Check status of the server continuously
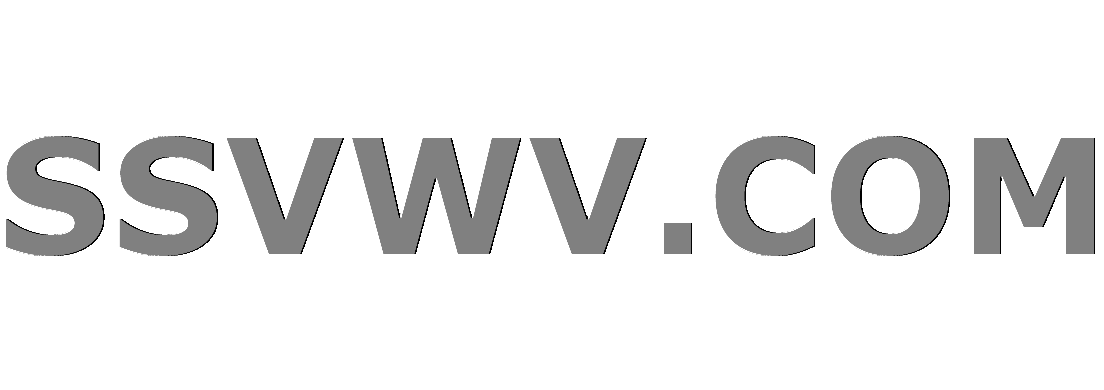
Multi tool use
up vote
3
down vote
favorite
I have C# based client-server architecture. The client will connect to server and exchange data.
I need to check the server status continuously and if the server goes down (removed from the network or shut down) the client needs to indicate that.
If I am using Ping
utility in the client program, which will be the best method in terms of performance i.e, monitor via a separate thread or through a background class?
public static bool GetPingResponse(string IpAddress, int timeout = 3000)
{
var ping = new Ping();
var reply = ping.Send(IpAddress, timeout);
if (reply.Status == IPStatus.Success)
{
return true;
}
else
{
return false;
}
}
Is there any other option better than Ping in terms of performance and consuming the resource?
c# client-server
add a comment |
up vote
3
down vote
favorite
I have C# based client-server architecture. The client will connect to server and exchange data.
I need to check the server status continuously and if the server goes down (removed from the network or shut down) the client needs to indicate that.
If I am using Ping
utility in the client program, which will be the best method in terms of performance i.e, monitor via a separate thread or through a background class?
public static bool GetPingResponse(string IpAddress, int timeout = 3000)
{
var ping = new Ping();
var reply = ping.Send(IpAddress, timeout);
if (reply.Status == IPStatus.Success)
{
return true;
}
else
{
return false;
}
}
Is there any other option better than Ping in terms of performance and consuming the resource?
c# client-server
How you connect to server? Are you using tcp?
– Adil
Nov 17 '12 at 13:16
yes tcp only.. But i need check the server powerup status. not only the tcp connection status.
– Olivarsham
Nov 17 '12 at 13:18
It might be worth thinking about whether you really need to know the server hardware is powered up, or whether you need to know whether a particular service is running on the server.
– Mike Sherrill 'Cat Recall'
Nov 17 '12 at 13:24
2
Even if you have continual response from the server, it doesn't mean that the server will be able to respond when you actually send a request to it - it could fail after the last "ping" and at any time up until it actually manages to send a response. You need to write code to cope with this situation anyway. As such, any pinging behaviour is just overhead that doesn't resolve any situation (you may still decide to do it to give hints to the user, but as I say, you need to write the other code as well)
– Damien_The_Unbeliever
Nov 17 '12 at 13:26
add a comment |
up vote
3
down vote
favorite
up vote
3
down vote
favorite
I have C# based client-server architecture. The client will connect to server and exchange data.
I need to check the server status continuously and if the server goes down (removed from the network or shut down) the client needs to indicate that.
