Get selected pin coordinate using MapKit framework (Objective-C)
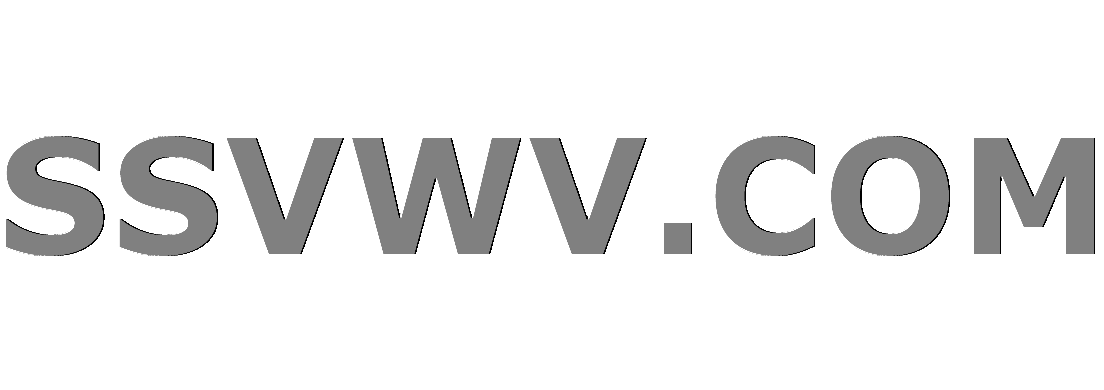
Multi tool use
I have a map region with about ten annotated MapPin objects (coordinates retrieved from a plist). In the code below 'locations' is a NSMutable array object containing the pin annotation latitude and longitude.
for (int i = 0; i<[locations count]; i++) {
MKCoordinateSpan span = MKCoordinateSpanMake(0, 0);
CLLocationCoordinate2D center = CLLocationCoordinate2DMake(0, 0);
pinRegion = MKCoordinateRegionMake(center, span);
MapPin *pin = [[MapPin alloc] init];
pinRegion.center.longitude = [locations[i][0] doubleValue];
pinRegion.center.latitude = [locations[i][1] doubleValue];
pin.title = names[i];
pin.coordinate = pinRegion.center;
[self.mapView addAnnotation:pin];
}
By selecting any pin I want to return its coordinate. I can inspect any pin object address like this:
NSLog(@"%@", self.mapView.selectedAnnotations);
... shows a unique address for a selected pin such as "<MapPin: 0x16d2f610>"
But I don't know how to access the objects coordinate properties such as longitude and latitude.
Please can you help?
Thank you!
objective-c mapkit mapkitannotation
add a comment |
I have a map region with about ten annotated MapPin objects (coordinates retrieved from a plist). In the code below 'locations' is a NSMutable array object containing the pin annotation latitude and longitude.
for (int i = 0; i<[locations count]; i++) {
MKCoordinateSpan span = MKCoordinateSpanMake(0, 0);
CLLocationCoordinate2D center = CLLocationCoordinate2DMake(0, 0);
pinRegion = MKCoordinateRegionMake(center, span);
MapPin *pin = [[MapPin alloc] init];
pinRegion.center.longitude = [locations[i][0] doubleValue];
pinRegion.center.latitude = [locations[i][1] doubleValue];
pin.title = names[i];
pin.coordinate = pinRegion.center;
[self.mapView addAnnotation:pin];
}
By selecting any pin I want to return its coordinate. I can inspect any pin object address like this:
NSLog(@"%@", self.mapView.selectedAnnotations);
... shows a unique address for a selected pin such as "<MapPin: 0x16d2f610>"
But I don't know how to access the objects coordinate properties such as longitude and latitude.
Please can you help?
Thank you!
objective-c mapkit mapkitannotation
add a comment |
I have a map region with about ten annotated MapPin objects (coordinates retrieved from a plist). In the code below 'locations' is a NSMutable array object containing the pin annotation latitude and longitude.
for (int i = 0; i<[locations count]; i++) {
MKCoordinateSpan span = MKCoordinateSpanMake(0, 0);
CLLocationCoordinate2D center = CLLocationCoordinate2DMake(0, 0);
pinRegion = MKCoordinateRegionMake(center, span);
MapPin *pin = [[MapPin alloc] init];
pinRegion.center.longitude = [locations[i][0] doubleValue];
pinRegion.center.latitude = [locations[i][1] doubleValue];
pin.title = names[i];
pin.coordinate = pinRegion.center;
[self.mapView addAnnotation:pin];
}
By selecting any pin I want to return its coordinate. I can inspect any pin object address like this:
NSLog(@"%@", self.mapView.selectedAnnotations);
... shows a unique address for a selected pin such as "<MapPin: 0x16d2f610>"
But I don't know how to access the objects coordinate properties such as longitude and latitude.
