spyOn not able to spy on the method of a dependent service
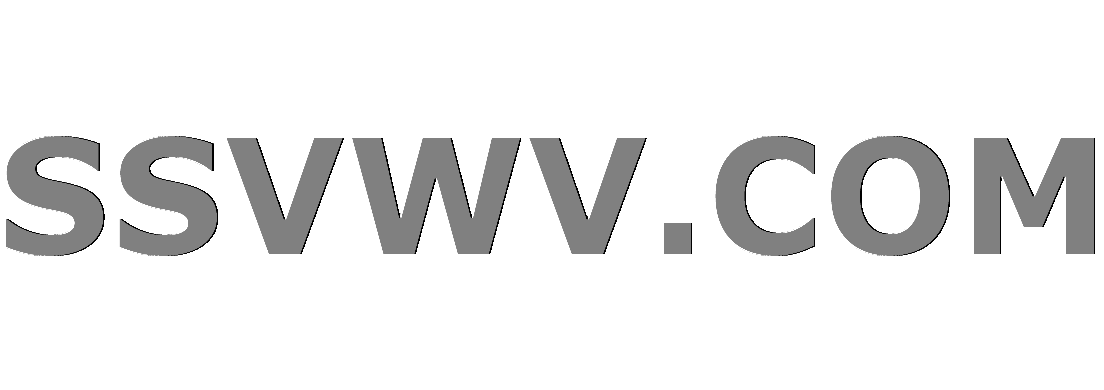
Multi tool use
up vote
0
down vote
favorite
I am testing AuthService
which sends user login info to server using another HelperService
.
public authServiceSigninUser(user:UserSigninInfo):any{
console.log('In authServiceSigninUser. contacting server at '+this.API_URL +this.SIGNIN_USER_URL +" with user data "+user+ " with httpOptions "+httpOptions.withCredentials + ","+httpOptions.headers ); //TODOM password should be sent in encrypted format.
let signinInfo= new UserSigninAPI(user);
let body = JSON.stringify(signinInfo);
return this.helperService.sendMessage(this.SIGNIN_USER_URL,body,httpOptions)
}
I am trying to test the authServiceSigninUser
method as follows but when I run the spec, I get error Error: <spyOn> : sendMessage() method does not exist
. Why?
Usage: spyOn(<object>, <methodName>)
describe('authServiceSigninUser test suite',()=>{
beforeEach(()=>{
TestBed.configureTestingModule({
imports: [HttpClientTestingModule],
providers: [AuthService, HelperService]
});
});
fit('should sign in user',()=>{
let spy:any;
let helper = TestBed.get(HelperService);
let authService = TestBed.get(AuthService);
let userSignIn = new UserSigninInfo("test@test.com","test");
let httpMock = TestBed.get(HttpTestingController);
spyOn(helper.sendMessage,'sendMessage');
let observable:Observable<HttpEvent<any>> = authService.authServiceSigninUser(userSignIn);
let subscription = observable.subscribe((event)=>{
console.log('event from authService',event);
});
const responseData = { result: 'success', ['additional-info']: 'login success' };
let httpEvent:HttpResponse<any> = new HttpResponse<any>({body:responseData});
expect(helper.sendMessage).toHaveBeenCalled();//ERROR here
const mockReq:TestRequest = httpMock.expectOne(environment.apiUrl+environment.signinUserUrl); //Expect that a single request has been made which matches the given URL, and return its mock
//once mocking of sending request is done, mock receiving a response. This will trigger the logic inside subscribe function
mockReq.flush(httpEvent); //flush method provides dummy values as response
httpMock.verify();//verify checks that there are no outstanding requests;
});
});
angular6 angular-test angular-testing
add a comment |
up vote
0
down vote
favorite
I am testing AuthService
which sends user login info to server using another HelperService
.
public authServiceSigninUser(user:UserSigninInfo):any{
console.log('In authServiceSigninUser. contacting server at '+this.API_URL +this.SIGNIN_USER_URL +" with user data "+user+ " with httpOptions "+httpOptions.withCredentials + ","+httpOptions.headers ); //TODOM password should be sent in encrypted format.
let signinInfo= new UserSigninAPI(user);
let body = JSON.stringify(signinInfo);
return this.helperService.sendMessage(this.SIGNIN_USER_URL,body,httpOptions)
}
I am trying to test the authServiceSigninUser
method as follows but when I run the spec, I get error Error: <spyOn> : sendMessage() method does not exist
. Why?
Usage: spyOn(<object>, <methodName>)
describe('authServiceSigninUser test suite',()=>{
beforeEach(()=>{
TestBed.configureTestingModule({
imports: [HttpClientTestingModule],
providers: [AuthService, HelperService]
});
});
fit('should sign in user',()=>{
let spy:any;
let helper = TestBed.get(HelperService);
let authService = TestBed.get(AuthService);
let userSignIn = new UserSigninInfo("test@test.com","test");
let httpMock = TestBed.get(HttpTestingController);
spyOn(helper.sendMessage,'sendMessage');
let observable:Observable<HttpEvent<any>> = authService.authServiceSigninUser(userSignIn);
let subscription = observable.subscribe((event)=>{
console.log('event from authService',event);
});
const responseData = { result: 'success', ['additional-info']: 'login success' };
let httpEvent:HttpResponse<any> = new HttpResponse<any>({body:responseData});
expect(helper.sendMessage).toHaveBeenCalled();//ERROR here
const mockReq:TestRequest = httpMock.expectOne(environment.apiUrl+environment.signinUserUrl); //Expect that a single request has been made which matches the given URL, and return its mock
//once mocking of sending request is done, mock receiving a response. This will trigger the logic inside subscribe function
mockReq.flush(httpEvent); //flush method provides dummy values as response
httpMock.verify();//verify checks that there are no outstanding requests;
});
});
angular6 angular-test angular-testing
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I am testing AuthService
which sends user login info to server using another HelperService
.
