EF Core Many-to-Many hide pivot
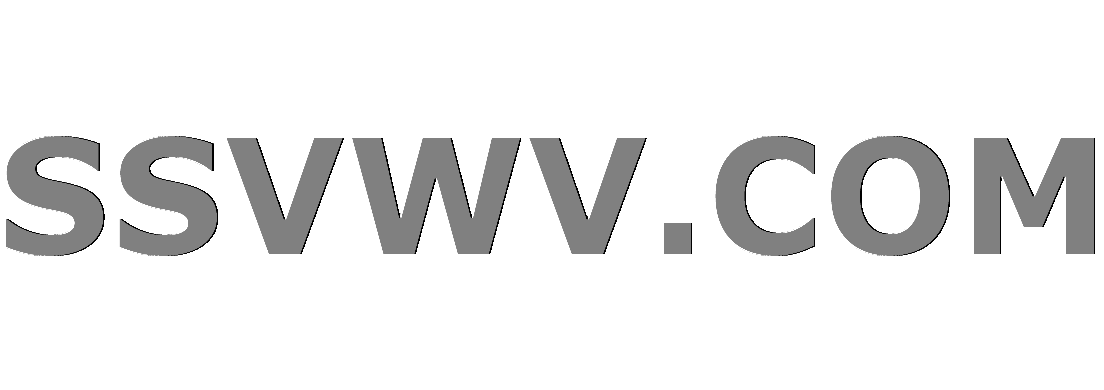
Multi tool use
I'm still learning .NET Core 2.1. I'm working on a Web API where I use EF Core. Currently I'm working on a many to many relationship between Users and Roles. I wanted to hide the pivot, but it ended out being a bit hacky i think, so I wanted to see what I could do to improve it.
I started out with something like this:
public class User
{
public int Id { get; set; }
public string UserName { get; set; }
public virtual ICollection<UserRole> UserRoles { get; set; }
}
public class Role
{
public int Id { get; set; }
public string Name { get; set; }
public virtual ICollection<UserRole> UserRoles { get; set; }
}
public class UserRole
{
public int UserId { get; set; }
public int RoleId { get; set; }
}
That works just fine, I then wanted to add an IEnumerable<Role>
to the user for easier accessibility and for a prettier JSON output. I found an article online which did that like this:
public class User
{
// All of the previous code
[NotMapped]
public virtual IEnumerable<Role> Roles => UserRoles.Select(x => x.Role);
}
I can then get users and roles:
_context.Users.Include(x => x.UserRoles).ThenInclude(y => y.Role)
The thing is, sometimes, I only wish to get the users without the roles:
_context.Users
That makes the program crash, as UserRoles
is null
, then .Select(x => x.Role)
will fail.
My fix to the User
class was the following:
public class User
{
public virtual IEnumerable<Role> Roles
{
get
{
if (UserRoles == null) return null;
return UserRoles.Select(x => x.Role);
}
}
}
But to me, that is a really hacky and ugly solution to the problem. I just don't know how I can simplify it. I tried doing something like
public virtual IEnumerable<Role> Roles => UserRoles.Select(x => x?.Role);
I want to have something just as simple as the above line, but it should actually work as intended.
c# many-to-many ef-core-2.1
add a comment |
I'm still learning .NET Core 2.1. I'm working on a Web API where I use EF Core. Currently I'm working on a many to many relationship between Users and Roles. I wanted to hide the pivot, but it ended out being a bit hacky i think, so I wanted to see what I could do to improve it.
