I wrote a bootloader but it always jumps to the failure section I wrote and does not load my stage 2
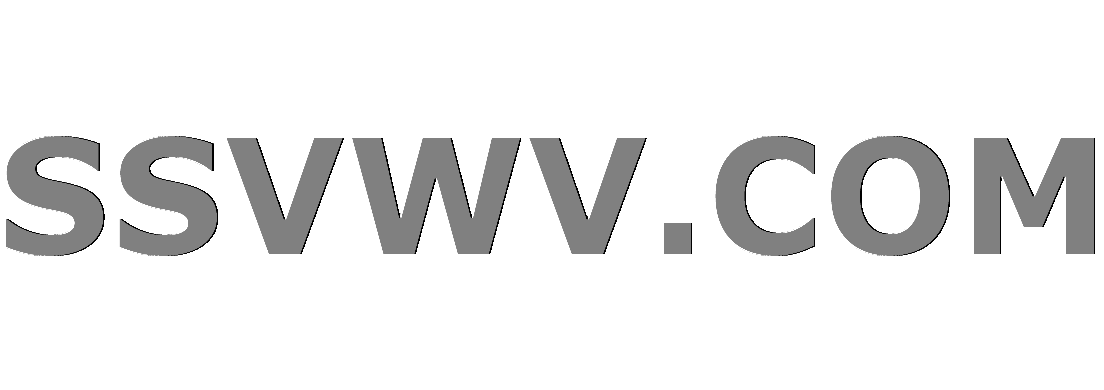
Multi tool use
I wrote a bootloader which should load my KRNLDR.SYS on a FAT12 formatted img file. But it doesn't work
Commands I used to create it:
nasm -fbin -o boot.bin boot.asm
dd if=/dev/zero of=test.img bs=512 count=2880
sudo losetup /dev/loop0 test.img
sudo mkdosfs -F 12 /dev/loop0
Now my system recognizes and mounts the device and I put my KRNLDR.SYS on it
Then I executed:
sudo dd if=boot.bin of=/dev/loop0 bs=512 count=1 conv=notrunc
sudo umount /dev/loop0
sudo losetup -d /dev/loop0
Now the file looks like a FAT12 floppy with a bootsector and the file KRNLDR.SYS
Now I execute it in QEMU:
qemu-system-i386 -device format=raw,file=test.img
And the output:
Operating System not found
Press any key to reboot
This is the output my bootloader prints to the screen if the file KRNLDR.SYS cannot be found by my bootloader
My Code:
org 0x0
bits 16
jmp word loader
bsOEMName: db "TestOS "
bpbBytesPerSector: dw 512
bpbSectorsPerCluster: db 1
bpbReservedSectors: dw 1
bpbNumberOfFATs: db 2
bpbNumberOfRootEntries: dw 224
bpbTotalSectors: dw 2880
bpbMedia: db 0xf0
bpbSectorsPerFAT: dw 9
bpbSectorsPerTrack: dw 18
bpbNumberOfHeads: dw 2
bpbHiddenSectors: dd 0
bpbTotalSectorsBig: dd 0
bsDriveNumber: db 0
bsReserved: db 0
bsExtendedBootSignature: db 0x29
bsVolumeID: dd 0x12345678
bsVolumeLabel: db "TestOS "
bsFileSystem: db "FAT12 "
;-------------------------------------------------------------------------------
; SI = Zero terminated string to print
;-------------------------------------------------------------------------------
printMsg:
push ax
.printStart:
lodsb
or al, al
jz .printEnd
mov ah, 0x0e
int 0x10
jmp .printStart
.printEnd:
pop ax
ret
;-------------------------------------------------------------------------------
; AX = Starting sector
; CX = Number of sectors to read
; ES:BX = Buffer
;-------------------------------------------------------------------------------
readSectors:
mov di, 0x0005
.readLoop:
push ax
push bx
push cx
call lbaToChs
mov ah, 0x02
mov al, 0x01
mov ch, byte [track]
mov cl, byte [sector]
mov dh, byte [head]
mov dl, byte [bsDriveNumber]
int 0x13
jnc .success
dec di
pop cx
pop bx
pop ax
jnz .readLoop
.success:
pop cx
pop bx
pop ax
inc ax
add bx, word [bpbBytesPerSector]
loop readSectors
ret
track: db 0
head: db 0
sector: db 0