Please can you help?
Thank you!
objective-c mapkit mapkitannotation
I have a map region with about ten annotated MapPin objects (coordinates retrieved from a plist). In the code below 'locations' is a NSMutable array object containing the pin annotation latitude and longitude.
for (int i = 0; i<[locations count]; i++) {
MKCoordinateSpan span = MKCoordinateSpanMake(0, 0);
CLLocationCoordinate2D center = CLLocationCoordinate2DMake(0, 0);
pinRegion = MKCoordinateRegionMake(center, span);
MapPin *pin = [[MapPin alloc] init];
pinRegion.center.longitude = [locations[i][0] doubleValue];
pinRegion.center.latitude = [locations[i][1] doubleValue];
pin.title = names[i];
pin.coordinate = pinRegion.center;
[self.mapView addAnnotation:pin];
}
By selecting any pin I want to return its coordinate. I can inspect any pin object address like this:
NSLog(@"%@", self.mapView.selectedAnnotations);
... shows a unique address for a selected pin such as "<MapPin: 0x16d2f610>"
But I don't know how to access the objects coordinate properties such as longitude and latitude.
Please can you help?
Thank you!
objective-c mapkit mapkitannotation
objective-c mapkit mapkitannotation
edited Nov 25 '18 at 12:29
awyr_agored
asked Nov 25 '18 at 12:21
awyr_agoredawyr_agored
249111
249111
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
Make sure your map view has its delegate assigned (in this example we will use your view controller)
mapView.delegate = self
Next handle the callback delegate for annotation taps like this:
Swift
func mapView(mapView: MKMapView, didSelectAnnotationView view: MKAnnotationView)
{
if let annotationCoordinate = view.annotation?.coordinate
{
print("User tapped on annotation with title: (annotationCoordinate")
}
}
Objective - C
- (void)mapView:(MKMapView *)mapView didSelectAnnotationView:(MKAnnotationView *)view{
NSLog(view.annotation.coordinate);
}
Let me know if you have any questions
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53467381%2fget-selected-pin-coordinate-using-mapkit-framework-objective-c%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
Make sure your map view has its delegate assigned (in this example we will use your view controller)
mapView.delegate = self
Next handle the callback delegate for annotation taps like this:
Swift
func mapView(mapView: MKMapView, didSelectAnnotationView view: MKAnnotationView)
{
if let annotationCoordinate = view.annotation?.coordinate
{
print("User tapped on annotation with title: (annotationCoordinate")
}
}
Objective - C
- (void)mapView:(MKMapView *)mapView didSelectAnnotationView:(MKAnnotationView *)view{
NSLog(view.annotation.coordinate);
}
Let me know if you have any questions
add a comment |
Make sure your map view has its delegate assigned (in this example we will use your view controller)
mapView.delegate = self
Next handle the callback delegate for annotation taps like this:
Swift
func mapView(mapView: MKMapView, didSelectAnnotationView view: MKAnnotationView)
{
if let annotationCoordinate = view.annotation?.coordinate
{
print("User tapped on annotation with title: (annotationCoordinate")
}
}
Objective - C
- (void)mapView:(MKMapView *)mapView didSelectAnnotationView:(MKAnnotationView *)view{
NSLog(view.annotation.coordinate);
}
Let me know if you have any questions
add a comment |
Make sure your map view has its delegate assigned (in this example we will use your view controller)
mapView.delegate = self
Next handle the callback delegate for annotation taps like this:
Swift
func mapView(mapView: MKMapView, didSelectAnnotationView view: MKAnnotationView)
{
if let annotationCoordinate = view.annotation?.coordinate
{
print("User tapped on annotation with title: (annotationCoordinate")
}
}
Objective - C
- (void)mapView:(MKMapView *)mapView didSelectAnnotationView:(MKAnnotationView *)view{
NSLog(view.annotation.coordinate);
}
Let me know if you have any questions
Make sure your map view has its delegate assigned (in this example we will use your view controller)
mapView.delegate = self
Next handle the callback delegate for annotation taps like this:
Swift
func mapView(mapView: MKMapView, didSelectAnnotationView view: MKAnnotationView)
{
if let annotationCoordinate = view.annotation?.coordinate
{
print("User tapped on annotation with title: (annotationCoordinate")
}
}
Objective - C
- (void)mapView:(MKMapView *)mapView didSelectAnnotationView:(MKAnnotationView *)view{
NSLog(view.annotation.coordinate);
}
Let me know if you have any questions
answered Nov 25 '18 at 14:32
cevalloacevalloa
1024
1024
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53467381%2fget-selected-pin-coordinate-using-mapkit-framework-objective-c%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
3QsAoceNZ27AXQNqGweTH,6