Recursive implementation of palindrome test [on hold]
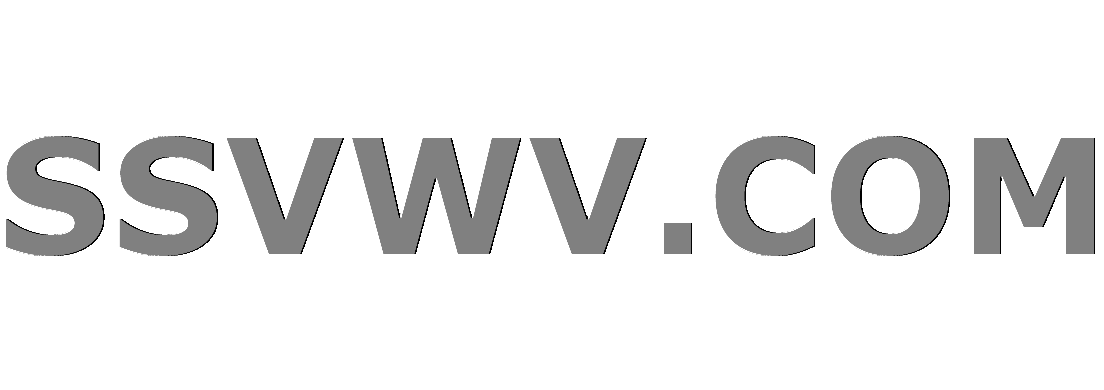
Multi tool use
up vote
0
down vote
favorite
I was asked to define a recursive function that
- takes in a string
and - returns True if the string is a palindrome, False if not
I thought since I must check string for whitespaces that could be the perfect opportunity to use a wrapper to include in my isPalindrome()
function.
My code:
def rem_spaces(string, g=''):
"""
parameters : string of type str;
returns : a string with all the spaces removed
"""
if len(string)==0:
return g
if string[0]!=' ':
return rem_spaces(string[1:], g+string[0])
return rem_spaces(string[1:], g)
def isPalindrome(string):
"""
parameters : string of type str
returns : True if the string is a palindrome, False if not
"""
string=rem_spaces(string)
if len(string) % 2 != 0:
return False
if len(string)==0:
return True
if string[0]==string[-1]:
return isPalindrome(string[1:-1])
return isPalindrome(string[1:-1])
print(isPalindrome('ferdihe '))
will output the following:
True
Is this an accepted way on how to use recursion/wrapper?
python recursion wrapper
put on hold as off-topic by l0b0, AJNeufeld, vnp, Martin R, Graipher 9 hours ago
This question appears to be off-topic. The users who voted to close gave this specific reason:
- "Code not implemented or not working as intended: Code Review is a community where programmers peer-review your working code to address issues such as security, maintainability, performance, and scalability. We require that the code be working correctly, to the best of the author's knowledge, before proceeding with a review." – l0b0, AJNeufeld, vnp, Martin R, Graipher
If this question can be reworded to fit the rules in the help center, please edit the question.
add a comment |
up vote
0
down vote
favorite
I was asked to define a recursive function that
- takes in a string
and - returns True if the string is a palindrome, False if not
I thought since I must check string for whitespaces that could be the perfect opportunity to use a wrapper to include in my isPalindrome()
function.
My code:
def rem_spaces(string, g=''):
"""
parameters : string of type str;
returns : a string with all the spaces removed
"""
if len(string)==0:
return g
if string[0]!=' ':
return rem_spaces(string[1:], g+string[0])
return rem_spaces(string[1:], g)
def isPalindrome(string):
"""
parameters : string of type str
returns : True if the string is a palindrome, False if not
"""
string=rem_spaces(string)
if len(string) % 2 != 0:
return False
if len(string)==0:
return True
if string[0]==string[-1]:
return isPalindrome(string[1:-1])
return isPalindrome(string[1:-1])
print(isPalindrome('ferdihe '))
will output the following:
True
Is this an accepted way on how to use recursion/wrapper?
python recursion wrapper
put on hold as off-topic by l0b0, AJNeufeld, vnp, Martin R, Graipher 9 hours ago
This question appears to be off-topic. The users who voted to close gave this specific reason:
- "Code not implemented or not working as intended: Code Review is a community where programmers peer-review your working code to address issues such as security, maintainability, performance, and scalability. We require that the code be working correctly, to the best of the author's knowledge, before proceeding with a review." – l0b0, AJNeufeld, vnp, Martin R, Graipher
If this question can be reworded to fit the rules in the help center, please edit the question.
2
Why would you remove spaces? Also, "ferdihe" isn't a palindrome, but you say it's considered to be one.
– l0b0
yesterday
What @l0b0 said. "Checking for spaces" sounds like you just want to runstrip()
before processing.
– Reinderien
yesterday
1
Your program does not recognize "aba" as a palindrome.
– Martin R
18 hours ago
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I was asked to define a recursive function that
- takes in a string
and - returns True if the string is a palindrome, False if not
I thought since I must check string for whitespaces that could be the perfect opportunity to use a wrapper to include in my isPalindrome()
function.