;-------------------------------------------------------------------------------
; AX = Logical sector
;-------------------------------------------------------------------------------
lbaToChs:
xor dx, dx
div word [bpbSectorsPerTrack]
inc dl
mov byte [sector], dl
xor dx, dx
div word [bpbNumberOfHeads]
mov byte [head], dl
mov byte [track], al
ret
;-------------------------------------------------------------------------------
; AX = Cluster number
;-------------------------------------------------------------------------------
clusterToLba:
sub ax, 0x0002
xor cx, cx
mov cl, byte [bpbSectorsPerCluster]
mul cx
ret
;-------------------------------------------------------------------------------
loader:
cli
mov ax, 0x07c0
mov es, ax
mov gs, ax
mov fs, ax
mov ds, ax
mov ax, 0x0000
mov ss, ax
mov sp, 0xffff
sti
mov byte [bsDriveNumber], dl
xor dx, dx
xor cx, cx
mov ax, 0x0020
mul word [bpbNumberOfRootEntries]
div word [bpbBytesPerSector]
xchg cx, ax ; Number of sectors of the root directory
mov al, byte [bpbNumberOfFATs]
mul word [bpbSectorsPerFAT]
add ax, word [bpbReservedSectors] ; Starting sector of the root directory
mov bx, 0x0200
call readSectors
mov cx, word [bpbNumberOfRootEntries]
mov di, 0x0200
searchRoot:
push cx
mov cx, 0x000b
mov si, stage2
push di
rep cmpsb
pop di
je loadFat
pop cx
add di, 0x0020
loop searchRoot
jmp failure
loadFat:
mov dx, [di + 26] ; Starting address of entry
xor ax, ax
mov al, byte [bpbNumberOfFATs]
mul word [bpbSectorsPerFAT] ; Number of sectors used by the FATs
mov word [cluster], dx
mov cx, ax
mov ax, word [bpbReservedSectors]
mov bx, 0x0200
call readSectors
mov ax, 0x0050
mov es, ax
mov bx, 0x0000
push bx
loadFile:
mov ax, word [cluster]
pop bx
call clusterToLba
xor cx, cx
mov cl, byte [bpbSectorsPerCluster]
call readSectors
push bx
mov ax, word [cluster]
mov cx, ax
mov dx, ax
shr dx, 1
add cx, dx
mov bx, 0x0200
add bx, cx
mov dx, [bx]
test ax, 1
jnz oddCluster
evenCluster:
and dx, 0b0000111111111111
jmp next
oddCluster:
shr dx, 4
next:
mov word [cluster], dx
cmp dx, 0x0ff0
jb loadFile
jmp 0x0050:0 ; Far jmp to KRNLDR.SYS
failure:
mov si, fail
call printMsg
mov si, anykey
call printMsg
mov ah, 0x00
int 0x16 ; Await key press
jmp 0xffff:0 ; Reboot with far jmp to BIOS
stage2: db "KRNLDR SYS"
fail: db "Operating system not found", 0xd, 0xa, 0x0
anykey: db "Press any key to reboot", 0xd, 0xa, 0x0
cluster: dw 0
times 510 - ($ - $$) db 0
dw 0xaa55
What can I do?