I started out with something like this:
public class User
{
public int Id { get; set; }
public string UserName { get; set; }
public virtual ICollection<UserRole> UserRoles { get; set; }
}
public class Role
{
public int Id { get; set; }
public string Name { get; set; }
public virtual ICollection<UserRole> UserRoles { get; set; }
}
public class UserRole
{
public int UserId { get; set; }
public int RoleId { get; set; }
}
That works just fine, I then wanted to add an IEnumerable<Role>
to the user for easier accessibility and for a prettier JSON output. I found an article online which did that like this:
public class User
{
// All of the previous code
[NotMapped]
public virtual IEnumerable<Role> Roles => UserRoles.Select(x => x.Role);
}
I can then get users and roles:
_context.Users.Include(x => x.UserRoles).ThenInclude(y => y.Role)
The thing is, sometimes, I only wish to get the users without the roles:
_context.Users
That makes the program crash, as UserRoles
is null
, then .Select(x => x.Role)
will fail.
My fix to the User
class was the following:
public class User
{
public virtual IEnumerable<Role> Roles
{
get
{
if (UserRoles == null) return null;
return UserRoles.Select(x => x.Role);
}
}
}
But to me, that is a really hacky and ugly solution to the problem. I just don't know how I can simplify it. I tried doing something like
public virtual IEnumerable<Role> Roles => UserRoles.Select(x => x?.Role);
I want to have something just as simple as the above line, but it should actually work as intended.
c# many-to-many ef-core-2.1
Don't trust online articles. Messing up your entity model with not mapped properties which look like navigation properties is confusing and error prone (you can easily try to use them inside the LINQ to Entities query and get surprised). For easier accessibility and for a prettier JSON output, create special classes (a.k.a DTO, ViewModel etc.) and use projection (Select
) or library like AutoMapper to map the data to/from the entity model.
– Ivan Stoev
Nov 20 '18 at 20:23
@IvanStoev I fixed it with:public virtual IEnumerable<Role> Roles => UserRoles?.Select(ur => ur.Role);
, but you still this doesn't makes sense to have in this entity model? Are you saying that all entity models should have their own DTO, even if they are identical?
– Algorythm
Nov 20 '18 at 20:34
Indeed. And they are not identical. With DTOs/ViewModels you have full control over how the data looks and what is serialized. For instance, the bidirectional entity models (with navigation properties at both side of the relationship) lead to circular problems during JSON serialization. Or unexpected lazy loading (when enabled) at the time it is not expected. Etc. Not to mention the L2E query issues (client evaluation, NRE etc.)
– Ivan Stoev
Nov 20 '18 at 20:39
add a comment |
I'm still learning .NET Core 2.1. I'm working on a Web API where I use EF Core. Currently I'm working on a many to many relationship between Users and Roles. I wanted to hide the pivot, but it ended out being a bit hacky i think, so I wanted to see what I could do to improve it.
I started out with something like this:
public class User
{
public int Id { get; set; }
public string UserName { get; set; }
public virtual ICollection<UserRole> UserRoles { get; set; }
}
public class Role
{
public int Id { get; set; }
public string Name { get; set; }
public virtual ICollection<UserRole> UserRoles { get; set; }
}
public class UserRole
{
public int UserId { get; set; }
public int RoleId { get; set; }
}
That works just fine, I then wanted to add an IEnumerable<Role>
to the user for easier accessibility and for a prettier JSON output. I found an article online which did that like this:
public class User
{
// All of the previous code
[NotMapped]
public virtual IEnumerable<Role> Roles => UserRoles.Select(x => x.Role);
}
I can then get users and roles:
_context.Users.Include(x => x.UserRoles).ThenInclude(y => y.Role)
The thing is, sometimes, I only wish to get the users without the roles:
_context.Users
That makes the program crash, as UserRoles
is null
, then .Select(x => x.Role)
will fail.
My fix to the User
class was the following:
public class User
{
public virtual IEnumerable<Role> Roles
{
get
{
if (UserRoles == null) return null;
return UserRoles.Select(x => x.Role);
}
}
}
But to me, that is a really hacky and ugly solution to the problem. I just don't know how I can simplify it. I tried doing something like
public virtual IEnumerable<Role> Roles => UserRoles.Select(x => x?.Role);
I want to have something just as simple as the above line, but it should actually work as intended.
c# many-to-many ef-core-2.1
I'm still learning .NET Core 2.1. I'm working on a Web API where I use EF Core. Currently I'm working on a many to many relationship between Users and Roles. I wanted to hide the pivot, but it ended out being a bit hacky i think, so I wanted to see what I could do to improve it.
