Deserealizing JSON object using Newtonsoft.Json
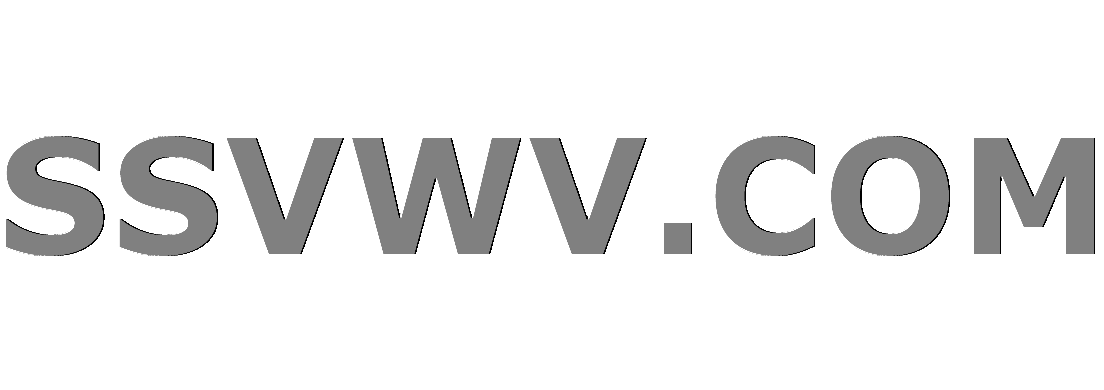
Multi tool use
I have API endpoint, that returning my JSON Object
Here is it
{
"results": [
{
"id": 182,
"title": "1-Day Private Beijing Tour to Tian'anmen Square, Forbidden City and Badaling Great Wall",
"price": "162",
"duration": "8",
"duration_type": "1",
"cover_image": {
"id": 308,
"img_path": "upload/images",
"img_file": "6d637884086151b30fe12db52fbaf5eb.jpg",
"status": "",
"created_at": "2018-02-27 02:25:36",
"updated_at": "2018-02-27 02:25:36",
"destination_id": "182",
"is_cover": "0",
"url": "https://api.xplorpal.com/upload/images/300x300/6d637884086151b30fe12db52fbaf5eb.jpg"
}
},
{
"id": 183,
"title": "One Day Private Beijing Tour to Mutianyu Great Wall and Summer Palace ",
"price": "197",
"duration": "8",
"duration_type": "1",
"cover_image": {
"id": 305,
"img_path": "upload/images",
"img_file": "1f8a09ddffb80ef9232f3511893ae5c4.jpg",
"status": "",
"created_at": "2018-02-27 02:22:19",
"updated_at": "2018-03-01 23:01:55",
"destination_id": "183",
"is_cover": "0",
"url": "https://api.xplorpal.com/upload/images/300x300/1f8a09ddffb80ef9232f3511893ae5c4.jpg"
}
}
]
}
I need to deserialize it
So I wrote this model
public class CoverImage
{
public int id { get; set; }
public string img_path { get; set; }
public string img_file { get; set; }
public string status { get; set; }
public string created_at { get; set; }
public string updated_at { get; set; }
public string destination_id { get; set; }
public string is_cover { get; set; }
public string url { get; set; }
}
public class Result
{
public int id { get; set; }
public string title { get; set; }
public string price { get; set; }
public string duration { get; set; }
public string duration_type { get; set; }
public CoverImage cover_image { get; set; }
}
public class RootObject
{
public List<Result> results { get; set; }
}
And trying to this like this
var responseExperiences = JsonConvert.DeserializeObject<IEnumerable<RootObject>>(content);
But when I run the project, I have this error:
Cannot deserialize the current JSON object (e.g. {"name":"value"}) into type 'System.Collections.Generic.IEnumerable`1[TravelApp.Models.GettingExperiences+Results]' because the type requires a JSON array (e.g. [1,2,3]) to deserialize correctly.
To fix this error either change the JSON to a JSON array (e.g. [1,2,3]) or change the deserialized type so that it is a normal .NET type (e.g. not a primitive type like integer, not a collection type like an array or List) that can be deserialized from a JSON object. JsonObjectAttribute can also be added to the type to force it to deserialize from a JSON object.
