How to resolve complex JSON and put it in list into list using Retrofit
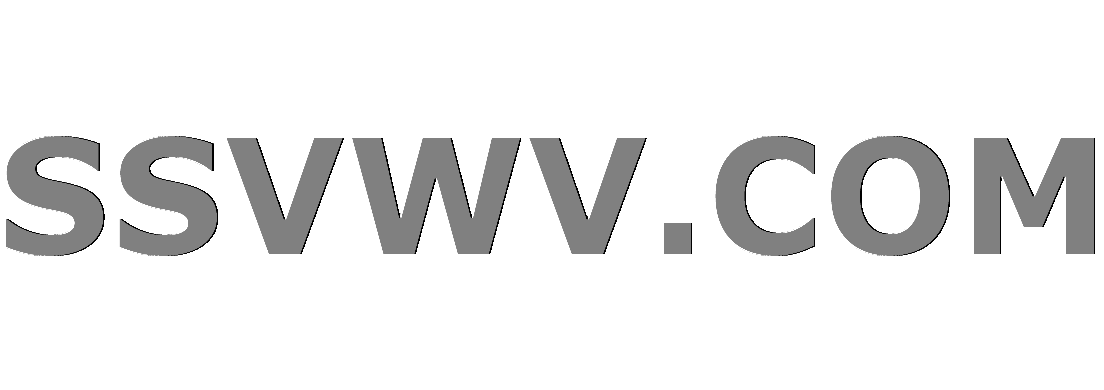
Multi tool use
up vote
0
down vote
favorite
I have JSON as shown below
{
"status" : "succesfull",
"items": [
{
"id" : "1",
"name": "apple",
"counrty": "USA"
},
{
"id": "2",
"name": "banana",
"country": "Jamaica"
},
{
"id":"3",
"name": "potatoes",
"counrty": "Belarus"
},
...
]
}
Let's say, country is varied, and I don't know which countries will be in list
I need to make a list as below:
Any solutions will be appreciated
public class Response {
String status;
ArrayList<Items> items;
}
public class Items{
String id;
String name;
String country;
}
java


add a comment |
up vote
0
down vote
favorite
I have JSON as shown below
{
"status" : "succesfull",
"items": [
{
"id" : "1",
"name": "apple",
"counrty": "USA"
},
{
"id": "2",
"name": "banana",
"country": "Jamaica"
},
{
"id":"3",
"name": "potatoes",
"counrty": "Belarus"
},
...
]
}
Let's say, country is varied, and I don't know which countries will be in list
I need to make a list as below:
Any solutions will be appreciated
public class Response {
String status;
ArrayList<Items> items;
}
public class Items{
String id;
String name;
String country;
}
java


You'll have to write some code to do the structure conversion.
– Henry
Nov 18 at 9:00
I don't know how to create list in the list
– Just Ahead
Nov 18 at 9:01
@JustAhead have you tried expandable listview - androidhive.info/2013/07/android-expandable-list-view-tutorial
– Navneet Krishna
Nov 18 at 10:15
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I have JSON as shown below
{
"status" : "succesfull",
"items": [
{
"id" : "1",
"name": "apple",
"counrty": "USA"
},
{
"id": "2",
"name": "banana",
"country": "Jamaica"
},
{
"id":"3",
"name": "potatoes",
"counrty": "Belarus"
},
...
]
}
Let's say, country is varied, and I don't know which countries will be in list
I need to make a list as below:
Any solutions will be appreciated
public class Response {
String status;
ArrayList<Items> items;
}
public class Items{
String id;
String name;
String country;
}
java