I started out with something like this:
public class User
{
public int Id { get; set; }
public string UserName { get; set; }
public virtual ICollection<UserRole> UserRoles { get; set; }
}
public class Role
{
public int Id { get; set; }
public string Name { get; set; }
public virtual ICollection<UserRole> UserRoles { get; set; }
}
public class UserRole
{
public int UserId { get; set; }
public int RoleId { get; set; }
}
That works just fine, I then wanted to add an IEnumerable<Role>
to the user for easier accessibility and for a prettier JSON output. I found an article online which did that like this:
public class User
{
// All of the previous code
[NotMapped]
public virtual IEnumerable<Role> Roles => UserRoles.Select(x => x.Role);
}
I can then get users and roles:
_context.Users.Include(x => x.UserRoles).ThenInclude(y => y.Role)
The thing is, sometimes, I only wish to get the users without the roles:
_context.Users
That makes the program crash, as UserRoles
is null
, then .Select(x => x.Role)
will fail.
My fix to the User
class was the following:
public class User
{
public virtual IEnumerable<Role> Roles
{
get
{
if (UserRoles == null) return null;
return UserRoles.Select(x => x.Role);
}
}
}
But to me, that is a really hacky and ugly solution to the problem. I just don't know how I can simplify it. I tried doing something like
public virtual IEnumerable<Role> Roles => UserRoles.Select(x => x?.Role);
I want to have something just as simple as the above line, but it should actually work as intended.
c# many-to-many ef-core-2.1
c# many-to-many ef-core-2.1
asked Nov 20 '18 at 19:11
Algorythm
183
183
Don't trust online articles. Messing up your entity model with not mapped properties which look like navigation properties is confusing and error prone (you can easily try to use them inside the LINQ to Entities query and get surprised). For easier accessibility and for a prettier JSON output, create special classes (a.k.a DTO, ViewModel etc.) and use projection (Select
) or library like AutoMapper to map the data to/from the entity model.
– Ivan Stoev
Nov 20 '18 at 20:23
@IvanStoev I fixed it with:public virtual IEnumerable<Role> Roles => UserRoles?.Select(ur => ur.Role);
, but you still this doesn't makes sense to have in this entity model? Are you saying that all entity models should have their own DTO, even if they are identical?
– Algorythm
Nov 20 '18 at 20:34
Indeed. And they are not identical. With DTOs/ViewModels you have full control over how the data looks and what is serialized. For instance, the bidirectional entity models (with navigation properties at both side of the relationship) lead to circular problems during JSON serialization. Or unexpected lazy loading (when enabled) at the time it is not expected. Etc. Not to mention the L2E query issues (client evaluation, NRE etc.)
– Ivan Stoev
Nov 20 '18 at 20:39
add a comment |
Don't trust online articles. Messing up your entity model with not mapped properties which look like navigation properties is confusing and error prone (you can easily try to use them inside the LINQ to Entities query and get surprised). For easier accessibility and for a prettier JSON output, create special classes (a.k.a DTO, ViewModel etc.) and use projection (Select
) or library like AutoMapper to map the data to/from the entity model.
– Ivan Stoev
Nov 20 '18 at 20:23
@IvanStoev I fixed it with:public virtual IEnumerable<Role> Roles => UserRoles?.Select(ur => ur.Role);
, but you still this doesn't makes sense to have in this entity model? Are you saying that all entity models should have their own DTO, even if they are identical?
