Modules and submodules files fully asynchronous loading
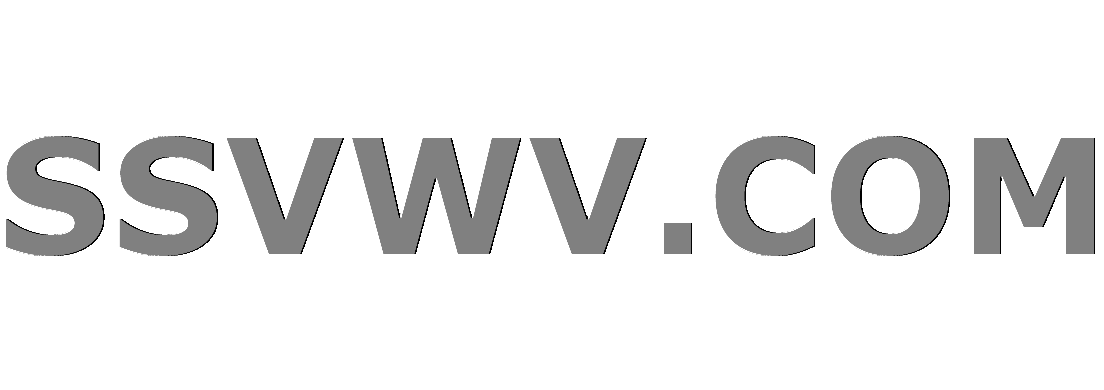
Multi tool use
I would like to have a fully asynchronous method for loading modules' files on the client side, without the need of extra tools, such as requireJS. My module template follows the "Revealing module pattern" template.
In my file root.js
I have
root = (function(thisModule){
//I'm stuck here, I know I need to add the already
//existent properties of thisModule into here
//when child.js is loaded first
function A(){...}
function B(){...} //public method
return{
B:B
};
})(root || {});
In my file child.js
I have
root = root || {};
root.child = (function(){
function C(){...}
function D(){...} //public methods
return{
D:D
};
})();
How do I rewrite the first module in root.js
such that the files' loading order is irrelevant? That is, the following code will never throw an exception.
$.when($.getScript("root.js"), $.getScript("child.js")).done(function(){
root.B;
root.child.D;
});
jquery performance asynchronous module getscript
|
show 2 more comments
I would like to have a fully asynchronous method for loading modules' files on the client side, without the need of extra tools, such as requireJS. My module template follows the "Revealing module pattern" template.
In my file root.js
I have
root = (function(thisModule){
//I'm stuck here, I know I need to add the already
//existent properties of thisModule into here
//when child.js is loaded first
function A(){...}
function B(){...} //public method
return{
B:B
};
})(root || {});
In my file child.js
I have
root = root || {};
root.child = (function(){
function C(){...}
function D(){...} //public methods
return{
D:D
};
})();
How do I rewrite the first module in root.js
such that the files' loading order is irrelevant? That is, the following code will never throw an exception.
$.when($.getScript("root.js"), $.getScript("child.js")).done(function(){
root.B;
root.child.D;
});
jquery performance asynchronous module getscript
So you want to be able to accessroot.B
androot.child.D
after both scripts are loaded, is that it?
– CertainPerformance
Nov 25 '18 at 1:22
I think promises are the way to go here. But you say you don't want to do that. Are you okay with the idea of polling until you see it load? Why the opposition to promises?
– mccambridge
Nov 25 '18 at 1:27
@mccambridge do you mean promises in thegetScript
? I want the loading of files to be fully async.
– João Pimentel Ferreira
Nov 25 '18 at 10:08
@CertainPerformance, yes, and independently of file loading order. Is it possible?
– João Pimentel Ferreira
Nov 25 '18 at 10:09
@CertainPerformance my problem is that whenchild.js
loads first,root.child
gets erased by the loading ofroot.js
– João Pimentel Ferreira
Nov 25 '18 at 10:20
|
show 2 more comments
I would like to have a fully asynchronous method for loading modules' files on the client side, without the need of extra tools, such as requireJS. My module template follows the "Revealing module pattern" template.
