calculate business days including holidays
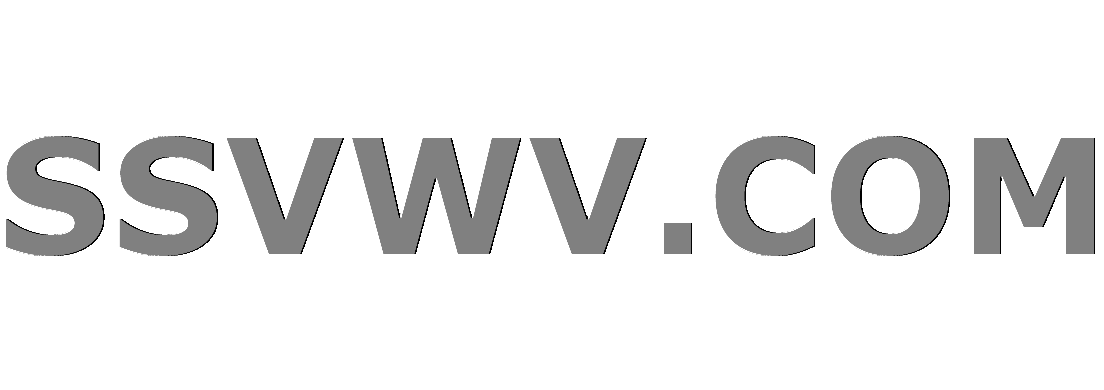
Multi tool use
i need to calculate the business days between two dates.
ex : we have holiday(in USA) on july4th. so if my dates are
date1 = 07/03/2012
date2 = 07/06/2012
no of business days b/w these dates should be 1 since july4th is holiday.
i have a below method to calclulate the business days which will only counts week ends but not holidays.
is there any way to calculate holidays also....please help me on this.
public static int getWorkingDaysBetweenTwoDates(Date startDate, Date endDate) {
Calendar startCal;
Calendar endCal;
startCal = Calendar.getInstance();
startCal.setTime(startDate);
endCal = Calendar.getInstance();
endCal.setTime(endDate);
int workDays = 0;
//Return 0 if start and end are the same
if (startCal.getTimeInMillis() == endCal.getTimeInMillis()) {
return 0;
}
if (startCal.getTimeInMillis() > endCal.getTimeInMillis()) {
startCal.setTime(endDate);
endCal.setTime(startDate);
}
do {
startCal.add(Calendar.DAY_OF_MONTH, 1);
if (startCal.get(Calendar.DAY_OF_WEEK) != Calendar.SATURDAY
&& startCal.get(Calendar.DAY_OF_WEEK) != Calendar.SUNDAY) {
++workDays;
}
} while (startCal.getTimeInMillis() < endCal.getTimeInMillis());
return workDays;
}
java date calendar
add a comment |
i need to calculate the business days between two dates.
ex : we have holiday(in USA) on july4th. so if my dates are
date1 = 07/03/2012
date2 = 07/06/2012
no of business days b/w these dates should be 1 since july4th is holiday.
i have a below method to calclulate the business days which will only counts week ends but not holidays.
is there any way to calculate holidays also....please help me on this.
public static int getWorkingDaysBetweenTwoDates(Date startDate, Date endDate) {
Calendar startCal;
Calendar endCal;
startCal = Calendar.getInstance();
startCal.setTime(startDate);
endCal = Calendar.getInstance();
endCal.setTime(endDate);
int workDays = 0;
//Return 0 if start and end are the same
if (startCal.getTimeInMillis() == endCal.getTimeInMillis()) {
return 0;
}
if (startCal.getTimeInMillis() > endCal.getTimeInMillis()) {
startCal.setTime(endDate);
endCal.setTime(startDate);
}
do {
startCal.add(Calendar.DAY_OF_MONTH, 1);
if (startCal.get(Calendar.DAY_OF_WEEK) != Calendar.SATURDAY
&& startCal.get(Calendar.DAY_OF_WEEK) != Calendar.SUNDAY) {
++workDays;
}
} while (startCal.getTimeInMillis() < endCal.getTimeInMillis());
return workDays;
}
java date calendar
You need to keep track of the knowledge of which days are holidays manually, AFAIK there's no built in function for that. Also, who uses do-while in java these days??
– TS-
Jun 1 '12 at 16:33
First of all you will need a list of all the holidays in a year beacuse no java class (i.e.Locale
specific class) provides this functionality. Then you will need to find how many from this list fall between the dates specified which is fairly straight-forward. Then you can delete that many days from the result of your above code.
– Surender Thakran
Jun 1 '12 at 16:37
thanks for ur reply. let's say if i have a list which contains all the holidays, can u please suggest me how to use that list or how to check that list dates in the condition.
– ran
Jun 1 '12 at 16:38
Duplicate of the Question Date api for managing off days in an year? but this one has better answers.
– Basil Bourque
Feb 17 '17 at 3:34
add a comment |
i need to calculate the business days between two dates.
ex : we have holiday(in USA) on july4th. so if my dates are
date1 = 07/03/2012
date2 = 07/06/2012
no of business days b/w these dates should be 1 since july4th is holiday.
i have a below method to calclulate the business days which will only counts week ends but not holidays.
is there any way to calculate holidays also....please help me on this.
public static int getWorkingDaysBetweenTwoDates(Date startDate, Date endDate) {
Calendar startCal;
Calendar endCal;
startCal = Calendar.getInstance();
startCal.setTime(startDate);
endCal = Calendar.getInstance();
endCal.setTime(endDate);
int workDays = 0;
//Return 0 if start and end are the same
if (startCal.getTimeInMillis() == endCal.getTimeInMillis()) {
return 0;
}
if (startCal.getTimeInMillis() > endCal.getTimeInMillis()) {
startCal.setTime(endDate);
endCal.setTime(startDate);
}
do {
startCal.add(Calendar.DAY_OF_MONTH, 1);
if (startCal.get(Calendar.DAY_OF_WEEK) != Calendar.SATURDAY
&& startCal.get(Calendar.DAY_OF_WEEK) != Calendar.SUNDAY) {
++workDays;
}
} while (startCal.getTimeInMillis() < endCal.getTimeInMillis());
return workDays;
}
java date calendar
i need to calculate the business days between two dates.
ex : we have holiday(in USA) on july4th. so if my dates are
date1 = 07/03/2012
date2 = 07/06/2012
no of business days b/w these dates should be 1 since july4th is holiday.
i have a below method to calclulate the business days which will only counts week ends but not holidays.
is there any way to calculate holidays also....please help me on this.
public static int getWorkingDaysBetweenTwoDates(Date startDate, Date endDate) {
Calendar startCal;
Calendar endCal;
startCal = Calendar.getInstance();
startCal.setTime(startDate);
endCal = Calendar.getInstance();
endCal.setTime(endDate);
int workDays = 0;
//Return 0 if start and end are the same
if (startCal.getTimeInMillis() == endCal.getTimeInMillis()) {
return 0;
}
if (startCal.getTimeInMillis() > endCal.getTimeInMillis()) {
startCal.setTime(endDate);
endCal.setTime(startDate);
}
do {
startCal.add(Calendar.DAY_OF_MONTH, 1);
if (startCal.get(Calendar.DAY_OF_WEEK) != Calendar.SATURDAY
&& startCal.get(Calendar.DAY_OF_WEEK) != Calendar.SUNDAY) {
++workDays;
}
} while (startCal.getTimeInMillis() < endCal.getTimeInMillis());
return workDays;
}
java date calendar
java date calendar
asked Jun 1 '12 at 16:31
ranran
2324613
2324613
You need to keep track of the knowledge of which days are holidays manually, AFAIK there's no built in function for that. Also, who uses do-while in java these days??
– TS-
Jun 1 '12 at 16:33
First of all you will need a list of all the holidays in a year beacuse no java class (i.e.Locale
specific class) provides this functionality. Then you will need to find how many from this list fall between the dates specified which is fairly straight-forward. Then you can delete that many days from the result of your above code.
– Surender Thakran
Jun 1 '12 at 16:37
thanks for ur reply. let's say if i have a list which contains all the holidays, can u please suggest me how to use that list or how to check that list dates in the condition.