I have JSON as shown below
{
"status" : "succesfull",
"items": [
{
"id" : "1",
"name": "apple",
"counrty": "USA"
},
{
"id": "2",
"name": "banana",
"country": "Jamaica"
},
{
"id":"3",
"name": "potatoes",
"counrty": "Belarus"
},
...
]
}
Let's say, country is varied, and I don't know which countries will be in list
I need to make a list as below:
Any solutions will be appreciated
public class Response {
String status;
ArrayList<Items> items;
}
public class Items{
String id;
String name;
String country;
}
java


java


asked Nov 18 at 8:57


Just Ahead
648
648
You'll have to write some code to do the structure conversion.
– Henry
Nov 18 at 9:00
I don't know how to create list in the list
– Just Ahead
Nov 18 at 9:01
@JustAhead have you tried expandable listview - androidhive.info/2013/07/android-expandable-list-view-tutorial
– Navneet Krishna
Nov 18 at 10:15
add a comment |
You'll have to write some code to do the structure conversion.
– Henry
Nov 18 at 9:00
I don't know how to create list in the list
– Just Ahead
Nov 18 at 9:01
@JustAhead have you tried expandable listview - androidhive.info/2013/07/android-expandable-list-view-tutorial
– Navneet Krishna
Nov 18 at 10:15
You'll have to write some code to do the structure conversion.
– Henry
Nov 18 at 9:00
You'll have to write some code to do the structure conversion.
– Henry
Nov 18 at 9:00
I don't know how to create list in the list
– Just Ahead
Nov 18 at 9:01
I don't know how to create list in the list
– Just Ahead
Nov 18 at 9:01
@JustAhead have you tried expandable listview - androidhive.info/2013/07/android-expandable-list-view-tutorial
– Navneet Krishna
Nov 18 at 10:15
@JustAhead have you tried expandable listview - androidhive.info/2013/07/android-expandable-list-view-tutorial
– Navneet Krishna
Nov 18 at 10:15
add a comment |
2 Answers
2
active
oldest
votes
up vote
0
down vote
You can do it this way, (sorry for bad formatting)
Make sure to add getter/setter methods in your structure class.
JSONObject jsonObj = new JSONObject(responseString);
Response response = new Response();
if(jsonObj.has("status")){
response.setStatus(jsonObj.getString("status"));
}
if(jsonObj.has("items")) {
JSONArray array = jsonObj.getJSONArray("items");
ArrayList<Items> list =new ArrayList();
for(i=0;i<array.length;i++){
JSONObject json = array.get(i);
Items item = new Items();
item.setId(json.getString("id"));
item.setName(json.getString("name"));
item.setCountry(json.getString("country"));
list.add(item);
}
}
response.setItems(list);
1
Is there any specific reason for not suggesting JSON parsing libraries like Gson, Jackson or Moshi in your answer? I'm not telling your procedure is wrong or shouldn't be followed but it makes code very long and tomorrow if we want to change anykey
in JSON structure this code style make developer's life worse. :)
– Shashanth
Nov 19 at 3:47
The disadvantage of using library is when you apply proguard rules and want to obfuscate your code, these classes will be excluded as the proguard rules for these library may be different, which itself is a huge reason for security purpose.
– Kinjal Rathod
Nov 19 at 5:03
add a comment |
up vote
0
down vote
Hi Just Ahead,
Put your JSON to any JSON parser like here http://www.jsonschema2pojo.org/
Than you need to select Parcelable / Serializable and you will get automatically generated POJO classes.
Than you have to set up retrofit instance with okHttp / gson, create dao interface where you implement your all methods to call your JSON.
New contributor
Wiktor Kalinowski is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
You can do it this way, (sorry for bad formatting)
Make sure to add getter/setter methods in your structure class.
JSONObject jsonObj = new JSONObject(responseString);
Response response = new Response();
if(jsonObj.has("status")){
response.setStatus(jsonObj.getString("status"));
}
if(jsonObj.has("items")) {
JSONArray array = jsonObj.getJSONArray("items");
ArrayList<Items> list =new ArrayList();
for(i=0;i<array.length;i++){
JSONObject json = array.get(i);
Items item = new Items();
item.setId(json.getString("id"));
item.setName(json.getString("name"));
item.setCountry(json.getString("country"));
list.add(item);
}
}
response.setItems(list);
1
