Populate a TableView with ObservableMap that has ObservableList as a value
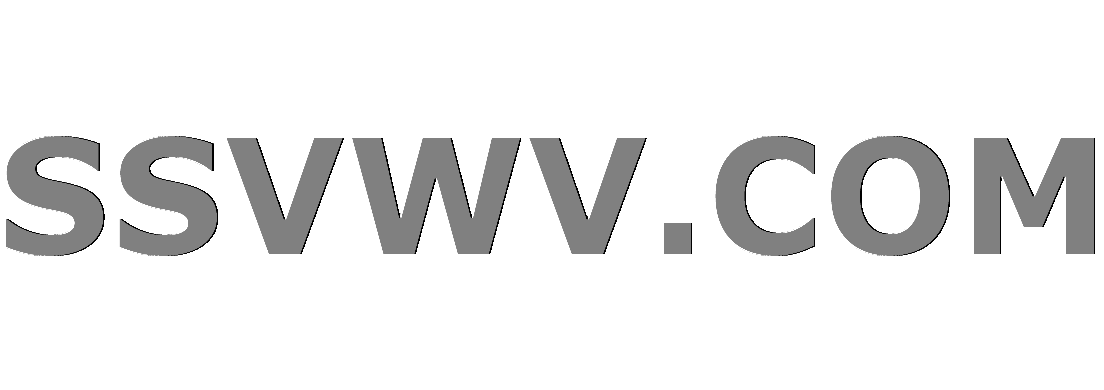
Multi tool use
up vote
1
down vote
favorite
I'm trying to create an ObservableMap that has ObservableList of custom objects as a value like this
Map<String, ObservableList<MyClass>> myMap = new HashMap<>();
ObservableMap<String, ObservableList<MyClass>> myObservableMap = FXCollections.observableMap(myMap);
After searching about binding of ObservableMap to tableview, I haven't found how it would be if the value is a collection. All that I've seen is binding of observable map that has a value of a custom class. I'm thinking that it should not be so different as I would only want the values of the keys to be binded to the tableview and keep track of the changes that would happen to the key and the values inside it.
Here's what I've done so far:
Map<String, ObservableList<DMSchedule>> myMap = new HashMap<>();
ObservableMap<String, ObservableList<DMSchedule>> myObservableMap = FXCollections.observableMap(myMap);
TableColumn<DMSchedule, String> dayColumn;
TableColumn<DMSchedule, String> subjectNameColumn;
TableColumn<DMSchedule, String> startTimeColumn;
TableColumn<DMSchedule, String> endTimeColumn;
@FXML
private TableView<DMSchedule> myTable;
@Override
public void initialize(URL url, ResourceBundle rb) {
final ListChangeListener<DMSchedule> listListener = new ListChangeListener<DMSchedule>() {
@Override
public void onChanged(ListChangeListener.Change<? extends DMSchedule> change) {
System.out.println("Detected a change!");
while (change.next()) {
System.out.println(change.getFrom());
System.out.println("Added on list? " + change.wasAdded());
System.out.println("Removed on list? " + change.wasRemoved());
}
}
};
dayColumn = new TableColumn<DMSchedule, String>("Day");
subjectNameColumn = new TableColumn<DMSchedule, String>("Subject");
startTimeColumn = new TableColumn<DMSchedule, String>("Start Time");
endTimeColumn = new TableColumn<DMSchedule, String>("End Time");
myObservableMap.addListener((MapChangeListener<String, ObservableList<DMSchedule>>) mapChange -> {
if (mapChange.wasAdded()) {
mapChange.getValueAdded().addListener(listListener);
}
if (mapChange.wasRemoved()) {
mapChange.getValueRemoved().addListener(listListener);
}
});
myObservableMap.put("M", FXCollections.observableArrayList());
myObservableMap.get("M").add(new DMSchedule("M", "07:00", "08:30", "testSubj", "testSection"));
myListOfMap.addAll(myObservableMap);
myTable.getColumns().addAll(dayColumn, subjectNameColumn, startTimeColumn, endTimeColumn);
myTable.setItems(myObservableMap);
And I'm kind of lost how will I set my ObservableList values to the table and columns.
I would want to interact with the table the same way as if I'm binding an ObservableList to the tableview. Like selecting tablerow would return MyClass
objects(assuming that a row factory has been made). I have already figured out how to add listeners for the change in value of keys and values inside a map. The only problem that I have left is to bind the ObservableList<MyClass>
of each keys inside myObservableMap
. How could I possibly do it? I hope that you could help me. Thanks!
java javafx observable
add a comment |
up vote
1
down vote
favorite
I'm trying to create an ObservableMap that has ObservableList of custom objects as a value like this
Map<String, ObservableList<MyClass>> myMap = new HashMap<>();
ObservableMap<String, ObservableList<MyClass>> myObservableMap = FXCollections.observableMap(myMap);
After searching about binding of ObservableMap to tableview, I haven't found how it would be if the value is a collection. All that I've seen is binding of observable map that has a value of a custom class. I'm thinking that it should not be so different as I would only want the values of the keys to be binded to the tableview and keep track of the changes that would happen to the key and the values inside it.