If I am using Ping
utility in the client program, which will be the best method in terms of performance i.e, monitor via a separate thread or through a background class?
public static bool GetPingResponse(string IpAddress, int timeout = 3000)
{
var ping = new Ping();
var reply = ping.Send(IpAddress, timeout);
if (reply.Status == IPStatus.Success)
{
return true;
}
else
{
return false;
}
}
Is there any other option better than Ping in terms of performance and consuming the resource?
c# client-server
I have C# based client-server architecture. The client will connect to server and exchange data.
I need to check the server status continuously and if the server goes down (removed from the network or shut down) the client needs to indicate that.
If I am using Ping
utility in the client program, which will be the best method in terms of performance i.e, monitor via a separate thread or through a background class?
public static bool GetPingResponse(string IpAddress, int timeout = 3000)
{
var ping = new Ping();
var reply = ping.Send(IpAddress, timeout);
if (reply.Status == IPStatus.Success)
{
return true;
}
else
{
return false;
}
}
Is there any other option better than Ping in terms of performance and consuming the resource?
c# client-server
c# client-server
edited Nov 19 at 23:40


Hille
856622
856622
asked Nov 17 '12 at 13:15
Olivarsham
97241742
97241742
How you connect to server? Are you using tcp?
– Adil
Nov 17 '12 at 13:16
yes tcp only.. But i need check the server powerup status. not only the tcp connection status.
– Olivarsham
Nov 17 '12 at 13:18
It might be worth thinking about whether you really need to know the server hardware is powered up, or whether you need to know whether a particular service is running on the server.
– Mike Sherrill 'Cat Recall'
Nov 17 '12 at 13:24
2
Even if you have continual response from the server, it doesn't mean that the server will be able to respond when you actually send a request to it - it could fail after the last "ping" and at any time up until it actually manages to send a response. You need to write code to cope with this situation anyway. As such, any pinging behaviour is just overhead that doesn't resolve any situation (you may still decide to do it to give hints to the user, but as I say, you need to write the other code as well)
– Damien_The_Unbeliever
Nov 17 '12 at 13:26
add a comment |
How you connect to server? Are you using tcp?
– Adil
Nov 17 '12 at 13:16
yes tcp only.. But i need check the server powerup status. not only the tcp connection status.
– Olivarsham
Nov 17 '12 at 13:18
It might be worth thinking about whether you really need to know the server hardware is powered up, or whether you need to know whether a particular service is running on the server.
– Mike Sherrill 'Cat Recall'
Nov 17 '12 at 13:24
2
Even if you have continual response from the server, it doesn't mean that the server will be able to respond when you actually send a request to it - it could fail after the last "ping" and at any time up until it actually manages to send a response. You need to write code to cope with this situation anyway. As such, any pinging behaviour is just overhead that doesn't resolve any situation (you may still decide to do it to give hints to the user, but as I say, you need to write the other code as well)
– Damien_The_Unbeliever
Nov 17 '12 at 13:26
How you connect to server? Are you using tcp?
– Adil
Nov 17 '12 at 13:16
How you connect to server? Are you using tcp?
– Adil
Nov 17 '12 at 13:16
yes tcp only.. But i need check the server powerup status. not only the tcp connection status.
– Olivarsham
Nov 17 '12 at 13:18
yes tcp only.. But i need check the server powerup status. not only the tcp connection status.
– Olivarsham
Nov 17 '12 at 13:18
It might be worth thinking about whether you really need to know the server hardware is powered up, or whether you need to know whether a particular service is running on the server.
– Mike Sherrill 'Cat Recall'
Nov 17 '12 at 13:24
It might be worth thinking about whether you really need to know the server hardware is powered up, or whether you need to know whether a particular service is running on the server.