Thanks for any help
assembly nasm x86-16 bootloader
add a comment |
I wrote a bootloader which should load my KRNLDR.SYS on a FAT12 formatted img file. But it doesn't work
Commands I used to create it:
nasm -fbin -o boot.bin boot.asm
dd if=/dev/zero of=test.img bs=512 count=2880
sudo losetup /dev/loop0 test.img
sudo mkdosfs -F 12 /dev/loop0
Now my system recognizes and mounts the device and I put my KRNLDR.SYS on it
Then I executed:
sudo dd if=boot.bin of=/dev/loop0 bs=512 count=1 conv=notrunc
sudo umount /dev/loop0
sudo losetup -d /dev/loop0
Now the file looks like a FAT12 floppy with a bootsector and the file KRNLDR.SYS
Now I execute it in QEMU:
qemu-system-i386 -device format=raw,file=test.img
And the output:
Operating System not found
Press any key to reboot
This is the output my bootloader prints to the screen if the file KRNLDR.SYS cannot be found by my bootloader
My Code:
org 0x0
bits 16
jmp word loader
bsOEMName: db "TestOS "
bpbBytesPerSector: dw 512
bpbSectorsPerCluster: db 1
bpbReservedSectors: dw 1
bpbNumberOfFATs: db 2
bpbNumberOfRootEntries: dw 224
bpbTotalSectors: dw 2880
bpbMedia: db 0xf0
bpbSectorsPerFAT: dw 9
bpbSectorsPerTrack: dw 18
bpbNumberOfHeads: dw 2
bpbHiddenSectors: dd 0
bpbTotalSectorsBig: dd 0
bsDriveNumber: db 0
bsReserved: db 0
bsExtendedBootSignature: db 0x29
bsVolumeID: dd 0x12345678
bsVolumeLabel: db "TestOS "
bsFileSystem: db "FAT12 "
;-------------------------------------------------------------------------------
; SI = Zero terminated string to print
;-------------------------------------------------------------------------------
printMsg:
push ax
.printStart:
lodsb
or al, al
jz .printEnd
mov ah, 0x0e
int 0x10
jmp .printStart
.printEnd:
pop ax
ret
;-------------------------------------------------------------------------------
; AX = Starting sector
; CX = Number of sectors to read
; ES:BX = Buffer
;-------------------------------------------------------------------------------
readSectors:
mov di, 0x0005
.readLoop:
push ax
push bx
push cx
call lbaToChs
mov ah, 0x02
mov al, 0x01
mov ch, byte [track]
mov cl, byte [sector]
mov dh, byte [head]
mov dl, byte [bsDriveNumber]
int 0x13
jnc .success
dec di
pop cx
pop bx
pop ax
jnz .readLoop
.success:
pop cx
pop bx
pop ax
inc ax
add bx, word [bpbBytesPerSector]
loop readSectors
ret
track: db 0
head: db 0
sector: db 0
;-------------------------------------------------------------------------------
; AX = Logical sector
;-------------------------------------------------------------------------------
lbaToChs:
xor dx, dx
div word [bpbSectorsPerTrack]
inc dl
mov byte [sector], dl
xor dx, dx
div word [bpbNumberOfHeads]
mov byte [head], dl
mov byte [track], al
ret
;-------------------------------------------------------------------------------
; AX = Cluster number
;-------------------------------------------------------------------------------
clusterToLba:
sub ax, 0x0002
xor cx, cx
mov cl, byte [bpbSectorsPerCluster]
mul cx
ret
;-------------------------------------------------------------------------------
loader:
cli
mov ax, 0x07c0
mov es, ax
mov gs, ax
mov fs, ax
mov ds, ax
mov ax, 0x0000
mov ss, ax
mov sp, 0xffff
sti
mov byte [bsDriveNumber], dl
xor dx, dx
xor cx, cx
mov ax, 0x0020
mul word [bpbNumberOfRootEntries]
div word [bpbBytesPerSector]