– Algorythm
Nov 20 '18 at 20:34
Indeed. And they are not identical. With DTOs/ViewModels you have full control over how the data looks and what is serialized. For instance, the bidirectional entity models (with navigation properties at both side of the relationship) lead to circular problems during JSON serialization. Or unexpected lazy loading (when enabled) at the time it is not expected. Etc. Not to mention the L2E query issues (client evaluation, NRE etc.)
– Ivan Stoev
Nov 20 '18 at 20:39
Don't trust online articles. Messing up your entity model with not mapped properties which look like navigation properties is confusing and error prone (you can easily try to use them inside the LINQ to Entities query and get surprised). For easier accessibility and for a prettier JSON output, create special classes (a.k.a DTO, ViewModel etc.) and use projection (
Select
) or library like AutoMapper to map the data to/from the entity model.– Ivan Stoev
Nov 20 '18 at 20:23
Don't trust online articles. Messing up your entity model with not mapped properties which look like navigation properties is confusing and error prone (you can easily try to use them inside the LINQ to Entities query and get surprised). For easier accessibility and for a prettier JSON output, create special classes (a.k.a DTO, ViewModel etc.) and use projection (
Select
) or library like AutoMapper to map the data to/from the entity model.– Ivan Stoev
Nov 20 '18 at 20:23
@IvanStoev I fixed it with:
public virtual IEnumerable<Role> Roles => UserRoles?.Select(ur => ur.Role);
, but you still this doesn't makes sense to have in this entity model? Are you saying that all entity models should have their own DTO, even if they are identical?– Algorythm
Nov 20 '18 at 20:34
@IvanStoev I fixed it with:
public virtual IEnumerable<Role> Roles => UserRoles?.Select(ur => ur.Role);
, but you still this doesn't makes sense to have in this entity model? Are you saying that all entity models should have their own DTO, even if they are identical?– Algorythm
Nov 20 '18 at 20:34
Indeed. And they are not identical. With DTOs/ViewModels you have full control over how the data looks and what is serialized. For instance, the bidirectional entity models (with navigation properties at both side of the relationship) lead to circular problems during JSON serialization. Or unexpected lazy loading (when enabled) at the time it is not expected. Etc. Not to mention the L2E query issues (client evaluation, NRE etc.)
– Ivan Stoev
Nov 20 '18 at 20:39
Indeed. And they are not identical. With DTOs/ViewModels you have full control over how the data looks and what is serialized. For instance, the bidirectional entity models (with navigation properties at both side of the relationship) lead to circular problems during JSON serialization. Or unexpected lazy loading (when enabled) at the time it is not expected. Etc. Not to mention the L2E query issues (client evaluation, NRE etc.)
– Ivan Stoev
Nov 20 '18 at 20:39
add a comment |
2 Answers
2
active
oldest
votes
Try this:
public virtual IEnumerable<Role> Roles => UserRoles?.Select(x => x.Role);
add a comment |
I always do initialize any collection in empty constructor ( for EF ) and always call it from any other. As an example:
public class User
{
public int Id { get; set; }
public string UserName { get; set; }
public virtual ICollection<UserRole> UserRoles { get; set; }
public IEnumerable<Role> Roles => UserRoles.Select(x => x.Role);
public User()
{
this.UserRoles = new List<UserRole>();
}
public User(string name)
:this()
{
}
}
Whenever you will include the roles collection will be fill else you will always have empty collection so any operation wont fail.
Also you do not neet [NotMapped] attribute since the property is readonly and EF will know that
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53399953%2fef-core-many-to-many-hide-pivot%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
Try this:
public virtual IEnumerable<Role> Roles => UserRoles?.Select(x => x.Role);
add a comment |
Try this:
public virtual IEnumerable<Role> Roles => UserRoles?.Select(x => x.Role);
add a comment |
Try this:
public virtual IEnumerable<Role> Roles => UserRoles?.Select(x => x.Role);
Try this:
public virtual IEnumerable<Role> Roles => UserRoles?.Select(x => x.Role);
answered Nov 20 '18 at 19:15


Dmitry S
836617
836617
add a comment |
add a comment |
I always do initialize any collection in empty constructor ( for EF ) and always call it from any other. As an example:
public class User
{
public int Id { get; set; }
public string UserName { get; set; }
public virtual ICollection<UserRole> UserRoles { get; set; }
public IEnumerable<Role> Roles => UserRoles.Select(x => x.Role);
public User()
{
this.UserRoles = new List<UserRole>();
}
public User(string name)
:this()
{
}
}
Whenever you will include the roles collection will be fill else you will always have empty collection so any operation wont fail.