How I can fix this?
c# .net json json.net
add a comment |
I have API endpoint, that returning my JSON Object
Here is it
{
"results": [
{
"id": 182,
"title": "1-Day Private Beijing Tour to Tian'anmen Square, Forbidden City and Badaling Great Wall",
"price": "162",
"duration": "8",
"duration_type": "1",
"cover_image": {
"id": 308,
"img_path": "upload/images",
"img_file": "6d637884086151b30fe12db52fbaf5eb.jpg",
"status": "",
"created_at": "2018-02-27 02:25:36",
"updated_at": "2018-02-27 02:25:36",
"destination_id": "182",
"is_cover": "0",
"url": "https://api.xplorpal.com/upload/images/300x300/6d637884086151b30fe12db52fbaf5eb.jpg"
}
},
{
"id": 183,
"title": "One Day Private Beijing Tour to Mutianyu Great Wall and Summer Palace ",
"price": "197",
"duration": "8",
"duration_type": "1",
"cover_image": {
"id": 305,
"img_path": "upload/images",
"img_file": "1f8a09ddffb80ef9232f3511893ae5c4.jpg",
"status": "",
"created_at": "2018-02-27 02:22:19",
"updated_at": "2018-03-01 23:01:55",
"destination_id": "183",
"is_cover": "0",
"url": "https://api.xplorpal.com/upload/images/300x300/1f8a09ddffb80ef9232f3511893ae5c4.jpg"
}
}
]
}
I need to deserialize it
So I wrote this model
public class CoverImage
{
public int id { get; set; }
public string img_path { get; set; }
public string img_file { get; set; }
public string status { get; set; }
public string created_at { get; set; }
public string updated_at { get; set; }
public string destination_id { get; set; }
public string is_cover { get; set; }
public string url { get; set; }
}
public class Result
{
public int id { get; set; }
public string title { get; set; }
public string price { get; set; }
public string duration { get; set; }
public string duration_type { get; set; }
public CoverImage cover_image { get; set; }
}
public class RootObject
{
public List<Result> results { get; set; }
}
And trying to this like this
var responseExperiences = JsonConvert.DeserializeObject<IEnumerable<RootObject>>(content);
But when I run the project, I have this error:
Cannot deserialize the current JSON object (e.g. {"name":"value"}) into type 'System.Collections.Generic.IEnumerable`1[TravelApp.Models.GettingExperiences+Results]' because the type requires a JSON array (e.g. [1,2,3]) to deserialize correctly.
To fix this error either change the JSON to a JSON array (e.g. [1,2,3]) or change the deserialized type so that it is a normal .NET type (e.g. not a primitive type like integer, not a collection type like an array or List) that can be deserialized from a JSON object. JsonObjectAttribute can also be added to the type to force it to deserialize from a JSON object.
How I can fix this?
c# .net json json.net
add a comment |
I have API endpoint, that returning my JSON Object
Here is it
{
"results": [
{
"id": 182,
"title": "1-Day Private Beijing Tour to Tian'anmen Square, Forbidden City and Badaling Great Wall",
"price": "162",
"duration": "8",
"duration_type": "1",
"cover_image": {
"id": 308,
"img_path": "upload/images",
"img_file": "6d637884086151b30fe12db52fbaf5eb.jpg",
"status": "",
"created_at": "2018-02-27 02:25:36",
"updated_at": "2018-02-27 02:25:36",
"destination_id": "182",
"is_cover": "0",
"url": "https://api.xplorpal.com/upload/images/300x300/6d637884086151b30fe12db52fbaf5eb.jpg"
}
},
{
"id": 183,
"title": "One Day Private Beijing Tour to Mutianyu Great Wall and Summer Palace ",
"price": "197",
"duration": "8",
"duration_type": "1",
"cover_image": {
"id": 305,
"img_path": "upload/images",
"img_file": "1f8a09ddffb80ef9232f3511893ae5c4.jpg",
"status": "",
"created_at": "2018-02-27 02:22:19",
"updated_at": "2018-03-01 23:01:55",
"destination_id": "183",
"is_cover": "0",
"url": "https://api.xplorpal.com/upload/images/300x300/1f8a09ddffb80ef9232f3511893ae5c4.jpg"
}
}
]
}
I need to deserialize it
So I wrote this model
public class CoverImage
{
public int id { get; set; }
public string img_path { get; set; }
public string img_file { get; set; }
public string status { get; set; }
public string created_at { get; set; }
public string updated_at { get; set; }
public string destination_id { get; set; }
public string is_cover { get; set; }
public string url { get; set; }
}
public class Result
{
public int id { get; set; }
public string title { get; set; }
public string price { get; set; }
public string duration { get; set; }
public string duration_type { get; set; }
public CoverImage cover_image { get; set; }
}
public class RootObject
{
public List<Result> results { get; set; }
}
And trying to this like this
var responseExperiences = JsonConvert.DeserializeObject<IEnumerable<RootObject>>(content);
But when I run the project, I have this error:
Cannot deserialize the current JSON object (e.g. {"name":"value"}) into type 'System.Collections.Generic.IEnumerable`1[TravelApp.Models.GettingExperiences+Results]' because the type requires a JSON array (e.g. [1,2,3]) to deserialize correctly.