– ran
Jun 1 '12 at 16:38
Duplicate of the Question Date api for managing off days in an year? but this one has better answers.
– Basil Bourque
Feb 17 '17 at 3:34
add a comment |
You need to keep track of the knowledge of which days are holidays manually, AFAIK there's no built in function for that. Also, who uses do-while in java these days??
– TS-
Jun 1 '12 at 16:33
First of all you will need a list of all the holidays in a year beacuse no java class (i.e.Locale
specific class) provides this functionality. Then you will need to find how many from this list fall between the dates specified which is fairly straight-forward. Then you can delete that many days from the result of your above code.
– Surender Thakran
Jun 1 '12 at 16:37
thanks for ur reply. let's say if i have a list which contains all the holidays, can u please suggest me how to use that list or how to check that list dates in the condition.
– ran
Jun 1 '12 at 16:38
Duplicate of the Question Date api for managing off days in an year? but this one has better answers.
– Basil Bourque
Feb 17 '17 at 3:34
You need to keep track of the knowledge of which days are holidays manually, AFAIK there's no built in function for that. Also, who uses do-while in java these days??
– TS-
Jun 1 '12 at 16:33
You need to keep track of the knowledge of which days are holidays manually, AFAIK there's no built in function for that. Also, who uses do-while in java these days??
– TS-
Jun 1 '12 at 16:33
First of all you will need a list of all the holidays in a year beacuse no java class (i.e.
Locale
specific class) provides this functionality. Then you will need to find how many from this list fall between the dates specified which is fairly straight-forward. Then you can delete that many days from the result of your above code.– Surender Thakran
Jun 1 '12 at 16:37
First of all you will need a list of all the holidays in a year beacuse no java class (i.e.
Locale
specific class) provides this functionality. Then you will need to find how many from this list fall between the dates specified which is fairly straight-forward. Then you can delete that many days from the result of your above code.– Surender Thakran
Jun 1 '12 at 16:37
thanks for ur reply. let's say if i have a list which contains all the holidays, can u please suggest me how to use that list or how to check that list dates in the condition.
– ran
Jun 1 '12 at 16:38
thanks for ur reply. let's say if i have a list which contains all the holidays, can u please suggest me how to use that list or how to check that list dates in the condition.
– ran
Jun 1 '12 at 16:38
Duplicate of the Question Date api for managing off days in an year? but this one has better answers.
– Basil Bourque
Feb 17 '17 at 3:34
Duplicate of the Question Date api for managing off days in an year? but this one has better answers.
– Basil Bourque
Feb 17 '17 at 3:34
add a comment |
5 Answers
5
active
oldest
votes
Let's pretend you have a list containing all the holidays, as you mentioned.
ArrayList<Integer> holidays = ...
Just add a condition to your if
condition in your do-while
:
do {
startCal.add(Calendar.DAY_OF_MONTH, 1);
if (startCal.get(Calendar.DAY_OF_WEEK) != Calendar.SATURDAY
&& startCal.get(Calendar.DAY_OF_WEEK) != Calendar.SUNDAY
&& !holidays.contains((Integer) startCal.get(Calendar.DAY_OF_YEAR))) {
++workDays;
}
} while (startCal.getTimeInMillis() < endCal.getTimeInMillis());
For simplicity's sake, I've assumed holiday
contains dates in the format identical to Calendar.DAY_OF_YEAR
.
thank you so much...i don't know how i missed this simple logic. but here instead of Calendar.DAY_OF_YEAR i am comparing it with the Calendar.getTime() which returns the full date. it working fine now
– ran
Jun 1 '12 at 19:20
Cheers, glad to be of service.
– SimplyPanda
Jun 1 '12 at 19:30
1
It's worth noting both examples here will calculate 1 day for the difference of 1 millisecons or more. Use a day_of_year and year comparison on the loop instead.
– Eddie
Oct 15 '12 at 12:17
add a comment |
Nager.Date
You can use the JSON API of Nager.Date project. It supports USA, Canada and Europe. The data available for every year you can save the information in your own database.
Example
//https://github.com/FasterXML/jackson-databind/
ObjectMapper mapper = new ObjectMapper();
MyValue value = mapper.readValue(new URL("http://date.nager.at/api/v1/get/US/2017"), PublicHoliday.class);
PublicHoliday.class
public class PublicHoliday
{
public String date;
public String localName;
public String name;
public String countryCode;
public Boolean fixed;
public Boolean countyOfficialHoliday;
public Boolean countyAdministrationHoliday;
public Boolean global;
public String counties;
public int launchYear;
}
Example JSON data retrieved.
[
{
"date": "2017-01-01",
"localName": "New Year's Day",
"name": "New Year's Day",
"countryCode": "US",
"fixed": true,
"countyOfficialHoliday": true,
"countyAdministrationHoliday": true,
"global": true,
"counties": null,
"launchYear": null
},
{
"date": "2017-01-16",
"localName": "Martin Luther King, Jr. Day",
"name": "Martin Luther King, Jr. Day",
"countryCode": "US",
"fixed": true,
"countyOfficialHoliday": true,
"countyAdministrationHoliday": true,
"global": true,
"counties": null,
"launchYear": null
},
{
"date": "2017-01-20",
"localName": "Inauguration Day",
"name": "Inauguration Day",
"countryCode": "US",
"fixed": true,
"countyOfficialHoliday": true,
"countyAdministrationHoliday": true,
"global": false,
"counties": [
"US-DC",
"US-LA",
"US-MD",
"US-VA"
],
"launchYear": null
},
{
"date": "2017-02-20",
"localName": "Washington's Birthday",
"name": "Presidents' Day",
"countryCode": "US",
"fixed": true,
"countyOfficialHoliday": true,
"countyAdministrationHoliday": true,
"global": true,
"counties": null,
"launchYear": null
},
{
"date": "2017-05-29",
"localName": "Memorial Day",
"name": "Memorial Day",
"countryCode": "US",
"fixed": true,
"countyOfficialHoliday": true,
"countyAdministrationHoliday": true,
"global": true,
"counties": null,
"launchYear": null
},
{
"date": "2017-07-04",
"localName": "Independence Day",
"name": "Independence Day",
"countryCode": "US",
"fixed": true,
"countyOfficialHoliday": true,
"countyAdministrationHoliday": true,
"global": true,
"counties": null,
"launchYear": null
},
{
"date": "2017-09-04",
"localName": "Labor Day",
"name": "Labor Day",
"countryCode": "US",
"fixed": true,
"countyOfficialHoliday": true,
"countyAdministrationHoliday": true,
"global": true,
"counties": null,
"launchYear": null
},
{
"date": "2017-09-09",
"localName": "Columbus Day",
"name": "Columbus Day",
"countryCode": "US",
"fixed": true,
"countyOfficialHoliday": true,
"countyAdministrationHoliday": true,
"global": false,
"counties": [
"US-AL",
"US-AZ",
"US-CO",
"US-CT",
"US-DC",
"US-GA",
"US-ID",
"US-IL",
"US-IN",
"US-IA",
"US-KS",
"US-KY",
"US-LA",
"US-ME",
"US-MD",
"US-MA",
"US-MS",
"US-MO",
"US-MT",
"US-NE",
"US-NH",
"US-NJ",
"US-NM",
"US-NY",
"US-NC",
"US-OH",
"US-OK",
"US-PA",
"US-RI",
"US-SC",
"US-TN",
"US-UT",
"US-VA",
"US-WV"
],
"launchYear": null
},
{
"date": "2017-11-10",
"localName": "Veterans Day",
"name": "Veterans Day",
"countryCode": "US",
"fixed": false,
"countyOfficialHoliday": true,
"countyAdministrationHoliday": true,
"global": true,
"counties": null,
"launchYear": null
},
{
"date": "2017-12-23",
"localName": "Thanksgiving Day",
"name": "Thanksgiving Day",
"countryCode": "US",
"fixed": true,
"countyOfficialHoliday": true,
"countyAdministrationHoliday": true,
"global": true,
"counties": null,
"launchYear": 1863
},
{
"date": "2017-12-25",
"localName": "Christmas Day",
"name": "Christmas Day",
"countryCode": "US",
"fixed": true,
"countyOfficialHoliday": true,
"countyAdministrationHoliday": true,
"global": true,
"counties": null,
"launchYear": null
}
]
1
You should follow the Java Naming Conventions: variable names always start with lowercase.
