Spring boot relationship is not initialized
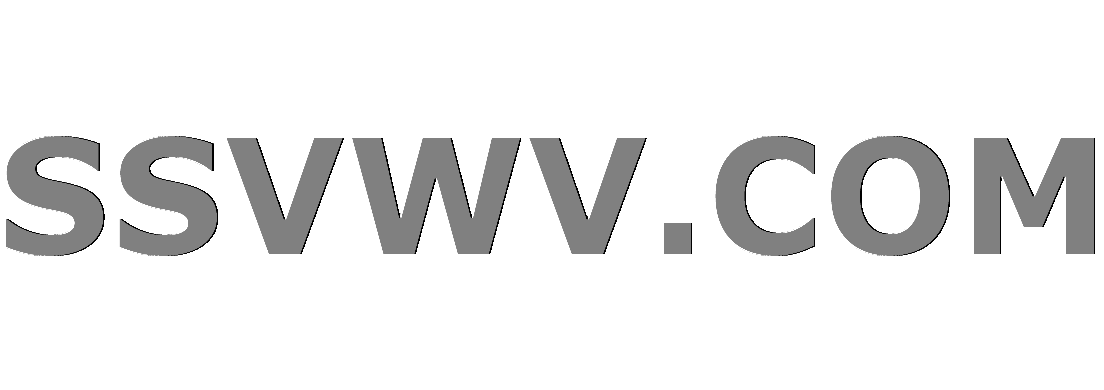
Multi tool use
I have a entity called origin and wallet. in this scenario origin has a wallet. But when i run the code the origin values are coming but the wallet values are not coming.
package com.mobios.model;
import java.util.Date;
@Entity
@Table(name = "origin")
public class Origin {
@Id
@Column(name = "acc_no", nullable = false)
private String acc_no;
@Column(name = "mobile_number", nullable = true)
private String mobile_number;
@ManyToOne
private Wallet wallet;
public Wallet getWallet() {
return wallet;
}
public void setWallet(Wallet wallet) {
this.wallet = wallet;
}
}
Following is my DAO
@Override
@SuppressWarnings({ "rawtypes", "unchecked" })
public Origin authenticateOrigin(String _userName, int _pin) {
Origin _origin;
String _query = "SELECT * FROM origin WHERE mobile_number= ? and pin= ?";
_origin = (Origin) _jdbcTemplate.queryForObject(_query, new Object
{ _userName, _pin },
new BeanPropertyRowMapper(Origin.class));
return _origin;
}
I cant find where i have done wrong.
I have edited my interface as follows
public interface LoginDao extends JpaRepository<Origin, String>{
/**
* Return the authenticated origin. If not will return null
*
* @return _origin
*/
Origin authenticateOrigin(String _userName, int _pin);
}
But i cannot understand how to call the query in this case. JPA generate some methods to get data from database. i dont need it. I need to get data by using customized queries
spring spring-boot
|
show 5 more comments
I have a entity called origin and wallet. in this scenario origin has a wallet. But when i run the code the origin values are coming but the wallet values are not coming.
package com.mobios.model;
import java.util.Date;
@Entity
@Table(name = "origin")
public class Origin {
@Id
@Column(name = "acc_no", nullable = false)
private String acc_no;
@Column(name = "mobile_number", nullable = true)
private String mobile_number;
@ManyToOne
private Wallet wallet;
public Wallet getWallet() {
return wallet;
}
public void setWallet(Wallet wallet) {
this.wallet = wallet;
}
}
Following is my DAO
@Override
@SuppressWarnings({ "rawtypes", "unchecked" })
public Origin authenticateOrigin(String _userName, int _pin) {
Origin _origin;
String _query = "SELECT * FROM origin WHERE mobile_number= ? and pin= ?";
_origin = (Origin) _jdbcTemplate.queryForObject(_query, new Object
{ _userName, _pin },
new BeanPropertyRowMapper(Origin.class));
return _origin;
}
I cant find where i have done wrong.
I have edited my interface as follows
public interface LoginDao extends JpaRepository<Origin, String>{
/**
* Return the authenticated origin. If not will return null
*
* @return _origin
*/
Origin authenticateOrigin(String _userName, int _pin);
}
But i cannot understand how to call the query in this case. JPA generate some methods to get data from database. i dont need it. I need to get data by using customized queries
spring spring-boot
Well, you're using SQL, completely bypassing the JPA EntityManager, to load only the origin columns. Why would the wallet be populated? Use a JPQL query, using the JPA API. And respect the Java naming conventions.
