one of the variables needed for gradient computation has been modified by an inplace operation
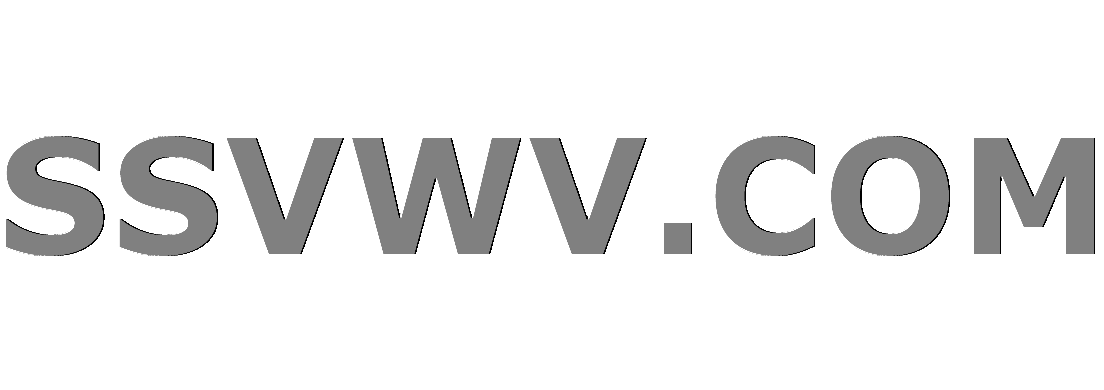
Multi tool use
Here is my LossFunction, when I use this function, it will make this error.
And I have tested that using the nn.L1Loss()
instead of my LossFunction, and the network is ok.
what should I do? Thanks for your help!
class LossV1(nn.Module):
def __init__(self,weight=1,pos_weight=1,scale_factor=2.5):
super(LossV1,self).__init__()
self.weight = weight
self.pos_weight = pos_weight
self.scale_factor = scale_factor
def forward(self,pred,truth):
objmask = torch.tensor(truth[:,:6,:,:],dtype=torch.float32,requires_grad=False)
#没有物体的Boxes,置信度损失*0.4
objmask[objmask<0.65] = 0.4
#辅助Boxes,系数0.8
objmask[(objmask>0.649)*(objmask<0.949)] = 0.8
objLoss = torch.sum(objmask*self.myBCEWithLogitsLoss(pred[:,:6,:,:],truth[:,:6,:,:]))
#没有物体的Boxes,只计算置信度损失
objmask[objmask<0.41] = 0
personLoss = torch.sum(objmask*self.myBCEWithLogitsLoss(pred[:,6:12,:,:],truth[:,6:12,:,:]))
carLoss = torch.sum(objmask*self.myBCEWithLogitsLoss(pred[:,12:18,:,:],truth[:,12:18,:,:]))
wLoss = torch.sum(objmask*self.myL2Loss(pred[:,18:24,:,:],truth[:,18:24,:,:]))
hLoss = torch.sum(objmask*self.myL2Loss(pred[:,24:,:,:],truth[:,24:,:,:]))
return objLoss+personLoss+carLoss+wLoss+hLoss
def myBCEWithLogitsLoss(self,x,y):
#pos_weight>1增加召回,pos_weight<1提高精度
return -self.weight*(self.pos_weight*y*torch.log(torch.sigmoid(x))+(1-y)*torch.log(1-torch.sigmoid(x)))
def myL2Loss(self,x,y):
return torch.pow(self.scale_factor*torch.sigmoid(x/self.scale_factor) - y,2)
python pytorch
add a comment |
Here is my LossFunction, when I use this function, it will make this error.