– MC Emperor
Jan 16 at 6:51
add a comment |
Since the accepted answer still uses the obsolete Calendar
class – here's my two cents using the new Java Date and Time API.
You have to get the dates of the holidays from somewhere, there is no standard Java library for it. That would be too localized anyway, since holidays heavily depends on your country and region. (except for widely known holidays, such as Christmas or Easter).
You could get them from a holiday API, for instance. In the code below I've hardcoded them as a List
of LocalDate
s.
Java 9
LocalDate startDate = LocalDate.of(2012, 3, 7);
LocalDate endDate = LocalDate.of(2012, 6, 7);
// I've hardcoded the holidays as LocalDates and put them in a List
final List<LocalDate> holidays = Arrays.asList(
LocalDate.of(2018, 7, 4)
);
List<LocalDate> allDates =
// Java 9 provides a method to return a stream with dates from the
// startdate to the given end date. Note that the end date itself is
// NOT included.
startDate.datesUntil(endDate)
// Retain all business days. Use static imports from
// java.time.DayOfWeek.*
.filter(t -> Stream.of(MONDAY, TUESDAY, WEDNESDAY, THURSDAY, FRIDAY)
.anyMatch(t.getDayOfWeek()::equals))
// Retain only dates not present in our holidays list
.filter(t -> !holidays.contains(t))
// Collect them into a List. If you only need to know the number of
// dates, you can also use .count()
.collect(Collectors.toList());
Java 8
The method LocalDate.datesUntil
is not available in Java 8, so you have to obtain a stream of all dates between those two dates in a different manner. We must first count the total number of days in between using the ChronoUnit.DAYS.between
method.
long numOfDaysBetween = ChronoUnit.DAYS.between(startDate, endDate);
Then we must generate a sequence of integers exactly as long as the number of days between the start and end date, and then create LocalDate
s from it, starting at the start date.
IntStream.iterate(0, i -> i + 1)
.limit(numOfDaysBetween)
.mapToObj(startDate::plusDays)
Now we have a Stream<LocalDate>
, and you can then use the remaining part of the Java 9 code.
add a comment |
I don't have any code samples or anything like that, but I did some searching for you and came across this Stack Overflow thread that has some links to web services that can return holiday dates for you, which may help you get to where you need to be: National holiday web service
The top answer in that thread links to this web service: http://www.holidaywebservice.com/
I'm not sure if using a web service for this type of thing is overkill or not, but surely there is a better way. I apologize, I am not the most experienced programmer so I can't help you as much as I'd like to.
1
It's not really overkill. You need to be getting the information from somewhere else as it's always subject to change.
– djechlin
Jun 1 '12 at 16:53
add a comment |
The fastest way I've found is using some math (borrowing from the SO post):
public static int totalBusinessDaysBetween(LocalDate start, LocalDate end) {
Objects.requireNonNull(start, "Start date must not be null");
Objects.requireNonNull(end, "End date must not be null");
long daysBetweenWithoutWeekends = calculateNumberOfDaysBetweenMinusWeekends(start, end);
final Set<LocalDate> holidayForYearRange = getUSFederalHolidayForYearRange(start.getYear(), end.getYear());
for (LocalDate localDate : holidayForYearRange) {
if (localDate.isAfter(start) && localDate.isBefore(end)) {
daysBetweenWithoutWeekends--;
}
}
return (int) daysBetweenWithoutWeekends;
}
And:
private static long calculateNumberOfDaysBetweenMinusWeekends(LocalDate start, LocalDate end) {
final DayOfWeek startW = start.getDayOfWeek();
final DayOfWeek endW = end.getDayOfWeek();
final long days = ChronoUnit.DAYS.between(start, end);
final long daysWithoutWeekends = days - 2 * ((days + startW.getValue()) / 7);
//adjust for starting and ending on a Sunday:
return daysWithoutWeekends + (startW == DayOfWeek.SUNDAY ? 1 : 0) + (endW == DayOfWeek.SUNDAY ? 1 : 0);
}
I put something more complete together here.
1
While this link may answer the question, it is better to include the essential parts of the answer here and provide the link for reference. Link-only answers can become invalid if the linked page changes. - From Review
– Nico Haase
Nov 29 '18 at 15:11
Thanks for the feedback.
– James Dunnam
Nov 29 '18 at 15:42
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f10854119%2fcalculate-business-days-including-holidays%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
5 Answers
5
active
oldest
votes
5 Answers
5
active
oldest
votes
active
oldest
votes
active
oldest
votes
Let's pretend you have a list containing all the holidays, as you mentioned.
ArrayList<Integer> holidays = ...
Just add a condition to your if
condition in your do-while
:
do {
startCal.add(Calendar.DAY_OF_MONTH, 1);
if (startCal.get(Calendar.DAY_OF_WEEK) != Calendar.SATURDAY
&& startCal.get(Calendar.DAY_OF_WEEK) != Calendar.SUNDAY
&& !holidays.contains((Integer) startCal.get(Calendar.DAY_OF_YEAR))) {
++workDays;
}
} while (startCal.getTimeInMillis() < endCal.getTimeInMillis());
For simplicity's sake, I've assumed holiday
contains dates in the format identical to Calendar.DAY_OF_YEAR
.
thank you so much...i don't know how i missed this simple logic. but here instead of Calendar.DAY_OF_YEAR i am comparing it with the Calendar.getTime() which returns the full date. it working fine now
– ran
Jun 1 '12 at 19:20
Cheers, glad to be of service.
– SimplyPanda
Jun 1 '12 at 19:30
1
It's worth noting both examples here will calculate 1 day for the difference of 1 millisecons or more. Use a day_of_year and year comparison on the loop instead.
– Eddie
Oct 15 '12 at 12:17
add a comment |
Let's pretend you have a list containing all the holidays, as you mentioned.
ArrayList<Integer> holidays = ...
Just add a condition to your if
condition in your do-while
:
do {
startCal.add(Calendar.DAY_OF_MONTH, 1);
if (startCal.get(Calendar.DAY_OF_WEEK) != Calendar.SATURDAY
&& startCal.get(Calendar.DAY_OF_WEEK) != Calendar.SUNDAY
&& !holidays.contains((Integer) startCal.get(Calendar.DAY_OF_YEAR))) {
++workDays;
}
} while (startCal.getTimeInMillis() < endCal.getTimeInMillis());
For simplicity's sake, I've assumed holiday
contains dates in the format identical to Calendar.DAY_OF_YEAR
.
thank you so much...i don't know how i missed this simple logic. but here instead of Calendar.DAY_OF_YEAR i am comparing it with the Calendar.getTime() which returns the full date. it working fine now
– ran
Jun 1 '12 at 19:20
Cheers, glad to be of service.
– SimplyPanda
Jun 1 '12 at 19:30
1
It's worth noting both examples here will calculate 1 day for the difference of 1 millisecons or more. Use a day_of_year and year comparison on the loop instead.
– Eddie
Oct 15 '12 at 12:17
add a comment |
Let's pretend you have a list containing all the holidays, as you mentioned.
ArrayList<Integer> holidays = ...
Just add a condition to your if
condition in your do-while
:
do {
startCal.add(Calendar.DAY_OF_MONTH, 1);
if (startCal.get(Calendar.DAY_OF_WEEK) != Calendar.SATURDAY
&& startCal.get(Calendar.DAY_OF_WEEK) != Calendar.SUNDAY
&& !holidays.contains((Integer) startCal.get(Calendar.DAY_OF_YEAR))) {
++workDays;
}
} while (startCal.getTimeInMillis() < endCal.getTimeInMillis());
For simplicity's sake, I've assumed holiday
contains dates in the format identical to Calendar.DAY_OF_YEAR
.
Let's pretend you have a list containing all the holidays, as you mentioned.
ArrayList<Integer> holidays = ...