My code:
def rem_spaces(string, g=''):
"""
parameters : string of type str;
returns : a string with all the spaces removed
"""
if len(string)==0:
return g
if string[0]!=' ':
return rem_spaces(string[1:], g+string[0])
return rem_spaces(string[1:], g)
def isPalindrome(string):
"""
parameters : string of type str
returns : True if the string is a palindrome, False if not
"""
string=rem_spaces(string)
if len(string) % 2 != 0:
return False
if len(string)==0:
return True
if string[0]==string[-1]:
return isPalindrome(string[1:-1])
return isPalindrome(string[1:-1])
print(isPalindrome('ferdihe '))
will output the following:
True
Is this an accepted way on how to use recursion/wrapper?
python recursion wrapper
I was asked to define a recursive function that
- takes in a string
and - returns True if the string is a palindrome, False if not
I thought since I must check string for whitespaces that could be the perfect opportunity to use a wrapper to include in my isPalindrome()
function.
My code:
def rem_spaces(string, g=''):
"""
parameters : string of type str;
returns : a string with all the spaces removed
"""
if len(string)==0:
return g
if string[0]!=' ':
return rem_spaces(string[1:], g+string[0])
return rem_spaces(string[1:], g)
def isPalindrome(string):
"""
parameters : string of type str
returns : True if the string is a palindrome, False if not
"""
string=rem_spaces(string)
if len(string) % 2 != 0:
return False
if len(string)==0:
return True
if string[0]==string[-1]:
return isPalindrome(string[1:-1])
return isPalindrome(string[1:-1])
print(isPalindrome('ferdihe '))
will output the following:
True
Is this an accepted way on how to use recursion/wrapper?
python recursion wrapper
python recursion wrapper
edited 18 hours ago
Toby Speight
22k536108
22k536108
asked yesterday
Mister Tusk
454
454
put on hold as off-topic by l0b0, AJNeufeld, vnp, Martin R, Graipher 9 hours ago
This question appears to be off-topic. The users who voted to close gave this specific reason:
- "Code not implemented or not working as intended: Code Review is a community where programmers peer-review your working code to address issues such as security, maintainability, performance, and scalability. We require that the code be working correctly, to the best of the author's knowledge, before proceeding with a review." – l0b0, AJNeufeld, vnp, Martin R, Graipher
If this question can be reworded to fit the rules in the help center, please edit the question.
put on hold as off-topic by l0b0, AJNeufeld, vnp, Martin R, Graipher 9 hours ago
This question appears to be off-topic. The users who voted to close gave this specific reason:
- "Code not implemented or not working as intended: Code Review is a community where programmers peer-review your working code to address issues such as security, maintainability, performance, and scalability. We require that the code be working correctly, to the best of the author's knowledge, before proceeding with a review." – l0b0, AJNeufeld, vnp, Martin R, Graipher
If this question can be reworded to fit the rules in the help center, please edit the question.
2
Why would you remove spaces? Also, "ferdihe" isn't a palindrome, but you say it's considered to be one.
– l0b0
yesterday
What @l0b0 said. "Checking for spaces" sounds like you just want to runstrip()
before processing.
– Reinderien
yesterday
1
Your program does not recognize "aba" as a palindrome.
– Martin R
18 hours ago
add a comment |
2
Why would you remove spaces? Also, "ferdihe" isn't a palindrome, but you say it's considered to be one.
– l0b0
yesterday
What @l0b0 said. "Checking for spaces" sounds like you just want to runstrip()
before processing.
– Reinderien
yesterday
1
Your program does not recognize "aba" as a palindrome.
– Martin R
18 hours ago
2
2
Why would you remove spaces? Also, "ferdihe" isn't a palindrome, but you say it's considered to be one.
– l0b0
yesterday
Why would you remove spaces? Also, "ferdihe" isn't a palindrome, but you say it's considered to be one.
– l0b0
yesterday
What @l0b0 said. "Checking for spaces" sounds like you just want to run
strip()
before processing.– Reinderien
yesterday
What @l0b0 said. "Checking for spaces" sounds like you just want to run
strip()
before processing.– Reinderien
yesterday
1
1
Your program does not recognize "aba" as a palindrome.
– Martin R
18 hours ago
Your program does not recognize "aba" as a palindrome.
– Martin R
18 hours ago
add a comment |
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
QthLVLW,5PdQ8hH2rDFGwwRZbUFM9,C,sBD0Os2fbmX3W6AvrUdjZXKWUBs,RV b97,dSTyIPMOc5zgkGBWyhj,vh,etvuc BM
2
Why would you remove spaces? Also, "ferdihe" isn't a palindrome, but you say it's considered to be one.
– l0b0
yesterday
What @l0b0 said. "Checking for spaces" sounds like you just want to run
strip()
before processing.– Reinderien
yesterday
1
Your program does not recognize "aba" as a palindrome.
– Martin R
18 hours ago