Pass dropdownlist value to controller
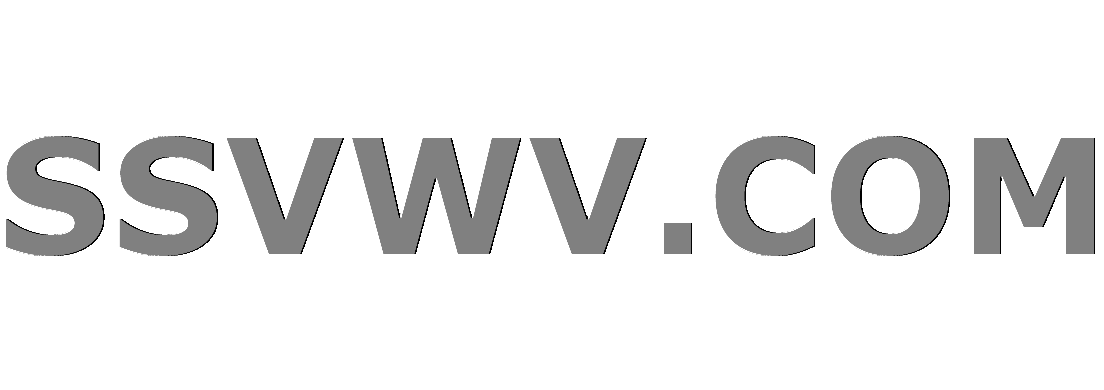
Multi tool use
I have dropdownlist and want to pass value in Controller. View
@using (Html.BeginForm())
{
@Html.DropDownList("dropOrg", ViewBag.dropOrg as SelectList)
<input type="submit" value="save" />
}
Controller
foreach (int tmp in org)
{
string s = tmp + " - " + orgNames[tmp];
SelectListItem item1 = new SelectListItem() { Text = s, Value = tmp.ToString() };
items.Add(item1);
}
ViewBag.dropOrg = items;
What should i do?
c# asp.net asp.net-mvc model-view-controller drop-down-menu
add a comment |
I have dropdownlist and want to pass value in Controller. View
@using (Html.BeginForm())
{
@Html.DropDownList("dropOrg", ViewBag.dropOrg as SelectList)
<input type="submit" value="save" />
}
Controller
foreach (int tmp in org)
{
string s = tmp + " - " + orgNames[tmp];
SelectListItem item1 = new SelectListItem() { Text = s, Value = tmp.ToString() };
items.Add(item1);
}
ViewBag.dropOrg = items;
What should i do?
c# asp.net asp.net-mvc model-view-controller drop-down-menu
How about taking a tutorial?
– Reniuz
Nov 26 '18 at 11:18
I would suggest using a DropDownListFor for this. If set on this, I would go with calling a javasript function and using jquery to get the value. Passing it to the controller using an ajax.
– JamesS
Nov 26 '18 at 11:20
Is there any easyer way to do it?
– Giorgi Asanidze
Nov 26 '18 at 11:39
Possible duplicate of How to get DropDownList SelectedValue in Controller in MVC
– Tetsuya Yamamoto
Nov 27 '18 at 1:29
add a comment |
I have dropdownlist and want to pass value in Controller. View
@using (Html.BeginForm())
{
@Html.DropDownList("dropOrg", ViewBag.dropOrg as SelectList)
<input type="submit" value="save" />
}
Controller
foreach (int tmp in org)
{
string s = tmp + " - " + orgNames[tmp];
SelectListItem item1 = new SelectListItem() { Text = s, Value = tmp.ToString() };
items.Add(item1);
}
ViewBag.dropOrg = items;
What should i do?
c# asp.net asp.net-mvc model-view-controller drop-down-menu
I have dropdownlist and want to pass value in Controller. View
@using (Html.BeginForm())
{
@Html.DropDownList("dropOrg", ViewBag.dropOrg as SelectList)
<input type="submit" value="save" />
}
Controller
foreach (int tmp in org)
{
string s = tmp + " - " + orgNames[tmp];
SelectListItem item1 = new SelectListItem() { Text = s, Value = tmp.ToString() };
items.Add(item1);
}
ViewBag.dropOrg = items;
What should i do?
c# asp.net asp.net-mvc model-view-controller drop-down-menu
c# asp.net asp.net-mvc model-view-controller drop-down-menu
edited Nov 26 '18 at 11:16
Reniuz
10.3k13556
10.3k13556
asked Nov 26 '18 at 11:12
Giorgi AsanidzeGiorgi Asanidze
63
63
How about taking a tutorial?