Just add a condition to your if
condition in your do-while
:
do {
startCal.add(Calendar.DAY_OF_MONTH, 1);
if (startCal.get(Calendar.DAY_OF_WEEK) != Calendar.SATURDAY
&& startCal.get(Calendar.DAY_OF_WEEK) != Calendar.SUNDAY
&& !holidays.contains((Integer) startCal.get(Calendar.DAY_OF_YEAR))) {
++workDays;
}
} while (startCal.getTimeInMillis() < endCal.getTimeInMillis());
For simplicity's sake, I've assumed holiday
contains dates in the format identical to Calendar.DAY_OF_YEAR
.
answered Jun 1 '12 at 16:53


SimplyPandaSimplyPanda
685716
685716
thank you so much...i don't know how i missed this simple logic. but here instead of Calendar.DAY_OF_YEAR i am comparing it with the Calendar.getTime() which returns the full date. it working fine now
– ran
Jun 1 '12 at 19:20
Cheers, glad to be of service.
– SimplyPanda
Jun 1 '12 at 19:30
1
It's worth noting both examples here will calculate 1 day for the difference of 1 millisecons or more. Use a day_of_year and year comparison on the loop instead.
– Eddie
Oct 15 '12 at 12:17
add a comment |
thank you so much...i don't know how i missed this simple logic. but here instead of Calendar.DAY_OF_YEAR i am comparing it with the Calendar.getTime() which returns the full date. it working fine now
– ran
Jun 1 '12 at 19:20
Cheers, glad to be of service.
– SimplyPanda
Jun 1 '12 at 19:30
1
It's worth noting both examples here will calculate 1 day for the difference of 1 millisecons or more. Use a day_of_year and year comparison on the loop instead.
– Eddie
Oct 15 '12 at 12:17
thank you so much...i don't know how i missed this simple logic. but here instead of Calendar.DAY_OF_YEAR i am comparing it with the Calendar.getTime() which returns the full date. it working fine now
– ran
Jun 1 '12 at 19:20
thank you so much...i don't know how i missed this simple logic. but here instead of Calendar.DAY_OF_YEAR i am comparing it with the Calendar.getTime() which returns the full date. it working fine now
– ran
Jun 1 '12 at 19:20
Cheers, glad to be of service.
– SimplyPanda
Jun 1 '12 at 19:30
Cheers, glad to be of service.
– SimplyPanda
Jun 1 '12 at 19:30
1
1
It's worth noting both examples here will calculate 1 day for the difference of 1 millisecons or more. Use a day_of_year and year comparison on the loop instead.
– Eddie
Oct 15 '12 at 12:17
It's worth noting both examples here will calculate 1 day for the difference of 1 millisecons or more. Use a day_of_year and year comparison on the loop instead.
– Eddie
Oct 15 '12 at 12:17
add a comment |
Nager.Date
You can use the JSON API of Nager.Date project. It supports USA, Canada and Europe. The data available for every year you can save the information in your own database.
Example
//https://github.com/FasterXML/jackson-databind/
ObjectMapper mapper = new ObjectMapper();
MyValue value = mapper.readValue(new URL("http://date.nager.at/api/v1/get/US/2017"), PublicHoliday.class);
PublicHoliday.class
public class PublicHoliday
{
public String date;
public String localName;
public String name;
public String countryCode;
public Boolean fixed;
public Boolean countyOfficialHoliday;
public Boolean countyAdministrationHoliday;
public Boolean global;
public String counties;
public int launchYear;
}
Example JSON data retrieved.
[
{
"date": "2017-01-01",
"localName": "New Year's Day",
"name": "New Year's Day",
"countryCode": "US",
"fixed": true,
"countyOfficialHoliday": true,
"countyAdministrationHoliday": true,
"global": true,
"counties": null,
"launchYear": null
},
{
"date": "2017-01-16",
"localName": "Martin Luther King, Jr. Day",
"name": "Martin Luther King, Jr. Day",
"countryCode": "US",
"fixed": true,
"countyOfficialHoliday": true,
"countyAdministrationHoliday": true,
"global": true,
"counties": null,
"launchYear": null
},
{
"date": "2017-01-20",
"localName": "Inauguration Day",
"name": "Inauguration Day",
"countryCode": "US",
"fixed": true,
"countyOfficialHoliday": true,
"countyAdministrationHoliday": true,
"global": false,
"counties": [
"US-DC",
"US-LA",
"US-MD",
"US-VA"
],
"launchYear": null
},
{
"date": "2017-02-20",
"localName": "Washington's Birthday",
"name": "Presidents' Day",
"countryCode": "US",
"fixed": true,
"countyOfficialHoliday": true,
"countyAdministrationHoliday": true,
"global": true,
"counties": null,
"launchYear": null
},
{
"date": "2017-05-29",
"localName": "Memorial Day",
"name": "Memorial Day",
"countryCode": "US",
"fixed": true,
"countyOfficialHoliday": true,
"countyAdministrationHoliday": true,
"global": true,
"counties": null,
"launchYear": null
},
{
"date": "2017-07-04",
"localName": "Independence Day",
"name": "Independence Day",
"countryCode": "US",
"fixed": true,
"countyOfficialHoliday": true,
"countyAdministrationHoliday": true,
"global": true,
"counties": null,
"launchYear": null
},
{
"date": "2017-09-04",
"localName": "Labor Day",
"name": "Labor Day",
"countryCode": "US",
"fixed": true,
"countyOfficialHoliday": true,
"countyAdministrationHoliday": true,
"global": true,
"counties": null,
"launchYear": null
},
{
"date": "2017-09-09",
"localName": "Columbus Day",
"name": "Columbus Day",
"countryCode": "US",
"fixed": true,
"countyOfficialHoliday": true,
"countyAdministrationHoliday": true,
"global": false,
"counties": [
"US-AL",
"US-AZ",
"US-CO",
"US-CT",
"US-DC",
"US-GA",
"US-ID",
"US-IL",
"US-IN",
"US-IA",
"US-KS",
"US-KY",
"US-LA",
"US-ME",
"US-MD",
"US-MA",
"US-MS",
"US-MO",
"US-MT",
"US-NE",
"US-NH",
"US-NJ",
"US-NM",
"US-NY",
"US-NC",
"US-OH",
"US-OK",
"US-PA",
"US-RI",
"US-SC",
"US-TN",
"US-UT",
"US-VA",
"US-WV"
],
"launchYear": null
},
{
"date": "2017-11-10",
"localName": "Veterans Day",
"name": "Veterans Day",
"countryCode": "US",
"fixed": false,
"countyOfficialHoliday": true,
"countyAdministrationHoliday": true,
"global": true,
"counties": null,
"launchYear": null
},
{
"date": "2017-12-23",
"localName": "Thanksgiving Day",
"name": "Thanksgiving Day",
"countryCode": "US",
"fixed": true,
"countyOfficialHoliday": true,
"countyAdministrationHoliday": true,
"global": true,
"counties": null,
"launchYear": 1863
},
{
"date": "2017-12-25",
"localName": "Christmas Day",
"name": "Christmas Day",
"countryCode": "US",
"fixed": true,
"countyOfficialHoliday": true,
"countyAdministrationHoliday": true,
"global": true,
"counties": null,
"launchYear": null
}
]
1
You should follow the Java Naming Conventions: variable names always start with lowercase.
– MC Emperor
Jan 16 at 6:51
add a comment |
Nager.Date
You can use the JSON API of Nager.Date project. It supports USA, Canada and Europe. The data available for every year you can save the information in your own database.
Example
//https://github.com/FasterXML/jackson-databind/
ObjectMapper mapper = new ObjectMapper();
MyValue value = mapper.readValue(new URL("http://date.nager.at/api/v1/get/US/2017"), PublicHoliday.class);
PublicHoliday.class
public class PublicHoliday
{
public String date;
public String localName;
public String name;
public String countryCode;
public Boolean fixed;
public Boolean countyOfficialHoliday;
public Boolean countyAdministrationHoliday;
public Boolean global;
public String counties;
public int launchYear;
}
Example JSON data retrieved.