xchg cx, ax ; Number of sectors of the root directory
mov al, byte [bpbNumberOfFATs]
mul word [bpbSectorsPerFAT]
add ax, word [bpbReservedSectors] ; Starting sector of the root directory
mov bx, 0x0200
call readSectors
mov cx, word [bpbNumberOfRootEntries]
mov di, 0x0200
searchRoot:
push cx
mov cx, 0x000b
mov si, stage2
push di
rep cmpsb
pop di
je loadFat
pop cx
add di, 0x0020
loop searchRoot
jmp failure
loadFat:
mov dx, [di + 26] ; Starting address of entry
xor ax, ax
mov al, byte [bpbNumberOfFATs]
mul word [bpbSectorsPerFAT] ; Number of sectors used by the FATs
mov word [cluster], dx
mov cx, ax
mov ax, word [bpbReservedSectors]
mov bx, 0x0200
call readSectors
mov ax, 0x0050
mov es, ax
mov bx, 0x0000
push bx
loadFile:
mov ax, word [cluster]
pop bx
call clusterToLba
xor cx, cx
mov cl, byte [bpbSectorsPerCluster]
call readSectors
push bx
mov ax, word [cluster]
mov cx, ax
mov dx, ax
shr dx, 1
add cx, dx
mov bx, 0x0200
add bx, cx
mov dx, [bx]
test ax, 1
jnz oddCluster
evenCluster:
and dx, 0b0000111111111111
jmp next
oddCluster:
shr dx, 4
next:
mov word [cluster], dx
cmp dx, 0x0ff0
jb loadFile
jmp 0x0050:0 ; Far jmp to KRNLDR.SYS
failure:
mov si, fail
call printMsg
mov si, anykey
call printMsg
mov ah, 0x00
int 0x16 ; Await key press
jmp 0xffff:0 ; Reboot with far jmp to BIOS
stage2: db "KRNLDR SYS"
fail: db "Operating system not found", 0xd, 0xa, 0x0
anykey: db "Press any key to reboot", 0xd, 0xa, 0x0
cluster: dw 0
times 510 - ($ - $$) db 0
dw 0xaa55
What can I do?
Thanks for any help
assembly nasm x86-16 bootloader
Have you considered using something like BOCHs to step through your code with its debugger? BOCHs has a built in debugger that works well in real mode. You can watch your code and see how it fails.
– Michael Petch
Nov 25 '18 at 13:42
I just set it up. Now I'm debugging
– Grevak
Nov 25 '18 at 14:06
add a comment |
I wrote a bootloader which should load my KRNLDR.SYS on a FAT12 formatted img file. But it doesn't work
Commands I used to create it:
nasm -fbin -o boot.bin boot.asm
dd if=/dev/zero of=test.img bs=512 count=2880
sudo losetup /dev/loop0 test.img
sudo mkdosfs -F 12 /dev/loop0
Now my system recognizes and mounts the device and I put my KRNLDR.SYS on it
Then I executed:
sudo dd if=boot.bin of=/dev/loop0 bs=512 count=1 conv=notrunc
sudo umount /dev/loop0
sudo losetup -d /dev/loop0
Now the file looks like a FAT12 floppy with a bootsector and the file KRNLDR.SYS
Now I execute it in QEMU:
qemu-system-i386 -device format=raw,file=test.img
And the output:
Operating System not found
Press any key to reboot
This is the output my bootloader prints to the screen if the file KRNLDR.SYS cannot be found by my bootloader
My Code:
org 0x0
bits 16
jmp word loader
bsOEMName: db "TestOS "
bpbBytesPerSector: dw 512
bpbSectorsPerCluster: db 1
bpbReservedSectors: dw 1
bpbNumberOfFATs: db 2
bpbNumberOfRootEntries: dw 224
bpbTotalSectors: dw 2880
bpbMedia: db 0xf0
bpbSectorsPerFAT: dw 9
bpbSectorsPerTrack: dw 18
bpbNumberOfHeads: dw 2
bpbHiddenSectors: dd 0
bpbTotalSectorsBig: dd 0
bsDriveNumber: db 0
bsReserved: db 0
bsExtendedBootSignature: db 0x29
bsVolumeID: dd 0x12345678
bsVolumeLabel: db "TestOS "
bsFileSystem: db "FAT12 "
;-------------------------------------------------------------------------------