– Mike Sherrill 'Cat Recall'
Nov 17 '12 at 13:24
2
2
Even if you have continual response from the server, it doesn't mean that the server will be able to respond when you actually send a request to it - it could fail after the last "ping" and at any time up until it actually manages to send a response. You need to write code to cope with this situation anyway. As such, any pinging behaviour is just overhead that doesn't resolve any situation (you may still decide to do it to give hints to the user, but as I say, you need to write the other code as well)
– Damien_The_Unbeliever
Nov 17 '12 at 13:26
Even if you have continual response from the server, it doesn't mean that the server will be able to respond when you actually send a request to it - it could fail after the last "ping" and at any time up until it actually manages to send a response. You need to write code to cope with this situation anyway. As such, any pinging behaviour is just overhead that doesn't resolve any situation (you may still decide to do it to give hints to the user, but as I say, you need to write the other code as well)
– Damien_The_Unbeliever
Nov 17 '12 at 13:26
add a comment |
1 Answer
1
active
oldest
votes
up vote
3
down vote
accepted
You'll want to make sure your call is representative of "being available". For example, if it's an http server, making a ping request just says it's reachable via the network. The web server could be down but the network stack is up. If that's the case, make a call to something that exercises more of the stack like http. If there's backend databases, the call could go through to the database server. It all depends on your definition of "available". If the point is to say the application is available, you should look into a call that exercises a path through the full stack.
It also depends on what the server has available and what's open via firewalls between the clients and the servers.
Ping uses ICMP which some sites choose to block via firewalls. If that's open, it's a cheap call with little overhead.
If that's blocked and it's a web server for example over http, you could have a cheap endpoint on the web server that you could call via http client in C#.
You'll want to poll on a background thread probably with a timer. Look into the BackgroundWorker class or ThreadPool.QueueUserWorkitem.
Finally, you could look into a monitoring solution - there's many out there which could monitor multiple facets of your server(s). If you go that route, the client could simply query the monitoring solution which might be better since you wouldn't have n clients polling your servers - just one monitoring stack.
On a side note, the last block of code can be simplified down to:
return reply.Status == IPStatus.Success;
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f13430926%2fcheck-status-of-the-server-continuously%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
3
down vote
accepted
You'll want to make sure your call is representative of "being available". For example, if it's an http server, making a ping request just says it's reachable via the network. The web server could be down but the network stack is up. If that's the case, make a call to something that exercises more of the stack like http. If there's backend databases, the call could go through to the database server. It all depends on your definition of "available". If the point is to say the application is available, you should look into a call that exercises a path through the full stack.
It also depends on what the server has available and what's open via firewalls between the clients and the servers.
Ping uses ICMP which some sites choose to block via firewalls. If that's open, it's a cheap call with little overhead.
If that's blocked and it's a web server for example over http, you could have a cheap endpoint on the web server that you could call via http client in C#.
You'll want to poll on a background thread probably with a timer. Look into the BackgroundWorker class or ThreadPool.QueueUserWorkitem.
Finally, you could look into a monitoring solution - there's many out there which could monitor multiple facets of your server(s). If you go that route, the client could simply query the monitoring solution which might be better since you wouldn't have n clients polling your servers - just one monitoring stack.
On a side note, the last block of code can be simplified down to:
return reply.Status == IPStatus.Success;
add a comment |
up vote
3
down vote
accepted
You'll want to make sure your call is representative of "being available". For example, if it's an http server, making a ping request just says it's reachable via the network. The web server could be down but the network stack is up. If that's the case, make a call to something that exercises more of the stack like http. If there's backend databases, the call could go through to the database server. It all depends on your definition of "available". If the point is to say the application is available, you should look into a call that exercises a path through the full stack.
It also depends on what the server has available and what's open via firewalls between the clients and the servers.
Ping uses ICMP which some sites choose to block via firewalls. If that's open, it's a cheap call with little overhead.
If that's blocked and it's a web server for example over http, you could have a cheap endpoint on the web server that you could call via http client in C#.
You'll want to poll on a background thread probably with a timer. Look into the BackgroundWorker class or ThreadPool.QueueUserWorkitem.