Is there any specific reason for not suggesting JSON parsing libraries like Gson, Jackson or Moshi in your answer? I'm not telling your procedure is wrong or shouldn't be followed but it makes code very long and tomorrow if we want to change anykey
in JSON structure this code style make developer's life worse. :)
– Shashanth
Nov 19 at 3:47
The disadvantage of using library is when you apply proguard rules and want to obfuscate your code, these classes will be excluded as the proguard rules for these library may be different, which itself is a huge reason for security purpose.
– Kinjal Rathod
Nov 19 at 5:03
add a comment |
up vote
0
down vote
You can do it this way, (sorry for bad formatting)
Make sure to add getter/setter methods in your structure class.
JSONObject jsonObj = new JSONObject(responseString);
Response response = new Response();
if(jsonObj.has("status")){
response.setStatus(jsonObj.getString("status"));
}
if(jsonObj.has("items")) {
JSONArray array = jsonObj.getJSONArray("items");
ArrayList<Items> list =new ArrayList();
for(i=0;i<array.length;i++){
JSONObject json = array.get(i);
Items item = new Items();
item.setId(json.getString("id"));
item.setName(json.getString("name"));
item.setCountry(json.getString("country"));
list.add(item);
}
}
response.setItems(list);
1
Is there any specific reason for not suggesting JSON parsing libraries like Gson, Jackson or Moshi in your answer? I'm not telling your procedure is wrong or shouldn't be followed but it makes code very long and tomorrow if we want to change anykey
in JSON structure this code style make developer's life worse. :)
– Shashanth
Nov 19 at 3:47
The disadvantage of using library is when you apply proguard rules and want to obfuscate your code, these classes will be excluded as the proguard rules for these library may be different, which itself is a huge reason for security purpose.
– Kinjal Rathod
Nov 19 at 5:03
add a comment |
up vote
0
down vote
up vote
0
down vote
You can do it this way, (sorry for bad formatting)
Make sure to add getter/setter methods in your structure class.
JSONObject jsonObj = new JSONObject(responseString);
Response response = new Response();
if(jsonObj.has("status")){
response.setStatus(jsonObj.getString("status"));
}
if(jsonObj.has("items")) {
JSONArray array = jsonObj.getJSONArray("items");
ArrayList<Items> list =new ArrayList();
for(i=0;i<array.length;i++){
JSONObject json = array.get(i);
Items item = new Items();
item.setId(json.getString("id"));
item.setName(json.getString("name"));
item.setCountry(json.getString("country"));
list.add(item);
}
}
response.setItems(list);
You can do it this way, (sorry for bad formatting)
Make sure to add getter/setter methods in your structure class.
JSONObject jsonObj = new JSONObject(responseString);
Response response = new Response();
if(jsonObj.has("status")){
response.setStatus(jsonObj.getString("status"));
}
if(jsonObj.has("items")) {
JSONArray array = jsonObj.getJSONArray("items");
ArrayList<Items> list =new ArrayList();
for(i=0;i<array.length;i++){
JSONObject json = array.get(i);
Items item = new Items();
item.setId(json.getString("id"));
item.setName(json.getString("name"));
item.setCountry(json.getString("country"));
list.add(item);
}
}
response.setItems(list);
edited Nov 18 at 10:10
answered Nov 18 at 10:03
Kinjal Rathod
33125
33125
1
Is there any specific reason for not suggesting JSON parsing libraries like Gson, Jackson or Moshi in your answer? I'm not telling your procedure is wrong or shouldn't be followed but it makes code very long and tomorrow if we want to change anykey
in JSON structure this code style make developer's life worse. :)
– Shashanth
Nov 19 at 3:47
The disadvantage of using library is when you apply proguard rules and want to obfuscate your code, these classes will be excluded as the proguard rules for these library may be different, which itself is a huge reason for security purpose.
– Kinjal Rathod
Nov 19 at 5:03
add a comment |
1
Is there any specific reason for not suggesting JSON parsing libraries like Gson, Jackson or Moshi in your answer? I'm not telling your procedure is wrong or shouldn't be followed but it makes code very long and tomorrow if we want to change anykey
in JSON structure this code style make developer's life worse. :)