In my file root.js
I have
root = (function(thisModule){
//I'm stuck here, I know I need to add the already
//existent properties of thisModule into here
//when child.js is loaded first
function A(){...}
function B(){...} //public method
return{
B:B
};
})(root || {});
In my file child.js
I have
root = root || {};
root.child = (function(){
function C(){...}
function D(){...} //public methods
return{
D:D
};
})();
How do I rewrite the first module in root.js
such that the files' loading order is irrelevant? That is, the following code will never throw an exception.
$.when($.getScript("root.js"), $.getScript("child.js")).done(function(){
root.B;
root.child.D;
});
jquery performance asynchronous module getscript
I would like to have a fully asynchronous method for loading modules' files on the client side, without the need of extra tools, such as requireJS. My module template follows the "Revealing module pattern" template.
In my file root.js
I have
root = (function(thisModule){
//I'm stuck here, I know I need to add the already
//existent properties of thisModule into here
//when child.js is loaded first
function A(){...}
function B(){...} //public method
return{
B:B
};
})(root || {});
In my file child.js
I have
root = root || {};
root.child = (function(){
function C(){...}
function D(){...} //public methods
return{
D:D
};
})();
How do I rewrite the first module in root.js
such that the files' loading order is irrelevant? That is, the following code will never throw an exception.
$.when($.getScript("root.js"), $.getScript("child.js")).done(function(){
root.B;
root.child.D;
});
jquery performance asynchronous module getscript
jquery performance asynchronous module getscript
edited Nov 26 '18 at 12:01
João Pimentel Ferreira
asked Nov 25 '18 at 1:18
João Pimentel FerreiraJoão Pimentel Ferreira
4,07322330
4,07322330
So you want to be able to accessroot.B
androot.child.D
after both scripts are loaded, is that it?
– CertainPerformance
Nov 25 '18 at 1:22
I think promises are the way to go here. But you say you don't want to do that. Are you okay with the idea of polling until you see it load? Why the opposition to promises?
– mccambridge
Nov 25 '18 at 1:27
@mccambridge do you mean promises in thegetScript
? I want the loading of files to be fully async.
– João Pimentel Ferreira
Nov 25 '18 at 10:08
@CertainPerformance, yes, and independently of file loading order. Is it possible?
– João Pimentel Ferreira
Nov 25 '18 at 10:09
@CertainPerformance my problem is that whenchild.js
loads first,root.child
gets erased by the loading ofroot.js
– João Pimentel Ferreira
Nov 25 '18 at 10:20
|
show 2 more comments
So you want to be able to accessroot.B
androot.child.D
after both scripts are loaded, is that it?
– CertainPerformance
Nov 25 '18 at 1:22
I think promises are the way to go here. But you say you don't want to do that. Are you okay with the idea of polling until you see it load? Why the opposition to promises?
– mccambridge
Nov 25 '18 at 1:27
@mccambridge do you mean promises in thegetScript
? I want the loading of files to be fully async.
– João Pimentel Ferreira
Nov 25 '18 at 10:08
@CertainPerformance, yes, and independently of file loading order. Is it possible?
– João Pimentel Ferreira
Nov 25 '18 at 10:09
@CertainPerformance my problem is that whenchild.js
loads first,root.child
gets erased by the loading ofroot.js
– João Pimentel Ferreira
Nov 25 '18 at 10:20
So you want to be able to access
root.B
and root.child.D
after both scripts are loaded, is that it?– CertainPerformance
Nov 25 '18 at 1:22
So you want to be able to access
root.B
and root.child.D
after both scripts are loaded, is that it?– CertainPerformance
Nov 25 '18 at 1:22
I think promises are the way to go here. But you say you don't want to do that. Are you okay with the idea of polling until you see it load? Why the opposition to promises?
– mccambridge
Nov 25 '18 at 1:27
I think promises are the way to go here. But you say you don't want to do that. Are you okay with the idea of polling until you see it load? Why the opposition to promises?