To fix this error either change the JSON to a JSON array (e.g. [1,2,3]) or change the deserialized type so that it is a normal .NET type (e.g. not a primitive type like integer, not a collection type like an array or List) that can be deserialized from a JSON object. JsonObjectAttribute can also be added to the type to force it to deserialize from a JSON object.
How I can fix this?
c# .net json json.net
I have API endpoint, that returning my JSON Object
Here is it
{
"results": [
{
"id": 182,
"title": "1-Day Private Beijing Tour to Tian'anmen Square, Forbidden City and Badaling Great Wall",
"price": "162",
"duration": "8",
"duration_type": "1",
"cover_image": {
"id": 308,
"img_path": "upload/images",
"img_file": "6d637884086151b30fe12db52fbaf5eb.jpg",
"status": "",
"created_at": "2018-02-27 02:25:36",
"updated_at": "2018-02-27 02:25:36",
"destination_id": "182",
"is_cover": "0",
"url": "https://api.xplorpal.com/upload/images/300x300/6d637884086151b30fe12db52fbaf5eb.jpg"
}
},
{
"id": 183,
"title": "One Day Private Beijing Tour to Mutianyu Great Wall and Summer Palace ",
"price": "197",
"duration": "8",
"duration_type": "1",
"cover_image": {
"id": 305,
"img_path": "upload/images",
"img_file": "1f8a09ddffb80ef9232f3511893ae5c4.jpg",
"status": "",
"created_at": "2018-02-27 02:22:19",
"updated_at": "2018-03-01 23:01:55",
"destination_id": "183",
"is_cover": "0",
"url": "https://api.xplorpal.com/upload/images/300x300/1f8a09ddffb80ef9232f3511893ae5c4.jpg"
}
}
]
}
I need to deserialize it
So I wrote this model
public class CoverImage
{
public int id { get; set; }
public string img_path { get; set; }
public string img_file { get; set; }
public string status { get; set; }
public string created_at { get; set; }
public string updated_at { get; set; }
public string destination_id { get; set; }
public string is_cover { get; set; }
public string url { get; set; }
}
public class Result
{
public int id { get; set; }
public string title { get; set; }
public string price { get; set; }
public string duration { get; set; }
public string duration_type { get; set; }
public CoverImage cover_image { get; set; }
}
public class RootObject
{
public List<Result> results { get; set; }
}
And trying to this like this
var responseExperiences = JsonConvert.DeserializeObject<IEnumerable<RootObject>>(content);
But when I run the project, I have this error:
Cannot deserialize the current JSON object (e.g. {"name":"value"}) into type 'System.Collections.Generic.IEnumerable`1[TravelApp.Models.GettingExperiences+Results]' because the type requires a JSON array (e.g. [1,2,3]) to deserialize correctly.
To fix this error either change the JSON to a JSON array (e.g. [1,2,3]) or change the deserialized type so that it is a normal .NET type (e.g. not a primitive type like integer, not a collection type like an array or List) that can be deserialized from a JSON object. JsonObjectAttribute can also be added to the type to force it to deserialize from a JSON object.