[
{
"date": "2017-01-01",
"localName": "New Year's Day",
"name": "New Year's Day",
"countryCode": "US",
"fixed": true,
"countyOfficialHoliday": true,
"countyAdministrationHoliday": true,
"global": true,
"counties": null,
"launchYear": null
},
{
"date": "2017-01-16",
"localName": "Martin Luther King, Jr. Day",
"name": "Martin Luther King, Jr. Day",
"countryCode": "US",
"fixed": true,
"countyOfficialHoliday": true,
"countyAdministrationHoliday": true,
"global": true,
"counties": null,
"launchYear": null
},
{
"date": "2017-01-20",
"localName": "Inauguration Day",
"name": "Inauguration Day",
"countryCode": "US",
"fixed": true,
"countyOfficialHoliday": true,
"countyAdministrationHoliday": true,
"global": false,
"counties": [
"US-DC",
"US-LA",
"US-MD",
"US-VA"
],
"launchYear": null
},
{
"date": "2017-02-20",
"localName": "Washington's Birthday",
"name": "Presidents' Day",
"countryCode": "US",
"fixed": true,
"countyOfficialHoliday": true,
"countyAdministrationHoliday": true,
"global": true,
"counties": null,
"launchYear": null
},
{
"date": "2017-05-29",
"localName": "Memorial Day",
"name": "Memorial Day",
"countryCode": "US",
"fixed": true,
"countyOfficialHoliday": true,
"countyAdministrationHoliday": true,
"global": true,
"counties": null,
"launchYear": null
},
{
"date": "2017-07-04",
"localName": "Independence Day",
"name": "Independence Day",
"countryCode": "US",
"fixed": true,
"countyOfficialHoliday": true,
"countyAdministrationHoliday": true,
"global": true,
"counties": null,
"launchYear": null
},
{
"date": "2017-09-04",
"localName": "Labor Day",
"name": "Labor Day",
"countryCode": "US",
"fixed": true,
"countyOfficialHoliday": true,
"countyAdministrationHoliday": true,
"global": true,
"counties": null,
"launchYear": null
},
{
"date": "2017-09-09",
"localName": "Columbus Day",
"name": "Columbus Day",
"countryCode": "US",
"fixed": true,
"countyOfficialHoliday": true,
"countyAdministrationHoliday": true,
"global": false,
"counties": [
"US-AL",
"US-AZ",
"US-CO",
"US-CT",
"US-DC",
"US-GA",
"US-ID",
"US-IL",
"US-IN",
"US-IA",
"US-KS",
"US-KY",
"US-LA",
"US-ME",
"US-MD",
"US-MA",
"US-MS",
"US-MO",
"US-MT",
"US-NE",
"US-NH",
"US-NJ",
"US-NM",
"US-NY",
"US-NC",
"US-OH",
"US-OK",
"US-PA",
"US-RI",
"US-SC",
"US-TN",
"US-UT",
"US-VA",
"US-WV"
],
"launchYear": null
},
{
"date": "2017-11-10",
"localName": "Veterans Day",
"name": "Veterans Day",
"countryCode": "US",
"fixed": false,
"countyOfficialHoliday": true,
"countyAdministrationHoliday": true,
"global": true,
"counties": null,
"launchYear": null
},
{
"date": "2017-12-23",
"localName": "Thanksgiving Day",
"name": "Thanksgiving Day",
"countryCode": "US",
"fixed": true,
"countyOfficialHoliday": true,
"countyAdministrationHoliday": true,
"global": true,
"counties": null,
"launchYear": 1863
},
{
"date": "2017-12-25",
"localName": "Christmas Day",
"name": "Christmas Day",
"countryCode": "US",
"fixed": true,
"countyOfficialHoliday": true,
"countyAdministrationHoliday": true,
"global": true,
"counties": null,
"launchYear": null
}
]
1
You should follow the Java Naming Conventions: variable names always start with lowercase.
– MC Emperor
Jan 16 at 6:51
add a comment |
Nager.Date
You can use the JSON API of Nager.Date project. It supports USA, Canada and Europe. The data available for every year you can save the information in your own database.
Example
//https://github.com/FasterXML/jackson-databind/
ObjectMapper mapper = new ObjectMapper();
MyValue value = mapper.readValue(new URL("http://date.nager.at/api/v1/get/US/2017"), PublicHoliday.class);
PublicHoliday.class
public class PublicHoliday
{
public String date;
public String localName;
public String name;
public String countryCode;
public Boolean fixed;
public Boolean countyOfficialHoliday;
public Boolean countyAdministrationHoliday;
public Boolean global;
public String counties;
public int launchYear;
}
Example JSON data retrieved.
[
{
"date": "2017-01-01",
"localName": "New Year's Day",
"name": "New Year's Day",
"countryCode": "US",
"fixed": true,
"countyOfficialHoliday": true,
"countyAdministrationHoliday": true,
"global": true,
"counties": null,
"launchYear": null
},
{
"date": "2017-01-16",
"localName": "Martin Luther King, Jr. Day",
"name": "Martin Luther King, Jr. Day",
"countryCode": "US",
"fixed": true,
"countyOfficialHoliday": true,
"countyAdministrationHoliday": true,
"global": true,
"counties": null,
"launchYear": null
},
{
"date": "2017-01-20",
"localName": "Inauguration Day",
"name": "Inauguration Day",
"countryCode": "US",
"fixed": true,
"countyOfficialHoliday": true,
"countyAdministrationHoliday": true,
"global": false,
"counties": [
"US-DC",
"US-LA",
"US-MD",
"US-VA"
],
"launchYear": null
},
{
"date": "2017-02-20",
"localName": "Washington's Birthday",
"name": "Presidents' Day",
"countryCode": "US",
"fixed": true,
"countyOfficialHoliday": true,
"countyAdministrationHoliday": true,
"global": true,
"counties": null,
"launchYear": null
},
{
"date": "2017-05-29",
"localName": "Memorial Day",
"name": "Memorial Day",
"countryCode": "US",
"fixed": true,
"countyOfficialHoliday": true,
"countyAdministrationHoliday": true,
"global": true,
"counties": null,
"launchYear": null
},
{
"date": "2017-07-04",
"localName": "Independence Day",
"name": "Independence Day",
"countryCode": "US",
"fixed": true,
"countyOfficialHoliday": true,
"countyAdministrationHoliday": true,
"global": true,
"counties": null,
"launchYear": null
},
{
"date": "2017-09-04",
"localName": "Labor Day",
"name": "Labor Day",
"countryCode": "US",
"fixed": true,
"countyOfficialHoliday": true,
"countyAdministrationHoliday": true,
"global": true,
"counties": null,
"launchYear": null
},
{
"date": "2017-09-09",
"localName": "Columbus Day",
"name": "Columbus Day",
"countryCode": "US",
"fixed": true,
"countyOfficialHoliday": true,
"countyAdministrationHoliday": true,
"global": false,
"counties": [
"US-AL",
"US-AZ",
"US-CO",
"US-CT",
"US-DC",
"US-GA",
"US-ID",
"US-IL",
"US-IN",
"US-IA",
"US-KS",
"US-KY",
"US-LA",
"US-ME",
"US-MD",
"US-MA",
"US-MS",
"US-MO",
"US-MT",
"US-NE",
"US-NH",
"US-NJ",
"US-NM",
"US-NY",
"US-NC",
"US-OH",
"US-OK",
"US-PA",
"US-RI",
"US-SC",
"US-TN",
"US-UT",
"US-VA",
"US-WV"
],
"launchYear": null
},
{
"date": "2017-11-10",
"localName": "Veterans Day",
"name": "Veterans Day",
"countryCode": "US",
"fixed": false,
"countyOfficialHoliday": true,
"countyAdministrationHoliday": true,
"global": true,
"counties": null,
"launchYear": null
},
{
"date": "2017-12-23",
"localName": "Thanksgiving Day",
"name": "Thanksgiving Day",
"countryCode": "US",
"fixed": true,
"countyOfficialHoliday": true,
"countyAdministrationHoliday": true,
"global": true,
"counties": null,
"launchYear": 1863
},
{
"date": "2017-12-25",
"localName": "Christmas Day",
"name": "Christmas Day",
"countryCode": "US",
"fixed": true,
"countyOfficialHoliday": true,
"countyAdministrationHoliday": true,
"global": true,
"counties": null,
"launchYear": null
}
]
Nager.Date
You can use the JSON API of Nager.Date project. It supports USA, Canada and Europe. The data available for every year you can save the information in your own database.
