Laravel Eloquent - Pivot Table
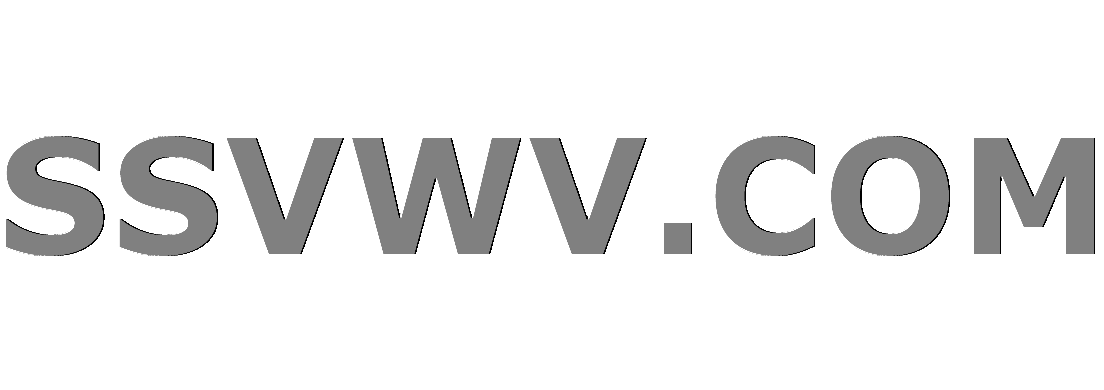
Multi tool use
up vote
0
down vote
favorite
i have this tables:
Attendances
id | member_id
1 | 1
2 | 2
3 | 3
Members
id | name
1 | Joe
2 | Jane
3 | David
Positions
id | position_name
1 | art
2 | singer
3 | dancer
member_position
member_id | position_id
1 | 2
1 | 1
1 | 3
2 | 1
2 | 2
3 | 3
From Attendances table, how can i select all who attended that are singers using laravel eloquent?
====EDIT=====
Attendance model:
public function member()
{
return $this->belongsTo('AppMember');
}
Member model:
public function positions()
{
return $this->belongsToMany('AppPosition');
}
Position model:
public function members()
{
return $this->belongsToMany('AppMember');
}
laravel
add a comment |
up vote
0
down vote
favorite
i have this tables:
Attendances
id | member_id
1 | 1
2 | 2
3 | 3
Members
id | name
1 | Joe
2 | Jane
3 | David
Positions
id | position_name
1 | art
2 | singer
3 | dancer
member_position
member_id | position_id
1 | 2
1 | 1
1 | 3
2 | 1
2 | 2
3 | 3
From Attendances table, how can i select all who attended that are singers using laravel eloquent?
====EDIT=====
Attendance model:
public function member()
{
return $this->belongsTo('AppMember');
}
Member model:
public function positions()
{
return $this->belongsToMany('AppPosition');
}
Position model:
public function members()
{
return $this->belongsToMany('AppMember');
}
laravel
Attach your models relations
– IndianCoding
Nov 17 at 14:29
@IndianCoding - added
– kapitan
Nov 17 at 14:33
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
i have this tables:
Attendances
id | member_id
1 | 1
2 | 2
3 | 3
Members
id | name
1 | Joe
2 | Jane
3 | David
Positions
id | position_name
1 | art
2 | singer
3 | dancer
member_position
member_id | position_id
1 | 2
1 | 1
1 | 3
2 | 1
2 | 2
3 | 3
From Attendances table, how can i select all who attended that are singers using laravel eloquent?
====EDIT=====
Attendance model:
public function member()
{
return $this->belongsTo('AppMember');
}
Member model:
public function positions()
{
return $this->belongsToMany('AppPosition');
}
Position model:
public function members()
{
return $this->belongsToMany('AppMember');
}
laravel
i have this tables:
Attendances
id | member_id
1 | 1
2 | 2
3 | 3
Members
id | name
1 | Joe
2 | Jane
3 | David
Positions
id | position_name
1 | art
2 | singer
3 | dancer
member_position
member_id | position_id
1 | 2
1 | 1
1 | 3
2 | 1
2 | 2
3 | 3
From Attendances table, how can i select all who attended that are singers using laravel eloquent?