Finally, you could look into a monitoring solution - there's many out there which could monitor multiple facets of your server(s). If you go that route, the client could simply query the monitoring solution which might be better since you wouldn't have n clients polling your servers - just one monitoring stack.
On a side note, the last block of code can be simplified down to:
return reply.Status == IPStatus.Success;
add a comment |
up vote
3
down vote
accepted
up vote
3
down vote
accepted
You'll want to make sure your call is representative of "being available". For example, if it's an http server, making a ping request just says it's reachable via the network. The web server could be down but the network stack is up. If that's the case, make a call to something that exercises more of the stack like http. If there's backend databases, the call could go through to the database server. It all depends on your definition of "available". If the point is to say the application is available, you should look into a call that exercises a path through the full stack.
It also depends on what the server has available and what's open via firewalls between the clients and the servers.
Ping uses ICMP which some sites choose to block via firewalls. If that's open, it's a cheap call with little overhead.
If that's blocked and it's a web server for example over http, you could have a cheap endpoint on the web server that you could call via http client in C#.
You'll want to poll on a background thread probably with a timer. Look into the BackgroundWorker class or ThreadPool.QueueUserWorkitem.
Finally, you could look into a monitoring solution - there's many out there which could monitor multiple facets of your server(s). If you go that route, the client could simply query the monitoring solution which might be better since you wouldn't have n clients polling your servers - just one monitoring stack.
On a side note, the last block of code can be simplified down to:
return reply.Status == IPStatus.Success;
You'll want to make sure your call is representative of "being available". For example, if it's an http server, making a ping request just says it's reachable via the network. The web server could be down but the network stack is up. If that's the case, make a call to something that exercises more of the stack like http. If there's backend databases, the call could go through to the database server. It all depends on your definition of "available". If the point is to say the application is available, you should look into a call that exercises a path through the full stack.
It also depends on what the server has available and what's open via firewalls between the clients and the servers.
Ping uses ICMP which some sites choose to block via firewalls. If that's open, it's a cheap call with little overhead.
If that's blocked and it's a web server for example over http, you could have a cheap endpoint on the web server that you could call via http client in C#.
You'll want to poll on a background thread probably with a timer. Look into the BackgroundWorker class or ThreadPool.QueueUserWorkitem.
Finally, you could look into a monitoring solution - there's many out there which could monitor multiple facets of your server(s). If you go that route, the client could simply query the monitoring solution which might be better since you wouldn't have n clients polling your servers - just one monitoring stack.
On a side note, the last block of code can be simplified down to:
return reply.Status == IPStatus.Success;
edited Nov 17 '12 at 13:42
answered Nov 17 '12 at 13:21


bryanmac
34.5k97489
34.5k97489
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f13430926%2fcheck-status-of-the-server-continuously%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
QSMuVO9hZ5,7,mmLRR0nnYivrIRcpYi8ItEpqamMnidXl4,oNY7vWq CL,6x1,t8LpJ2MdUVhlxAt6v,uo5M
How you connect to server? Are you using tcp?
– Adil
Nov 17 '12 at 13:16
yes tcp only.. But i need check the server powerup status. not only the tcp connection status.
– Olivarsham
Nov 17 '12 at 13:18
It might be worth thinking about whether you really need to know the server hardware is powered up, or whether you need to know whether a particular service is running on the server.
– Mike Sherrill 'Cat Recall'
Nov 17 '12 at 13:24
2
Even if you have continual response from the server, it doesn't mean that the server will be able to respond when you actually send a request to it - it could fail after the last "ping" and at any time up until it actually manages to send a response. You need to write code to cope with this situation anyway. As such, any pinging behaviour is just overhead that doesn't resolve any situation (you may still decide to do it to give hints to the user, but as I say, you need to write the other code as well)
– Damien_The_Unbeliever
Nov 17 '12 at 13:26