Example
//https://github.com/FasterXML/jackson-databind/
ObjectMapper mapper = new ObjectMapper();
MyValue value = mapper.readValue(new URL("http://date.nager.at/api/v1/get/US/2017"), PublicHoliday.class);
PublicHoliday.class
public class PublicHoliday
{
public String date;
public String localName;
public String name;
public String countryCode;
public Boolean fixed;
public Boolean countyOfficialHoliday;
public Boolean countyAdministrationHoliday;
public Boolean global;
public String counties;
public int launchYear;
}
Example JSON data retrieved.
[
{
"date": "2017-01-01",
"localName": "New Year's Day",
"name": "New Year's Day",
"countryCode": "US",
"fixed": true,
"countyOfficialHoliday": true,
"countyAdministrationHoliday": true,
"global": true,
"counties": null,
"launchYear": null
},
{
"date": "2017-01-16",
"localName": "Martin Luther King, Jr. Day",
"name": "Martin Luther King, Jr. Day",
"countryCode": "US",
"fixed": true,
"countyOfficialHoliday": true,
"countyAdministrationHoliday": true,
"global": true,
"counties": null,
"launchYear": null
},
{
"date": "2017-01-20",
"localName": "Inauguration Day",
"name": "Inauguration Day",
"countryCode": "US",
"fixed": true,
"countyOfficialHoliday": true,
"countyAdministrationHoliday": true,
"global": false,
"counties": [
"US-DC",
"US-LA",
"US-MD",
"US-VA"
],
"launchYear": null
},
{
"date": "2017-02-20",
"localName": "Washington's Birthday",
"name": "Presidents' Day",
"countryCode": "US",
"fixed": true,
"countyOfficialHoliday": true,
"countyAdministrationHoliday": true,
"global": true,
"counties": null,
"launchYear": null
},
{
"date": "2017-05-29",
"localName": "Memorial Day",
"name": "Memorial Day",
"countryCode": "US",
"fixed": true,
"countyOfficialHoliday": true,
"countyAdministrationHoliday": true,
"global": true,
"counties": null,
"launchYear": null
},
{
"date": "2017-07-04",
"localName": "Independence Day",
"name": "Independence Day",
"countryCode": "US",
"fixed": true,
"countyOfficialHoliday": true,
"countyAdministrationHoliday": true,
"global": true,
"counties": null,
"launchYear": null
},
{
"date": "2017-09-04",
"localName": "Labor Day",
"name": "Labor Day",
"countryCode": "US",
"fixed": true,
"countyOfficialHoliday": true,
"countyAdministrationHoliday": true,
"global": true,
"counties": null,
"launchYear": null
},
{
"date": "2017-09-09",
"localName": "Columbus Day",
"name": "Columbus Day",
"countryCode": "US",
"fixed": true,
"countyOfficialHoliday": true,
"countyAdministrationHoliday": true,
"global": false,
"counties": [
"US-AL",
"US-AZ",
"US-CO",
"US-CT",
"US-DC",
"US-GA",
"US-ID",
"US-IL",
"US-IN",
"US-IA",
"US-KS",
"US-KY",
"US-LA",
"US-ME",
"US-MD",
"US-MA",
"US-MS",
"US-MO",
"US-MT",
"US-NE",
"US-NH",
"US-NJ",
"US-NM",
"US-NY",
"US-NC",
"US-OH",
"US-OK",
"US-PA",
"US-RI",
"US-SC",
"US-TN",
"US-UT",
"US-VA",
"US-WV"
],
"launchYear": null
},
{
"date": "2017-11-10",
"localName": "Veterans Day",
"name": "Veterans Day",
"countryCode": "US",
"fixed": false,
"countyOfficialHoliday": true,
"countyAdministrationHoliday": true,
"global": true,
"counties": null,
"launchYear": null
},
{
"date": "2017-12-23",
"localName": "Thanksgiving Day",
"name": "Thanksgiving Day",
"countryCode": "US",
"fixed": true,
"countyOfficialHoliday": true,
"countyAdministrationHoliday": true,
"global": true,
"counties": null,
"launchYear": 1863
},
{
"date": "2017-12-25",
"localName": "Christmas Day",
"name": "Christmas Day",
"countryCode": "US",
"fixed": true,
"countyOfficialHoliday": true,
"countyAdministrationHoliday": true,
"global": true,
"counties": null,
"launchYear": null
}
]
edited Feb 21 at 12:53
answered Feb 9 '17 at 21:27


live2live2
1,53611524
1,53611524
1
You should follow the Java Naming Conventions: variable names always start with lowercase.
– MC Emperor
Jan 16 at 6:51
add a comment |
1
You should follow the Java Naming Conventions: variable names always start with lowercase.
– MC Emperor
Jan 16 at 6:51
1
1
You should follow the Java Naming Conventions: variable names always start with lowercase.
– MC Emperor
Jan 16 at 6:51
You should follow the Java Naming Conventions: variable names always start with lowercase.
– MC Emperor
Jan 16 at 6:51
add a comment |
Since the accepted answer still uses the obsolete Calendar
class – here's my two cents using the new Java Date and Time API.
You have to get the dates of the holidays from somewhere, there is no standard Java library for it. That would be too localized anyway, since holidays heavily depends on your country and region. (except for widely known holidays, such as Christmas or Easter).
You could get them from a holiday API, for instance. In the code below I've hardcoded them as a List
of LocalDate
s.
Java 9
LocalDate startDate = LocalDate.of(2012, 3, 7);
LocalDate endDate = LocalDate.of(2012, 6, 7);
// I've hardcoded the holidays as LocalDates and put them in a List
final List<LocalDate> holidays = Arrays.asList(
LocalDate.of(2018, 7, 4)
);
List<LocalDate> allDates =
// Java 9 provides a method to return a stream with dates from the
// startdate to the given end date. Note that the end date itself is
// NOT included.
startDate.datesUntil(endDate)
// Retain all business days. Use static imports from
// java.time.DayOfWeek.*
.filter(t -> Stream.of(MONDAY, TUESDAY, WEDNESDAY, THURSDAY, FRIDAY)
.anyMatch(t.getDayOfWeek()::equals))
// Retain only dates not present in our holidays list
.filter(t -> !holidays.contains(t))
// Collect them into a List. If you only need to know the number of
// dates, you can also use .count()
.collect(Collectors.toList());
Java 8
The method LocalDate.datesUntil
is not available in Java 8, so you have to obtain a stream of all dates between those two dates in a different manner. We must first count the total number of days in between using the ChronoUnit.DAYS.between
method.
long numOfDaysBetween = ChronoUnit.DAYS.between(startDate, endDate);
Then we must generate a sequence of integers exactly as long as the number of days between the start and end date, and then create LocalDate
s from it, starting at the start date.
IntStream.iterate(0, i -> i + 1)
.limit(numOfDaysBetween)
.mapToObj(startDate::plusDays)
Now we have a Stream<LocalDate>
, and you can then use the remaining part of the Java 9 code.
add a comment |
Since the accepted answer still uses the obsolete Calendar
class – here's my two cents using the new Java Date and Time API.
You have to get the dates of the holidays from somewhere, there is no standard Java library for it. That would be too localized anyway, since holidays heavily depends on your country and region. (except for widely known holidays, such as Christmas or Easter).
You could get them from a holiday API, for instance. In the code below I've hardcoded them as a List
of LocalDate
s.