– JB Nizet
Nov 26 '18 at 6:43
Can you explain how to overcome this problem @JBNizet
– K Rajitha
Nov 26 '18 at 6:48
I have already. Use the JPA API (EntityManager) to execute a JPQL query. docs.oracle.com/javaee/7/api/javax/persistence/…. In a Spring world, you would typically use a Spring-data-jpa repository, with a method with a well-defined name (where the query would automatically be inferred fromt the method name), or annotated with@Query
to specify the JPQL query. If you have no idea of what I am talking about, then it means you have to start learning how JPA works before using it.
– JB Nizet
Nov 26 '18 at 6:51
I have referred but i cannot use those methods to fix my problem. Can you give solution to fix this please @JBNizet
– K Rajitha
Nov 26 '18 at 7:59
Edit your question, and show what you have tried. 1 hour is very short to learn JPA.
– JB Nizet
Nov 26 '18 at 8:00
|
show 5 more comments
I have a entity called origin and wallet. in this scenario origin has a wallet. But when i run the code the origin values are coming but the wallet values are not coming.
package com.mobios.model;
import java.util.Date;
@Entity
@Table(name = "origin")
public class Origin {
@Id
@Column(name = "acc_no", nullable = false)
private String acc_no;
@Column(name = "mobile_number", nullable = true)
private String mobile_number;
@ManyToOne
private Wallet wallet;
public Wallet getWallet() {
return wallet;
}
public void setWallet(Wallet wallet) {
this.wallet = wallet;
}
}
Following is my DAO
@Override
@SuppressWarnings({ "rawtypes", "unchecked" })
public Origin authenticateOrigin(String _userName, int _pin) {
Origin _origin;
String _query = "SELECT * FROM origin WHERE mobile_number= ? and pin= ?";
_origin = (Origin) _jdbcTemplate.queryForObject(_query, new Object
{ _userName, _pin },
new BeanPropertyRowMapper(Origin.class));
return _origin;
}
I cant find where i have done wrong.
I have edited my interface as follows
public interface LoginDao extends JpaRepository<Origin, String>{
/**
* Return the authenticated origin. If not will return null
*
* @return _origin
*/
Origin authenticateOrigin(String _userName, int _pin);
}
But i cannot understand how to call the query in this case. JPA generate some methods to get data from database. i dont need it. I need to get data by using customized queries
spring spring-boot
I have a entity called origin and wallet. in this scenario origin has a wallet. But when i run the code the origin values are coming but the wallet values are not coming.
package com.mobios.model;
import java.util.Date;
@Entity
@Table(name = "origin")
public class Origin {
@Id
@Column(name = "acc_no", nullable = false)
private String acc_no;
@Column(name = "mobile_number", nullable = true)
private String mobile_number;
@ManyToOne
private Wallet wallet;
public Wallet getWallet() {
return wallet;
}
public void setWallet(Wallet wallet) {
this.wallet = wallet;
}
}
Following is my DAO
@Override
@SuppressWarnings({ "rawtypes", "unchecked" })
public Origin authenticateOrigin(String _userName, int _pin) {
Origin _origin;
String _query = "SELECT * FROM origin WHERE mobile_number= ? and pin= ?";
_origin = (Origin) _jdbcTemplate.queryForObject(_query, new Object
{ _userName, _pin },
new BeanPropertyRowMapper(Origin.class));
return _origin;
}
I cant find where i have done wrong.
I have edited my interface as follows
public interface LoginDao extends JpaRepository<Origin, String>{
/**
* Return the authenticated origin. If not will return null
*
* @return _origin
*/
Origin authenticateOrigin(String _userName, int _pin);
}
But i cannot understand how to call the query in this case. JPA generate some methods to get data from database. i dont need it. I need to get data by using customized queries
spring spring-boot
spring spring-boot
edited Nov 26 '18 at 8:03
K Rajitha
asked Nov 26 '18 at 6:36
K RajithaK Rajitha
159213
159213
Well, you're using SQL, completely bypassing the JPA EntityManager, to load only the origin columns. Why would the wallet be populated? Use a JPQL query, using the JPA API. And respect the Java naming conventions.
– JB Nizet
Nov 26 '18 at 6:43
Can you explain how to overcome this problem @JBNizet
– K Rajitha
Nov 26 '18 at 6:48
I have already. Use the JPA API (EntityManager) to execute a JPQL query. docs.oracle.com/javaee/7/api/javax/persistence/…. In a Spring world, you would typically use a Spring-data-jpa repository, with a method with a well-defined name (where the query would automatically be inferred fromt the method name), or annotated with@Query
to specify the JPQL query. If you have no idea of what I am talking about, then it means you have to start learning how JPA works before using it.
– JB Nizet
Nov 26 '18 at 6:51
I have referred but i cannot use those methods to fix my problem. Can you give solution to fix this please @JBNizet
– K Rajitha
Nov 26 '18 at 7:59
Edit your question, and show what you have tried. 1 hour is very short to learn JPA.