Here's what I've done so far:
Map<String, ObservableList<DMSchedule>> myMap = new HashMap<>();
ObservableMap<String, ObservableList<DMSchedule>> myObservableMap = FXCollections.observableMap(myMap);
TableColumn<DMSchedule, String> dayColumn;
TableColumn<DMSchedule, String> subjectNameColumn;
TableColumn<DMSchedule, String> startTimeColumn;
TableColumn<DMSchedule, String> endTimeColumn;
@FXML
private TableView<DMSchedule> myTable;
@Override
public void initialize(URL url, ResourceBundle rb) {
final ListChangeListener<DMSchedule> listListener = new ListChangeListener<DMSchedule>() {
@Override
public void onChanged(ListChangeListener.Change<? extends DMSchedule> change) {
System.out.println("Detected a change!");
while (change.next()) {
System.out.println(change.getFrom());
System.out.println("Added on list? " + change.wasAdded());
System.out.println("Removed on list? " + change.wasRemoved());
}
}
};
dayColumn = new TableColumn<DMSchedule, String>("Day");
subjectNameColumn = new TableColumn<DMSchedule, String>("Subject");
startTimeColumn = new TableColumn<DMSchedule, String>("Start Time");
endTimeColumn = new TableColumn<DMSchedule, String>("End Time");
myObservableMap.addListener((MapChangeListener<String, ObservableList<DMSchedule>>) mapChange -> {
if (mapChange.wasAdded()) {
mapChange.getValueAdded().addListener(listListener);
}
if (mapChange.wasRemoved()) {
mapChange.getValueRemoved().addListener(listListener);
}
});
myObservableMap.put("M", FXCollections.observableArrayList());
myObservableMap.get("M").add(new DMSchedule("M", "07:00", "08:30", "testSubj", "testSection"));
myListOfMap.addAll(myObservableMap);
myTable.getColumns().addAll(dayColumn, subjectNameColumn, startTimeColumn, endTimeColumn);
myTable.setItems(myObservableMap);
And I'm kind of lost how will I set my ObservableList values to the table and columns.
I would want to interact with the table the same way as if I'm binding an ObservableList to the tableview. Like selecting tablerow would return MyClass
objects(assuming that a row factory has been made). I have already figured out how to add listeners for the change in value of keys and values inside a map. The only problem that I have left is to bind the ObservableList<MyClass>
of each keys inside myObservableMap
. How could I possibly do it? I hope that you could help me. Thanks!
java javafx observable
Can you show what you have done so far?
– Gnas
Nov 17 at 15:44
add a comment |
up vote
1
down vote
favorite
up vote
1
down vote
favorite
I'm trying to create an ObservableMap that has ObservableList of custom objects as a value like this
Map<String, ObservableList<MyClass>> myMap = new HashMap<>();
ObservableMap<String, ObservableList<MyClass>> myObservableMap = FXCollections.observableMap(myMap);
After searching about binding of ObservableMap to tableview, I haven't found how it would be if the value is a collection. All that I've seen is binding of observable map that has a value of a custom class. I'm thinking that it should not be so different as I would only want the values of the keys to be binded to the tableview and keep track of the changes that would happen to the key and the values inside it.
Here's what I've done so far:
Map<String, ObservableList<DMSchedule>> myMap = new HashMap<>();
ObservableMap<String, ObservableList<DMSchedule>> myObservableMap = FXCollections.observableMap(myMap);
TableColumn<DMSchedule, String> dayColumn;
TableColumn<DMSchedule, String> subjectNameColumn;
TableColumn<DMSchedule, String> startTimeColumn;
TableColumn<DMSchedule, String> endTimeColumn;
@FXML
private TableView<DMSchedule> myTable;
@Override
public void initialize(URL url, ResourceBundle rb) {
final ListChangeListener<DMSchedule> listListener = new ListChangeListener<DMSchedule>() {
@Override
public void onChanged(ListChangeListener.Change<? extends DMSchedule> change) {
System.out.println("Detected a change!");
while (change.next()) {
System.out.println(change.getFrom());
System.out.println("Added on list? " + change.wasAdded());
System.out.println("Removed on list? " + change.wasRemoved());
}
}
};
dayColumn = new TableColumn<DMSchedule, String>("Day");
subjectNameColumn = new TableColumn<DMSchedule, String>("Subject");
startTimeColumn = new TableColumn<DMSchedule, String>("Start Time");
endTimeColumn = new TableColumn<DMSchedule, String>("End Time");
myObservableMap.addListener((MapChangeListener<String, ObservableList<DMSchedule>>) mapChange -> {
if (mapChange.wasAdded()) {
mapChange.getValueAdded().addListener(listListener);
}
if (mapChange.wasRemoved()) {
mapChange.getValueRemoved().addListener(listListener);
}
});
myObservableMap.put("M", FXCollections.observableArrayList());
myObservableMap.get("M").add(new DMSchedule("M", "07:00", "08:30", "testSubj", "testSection"));
myListOfMap.addAll(myObservableMap);
myTable.getColumns().addAll(dayColumn, subjectNameColumn, startTimeColumn, endTimeColumn);
myTable.setItems(myObservableMap);
And I'm kind of lost how will I set my ObservableList values to the table and columns.