Java 9
LocalDate startDate = LocalDate.of(2012, 3, 7);
LocalDate endDate = LocalDate.of(2012, 6, 7);
// I've hardcoded the holidays as LocalDates and put them in a List
final List<LocalDate> holidays = Arrays.asList(
LocalDate.of(2018, 7, 4)
);
List<LocalDate> allDates =
// Java 9 provides a method to return a stream with dates from the
// startdate to the given end date. Note that the end date itself is
// NOT included.
startDate.datesUntil(endDate)
// Retain all business days. Use static imports from
// java.time.DayOfWeek.*
.filter(t -> Stream.of(MONDAY, TUESDAY, WEDNESDAY, THURSDAY, FRIDAY)
.anyMatch(t.getDayOfWeek()::equals))
// Retain only dates not present in our holidays list
.filter(t -> !holidays.contains(t))
// Collect them into a List. If you only need to know the number of
// dates, you can also use .count()
.collect(Collectors.toList());
Java 8
The method LocalDate.datesUntil
is not available in Java 8, so you have to obtain a stream of all dates between those two dates in a different manner. We must first count the total number of days in between using the ChronoUnit.DAYS.between
method.
long numOfDaysBetween = ChronoUnit.DAYS.between(startDate, endDate);
Then we must generate a sequence of integers exactly as long as the number of days between the start and end date, and then create LocalDate
s from it, starting at the start date.
IntStream.iterate(0, i -> i + 1)
.limit(numOfDaysBetween)
.mapToObj(startDate::plusDays)
Now we have a Stream<LocalDate>
, and you can then use the remaining part of the Java 9 code.
add a comment |
Since the accepted answer still uses the obsolete Calendar
class – here's my two cents using the new Java Date and Time API.
You have to get the dates of the holidays from somewhere, there is no standard Java library for it. That would be too localized anyway, since holidays heavily depends on your country and region. (except for widely known holidays, such as Christmas or Easter).
You could get them from a holiday API, for instance. In the code below I've hardcoded them as a List
of LocalDate
s.
Java 9
LocalDate startDate = LocalDate.of(2012, 3, 7);
LocalDate endDate = LocalDate.of(2012, 6, 7);
// I've hardcoded the holidays as LocalDates and put them in a List
final List<LocalDate> holidays = Arrays.asList(
LocalDate.of(2018, 7, 4)
);
List<LocalDate> allDates =
// Java 9 provides a method to return a stream with dates from the
// startdate to the given end date. Note that the end date itself is
// NOT included.
startDate.datesUntil(endDate)
// Retain all business days. Use static imports from
// java.time.DayOfWeek.*
.filter(t -> Stream.of(MONDAY, TUESDAY, WEDNESDAY, THURSDAY, FRIDAY)
.anyMatch(t.getDayOfWeek()::equals))
// Retain only dates not present in our holidays list
.filter(t -> !holidays.contains(t))
// Collect them into a List. If you only need to know the number of
// dates, you can also use .count()
.collect(Collectors.toList());
Java 8
The method LocalDate.datesUntil
is not available in Java 8, so you have to obtain a stream of all dates between those two dates in a different manner. We must first count the total number of days in between using the ChronoUnit.DAYS.between
method.
long numOfDaysBetween = ChronoUnit.DAYS.between(startDate, endDate);
Then we must generate a sequence of integers exactly as long as the number of days between the start and end date, and then create LocalDate
s from it, starting at the start date.
IntStream.iterate(0, i -> i + 1)
.limit(numOfDaysBetween)
.mapToObj(startDate::plusDays)
Now we have a Stream<LocalDate>
, and you can then use the remaining part of the Java 9 code.
Since the accepted answer still uses the obsolete Calendar
class – here's my two cents using the new Java Date and Time API.
You have to get the dates of the holidays from somewhere, there is no standard Java library for it. That would be too localized anyway, since holidays heavily depends on your country and region. (except for widely known holidays, such as Christmas or Easter).
You could get them from a holiday API, for instance. In the code below I've hardcoded them as a List
of LocalDate
s.
Java 9
LocalDate startDate = LocalDate.of(2012, 3, 7);
LocalDate endDate = LocalDate.of(2012, 6, 7);
// I've hardcoded the holidays as LocalDates and put them in a List
final List<LocalDate> holidays = Arrays.asList(
LocalDate.of(2018, 7, 4)
);
List<LocalDate> allDates =
// Java 9 provides a method to return a stream with dates from the
// startdate to the given end date. Note that the end date itself is
// NOT included.
startDate.datesUntil(endDate)
// Retain all business days. Use static imports from
// java.time.DayOfWeek.*
.filter(t -> Stream.of(MONDAY, TUESDAY, WEDNESDAY, THURSDAY, FRIDAY)
.anyMatch(t.getDayOfWeek()::equals))
// Retain only dates not present in our holidays list
.filter(t -> !holidays.contains(t))
// Collect them into a List. If you only need to know the number of
// dates, you can also use .count()
.collect(Collectors.toList());
Java 8
The method LocalDate.datesUntil
is not available in Java 8, so you have to obtain a stream of all dates between those two dates in a different manner. We must first count the total number of days in between using the ChronoUnit.DAYS.between
method.
long numOfDaysBetween = ChronoUnit.DAYS.between(startDate, endDate);
Then we must generate a sequence of integers exactly as long as the number of days between the start and end date, and then create LocalDate
s from it, starting at the start date.
IntStream.iterate(0, i -> i + 1)
.limit(numOfDaysBetween)
.mapToObj(startDate::plusDays)
Now we have a Stream<LocalDate>
, and you can then use the remaining part of the Java 9 code.
edited Nov 26 '18 at 10:10
answered Nov 26 '18 at 9:47


MC EmperorMC Emperor
9,050125690
9,050125690
add a comment |
add a comment |
I don't have any code samples or anything like that, but I did some searching for you and came across this Stack Overflow thread that has some links to web services that can return holiday dates for you, which may help you get to where you need to be: National holiday web service
The top answer in that thread links to this web service: http://www.holidaywebservice.com/
I'm not sure if using a web service for this type of thing is overkill or not, but surely there is a better way. I apologize, I am not the most experienced programmer so I can't help you as much as I'd like to.
1
It's not really overkill. You need to be getting the information from somewhere else as it's always subject to change.
– djechlin
Jun 1 '12 at 16:53
add a comment |
I don't have any code samples or anything like that, but I did some searching for you and came across this Stack Overflow thread that has some links to web services that can return holiday dates for you, which may help you get to where you need to be: National holiday web service
The top answer in that thread links to this web service: http://www.holidaywebservice.com/
I'm not sure if using a web service for this type of thing is overkill or not, but surely there is a better way. I apologize, I am not the most experienced programmer so I can't help you as much as I'd like to.
1
It's not really overkill. You need to be getting the information from somewhere else as it's always subject to change.
– djechlin
Jun 1 '12 at 16:53
add a comment |
I don't have any code samples or anything like that, but I did some searching for you and came across this Stack Overflow thread that has some links to web services that can return holiday dates for you, which may help you get to where you need to be: National holiday web service
The top answer in that thread links to this web service: http://www.holidaywebservice.com/
I'm not sure if using a web service for this type of thing is overkill or not, but surely there is a better way. I apologize, I am not the most experienced programmer so I can't help you as much as I'd like to.
I don't have any code samples or anything like that, but I did some searching for you and came across this Stack Overflow thread that has some links to web services that can return holiday dates for you, which may help you get to where you need to be: National holiday web service
The top answer in that thread links to this web service: http://www.holidaywebservice.com/
I'm not sure if using a web service for this type of thing is overkill or not, but surely there is a better way. I apologize, I am not the most experienced programmer so I can't help you as much as I'd like to.
edited May 23 '17 at 12:25
Community♦
11
11
answered Jun 1 '12 at 16:37
soberingsobering
339110
339110
1
It's not really overkill. You need to be getting the information from somewhere else as it's always subject to change.
– djechlin
Jun 1 '12 at 16:53
add a comment |
1
It's not really overkill. You need to be getting the information from somewhere else as it's always subject to change.
– djechlin
Jun 1 '12 at 16:53
1
1
It's not really overkill. You need to be getting the information from somewhere else as it's always subject to change.
– djechlin
Jun 1 '12 at 16:53
It's not really overkill. You need to be getting the information from somewhere else as it's always subject to change.