– Shashanth
Nov 19 at 3:47
The disadvantage of using library is when you apply proguard rules and want to obfuscate your code, these classes will be excluded as the proguard rules for these library may be different, which itself is a huge reason for security purpose.
– Kinjal Rathod
Nov 19 at 5:03
1
1
Is there any specific reason for not suggesting JSON parsing libraries like Gson, Jackson or Moshi in your answer? I'm not telling your procedure is wrong or shouldn't be followed but it makes code very long and tomorrow if we want to change any
key
in JSON structure this code style make developer's life worse. :)– Shashanth
Nov 19 at 3:47
Is there any specific reason for not suggesting JSON parsing libraries like Gson, Jackson or Moshi in your answer? I'm not telling your procedure is wrong or shouldn't be followed but it makes code very long and tomorrow if we want to change any
key
in JSON structure this code style make developer's life worse. :)– Shashanth
Nov 19 at 3:47
The disadvantage of using library is when you apply proguard rules and want to obfuscate your code, these classes will be excluded as the proguard rules for these library may be different, which itself is a huge reason for security purpose.
– Kinjal Rathod
Nov 19 at 5:03
The disadvantage of using library is when you apply proguard rules and want to obfuscate your code, these classes will be excluded as the proguard rules for these library may be different, which itself is a huge reason for security purpose.
– Kinjal Rathod
Nov 19 at 5:03
add a comment |
up vote
0
down vote
Hi Just Ahead,
Put your JSON to any JSON parser like here http://www.jsonschema2pojo.org/
Than you need to select Parcelable / Serializable and you will get automatically generated POJO classes.
Than you have to set up retrofit instance with okHttp / gson, create dao interface where you implement your all methods to call your JSON.
New contributor
Wiktor Kalinowski is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
up vote
0
down vote
Hi Just Ahead,
Put your JSON to any JSON parser like here http://www.jsonschema2pojo.org/
Than you need to select Parcelable / Serializable and you will get automatically generated POJO classes.
Than you have to set up retrofit instance with okHttp / gson, create dao interface where you implement your all methods to call your JSON.
New contributor
Wiktor Kalinowski is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
up vote
0
down vote
up vote
0
down vote
Hi Just Ahead,
Put your JSON to any JSON parser like here http://www.jsonschema2pojo.org/
Than you need to select Parcelable / Serializable and you will get automatically generated POJO classes.
Than you have to set up retrofit instance with okHttp / gson, create dao interface where you implement your all methods to call your JSON.
New contributor
Wiktor Kalinowski is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
Hi Just Ahead,
Put your JSON to any JSON parser like here http://www.jsonschema2pojo.org/
Than you need to select Parcelable / Serializable and you will get automatically generated POJO classes.
Than you have to set up retrofit instance with okHttp / gson, create dao interface where you implement your all methods to call your JSON.
New contributor
Wiktor Kalinowski is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
Wiktor Kalinowski is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
answered Nov 18 at 10:45


Wiktor Kalinowski
112
112
New contributor
Wiktor Kalinowski is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
Wiktor Kalinowski is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
Wiktor Kalinowski is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
add a comment |
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53359259%2fhow-to-resolve-complex-json-and-put-it-in-list-into-list-using-retrofit%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
FF3ZUKEWJOiiP,g8E C7j7ruN9YVWFXSUD5s,x
You'll have to write some code to do the structure conversion.
– Henry
Nov 18 at 9:00
I don't know how to create list in the list
– Just Ahead
Nov 18 at 9:01
@JustAhead have you tried expandable listview - androidhive.info/2013/07/android-expandable-list-view-tutorial
– Navneet Krishna
Nov 18 at 10:15