; SI = Zero terminated string to print
;-------------------------------------------------------------------------------
printMsg:
push ax
.printStart:
lodsb
or al, al
jz .printEnd
mov ah, 0x0e
int 0x10
jmp .printStart
.printEnd:
pop ax
ret
;-------------------------------------------------------------------------------
; AX = Starting sector
; CX = Number of sectors to read
; ES:BX = Buffer
;-------------------------------------------------------------------------------
readSectors:
mov di, 0x0005
.readLoop:
push ax
push bx
push cx
call lbaToChs
mov ah, 0x02
mov al, 0x01
mov ch, byte [track]
mov cl, byte [sector]
mov dh, byte [head]
mov dl, byte [bsDriveNumber]
int 0x13
jnc .success
dec di
pop cx
pop bx
pop ax
jnz .readLoop
.success:
pop cx
pop bx
pop ax
inc ax
add bx, word [bpbBytesPerSector]
loop readSectors
ret
track: db 0
head: db 0
sector: db 0
;-------------------------------------------------------------------------------
; AX = Logical sector
;-------------------------------------------------------------------------------
lbaToChs:
xor dx, dx
div word [bpbSectorsPerTrack]
inc dl
mov byte [sector], dl
xor dx, dx
div word [bpbNumberOfHeads]
mov byte [head], dl
mov byte [track], al
ret
;-------------------------------------------------------------------------------
; AX = Cluster number
;-------------------------------------------------------------------------------
clusterToLba:
sub ax, 0x0002
xor cx, cx
mov cl, byte [bpbSectorsPerCluster]
mul cx
ret
;-------------------------------------------------------------------------------
loader:
cli
mov ax, 0x07c0
mov es, ax
mov gs, ax
mov fs, ax
mov ds, ax
mov ax, 0x0000
mov ss, ax
mov sp, 0xffff
sti
mov byte [bsDriveNumber], dl
xor dx, dx
xor cx, cx
mov ax, 0x0020
mul word [bpbNumberOfRootEntries]
div word [bpbBytesPerSector]
xchg cx, ax ; Number of sectors of the root directory
mov al, byte [bpbNumberOfFATs]
mul word [bpbSectorsPerFAT]
add ax, word [bpbReservedSectors] ; Starting sector of the root directory
mov bx, 0x0200
call readSectors
mov cx, word [bpbNumberOfRootEntries]
mov di, 0x0200
searchRoot:
push cx
mov cx, 0x000b
mov si, stage2
push di
rep cmpsb
pop di
je loadFat
pop cx
add di, 0x0020
loop searchRoot
jmp failure
loadFat:
mov dx, [di + 26] ; Starting address of entry
xor ax, ax
mov al, byte [bpbNumberOfFATs]
mul word [bpbSectorsPerFAT] ; Number of sectors used by the FATs
mov word [cluster], dx
mov cx, ax
mov ax, word [bpbReservedSectors]
mov bx, 0x0200
call readSectors
mov ax, 0x0050
mov es, ax
mov bx, 0x0000
push bx
loadFile:
mov ax, word [cluster]
pop bx
call clusterToLba
xor cx, cx
mov cl, byte [bpbSectorsPerCluster]
call readSectors
push bx
mov ax, word [cluster]
mov cx, ax
mov dx, ax
shr dx, 1
add cx, dx
mov bx, 0x0200
add bx, cx
mov dx, [bx]
test ax, 1
jnz oddCluster
evenCluster:
and dx, 0b0000111111111111
jmp next
oddCluster:
shr dx, 4
next:
mov word [cluster], dx
cmp dx, 0x0ff0
jb loadFile
jmp 0x0050:0 ; Far jmp to KRNLDR.SYS
failure:
mov si, fail
call printMsg
mov si, anykey
call printMsg
mov ah, 0x00
int 0x16 ; Await key press
jmp 0xffff:0 ; Reboot with far jmp to BIOS
stage2: db "KRNLDR SYS"
fail: db "Operating system not found", 0xd, 0xa, 0x0
anykey: db "Press any key to reboot", 0xd, 0xa, 0x0
cluster: dw 0
times 510 - ($ - $$) db 0
dw 0xaa55
What can I do?