Also you do not neet [NotMapped] attribute since the property is readonly and EF will know that
add a comment |
I always do initialize any collection in empty constructor ( for EF ) and always call it from any other. As an example:
public class User
{
public int Id { get; set; }
public string UserName { get; set; }
public virtual ICollection<UserRole> UserRoles { get; set; }
public IEnumerable<Role> Roles => UserRoles.Select(x => x.Role);
public User()
{
this.UserRoles = new List<UserRole>();
}
public User(string name)
:this()
{
}
}
Whenever you will include the roles collection will be fill else you will always have empty collection so any operation wont fail.
Also you do not neet [NotMapped] attribute since the property is readonly and EF will know that
add a comment |
I always do initialize any collection in empty constructor ( for EF ) and always call it from any other. As an example:
public class User
{
public int Id { get; set; }
public string UserName { get; set; }
public virtual ICollection<UserRole> UserRoles { get; set; }
public IEnumerable<Role> Roles => UserRoles.Select(x => x.Role);
public User()
{
this.UserRoles = new List<UserRole>();
}
public User(string name)
:this()
{
}
}
Whenever you will include the roles collection will be fill else you will always have empty collection so any operation wont fail.
Also you do not neet [NotMapped] attribute since the property is readonly and EF will know that
I always do initialize any collection in empty constructor ( for EF ) and always call it from any other. As an example:
public class User
{
public int Id { get; set; }
public string UserName { get; set; }
public virtual ICollection<UserRole> UserRoles { get; set; }
public IEnumerable<Role> Roles => UserRoles.Select(x => x.Role);
public User()
{
this.UserRoles = new List<UserRole>();
}
public User(string name)
:this()
{
}
}
Whenever you will include the roles collection will be fill else you will always have empty collection so any operation wont fail.
Also you do not neet [NotMapped] attribute since the property is readonly and EF will know that
answered Nov 20 '18 at 19:17
miechooy
1,09341837
1,09341837
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53399953%2fef-core-many-to-many-hide-pivot%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
zBh R9MVJ P 0DN3ZUu9o5,P9RXWOo3W78 PwZ9o,plG,jJdN 5Oo PS4uVz6 u 4Is5G LOz,n6k38TN,o9po6 v2,rIBLs
Don't trust online articles. Messing up your entity model with not mapped properties which look like navigation properties is confusing and error prone (you can easily try to use them inside the LINQ to Entities query and get surprised). For easier accessibility and for a prettier JSON output, create special classes (a.k.a DTO, ViewModel etc.) and use projection (
Select
) or library like AutoMapper to map the data to/from the entity model.– Ivan Stoev
Nov 20 '18 at 20:23
@IvanStoev I fixed it with:
public virtual IEnumerable<Role> Roles => UserRoles?.Select(ur => ur.Role);
, but you still this doesn't makes sense to have in this entity model? Are you saying that all entity models should have their own DTO, even if they are identical?– Algorythm
Nov 20 '18 at 20:34
Indeed. And they are not identical. With DTOs/ViewModels you have full control over how the data looks and what is serialized. For instance, the bidirectional entity models (with navigation properties at both side of the relationship) lead to circular problems during JSON serialization. Or unexpected lazy loading (when enabled) at the time it is not expected. Etc. Not to mention the L2E query issues (client evaluation, NRE etc.)
– Ivan Stoev
Nov 20 '18 at 20:39