And I have tested that using the nn.L1Loss()
instead of my LossFunction, and the network is ok.
what should I do? Thanks for your help!
class LossV1(nn.Module):
def __init__(self,weight=1,pos_weight=1,scale_factor=2.5):
super(LossV1,self).__init__()
self.weight = weight
self.pos_weight = pos_weight
self.scale_factor = scale_factor
def forward(self,pred,truth):
objmask = torch.tensor(truth[:,:6,:,:],dtype=torch.float32,requires_grad=False)
#没有物体的Boxes,置信度损失*0.4
objmask[objmask<0.65] = 0.4
#辅助Boxes,系数0.8
objmask[(objmask>0.649)*(objmask<0.949)] = 0.8
objLoss = torch.sum(objmask*self.myBCEWithLogitsLoss(pred[:,:6,:,:],truth[:,:6,:,:]))
#没有物体的Boxes,只计算置信度损失
objmask[objmask<0.41] = 0
personLoss = torch.sum(objmask*self.myBCEWithLogitsLoss(pred[:,6:12,:,:],truth[:,6:12,:,:]))
carLoss = torch.sum(objmask*self.myBCEWithLogitsLoss(pred[:,12:18,:,:],truth[:,12:18,:,:]))
wLoss = torch.sum(objmask*self.myL2Loss(pred[:,18:24,:,:],truth[:,18:24,:,:]))
hLoss = torch.sum(objmask*self.myL2Loss(pred[:,24:,:,:],truth[:,24:,:,:]))
return objLoss+personLoss+carLoss+wLoss+hLoss
def myBCEWithLogitsLoss(self,x,y):
#pos_weight>1增加召回,pos_weight<1提高精度
return -self.weight*(self.pos_weight*y*torch.log(torch.sigmoid(x))+(1-y)*torch.log(1-torch.sigmoid(x)))
def myL2Loss(self,x,y):
return torch.pow(self.scale_factor*torch.sigmoid(x/self.scale_factor) - y,2)
python pytorch
The code seems to have no INPLACE operation
– heiheihei
Nov 25 '18 at 13:19
add a comment |
Here is my LossFunction, when I use this function, it will make this error.
And I have tested that using the nn.L1Loss()
instead of my LossFunction, and the network is ok.
what should I do? Thanks for your help!
class LossV1(nn.Module):
def __init__(self,weight=1,pos_weight=1,scale_factor=2.5):
super(LossV1,self).__init__()
self.weight = weight
self.pos_weight = pos_weight
self.scale_factor = scale_factor
def forward(self,pred,truth):
objmask = torch.tensor(truth[:,:6,:,:],dtype=torch.float32,requires_grad=False)
#没有物体的Boxes,置信度损失*0.4
objmask[objmask<0.65] = 0.4
#辅助Boxes,系数0.8
objmask[(objmask>0.649)*(objmask<0.949)] = 0.8
objLoss = torch.sum(objmask*self.myBCEWithLogitsLoss(pred[:,:6,:,:],truth[:,:6,:,:]))
#没有物体的Boxes,只计算置信度损失
objmask[objmask<0.41] = 0
personLoss = torch.sum(objmask*self.myBCEWithLogitsLoss(pred[:,6:12,:,:],truth[:,6:12,:,:]))
carLoss = torch.sum(objmask*self.myBCEWithLogitsLoss(pred[:,12:18,:,:],truth[:,12:18,:,:]))
wLoss = torch.sum(objmask*self.myL2Loss(pred[:,18:24,:,:],truth[:,18:24,:,:]))
hLoss = torch.sum(objmask*self.myL2Loss(pred[:,24:,:,:],truth[:,24:,:,:]))
return objLoss+personLoss+carLoss+wLoss+hLoss
def myBCEWithLogitsLoss(self,x,y):
#pos_weight>1增加召回,pos_weight<1提高精度
return -self.weight*(self.pos_weight*y*torch.log(torch.sigmoid(x))+(1-y)*torch.log(1-torch.sigmoid(x)))
def myL2Loss(self,x,y):
return torch.pow(self.scale_factor*torch.sigmoid(x/self.scale_factor) - y,2)
python pytorch
Here is my LossFunction, when I use this function, it will make this error.