– mccambridge
Nov 25 '18 at 1:27
@mccambridge do you mean promises in the
getScript
? I want the loading of files to be fully async.– João Pimentel Ferreira
Nov 25 '18 at 10:08
@mccambridge do you mean promises in the
getScript
? I want the loading of files to be fully async.– João Pimentel Ferreira
Nov 25 '18 at 10:08
@CertainPerformance, yes, and independently of file loading order. Is it possible?
– João Pimentel Ferreira
Nov 25 '18 at 10:09
@CertainPerformance, yes, and independently of file loading order. Is it possible?
– João Pimentel Ferreira
Nov 25 '18 at 10:09
@CertainPerformance my problem is that when
child.js
loads first, root.child
gets erased by the loading of root.js
– João Pimentel Ferreira
Nov 25 '18 at 10:20
@CertainPerformance my problem is that when
child.js
loads first, root.child
gets erased by the loading of root.js
– João Pimentel Ferreira
Nov 25 '18 at 10:20
|
show 2 more comments
1 Answer
1
active
oldest
votes
The simplest tweak to your code would be to preserve the contents of thisModule
- just assign to the B
property of thisModule
and then return thisModule
instead of returning only { B:B }
:
var root = (function(thisModule){
function A(){ }
function B(){ } //public method
thisModule.B = B;
return thisModule;
})(root || {});
If root
is meant to be global, then it might be a bit clearer if you explicitly refer to window.root
, otherwise you might encounter bugs if you accidentally put that snippet somewhere other than at the top level:
window.root = (function(thisModule){ ...
As a side note, assuming your build process uses Babel (which any serious project should), you can consider using shorthand properties to reduce syntax noise, eg:
return{ D };
rather than
return{ D: D };
which could be helpful if the method names are long - less syntax noise.
thanks a lot, really! My project usesuglify-js
to compact the js code.
– João Pimentel Ferreira
Nov 25 '18 at 12:03
I rewrote my question to clarify exactly what was my point, I hope it is ok for you. Anyway you have already given the solution.
– João Pimentel Ferreira
Nov 26 '18 at 11:56
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53463885%2fmodules-and-submodules-files-fully-asynchronous-loading%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
The simplest tweak to your code would be to preserve the contents of thisModule
- just assign to the B
property of thisModule
and then return thisModule
instead of returning only { B:B }
:
var root = (function(thisModule){
function A(){ }
function B(){ } //public method
thisModule.B = B;
return thisModule;
})(root || {});
If root
is meant to be global, then it might be a bit clearer if you explicitly refer to window.root
, otherwise you might encounter bugs if you accidentally put that snippet somewhere other than at the top level:
window.root = (function(thisModule){ ...
As a side note, assuming your build process uses Babel (which any serious project should), you can consider using shorthand properties to reduce syntax noise, eg:
return{ D };
rather than
return{ D: D };
which could be helpful if the method names are long - less syntax noise.
thanks a lot, really! My project usesuglify-js
to compact the js code.
– João Pimentel Ferreira
Nov 25 '18 at 12:03
I rewrote my question to clarify exactly what was my point, I hope it is ok for you. Anyway you have already given the solution.
– João Pimentel Ferreira
Nov 26 '18 at 11:56
add a comment |
The simplest tweak to your code would be to preserve the contents of thisModule
- just assign to the B
property of thisModule
and then return thisModule
instead of returning only { B:B }
:
var root = (function(thisModule){
function A(){ }
function B(){ } //public method
thisModule.B = B;
return thisModule;
})(root || {});
If root
is meant to be global, then it might be a bit clearer if you explicitly refer to window.root
, otherwise you might encounter bugs if you accidentally put that snippet somewhere other than at the top level:
window.root = (function(thisModule){ ...
As a side note, assuming your build process uses Babel (which any serious project should), you can consider using shorthand properties to reduce syntax noise, eg:
return{ D };
rather than
return{ D: D };
which could be helpful if the method names are long - less syntax noise.
thanks a lot, really! My project usesuglify-js
to compact the js code.
– João Pimentel Ferreira
Nov 25 '18 at 12:03
I rewrote my question to clarify exactly what was my point, I hope it is ok for you. Anyway you have already given the solution.