public authServiceSigninUser(user:UserSigninInfo):any{
console.log('In authServiceSigninUser. contacting server at '+this.API_URL +this.SIGNIN_USER_URL +" with user data "+user+ " with httpOptions "+httpOptions.withCredentials + ","+httpOptions.headers ); //TODOM password should be sent in encrypted format.
let signinInfo= new UserSigninAPI(user);
let body = JSON.stringify(signinInfo);
return this.helperService.sendMessage(this.SIGNIN_USER_URL,body,httpOptions)
}
I am trying to test the authServiceSigninUser
method as follows but when I run the spec, I get error Error: <spyOn> : sendMessage() method does not exist
. Why?
Usage: spyOn(<object>, <methodName>)
describe('authServiceSigninUser test suite',()=>{
beforeEach(()=>{
TestBed.configureTestingModule({
imports: [HttpClientTestingModule],
providers: [AuthService, HelperService]
});
});
fit('should sign in user',()=>{
let spy:any;
let helper = TestBed.get(HelperService);
let authService = TestBed.get(AuthService);
let userSignIn = new UserSigninInfo("test@test.com","test");
let httpMock = TestBed.get(HttpTestingController);
spyOn(helper.sendMessage,'sendMessage');
let observable:Observable<HttpEvent<any>> = authService.authServiceSigninUser(userSignIn);
let subscription = observable.subscribe((event)=>{
console.log('event from authService',event);
});
const responseData = { result: 'success', ['additional-info']: 'login success' };
let httpEvent:HttpResponse<any> = new HttpResponse<any>({body:responseData});
expect(helper.sendMessage).toHaveBeenCalled();//ERROR here
const mockReq:TestRequest = httpMock.expectOne(environment.apiUrl+environment.signinUserUrl); //Expect that a single request has been made which matches the given URL, and return its mock
//once mocking of sending request is done, mock receiving a response. This will trigger the logic inside subscribe function
mockReq.flush(httpEvent); //flush method provides dummy values as response
httpMock.verify();//verify checks that there are no outstanding requests;
});
});
angular6 angular-test angular-testing
I am testing AuthService
which sends user login info to server using another HelperService
.
public authServiceSigninUser(user:UserSigninInfo):any{
console.log('In authServiceSigninUser. contacting server at '+this.API_URL +this.SIGNIN_USER_URL +" with user data "+user+ " with httpOptions "+httpOptions.withCredentials + ","+httpOptions.headers ); //TODOM password should be sent in encrypted format.
let signinInfo= new UserSigninAPI(user);
let body = JSON.stringify(signinInfo);
return this.helperService.sendMessage(this.SIGNIN_USER_URL,body,httpOptions)
}
I am trying to test the authServiceSigninUser
method as follows but when I run the spec, I get error Error: <spyOn> : sendMessage() method does not exist
. Why?
Usage: spyOn(<object>, <methodName>)
describe('authServiceSigninUser test suite',()=>{
beforeEach(()=>{
TestBed.configureTestingModule({
imports: [HttpClientTestingModule],
providers: [AuthService, HelperService]
});
});
fit('should sign in user',()=>{
let spy:any;
let helper = TestBed.get(HelperService);
let authService = TestBed.get(AuthService);
let userSignIn = new UserSigninInfo("test@test.com","test");
let httpMock = TestBed.get(HttpTestingController);
spyOn(helper.sendMessage,'sendMessage');
let observable:Observable<HttpEvent<any>> = authService.authServiceSigninUser(userSignIn);
let subscription = observable.subscribe((event)=>{
console.log('event from authService',event);
});
const responseData = { result: 'success', ['additional-info']: 'login success' };
let httpEvent:HttpResponse<any> = new HttpResponse<any>({body:responseData});
expect(helper.sendMessage).toHaveBeenCalled();//ERROR here
const mockReq:TestRequest = httpMock.expectOne(environment.apiUrl+environment.signinUserUrl); //Expect that a single request has been made which matches the given URL, and return its mock
//once mocking of sending request is done, mock receiving a response. This will trigger the logic inside subscribe function
mockReq.flush(httpEvent); //flush method provides dummy values as response
httpMock.verify();//verify checks that there are no outstanding requests;
});
});
angular6 angular-test angular-testing
angular6 angular-test angular-testing
asked Nov 17 at 14:26
Manu Chadha
2,49411235
2,49411235
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
up vote
0
down vote
It seems the correct way is spyOn(helper,'sendMessage');
, not spyOn(helper.sendMessage,'sendMessage');
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
It seems the correct way is spyOn(helper,'sendMessage');
, not spyOn(helper.sendMessage,'sendMessage');
add a comment |
up vote
0
down vote
It seems the correct way is spyOn(helper,'sendMessage');
, not spyOn(helper.sendMessage,'sendMessage');
add a comment |
up vote
0
down vote
up vote
0
down vote
It seems the correct way is spyOn(helper,'sendMessage');
, not spyOn(helper.sendMessage,'sendMessage');
It seems the correct way is spyOn(helper,'sendMessage');
, not spyOn(helper.sendMessage,'sendMessage');
answered Nov 17 at 14:54
Manu Chadha
2,49411235
2,49411235
add a comment |
add a comment |
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53352134%2fspyon-not-able-to-spy-on-the-method-of-a-dependent-service%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
ya87mn n75DPv5A muaANFVv52Qb4BWLEMdT1ExUkqaJS,O5tAPdFV4Yu MB t0,Budmze,LU44bA4O