And I have tested that using the nn.L1Loss()
instead of my LossFunction, and the network is ok.
what should I do? Thanks for your help!
class LossV1(nn.Module):
def __init__(self,weight=1,pos_weight=1,scale_factor=2.5):
super(LossV1,self).__init__()
self.weight = weight
self.pos_weight = pos_weight
self.scale_factor = scale_factor
def forward(self,pred,truth):
objmask = torch.tensor(truth[:,:6,:,:],dtype=torch.float32,requires_grad=False)
#没有物体的Boxes,置信度损失*0.4
objmask[objmask<0.65] = 0.4
#辅助Boxes,系数0.8
objmask[(objmask>0.649)*(objmask<0.949)] = 0.8
objLoss = torch.sum(objmask*self.myBCEWithLogitsLoss(pred[:,:6,:,:],truth[:,:6,:,:]))
#没有物体的Boxes,只计算置信度损失
objmask[objmask<0.41] = 0
personLoss = torch.sum(objmask*self.myBCEWithLogitsLoss(pred[:,6:12,:,:],truth[:,6:12,:,:]))
carLoss = torch.sum(objmask*self.myBCEWithLogitsLoss(pred[:,12:18,:,:],truth[:,12:18,:,:]))
wLoss = torch.sum(objmask*self.myL2Loss(pred[:,18:24,:,:],truth[:,18:24,:,:]))
hLoss = torch.sum(objmask*self.myL2Loss(pred[:,24:,:,:],truth[:,24:,:,:]))
return objLoss+personLoss+carLoss+wLoss+hLoss
def myBCEWithLogitsLoss(self,x,y):
#pos_weight>1增加召回,pos_weight<1提高精度
return -self.weight*(self.pos_weight*y*torch.log(torch.sigmoid(x))+(1-y)*torch.log(1-torch.sigmoid(x)))
def myL2Loss(self,x,y):
return torch.pow(self.scale_factor*torch.sigmoid(x/self.scale_factor) - y,2)
python pytorch
python pytorch
edited Nov 25 '18 at 17:10
Umang Gupta
3,51111739
3,51111739
asked Nov 25 '18 at 12:31
heiheiheiheiheihei
689
689
The code seems to have no INPLACE operation
– heiheihei
Nov 25 '18 at 13:19
add a comment |
The code seems to have no INPLACE operation
– heiheihei
Nov 25 '18 at 13:19
The code seems to have no INPLACE operation
– heiheihei
Nov 25 '18 at 13:19
The code seems to have no INPLACE operation
– heiheihei
Nov 25 '18 at 13:19
add a comment |
1 Answer
1
active
oldest
votes
I just remove the objmask
,and compute it in my generation function,then pass it on to LossFunction with truth label,and the network works.
I can't understand I have already done that making the requires_grad=False
,why pytroch still compute the gradient.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53467460%2fone-of-the-variables-needed-for-gradient-computation-has-been-modified-by-an-inp%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
I just remove the objmask
,and compute it in my generation function,then pass it on to LossFunction with truth label,and the network works.
I can't understand I have already done that making the requires_grad=False
,why pytroch still compute the gradient.
add a comment |
I just remove the objmask
,and compute it in my generation function,then pass it on to LossFunction with truth label,and the network works.
I can't understand I have already done that making the requires_grad=False
,why pytroch still compute the gradient.
add a comment |
I just remove the objmask
,and compute it in my generation function,then pass it on to LossFunction with truth label,and the network works.
I can't understand I have already done that making the requires_grad=False
,why pytroch still compute the gradient.
I just remove the objmask
,and compute it in my generation function,then pass it on to LossFunction with truth label,and the network works.
I can't understand I have already done that making the requires_grad=False
,why pytroch still compute the gradient.
answered Nov 26 '18 at 6:03
heiheiheiheiheihei
689
689
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53467460%2fone-of-the-variables-needed-for-gradient-computation-has-been-modified-by-an-inp%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
xHSarluiVSWy7JgolTZ5J,UTCMI3yzoQP z MOeQiAeUNpFW0ccgjAJzpkY6X,cJ0MtdC215cQ
The code seems to have no INPLACE operation
– heiheihei
Nov 25 '18 at 13:19