– Reniuz
Nov 26 '18 at 11:18
I would suggest using a DropDownListFor for this. If set on this, I would go with calling a javasript function and using jquery to get the value. Passing it to the controller using an ajax.
– JamesS
Nov 26 '18 at 11:20
Is there any easyer way to do it?
– Giorgi Asanidze
Nov 26 '18 at 11:39
Possible duplicate of How to get DropDownList SelectedValue in Controller in MVC
– Tetsuya Yamamoto
Nov 27 '18 at 1:29
add a comment |
How about taking a tutorial?
– Reniuz
Nov 26 '18 at 11:18
I would suggest using a DropDownListFor for this. If set on this, I would go with calling a javasript function and using jquery to get the value. Passing it to the controller using an ajax.
– JamesS
Nov 26 '18 at 11:20
Is there any easyer way to do it?
– Giorgi Asanidze
Nov 26 '18 at 11:39
Possible duplicate of How to get DropDownList SelectedValue in Controller in MVC
– Tetsuya Yamamoto
Nov 27 '18 at 1:29
How about taking a tutorial?
– Reniuz
Nov 26 '18 at 11:18
How about taking a tutorial?
– Reniuz
Nov 26 '18 at 11:18
I would suggest using a DropDownListFor for this. If set on this, I would go with calling a javasript function and using jquery to get the value. Passing it to the controller using an ajax.
– JamesS
Nov 26 '18 at 11:20
I would suggest using a DropDownListFor for this. If set on this, I would go with calling a javasript function and using jquery to get the value. Passing it to the controller using an ajax.
– JamesS
Nov 26 '18 at 11:20
Is there any easyer way to do it?
– Giorgi Asanidze
Nov 26 '18 at 11:39
Is there any easyer way to do it?
– Giorgi Asanidze
Nov 26 '18 at 11:39
Possible duplicate of How to get DropDownList SelectedValue in Controller in MVC
– Tetsuya Yamamoto
Nov 27 '18 at 1:29
Possible duplicate of How to get DropDownList SelectedValue in Controller in MVC
– Tetsuya Yamamoto
Nov 27 '18 at 1:29
add a comment |
1 Answer
1
active
oldest
votes
It will be better if you create ViewModel for your View:
public class SampleViewModel
{
public string DropDownListValue { get; set; }
}
then in your controller's get method:
public ActionResult SomeAction()
{
var org = GetOrg(); //your org
var orgNames = GetOrgNames(); //your orgNames
// . . .
ViewBag.DropDownListValue = new SelectList(org.Select(s =>
new SampleViewModel
{
DropDownListValue = $"{s} - {orgNames[s]}"
}, "DropDownListValue", "DropDownListValue");
return View(new SampleViewModel())
}
your SomeAction
View:
@model YourAppNamespace.SampleViewModel
<h1>Hello Stranger</h1>
@using (Html.BeginForm())
{
@Html.DropDownList("DropDownListValue")
<input type="submit" value="Submit"/>
}
Note that:
The
DropDownList
helper used to create an HTML select list
requires aIEnumerable<SelectListItem>
, either explicitly or
implicitly. That is, you can pass theIEnumerable<SelectListItem>
explicitly to theDropDownList
helper or you can add the
IEnumerable<SelectListItem>
to theViewBag
using the same name for
theSelectListItem
as the model property.
We have used here implicit passing, that is we have used same name for SelectListItem
and ViewBag
(which is DropDownListValue
).
Then when you hit Submit
, you need HttpPost
method for SomeAction
:
[HttpPost]
public ActionResult SomeAction(SampleViewModel model)
{
var org = GetOrg(); //your org
var orgNames = GetOrgNames(); //your orgNames
//. . . Validation etc..
ViewBag.DropDownListValue = new SelectList(org.Select(s =>
new SampleViewModel
{
DropDownListValue = $"{s} - {orgNames[s]}"
}, "DropDownListValue", "DropDownListValue", model.DropDownListValue);
var doSomething = model.DropDownListValue; //Your selected value from DropDownList
return View(model)
}
References: DotNetFiddle Example,
Using the DropDownList Helper with ASP.NET MVC
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53479896%2fpass-dropdownlist-value-to-controller%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
It will be better if you create ViewModel for your View:
public class SampleViewModel
{
public string DropDownListValue { get; set; }
}
then in your controller's get method:
public ActionResult SomeAction()
{
var org = GetOrg(); //your org
var orgNames = GetOrgNames(); //your orgNames
// . . .