Thanks for any help
assembly nasm x86-16 bootloader
I wrote a bootloader which should load my KRNLDR.SYS on a FAT12 formatted img file. But it doesn't work
Commands I used to create it:
nasm -fbin -o boot.bin boot.asm
dd if=/dev/zero of=test.img bs=512 count=2880
sudo losetup /dev/loop0 test.img
sudo mkdosfs -F 12 /dev/loop0
Now my system recognizes and mounts the device and I put my KRNLDR.SYS on it
Then I executed:
sudo dd if=boot.bin of=/dev/loop0 bs=512 count=1 conv=notrunc
sudo umount /dev/loop0
sudo losetup -d /dev/loop0
Now the file looks like a FAT12 floppy with a bootsector and the file KRNLDR.SYS
Now I execute it in QEMU:
qemu-system-i386 -device format=raw,file=test.img
And the output:
Operating System not found
Press any key to reboot
This is the output my bootloader prints to the screen if the file KRNLDR.SYS cannot be found by my bootloader
My Code:
org 0x0
bits 16
jmp word loader
bsOEMName: db "TestOS "
bpbBytesPerSector: dw 512
bpbSectorsPerCluster: db 1
bpbReservedSectors: dw 1
bpbNumberOfFATs: db 2
bpbNumberOfRootEntries: dw 224
bpbTotalSectors: dw 2880
bpbMedia: db 0xf0
bpbSectorsPerFAT: dw 9
bpbSectorsPerTrack: dw 18
bpbNumberOfHeads: dw 2
bpbHiddenSectors: dd 0
bpbTotalSectorsBig: dd 0
bsDriveNumber: db 0
bsReserved: db 0
bsExtendedBootSignature: db 0x29
bsVolumeID: dd 0x12345678
bsVolumeLabel: db "TestOS "
bsFileSystem: db "FAT12 "
;-------------------------------------------------------------------------------
; SI = Zero terminated string to print
;-------------------------------------------------------------------------------
printMsg:
push ax
.printStart:
lodsb
or al, al
jz .printEnd
mov ah, 0x0e
int 0x10
jmp .printStart
.printEnd:
pop ax
ret
;-------------------------------------------------------------------------------
; AX = Starting sector
; CX = Number of sectors to read
; ES:BX = Buffer
;-------------------------------------------------------------------------------
readSectors:
mov di, 0x0005
.readLoop:
push ax
push bx
push cx
call lbaToChs
mov ah, 0x02
mov al, 0x01
mov ch, byte [track]
mov cl, byte [sector]
mov dh, byte [head]
mov dl, byte [bsDriveNumber]
int 0x13
jnc .success
dec di
pop cx
pop bx
pop ax
jnz .readLoop
.success:
pop cx
pop bx
pop ax
inc ax
add bx, word [bpbBytesPerSector]
loop readSectors
ret
track: db 0
head: db 0
sector: db 0
;-------------------------------------------------------------------------------
; AX = Logical sector
;-------------------------------------------------------------------------------
lbaToChs:
xor dx, dx
div word [bpbSectorsPerTrack]
inc dl
mov byte [sector], dl
xor dx, dx
div word [bpbNumberOfHeads]
mov byte [head], dl
mov byte [track], al
ret
;-------------------------------------------------------------------------------
; AX = Cluster number
;-------------------------------------------------------------------------------
clusterToLba:
sub ax, 0x0002
xor cx, cx
mov cl, byte [bpbSectorsPerCluster]
mul cx
ret
;-------------------------------------------------------------------------------
loader:
cli
mov ax, 0x07c0
mov es, ax
mov gs, ax
mov fs, ax
mov ds, ax
mov ax, 0x0000
mov ss, ax
mov sp, 0xffff
sti
mov byte [bsDriveNumber], dl
xor dx, dx
xor cx, cx
mov ax, 0x0020
mul word [bpbNumberOfRootEntries]
div word [bpbBytesPerSector]
xchg cx, ax ; Number of sectors of the root directory
mov al, byte [bpbNumberOfFATs]
mul word [bpbSectorsPerFAT]
add ax, word [bpbReservedSectors] ; Starting sector of the root directory