How I can fix this?
c# .net json json.net
c# .net json json.net
asked Nov 25 '18 at 12:20


Eugene SukhEugene Sukh
403112
403112
add a comment |
add a comment |
3 Answers
3
active
oldest
votes
your JSON shows one object result
corresponding to RootObject
.
But you are trying to deserialize an array (IEnumerable<>
) of RootObject
You should use this to deserialiaze the JSON showed :
JsonConvert.DeserializeObject<RootObject>(content);
Yeah, it fixed issue. Thanks
– Eugene Sukh
Nov 25 '18 at 12:30
add a comment |
Your API returns an object with a single property named result
, not a collection. You should deserialize into a RootObject
object.
add a comment |
Your api return single object named result not a collection you simply need to desearilize as a single object like.
var responseExperiences = JsonConvert.DeserializeObject<RootObject>(content);
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53467372%2fdeserealizing-json-object-using-newtonsoft-json%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
your JSON shows one object result
corresponding to RootObject
.
But you are trying to deserialize an array (IEnumerable<>
) of RootObject
You should use this to deserialiaze the JSON showed :
JsonConvert.DeserializeObject<RootObject>(content);
Yeah, it fixed issue. Thanks
– Eugene Sukh
Nov 25 '18 at 12:30
add a comment |
your JSON shows one object result
corresponding to RootObject
.
But you are trying to deserialize an array (IEnumerable<>
) of RootObject
You should use this to deserialiaze the JSON showed :
JsonConvert.DeserializeObject<RootObject>(content);
Yeah, it fixed issue. Thanks
– Eugene Sukh
Nov 25 '18 at 12:30
add a comment |
your JSON shows one object result
corresponding to RootObject
.
But you are trying to deserialize an array (IEnumerable<>
) of RootObject
You should use this to deserialiaze the JSON showed :
JsonConvert.DeserializeObject<RootObject>(content);
your JSON shows one object result
corresponding to RootObject
.
But you are trying to deserialize an array (IEnumerable<>
) of RootObject
You should use this to deserialiaze the JSON showed :
JsonConvert.DeserializeObject<RootObject>(content);
edited Nov 25 '18 at 12:39
answered Nov 25 '18 at 12:24
Pac0Pac0
8,21622848
8,21622848
Yeah, it fixed issue. Thanks
– Eugene Sukh
Nov 25 '18 at 12:30
add a comment |
Yeah, it fixed issue. Thanks
– Eugene Sukh
Nov 25 '18 at 12:30
Yeah, it fixed issue. Thanks
– Eugene Sukh
Nov 25 '18 at 12:30
Yeah, it fixed issue. Thanks
– Eugene Sukh
Nov 25 '18 at 12:30
add a comment |
Your API returns an object with a single property named result
, not a collection. You should deserialize into a RootObject
object.
add a comment |
Your API returns an object with a single property named result
, not a collection. You should deserialize into a RootObject
object.
add a comment |
Your API returns an object with a single property named result
, not a collection. You should deserialize into a RootObject
object.
Your API returns an object with a single property named result
, not a collection. You should deserialize into a RootObject
object.
answered Nov 25 '18 at 12:23


Tsahi AsherTsahi Asher
1,190922
1,190922
add a comment |
add a comment |
Your api return single object named result not a collection you simply need to desearilize as a single object like.
var responseExperiences = JsonConvert.DeserializeObject<RootObject>(content);
add a comment |
Your api return single object named result not a collection you simply need to desearilize as a single object like.
var responseExperiences = JsonConvert.DeserializeObject<RootObject>(content);
add a comment |
Your api return single object named result not a collection you simply need to desearilize as a single object like.
var responseExperiences = JsonConvert.DeserializeObject<RootObject>(content);
Your api return single object named result not a collection you simply need to desearilize as a single object like.
var responseExperiences = JsonConvert.DeserializeObject<RootObject>(content);
answered Nov 25 '18 at 12:26


Hamza HaiderHamza Haider
664416
664416
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53467372%2fdeserealizing-json-object-using-newtonsoft-json%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
npGT Uyezx1dvdq rQIC4,5qy I7 SEOp9GHg63 HwCYCt4zgj5ETftEIFDeuz g,wq