– João Pimentel Ferreira
Nov 26 '18 at 11:56
add a comment |
The simplest tweak to your code would be to preserve the contents of thisModule
- just assign to the B
property of thisModule
and then return thisModule
instead of returning only { B:B }
:
var root = (function(thisModule){
function A(){ }
function B(){ } //public method
thisModule.B = B;
return thisModule;
})(root || {});
If root
is meant to be global, then it might be a bit clearer if you explicitly refer to window.root
, otherwise you might encounter bugs if you accidentally put that snippet somewhere other than at the top level:
window.root = (function(thisModule){ ...
As a side note, assuming your build process uses Babel (which any serious project should), you can consider using shorthand properties to reduce syntax noise, eg:
return{ D };
rather than
return{ D: D };
which could be helpful if the method names are long - less syntax noise.
The simplest tweak to your code would be to preserve the contents of thisModule
- just assign to the B
property of thisModule
and then return thisModule
instead of returning only { B:B }
:
var root = (function(thisModule){
function A(){ }
function B(){ } //public method
thisModule.B = B;
return thisModule;
})(root || {});
If root
is meant to be global, then it might be a bit clearer if you explicitly refer to window.root
, otherwise you might encounter bugs if you accidentally put that snippet somewhere other than at the top level:
window.root = (function(thisModule){ ...
As a side note, assuming your build process uses Babel (which any serious project should), you can consider using shorthand properties to reduce syntax noise, eg:
return{ D };
rather than
return{ D: D };
which could be helpful if the method names are long - less syntax noise.
edited Nov 25 '18 at 11:05
answered Nov 25 '18 at 10:59
CertainPerformanceCertainPerformance
91.6k165380
91.6k165380
thanks a lot, really! My project usesuglify-js
to compact the js code.
– João Pimentel Ferreira
Nov 25 '18 at 12:03
I rewrote my question to clarify exactly what was my point, I hope it is ok for you. Anyway you have already given the solution.
– João Pimentel Ferreira
Nov 26 '18 at 11:56
add a comment |
thanks a lot, really! My project usesuglify-js
to compact the js code.
– João Pimentel Ferreira
Nov 25 '18 at 12:03
I rewrote my question to clarify exactly what was my point, I hope it is ok for you. Anyway you have already given the solution.
– João Pimentel Ferreira
Nov 26 '18 at 11:56
thanks a lot, really! My project uses
uglify-js
to compact the js code.– João Pimentel Ferreira
Nov 25 '18 at 12:03
thanks a lot, really! My project uses
uglify-js
to compact the js code.– João Pimentel Ferreira
Nov 25 '18 at 12:03
I rewrote my question to clarify exactly what was my point, I hope it is ok for you. Anyway you have already given the solution.
– João Pimentel Ferreira
Nov 26 '18 at 11:56
I rewrote my question to clarify exactly what was my point, I hope it is ok for you. Anyway you have already given the solution.
– João Pimentel Ferreira
Nov 26 '18 at 11:56
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53463885%2fmodules-and-submodules-files-fully-asynchronous-loading%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
ylxSln,r4KBrSlkCFSwu20Q3bKYTqSoNQL3t8YLl9bp773NM8OmABVp MO Qz9K
So you want to be able to access
root.B
androot.child.D
after both scripts are loaded, is that it?– CertainPerformance
Nov 25 '18 at 1:22
I think promises are the way to go here. But you say you don't want to do that. Are you okay with the idea of polling until you see it load? Why the opposition to promises?
– mccambridge
Nov 25 '18 at 1:27
@mccambridge do you mean promises in the
getScript
? I want the loading of files to be fully async.– João Pimentel Ferreira
Nov 25 '18 at 10:08
@CertainPerformance, yes, and independently of file loading order. Is it possible?
– João Pimentel Ferreira
Nov 25 '18 at 10:09
@CertainPerformance my problem is that when
child.js
loads first,root.child
gets erased by the loading ofroot.js
– João Pimentel Ferreira
Nov 25 '18 at 10:20