mov bx, 0x0200
call readSectors
mov cx, word [bpbNumberOfRootEntries]
mov di, 0x0200
searchRoot:
push cx
mov cx, 0x000b
mov si, stage2
push di
rep cmpsb
pop di
je loadFat
pop cx
add di, 0x0020
loop searchRoot
jmp failure
loadFat:
mov dx, [di + 26] ; Starting address of entry
xor ax, ax
mov al, byte [bpbNumberOfFATs]
mul word [bpbSectorsPerFAT] ; Number of sectors used by the FATs
mov word [cluster], dx
mov cx, ax
mov ax, word [bpbReservedSectors]
mov bx, 0x0200
call readSectors
mov ax, 0x0050
mov es, ax
mov bx, 0x0000
push bx
loadFile:
mov ax, word [cluster]
pop bx
call clusterToLba
xor cx, cx
mov cl, byte [bpbSectorsPerCluster]
call readSectors
push bx
mov ax, word [cluster]
mov cx, ax
mov dx, ax
shr dx, 1
add cx, dx
mov bx, 0x0200
add bx, cx
mov dx, [bx]
test ax, 1
jnz oddCluster
evenCluster:
and dx, 0b0000111111111111
jmp next
oddCluster:
shr dx, 4
next:
mov word [cluster], dx
cmp dx, 0x0ff0
jb loadFile
jmp 0x0050:0 ; Far jmp to KRNLDR.SYS
failure:
mov si, fail
call printMsg
mov si, anykey
call printMsg
mov ah, 0x00
int 0x16 ; Await key press
jmp 0xffff:0 ; Reboot with far jmp to BIOS
stage2: db "KRNLDR SYS"
fail: db "Operating system not found", 0xd, 0xa, 0x0
anykey: db "Press any key to reboot", 0xd, 0xa, 0x0
cluster: dw 0
times 510 - ($ - $$) db 0
dw 0xaa55
What can I do?
Thanks for any help
assembly nasm x86-16 bootloader
assembly nasm x86-16 bootloader
edited Nov 25 '18 at 23:42
Michael Petch
26.6k556105
26.6k556105
asked Nov 25 '18 at 12:24
GrevakGrevak
11210
11210
Have you considered using something like BOCHs to step through your code with its debugger? BOCHs has a built in debugger that works well in real mode. You can watch your code and see how it fails.
– Michael Petch
Nov 25 '18 at 13:42
I just set it up. Now I'm debugging
– Grevak
Nov 25 '18 at 14:06
add a comment |
Have you considered using something like BOCHs to step through your code with its debugger? BOCHs has a built in debugger that works well in real mode. You can watch your code and see how it fails.
– Michael Petch
Nov 25 '18 at 13:42
I just set it up. Now I'm debugging
– Grevak
Nov 25 '18 at 14:06
Have you considered using something like BOCHs to step through your code with its debugger? BOCHs has a built in debugger that works well in real mode. You can watch your code and see how it fails.
– Michael Petch
Nov 25 '18 at 13:42
Have you considered using something like BOCHs to step through your code with its debugger? BOCHs has a built in debugger that works well in real mode. You can watch your code and see how it fails.
– Michael Petch
Nov 25 '18 at 13:42
I just set it up. Now I'm debugging
– Grevak
Nov 25 '18 at 14:06
I just set it up. Now I'm debugging
– Grevak
Nov 25 '18 at 14:06
add a comment |
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53467404%2fi-wrote-a-bootloader-but-it-always-jumps-to-the-failure-section-i-wrote-and-does%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53467404%2fi-wrote-a-bootloader-but-it-always-jumps-to-the-failure-section-i-wrote-and-does%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
my8U0b4bnV4jPvPVAbbx 9,E
Have you considered using something like BOCHs to step through your code with its debugger? BOCHs has a built in debugger that works well in real mode. You can watch your code and see how it fails.
– Michael Petch
Nov 25 '18 at 13:42
I just set it up. Now I'm debugging
– Grevak
Nov 25 '18 at 14:06