variable itself is exactly what I was hoping against. I essentially want to have: func(#Some Memory Address or variable) to create the output and directly push it to whatever the variable used as input was.
– BenJam_Brady
Nov 28 '18 at 0:30
2
2
What are you trying to do with this?
foo = func1(foo)
is a much better solution most (all?) cases. There are some horrible hacks with stack frames that I think can do this, but I've made my living for the past 15 years writing Python, and I've never needed them.– cco
Nov 25 '18 at 1:28
What are you trying to do with this?
foo = func1(foo)
is a much better solution most (all?) cases. There are some horrible hacks with stack frames that I think can do this, but I've made my living for the past 15 years writing Python, and I've never needed them.– cco
Nov 25 '18 at 1:28
2
2
When you call a function defined as
func1(i)
, you are NOT passing a variable to it - you are passing a value. Perhaps that value came from a variable, but there is absolutely no connection from the value to any variable(s) that might have held it.– jasonharper
Nov 25 '18 at 1:53
When you call a function defined as
func1(i)
, you are NOT passing a variable to it - you are passing a value. Perhaps that value came from a variable, but there is absolutely no connection from the value to any variable(s) that might have held it.– jasonharper
Nov 25 '18 at 1:53
Okay, the input being a value instead of the variable itself is exactly what I was hoping against. I essentially want to have: func(#Some Memory Address or variable) to create the output and directly push it to whatever the variable used as input was.
– BenJam_Brady
Nov 28 '18 at 0:30
Okay, the input being a value instead of the variable itself is exactly what I was hoping against. I essentially want to have: func(#Some Memory Address or variable) to create the output and directly push it to whatever the variable used as input was.
– BenJam_Brady
Nov 28 '18 at 0:30
add a comment |
1 Answer
1
active
oldest
votes
If I got it right, your problem is:
I've bound a name to some object at module level; now I want to write a function that changes the binding of that global name to another object passed as an argument to the function.
In Python global names can be referenced inside a function (provided that you don't bind another object to the same name), but to change their binding you must first declare those names as global
. The reason is simple: by default all names bound inside a function have function scope.
GLOBAL_NAME = 12
ANOTHER_GLOBAL_NAME = 50
def func(value):
global GLOBAL_NAME
GLOBAL_NAME = value
print(GLOBAL_NAME, ANOTHER_GLOBAL_NAME)
When you call func(33)
, GLOBAL_NAME
will be bound to the object 33
. ANOTHER_GLOBAL_NAME
is just a reference to a global name; since that name is not bound inside the function, Python look for that name in the global scope.
For further insights on Python scope enclosing you can refer to the Python documentation on the global statement, to PEP-0227 and to PEP-3104.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53463867%2fhow-to-set-an-arbitrary-variable-as-global-in-python%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
If I got it right, your problem is:
I've bound a name to some object at module level; now I want to write a function that changes the binding of that global name to another object passed as an argument to the function.
In Python global names can be referenced inside a function (provided that you don't bind another object to the same name), but to change their binding you must first declare those names as global
. The reason is simple: by default all names bound inside a function have function scope.
GLOBAL_NAME = 12
ANOTHER_GLOBAL_NAME = 50
def func(value):
global GLOBAL_NAME
GLOBAL_NAME = value
print(GLOBAL_NAME, ANOTHER_GLOBAL_NAME)
When you call func(33)
, GLOBAL_NAME
will be bound to the object 33
. ANOTHER_GLOBAL_NAME
is just a reference to a global name; since that name is not bound inside the function, Python look for that name in the global scope.
For further insights on Python scope enclosing you can refer to the Python documentation on the global statement, to PEP-0227 and to PEP-3104.
add a comment |
If I got it right, your problem is:
I've bound a name to some object at module level; now I want to write a function that changes the binding of that global name to another object passed as an argument to the function.
In Python global names can be referenced inside a function (provided that you don't bind another object to the same name), but to change their binding you must first declare those names as global
. The reason is simple: by default all names bound inside a function have function scope.
GLOBAL_NAME = 12
ANOTHER_GLOBAL_NAME = 50
def func(value):
global GLOBAL_NAME
GLOBAL_NAME = value
print(GLOBAL_NAME, ANOTHER_GLOBAL_NAME)
When you call func(33)
, GLOBAL_NAME
will be bound to the object 33
. ANOTHER_GLOBAL_NAME
is just a reference to a global name; since that name is not bound inside the function, Python look for that name in the global scope.
For further insights on Python scope enclosing you can refer to the Python documentation on the global statement, to PEP-0227 and to PEP-3104.
add a comment |
If I got it right, your problem is:
I've bound a name to some object at module level; now I want to write a function that changes the binding of that global name to another object passed as an argument to the function.
In Python global names can be referenced inside a function (provided that you don't bind another object to the same name), but to change their binding you must first declare those names as global
. The reason is simple: by default all names bound inside a function have function scope.
GLOBAL_NAME = 12
ANOTHER_GLOBAL_NAME = 50
def func(value):
global GLOBAL_NAME
GLOBAL_NAME = value
print(GLOBAL_NAME, ANOTHER_GLOBAL_NAME)
When you call func(33)
, GLOBAL_NAME
will be bound to the object 33
. ANOTHER_GLOBAL_NAME
is just a reference to a global name; since that name is not bound inside the function, Python look for that name in the global scope.
For further insights on Python scope enclosing you can refer to the Python documentation on the global statement, to PEP-0227 and to PEP-3104.
If I got it right, your problem is:
I've bound a name to some object at module level; now I want to write a function that changes the binding of that global name to another object passed as an argument to the function.
In Python global names can be referenced inside a function (provided that you don't bind another object to the same name), but to change their binding you must first declare those names as global
. The reason is simple: by default all names bound inside a function have function scope.
GLOBAL_NAME = 12
ANOTHER_GLOBAL_NAME = 50
def func(value):
global GLOBAL_NAME
GLOBAL_NAME = value
print(GLOBAL_NAME, ANOTHER_GLOBAL_NAME)
When you call func(33)
, GLOBAL_NAME
will be bound to the object 33
. ANOTHER_GLOBAL_NAME
is just a reference to a global name; since that name is not bound inside the function, Python look for that name in the global scope.
For further insights on Python scope enclosing you can refer to the Python documentation on the global statement, to PEP-0227 and to PEP-3104.
edited Nov 25 '18 at 3:16
answered Nov 25 '18 at 3:08
A. SematA. Semat
584
584
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53463867%2fhow-to-set-an-arbitrary-variable-as-global-in-python%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
eO ECiaNE6BhjNF BNQHdEFCOr5g,m,ESqqmJyHHR,zTpqzE5s,X
2
What are you trying to do with this?
foo = func1(foo)
is a much better solution most (all?) cases. There are some horrible hacks with stack frames that I think can do this, but I've made my living for the past 15 years writing Python, and I've never needed them.– cco
Nov 25 '18 at 1:28
2
When you call a function defined as
func1(i)
, you are NOT passing a variable to it - you are passing a value. Perhaps that value came from a variable, but there is absolutely no connection from the value to any variable(s) that might have held it.– jasonharper
Nov 25 '18 at 1:53
Okay, the input being a value instead of the variable itself is exactly what I was hoping against. I essentially want to have: func(#Some Memory Address or variable) to create the output and directly push it to whatever the variable used as input was.
– BenJam_Brady
Nov 28 '18 at 0:30