– djechlin
Jun 1 '12 at 16:53
add a comment |
The fastest way I've found is using some math (borrowing from the SO post):
public static int totalBusinessDaysBetween(LocalDate start, LocalDate end) {
Objects.requireNonNull(start, "Start date must not be null");
Objects.requireNonNull(end, "End date must not be null");
long daysBetweenWithoutWeekends = calculateNumberOfDaysBetweenMinusWeekends(start, end);
final Set<LocalDate> holidayForYearRange = getUSFederalHolidayForYearRange(start.getYear(), end.getYear());
for (LocalDate localDate : holidayForYearRange) {
if (localDate.isAfter(start) && localDate.isBefore(end)) {
daysBetweenWithoutWeekends--;
}
}
return (int) daysBetweenWithoutWeekends;
}
And:
private static long calculateNumberOfDaysBetweenMinusWeekends(LocalDate start, LocalDate end) {
final DayOfWeek startW = start.getDayOfWeek();
final DayOfWeek endW = end.getDayOfWeek();
final long days = ChronoUnit.DAYS.between(start, end);
final long daysWithoutWeekends = days - 2 * ((days + startW.getValue()) / 7);
//adjust for starting and ending on a Sunday:
return daysWithoutWeekends + (startW == DayOfWeek.SUNDAY ? 1 : 0) + (endW == DayOfWeek.SUNDAY ? 1 : 0);
}
I put something more complete together here.
1
While this link may answer the question, it is better to include the essential parts of the answer here and provide the link for reference. Link-only answers can become invalid if the linked page changes. - From Review
– Nico Haase
Nov 29 '18 at 15:11
Thanks for the feedback.
– James Dunnam
Nov 29 '18 at 15:42
add a comment |
The fastest way I've found is using some math (borrowing from the SO post):
public static int totalBusinessDaysBetween(LocalDate start, LocalDate end) {
Objects.requireNonNull(start, "Start date must not be null");
Objects.requireNonNull(end, "End date must not be null");
long daysBetweenWithoutWeekends = calculateNumberOfDaysBetweenMinusWeekends(start, end);
final Set<LocalDate> holidayForYearRange = getUSFederalHolidayForYearRange(start.getYear(), end.getYear());
for (LocalDate localDate : holidayForYearRange) {
if (localDate.isAfter(start) && localDate.isBefore(end)) {
daysBetweenWithoutWeekends--;
}
}
return (int) daysBetweenWithoutWeekends;
}
And:
private static long calculateNumberOfDaysBetweenMinusWeekends(LocalDate start, LocalDate end) {
final DayOfWeek startW = start.getDayOfWeek();
final DayOfWeek endW = end.getDayOfWeek();
final long days = ChronoUnit.DAYS.between(start, end);
final long daysWithoutWeekends = days - 2 * ((days + startW.getValue()) / 7);
//adjust for starting and ending on a Sunday:
return daysWithoutWeekends + (startW == DayOfWeek.SUNDAY ? 1 : 0) + (endW == DayOfWeek.SUNDAY ? 1 : 0);
}
I put something more complete together here.
1
While this link may answer the question, it is better to include the essential parts of the answer here and provide the link for reference. Link-only answers can become invalid if the linked page changes. - From Review
– Nico Haase
Nov 29 '18 at 15:11
Thanks for the feedback.
– James Dunnam
Nov 29 '18 at 15:42
add a comment |
The fastest way I've found is using some math (borrowing from the SO post):
public static int totalBusinessDaysBetween(LocalDate start, LocalDate end) {
Objects.requireNonNull(start, "Start date must not be null");
Objects.requireNonNull(end, "End date must not be null");
long daysBetweenWithoutWeekends = calculateNumberOfDaysBetweenMinusWeekends(start, end);
final Set<LocalDate> holidayForYearRange = getUSFederalHolidayForYearRange(start.getYear(), end.getYear());
for (LocalDate localDate : holidayForYearRange) {
if (localDate.isAfter(start) && localDate.isBefore(end)) {
daysBetweenWithoutWeekends--;
}
}
return (int) daysBetweenWithoutWeekends;
}
And:
private static long calculateNumberOfDaysBetweenMinusWeekends(LocalDate start, LocalDate end) {
final DayOfWeek startW = start.getDayOfWeek();
final DayOfWeek endW = end.getDayOfWeek();
final long days = ChronoUnit.DAYS.between(start, end);
final long daysWithoutWeekends = days - 2 * ((days + startW.getValue()) / 7);
//adjust for starting and ending on a Sunday:
return daysWithoutWeekends + (startW == DayOfWeek.SUNDAY ? 1 : 0) + (endW == DayOfWeek.SUNDAY ? 1 : 0);
}
I put something more complete together here.
The fastest way I've found is using some math (borrowing from the SO post):
public static int totalBusinessDaysBetween(LocalDate start, LocalDate end) {
Objects.requireNonNull(start, "Start date must not be null");
Objects.requireNonNull(end, "End date must not be null");
long daysBetweenWithoutWeekends = calculateNumberOfDaysBetweenMinusWeekends(start, end);
final Set<LocalDate> holidayForYearRange = getUSFederalHolidayForYearRange(start.getYear(), end.getYear());
for (LocalDate localDate : holidayForYearRange) {
if (localDate.isAfter(start) && localDate.isBefore(end)) {
daysBetweenWithoutWeekends--;
}
}
return (int) daysBetweenWithoutWeekends;
}
And:
private static long calculateNumberOfDaysBetweenMinusWeekends(LocalDate start, LocalDate end) {
final DayOfWeek startW = start.getDayOfWeek();
final DayOfWeek endW = end.getDayOfWeek();
final long days = ChronoUnit.DAYS.between(start, end);
final long daysWithoutWeekends = days - 2 * ((days + startW.getValue()) / 7);
//adjust for starting and ending on a Sunday:
return daysWithoutWeekends + (startW == DayOfWeek.SUNDAY ? 1 : 0) + (endW == DayOfWeek.SUNDAY ? 1 : 0);
}
I put something more complete together here.
edited Nov 29 '18 at 15:39
answered Nov 29 '18 at 14:12


James DunnamJames Dunnam
194
194
1
While this link may answer the question, it is better to include the essential parts of the answer here and provide the link for reference. Link-only answers can become invalid if the linked page changes. - From Review
– Nico Haase
Nov 29 '18 at 15:11
Thanks for the feedback.
– James Dunnam
Nov 29 '18 at 15:42
add a comment |
1
While this link may answer the question, it is better to include the essential parts of the answer here and provide the link for reference. Link-only answers can become invalid if the linked page changes. - From Review
– Nico Haase
Nov 29 '18 at 15:11
Thanks for the feedback.
– James Dunnam
Nov 29 '18 at 15:42
1
1
While this link may answer the question, it is better to include the essential parts of the answer here and provide the link for reference. Link-only answers can become invalid if the linked page changes. - From Review
– Nico Haase
Nov 29 '18 at 15:11
While this link may answer the question, it is better to include the essential parts of the answer here and provide the link for reference. Link-only answers can become invalid if the linked page changes. - From Review
– Nico Haase
Nov 29 '18 at 15:11
Thanks for the feedback.
– James Dunnam
Nov 29 '18 at 15:42
Thanks for the feedback.
– James Dunnam
Nov 29 '18 at 15:42
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f10854119%2fcalculate-business-days-including-holidays%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
f1Ei7nauldIBG4H 2GOl6O8r5h eppko2Ptxwid,B8TE8y,E8ctq4Ztef23h,JTE1W
You need to keep track of the knowledge of which days are holidays manually, AFAIK there's no built in function for that. Also, who uses do-while in java these days??
– TS-
Jun 1 '12 at 16:33
First of all you will need a list of all the holidays in a year beacuse no java class (i.e.
Locale
specific class) provides this functionality. Then you will need to find how many from this list fall between the dates specified which is fairly straight-forward. Then you can delete that many days from the result of your above code.– Surender Thakran
Jun 1 '12 at 16:37
thanks for ur reply. let's say if i have a list which contains all the holidays, can u please suggest me how to use that list or how to check that list dates in the condition.
– ran
Jun 1 '12 at 16:38
Duplicate of the Question Date api for managing off days in an year? but this one has better answers.
– Basil Bourque
Feb 17 '17 at 3:34