====EDIT=====
Attendance model:
public function member()
{
return $this->belongsTo('AppMember');
}
Member model:
public function positions()
{
return $this->belongsToMany('AppPosition');
}
Position model:
public function members()
{
return $this->belongsToMany('AppMember');
}
laravel
laravel
edited Nov 17 at 14:33
asked Nov 17 at 14:18
kapitan
1389
1389
Attach your models relations
– IndianCoding
Nov 17 at 14:29
@IndianCoding - added
– kapitan
Nov 17 at 14:33
add a comment |
Attach your models relations
– IndianCoding
Nov 17 at 14:29
@IndianCoding - added
– kapitan
Nov 17 at 14:33
Attach your models relations
– IndianCoding
Nov 17 at 14:29
Attach your models relations
– IndianCoding
Nov 17 at 14:29
@IndianCoding - added
– kapitan
Nov 17 at 14:33
@IndianCoding - added
– kapitan
Nov 17 at 14:33
add a comment |
2 Answers
2
active
oldest
votes
up vote
1
down vote
accepted
You should use whereHas method with nested relations.
$attendances = Attendance::with('member')
->whereHas('member.positions', function($query){
$query->where('id', 2);
})
->get();
foreach($attendances as $attendance){
echo $attendance->member->id;
}
New contributor
IndianCoding is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
up vote
1
down vote
I'm positive this will give you the attendances whose member is a singer, with a nested member relationship model.
$attendances = Attendance::whereHas('member.positions', function($query) {
$query->where('position_name', 'singers');
})->with('member')->get();
And then, you can iterate through your results as so:
foreach($attendances as $attendance){
echo $attendance->member->id;
echo $attendance->member->name;
}
add a comment |
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
1
down vote
accepted
You should use whereHas method with nested relations.
$attendances = Attendance::with('member')
->whereHas('member.positions', function($query){
$query->where('id', 2);
})
->get();
foreach($attendances as $attendance){
echo $attendance->member->id;
}
New contributor
IndianCoding is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
up vote
1
down vote
accepted
You should use whereHas method with nested relations.
$attendances = Attendance::with('member')
->whereHas('member.positions', function($query){
$query->where('id', 2);
})
->get();
foreach($attendances as $attendance){
echo $attendance->member->id;
}
New contributor
IndianCoding is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
up vote
1
down vote
accepted
up vote
1
down vote
accepted
You should use whereHas method with nested relations.
$attendances = Attendance::with('member')
->whereHas('member.positions', function($query){
$query->where('id', 2);
})
->get();
foreach($attendances as $attendance){
echo $attendance->member->id;
}
New contributor
IndianCoding is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
You should use whereHas method with nested relations.
$attendances = Attendance::with('member')
->whereHas('member.positions', function($query){
$query->where('id', 2);
})
->get();
foreach($attendances as $attendance){
echo $attendance->member->id;
}
New contributor
IndianCoding is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
IndianCoding is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
answered Nov 17 at 14:41
IndianCoding
2967
2967
New contributor
IndianCoding is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
IndianCoding is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
IndianCoding is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
add a comment |
up vote
1
down vote
I'm positive this will give you the attendances whose member is a singer, with a nested member relationship model.
$attendances = Attendance::whereHas('member.positions', function($query) {
$query->where('position_name', 'singers');
})->with('member')->get();
And then, you can iterate through your results as so:
foreach($attendances as $attendance){
echo $attendance->member->id;
echo $attendance->member->name;
}
add a comment |
up vote
1
down vote
I'm positive this will give you the attendances whose member is a singer, with a nested member relationship model.
$attendances = Attendance::whereHas('member.positions', function($query) {
$query->where('position_name', 'singers');
})->with('member')->get();
And then, you can iterate through your results as so:
foreach($attendances as $attendance){
echo $attendance->member->id;
echo $attendance->member->name;
}
add a comment |
up vote
1
down vote
up vote
1
down vote
I'm positive this will give you the attendances whose member is a singer, with a nested member relationship model.
$attendances = Attendance::whereHas('member.positions', function($query) {
$query->where('position_name', 'singers');
})->with('member')->get();
And then, you can iterate through your results as so:
foreach($attendances as $attendance){
echo $attendance->member->id;
echo $attendance->member->name;
}
I'm positive this will give you the attendances whose member is a singer, with a nested member relationship model.
$attendances = Attendance::whereHas('member.positions', function($query) {
$query->where('position_name', 'singers');
})->with('member')->get();
And then, you can iterate through your results as so:
foreach($attendances as $attendance){
echo $attendance->member->id;
echo $attendance->member->name;
}
answered Nov 17 at 14:50
Oniya Daniel
198213
198213
add a comment |
add a comment |
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53352069%2flaravel-eloquent-pivot-table%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
pdz,8Sm ulMJq5egbK3,xm7Zt2dTybTn,nKe7b
Attach your models relations
– IndianCoding
Nov 17 at 14:29
@IndianCoding - added
– kapitan
Nov 17 at 14:33