ViewBag.DropDownListValue = new SelectList(org.Select(s =>
new SampleViewModel
{
DropDownListValue = $"{s} - {orgNames[s]}"
}, "DropDownListValue", "DropDownListValue");
return View(new SampleViewModel())
}
your SomeAction
View:
@model YourAppNamespace.SampleViewModel
<h1>Hello Stranger</h1>
@using (Html.BeginForm())
{
@Html.DropDownList("DropDownListValue")
<input type="submit" value="Submit"/>
}
Note that:
The
DropDownList
helper used to create an HTML select list
requires aIEnumerable<SelectListItem>
, either explicitly or
implicitly. That is, you can pass theIEnumerable<SelectListItem>
explicitly to theDropDownList
helper or you can add the
IEnumerable<SelectListItem>
to theViewBag
using the same name for
theSelectListItem
as the model property.
We have used here implicit passing, that is we have used same name for SelectListItem
and ViewBag
(which is DropDownListValue
).
Then when you hit Submit
, you need HttpPost
method for SomeAction
:
[HttpPost]
public ActionResult SomeAction(SampleViewModel model)
{
var org = GetOrg(); //your org
var orgNames = GetOrgNames(); //your orgNames
//. . . Validation etc..
ViewBag.DropDownListValue = new SelectList(org.Select(s =>
new SampleViewModel
{
DropDownListValue = $"{s} - {orgNames[s]}"
}, "DropDownListValue", "DropDownListValue", model.DropDownListValue);
var doSomething = model.DropDownListValue; //Your selected value from DropDownList
return View(model)
}
References: DotNetFiddle Example,
Using the DropDownList Helper with ASP.NET MVC
add a comment |
It will be better if you create ViewModel for your View:
public class SampleViewModel
{
public string DropDownListValue { get; set; }
}
then in your controller's get method:
public ActionResult SomeAction()
{
var org = GetOrg(); //your org
var orgNames = GetOrgNames(); //your orgNames
// . . .
ViewBag.DropDownListValue = new SelectList(org.Select(s =>
new SampleViewModel
{
DropDownListValue = $"{s} - {orgNames[s]}"
}, "DropDownListValue", "DropDownListValue");
return View(new SampleViewModel())
}
your SomeAction
View:
@model YourAppNamespace.SampleViewModel
<h1>Hello Stranger</h1>
@using (Html.BeginForm())
{
@Html.DropDownList("DropDownListValue")
<input type="submit" value="Submit"/>
}
Note that:
The
DropDownList
helper used to create an HTML select list
requires aIEnumerable<SelectListItem>
, either explicitly or
implicitly. That is, you can pass theIEnumerable<SelectListItem>
explicitly to theDropDownList
helper or you can add the
IEnumerable<SelectListItem>
to theViewBag
using the same name for
theSelectListItem
as the model property.
We have used here implicit passing, that is we have used same name for SelectListItem
and ViewBag
(which is DropDownListValue
).
Then when you hit Submit
, you need HttpPost
method for SomeAction
:
[HttpPost]
public ActionResult SomeAction(SampleViewModel model)
{
var org = GetOrg(); //your org
var orgNames = GetOrgNames(); //your orgNames
//. . . Validation etc..
ViewBag.DropDownListValue = new SelectList(org.Select(s =>
new SampleViewModel
{
DropDownListValue = $"{s} - {orgNames[s]}"
}, "DropDownListValue", "DropDownListValue", model.DropDownListValue);
var doSomething = model.DropDownListValue; //Your selected value from DropDownList
return View(model)
}
References: DotNetFiddle Example,
Using the DropDownList Helper with ASP.NET MVC
add a comment |
It will be better if you create ViewModel for your View:
public class SampleViewModel
{
public string DropDownListValue { get; set; }
}
then in your controller's get method:
public ActionResult SomeAction()
{
var org = GetOrg(); //your org
var orgNames = GetOrgNames(); //your orgNames
// . . .