– JB Nizet
Nov 26 '18 at 8:00
|
show 5 more comments
Well, you're using SQL, completely bypassing the JPA EntityManager, to load only the origin columns. Why would the wallet be populated? Use a JPQL query, using the JPA API. And respect the Java naming conventions.
– JB Nizet
Nov 26 '18 at 6:43
Can you explain how to overcome this problem @JBNizet
– K Rajitha
Nov 26 '18 at 6:48
I have already. Use the JPA API (EntityManager) to execute a JPQL query. docs.oracle.com/javaee/7/api/javax/persistence/…. In a Spring world, you would typically use a Spring-data-jpa repository, with a method with a well-defined name (where the query would automatically be inferred fromt the method name), or annotated with@Query
to specify the JPQL query. If you have no idea of what I am talking about, then it means you have to start learning how JPA works before using it.
– JB Nizet
Nov 26 '18 at 6:51
I have referred but i cannot use those methods to fix my problem. Can you give solution to fix this please @JBNizet
– K Rajitha
Nov 26 '18 at 7:59
Edit your question, and show what you have tried. 1 hour is very short to learn JPA.
– JB Nizet
Nov 26 '18 at 8:00
Well, you're using SQL, completely bypassing the JPA EntityManager, to load only the origin columns. Why would the wallet be populated? Use a JPQL query, using the JPA API. And respect the Java naming conventions.
– JB Nizet
Nov 26 '18 at 6:43
Well, you're using SQL, completely bypassing the JPA EntityManager, to load only the origin columns. Why would the wallet be populated? Use a JPQL query, using the JPA API. And respect the Java naming conventions.
– JB Nizet
Nov 26 '18 at 6:43
Can you explain how to overcome this problem @JBNizet
– K Rajitha
Nov 26 '18 at 6:48
Can you explain how to overcome this problem @JBNizet
– K Rajitha
Nov 26 '18 at 6:48
I have already. Use the JPA API (EntityManager) to execute a JPQL query. docs.oracle.com/javaee/7/api/javax/persistence/…. In a Spring world, you would typically use a Spring-data-jpa repository, with a method with a well-defined name (where the query would automatically be inferred fromt the method name), or annotated with
@Query
to specify the JPQL query. If you have no idea of what I am talking about, then it means you have to start learning how JPA works before using it.– JB Nizet
Nov 26 '18 at 6:51
I have already. Use the JPA API (EntityManager) to execute a JPQL query. docs.oracle.com/javaee/7/api/javax/persistence/…. In a Spring world, you would typically use a Spring-data-jpa repository, with a method with a well-defined name (where the query would automatically be inferred fromt the method name), or annotated with
@Query
to specify the JPQL query. If you have no idea of what I am talking about, then it means you have to start learning how JPA works before using it.– JB Nizet
Nov 26 '18 at 6:51
I have referred but i cannot use those methods to fix my problem. Can you give solution to fix this please @JBNizet
– K Rajitha
Nov 26 '18 at 7:59
I have referred but i cannot use those methods to fix my problem. Can you give solution to fix this please @JBNizet
– K Rajitha
Nov 26 '18 at 7:59
Edit your question, and show what you have tried. 1 hour is very short to learn JPA.
– JB Nizet
Nov 26 '18 at 8:00
Edit your question, and show what you have tried. 1 hour is very short to learn JPA.
– JB Nizet
Nov 26 '18 at 8:00
|
show 5 more comments
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53475847%2fspring-boot-relationship-is-not-initialized%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53475847%2fspring-boot-relationship-is-not-initialized%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
1ivoGE,KPcgyDinJBo vyw89,H9uZHAbK7
Well, you're using SQL, completely bypassing the JPA EntityManager, to load only the origin columns. Why would the wallet be populated? Use a JPQL query, using the JPA API. And respect the Java naming conventions.
– JB Nizet
Nov 26 '18 at 6:43
Can you explain how to overcome this problem @JBNizet
– K Rajitha
Nov 26 '18 at 6:48
I have already. Use the JPA API (EntityManager) to execute a JPQL query. docs.oracle.com/javaee/7/api/javax/persistence/…. In a Spring world, you would typically use a Spring-data-jpa repository, with a method with a well-defined name (where the query would automatically be inferred fromt the method name), or annotated with
@Query
to specify the JPQL query. If you have no idea of what I am talking about, then it means you have to start learning how JPA works before using it.– JB Nizet
Nov 26 '18 at 6:51
I have referred but i cannot use those methods to fix my problem. Can you give solution to fix this please @JBNizet
– K Rajitha
Nov 26 '18 at 7:59
Edit your question, and show what you have tried. 1 hour is very short to learn JPA.
– JB Nizet
Nov 26 '18 at 8:00