I would want to interact with the table the same way as if I'm binding an ObservableList to the tableview. Like selecting tablerow would return MyClass
objects(assuming that a row factory has been made). I have already figured out how to add listeners for the change in value of keys and values inside a map. The only problem that I have left is to bind the ObservableList<MyClass>
of each keys inside myObservableMap
. How could I possibly do it? I hope that you could help me. Thanks!
java javafx observable
I'm trying to create an ObservableMap that has ObservableList of custom objects as a value like this
Map<String, ObservableList<MyClass>> myMap = new HashMap<>();
ObservableMap<String, ObservableList<MyClass>> myObservableMap = FXCollections.observableMap(myMap);
After searching about binding of ObservableMap to tableview, I haven't found how it would be if the value is a collection. All that I've seen is binding of observable map that has a value of a custom class. I'm thinking that it should not be so different as I would only want the values of the keys to be binded to the tableview and keep track of the changes that would happen to the key and the values inside it.
Here's what I've done so far:
Map<String, ObservableList<DMSchedule>> myMap = new HashMap<>();
ObservableMap<String, ObservableList<DMSchedule>> myObservableMap = FXCollections.observableMap(myMap);
TableColumn<DMSchedule, String> dayColumn;
TableColumn<DMSchedule, String> subjectNameColumn;
TableColumn<DMSchedule, String> startTimeColumn;
TableColumn<DMSchedule, String> endTimeColumn;
@FXML
private TableView<DMSchedule> myTable;
@Override
public void initialize(URL url, ResourceBundle rb) {
final ListChangeListener<DMSchedule> listListener = new ListChangeListener<DMSchedule>() {
@Override
public void onChanged(ListChangeListener.Change<? extends DMSchedule> change) {
System.out.println("Detected a change!");
while (change.next()) {
System.out.println(change.getFrom());
System.out.println("Added on list? " + change.wasAdded());
System.out.println("Removed on list? " + change.wasRemoved());
}
}
};
dayColumn = new TableColumn<DMSchedule, String>("Day");
subjectNameColumn = new TableColumn<DMSchedule, String>("Subject");
startTimeColumn = new TableColumn<DMSchedule, String>("Start Time");
endTimeColumn = new TableColumn<DMSchedule, String>("End Time");
myObservableMap.addListener((MapChangeListener<String, ObservableList<DMSchedule>>) mapChange -> {
if (mapChange.wasAdded()) {
mapChange.getValueAdded().addListener(listListener);
}
if (mapChange.wasRemoved()) {
mapChange.getValueRemoved().addListener(listListener);
}
});
myObservableMap.put("M", FXCollections.observableArrayList());
myObservableMap.get("M").add(new DMSchedule("M", "07:00", "08:30", "testSubj", "testSection"));
myListOfMap.addAll(myObservableMap);
myTable.getColumns().addAll(dayColumn, subjectNameColumn, startTimeColumn, endTimeColumn);
myTable.setItems(myObservableMap);
And I'm kind of lost how will I set my ObservableList values to the table and columns.
I would want to interact with the table the same way as if I'm binding an ObservableList to the tableview. Like selecting tablerow would return MyClass
objects(assuming that a row factory has been made). I have already figured out how to add listeners for the change in value of keys and values inside a map. The only problem that I have left is to bind the ObservableList<MyClass>
of each keys inside myObservableMap
. How could I possibly do it? I hope that you could help me. Thanks!
java javafx observable
java javafx observable
edited Nov 17 at 18:14
asked Nov 17 at 14:32
Fatnam
165
165
Can you show what you have done so far?
– Gnas
Nov 17 at 15:44
add a comment |
Can you show what you have done so far?
– Gnas
Nov 17 at 15:44
Can you show what you have done so far?
– Gnas
Nov 17 at 15:44
Can you show what you have done so far?
– Gnas
Nov 17 at 15:44
add a comment |
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53352182%2fpopulate-a-tableview-with-observablemap-that-has-observablelist-as-a-value%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
BKtcShS,21ItXUJOjbpRvyBlZZK75qQOGCo,wM6KTCIZ91mXUL4UBWD WO d3naaS q,XjX7 sMfvpCp,lZb,bagUm9Ro4N9SNqEUrKCt6u
Can you show what you have done so far?
– Gnas
Nov 17 at 15:44