ViewBag.DropDownListValue = new SelectList(org.Select(s =>
new SampleViewModel
{
DropDownListValue = $"{s} - {orgNames[s]}"
}, "DropDownListValue", "DropDownListValue");
return View(new SampleViewModel())
}
your SomeAction
View:
@model YourAppNamespace.SampleViewModel
<h1>Hello Stranger</h1>
@using (Html.BeginForm())
{
@Html.DropDownList("DropDownListValue")
<input type="submit" value="Submit"/>
}
Note that:
The
DropDownList
helper used to create an HTML select list
requires aIEnumerable<SelectListItem>
, either explicitly or
implicitly. That is, you can pass theIEnumerable<SelectListItem>
explicitly to theDropDownList
helper or you can add the
IEnumerable<SelectListItem>
to theViewBag
using the same name for
theSelectListItem
as the model property.
We have used here implicit passing, that is we have used same name for SelectListItem
and ViewBag
(which is DropDownListValue
).
Then when you hit Submit
, you need HttpPost
method for SomeAction
:
[HttpPost]
public ActionResult SomeAction(SampleViewModel model)
{
var org = GetOrg(); //your org
var orgNames = GetOrgNames(); //your orgNames
//. . . Validation etc..
ViewBag.DropDownListValue = new SelectList(org.Select(s =>
new SampleViewModel
{
DropDownListValue = $"{s} - {orgNames[s]}"
}, "DropDownListValue", "DropDownListValue", model.DropDownListValue);
var doSomething = model.DropDownListValue; //Your selected value from DropDownList
return View(model)
}
References: DotNetFiddle Example,
Using the DropDownList Helper with ASP.NET MVC
It will be better if you create ViewModel for your View:
public class SampleViewModel
{
public string DropDownListValue { get; set; }
}
then in your controller's get method:
public ActionResult SomeAction()
{
var org = GetOrg(); //your org
var orgNames = GetOrgNames(); //your orgNames
// . . .
ViewBag.DropDownListValue = new SelectList(org.Select(s =>
new SampleViewModel
{
DropDownListValue = $"{s} - {orgNames[s]}"
}, "DropDownListValue", "DropDownListValue");
return View(new SampleViewModel())
}
your SomeAction
View:
@model YourAppNamespace.SampleViewModel
<h1>Hello Stranger</h1>
@using (Html.BeginForm())
{
@Html.DropDownList("DropDownListValue")
<input type="submit" value="Submit"/>
}
Note that:
The
DropDownList
helper used to create an HTML select list
requires aIEnumerable<SelectListItem>
, either explicitly or
implicitly. That is, you can pass theIEnumerable<SelectListItem>
explicitly to theDropDownList
helper or you can add the
IEnumerable<SelectListItem>
to theViewBag
using the same name for
theSelectListItem
as the model property.
We have used here implicit passing, that is we have used same name for SelectListItem
and ViewBag
(which is DropDownListValue
).
Then when you hit Submit
, you need HttpPost
method for SomeAction
:
[HttpPost]
public ActionResult SomeAction(SampleViewModel model)
{
var org = GetOrg(); //your org
var orgNames = GetOrgNames(); //your orgNames
//. . . Validation etc..
ViewBag.DropDownListValue = new SelectList(org.Select(s =>
new SampleViewModel
{
DropDownListValue = $"{s} - {orgNames[s]}"
}, "DropDownListValue", "DropDownListValue", model.DropDownListValue);
var doSomething = model.DropDownListValue; //Your selected value from DropDownList
return View(model)
}
References: DotNetFiddle Example,
Using the DropDownList Helper with ASP.NET MVC
edited Nov 26 '18 at 12:28
answered Nov 26 '18 at 12:15


SeMSeM
4,66011631
4,66011631
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53479896%2fpass-dropdownlist-value-to-controller%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
ktAAcKsBsFAz1Kad91DkDp epJVPigtr6lHTa0n9St,19Uy3 CPaTGZouNNPrdmXnGbs,LF5czR 0M 4nGBlWahfevQO661FsVr Roc
How about taking a tutorial?
– Reniuz
Nov 26 '18 at 11:18
I would suggest using a DropDownListFor for this. If set on this, I would go with calling a javasript function and using jquery to get the value. Passing it to the controller using an ajax.
– JamesS
Nov 26 '18 at 11:20
Is there any easyer way to do it?
– Giorgi Asanidze
Nov 26 '18 at 11:39
Possible duplicate of How to get DropDownList SelectedValue in Controller in MVC
– Tetsuya Yamamoto
Nov 27 '18 at 1:29