Mars Rover challenge in Ruby [on hold]
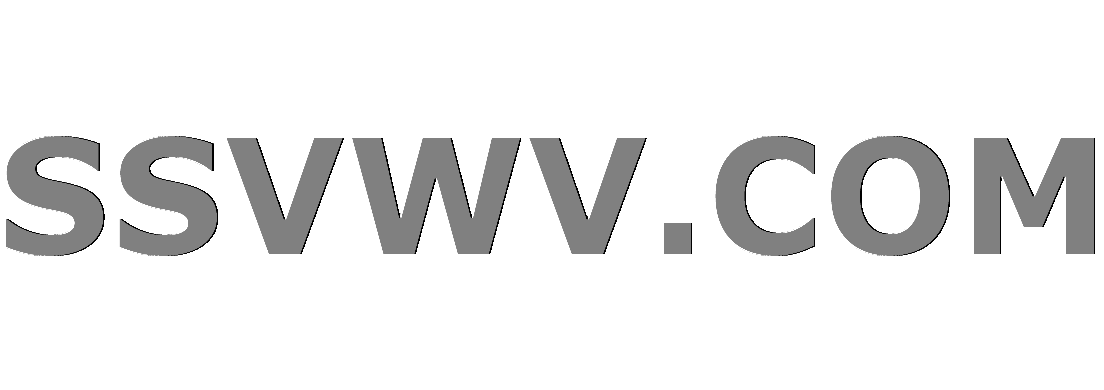
Multi tool use
$begingroup$
I have written a simplified version of the Mars Rover challenge in Ruby. I would like to try and rewrite it in JavaScript. Before that, would you review it for best coding practices perhaps and provide any advice about how to recode it JavaScript.
Working Code
class Rover
def initialize(x, y, direction)
@x = x
@y = y
@direction = direction
end
def instruction(position)
position.each do |input|
if input == 'L'
left
elsif input == 'R'
right
else
move
end
end
puts "this is where the rover should be: x= #{@x} y= #{@y} facing= #{@direction}"
end
def right
if @direction == 'S'
puts "the rover is facing West."
@direction = 'W'
elsif @direction == 'N'
puts "the rover is facting East"
@direction = 'E'
elsif @direction == 'W'
puts "the rover is facting North"
@direction = 'N'
else
puts "the rover is facing South"
@direction = 'S'
end
end
def left
if @direction == 'N'
puts "the rover is facing North."
@direction = 'W'
elsif @direction == 'W'
puts "the rover is facting West"
@direction = 'S'
elsif @direction == 'E'
puts "the rover is facting East"
@direction = 'N'
else
puts "the rover is facing South"
@direction = 'E'
end
end
def move
if @direction == 'N'
@y += 1
elsif @direction == 'E'
@x += 1
elsif @direction == 'S'
@y -= 1
else
@x -= 1
end
end
end
#instance of our rovers with direction x, y, direction facing N, E, S, or W
mars_rover_a = Rover.new(0,0,'N')
# mars_rover_a.move()
mars_rover_b = Rover.new(1,1,'E')
#call the instruction for each instance of the object rover
mars_rover_a.instruction(['L','M','R','M','L','M','R','R','M'])
mars_rover_b.instruction(['R','M','M','L','M','L','L','M'])
javascript ruby
New contributor
Kathy Toufighi is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
$endgroup$
put on hold as off-topic by Jamal♦ 35 mins ago
This question appears to be off-topic. The users who voted to close gave this specific reason:
- "Code not implemented or not working as intended: Code Review is a community where programmers peer-review your working code to address issues such as security, maintainability, performance, and scalability. We require that the code be working correctly, to the best of the author's knowledge, before proceeding with a review." – Jamal
If this question can be reworded to fit the rules in the help center, please edit the question.
add a comment |
$begingroup$
I have written a simplified version of the Mars Rover challenge in Ruby. I would like to try and rewrite it in JavaScript. Before that, would you review it for best coding practices perhaps and provide any advice about how to recode it JavaScript.
Working Code
class Rover
def initialize(x, y, direction)
@x = x
@y = y
@direction = direction
end
def instruction(position)
position.each do |input|
if input == 'L'
left
elsif input == 'R'
right
else
move
end
end
puts "this is where the rover should be: x= #{@x} y= #{@y} facing= #{@direction}"
end
def right
if @direction == 'S'
puts "the rover is facing West."
@direction = 'W'
elsif @direction == 'N'
puts "the rover is facting East"
@direction = 'E'
elsif @direction == 'W'
puts "the rover is facting North"
@direction = 'N'
else
puts "the rover is facing South"
@direction = 'S'
end
end
def left
if @direction == 'N'
puts "the rover is facing North."
@direction = 'W'
elsif @direction == 'W'
puts "the rover is facting West"
@direction = 'S'
elsif @direction == 'E'
puts "the rover is facting East"
@direction = 'N'
else
puts "the rover is facing South"
@direction = 'E'
end
end
def move
if @direction == 'N'
@y += 1
elsif @direction == 'E'
@x += 1
elsif @direction == 'S'
@y -= 1
else
@x -= 1
end
end
end
#instance of our rovers with direction x, y, direction facing N, E, S, or W
mars_rover_a = Rover.new(0,0,'N')
# mars_rover_a.move()
mars_rover_b = Rover.new(1,1,'E')
#call the instruction for each instance of the object rover
mars_rover_a.instruction(['L','M','R','M','L','M','R','R','M'])
mars_rover_b.instruction(['R','M','M','L','M','L','L','M'])
javascript ruby
New contributor
Kathy Toufighi is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
$endgroup$
put on hold as off-topic by Jamal♦ 35 mins ago
This question appears to be off-topic. The users who voted to close gave this specific reason:
- "Code not implemented or not working as intended: Code Review is a community where programmers peer-review your working code to address issues such as security, maintainability, performance, and scalability. We require that the code be working correctly, to the best of the author's knowledge, before proceeding with a review." – Jamal
If this question can be reworded to fit the rules in the help center, please edit the question.
add a comment |
$begingroup$
I have written a simplified version of the Mars Rover challenge in Ruby. I would like to try and rewrite it in JavaScript. Before that, would you review it for best coding practices perhaps and provide any advice about how to recode it JavaScript.
Working Code
class Rover
def initialize(x, y, direction)
@x = x
@y = y
@direction = direction
end
def instruction(position)
position.each do |input|
if input == 'L'
left
elsif input == 'R'
right
else
move
end
end
puts "this is where the rover should be: x= #{@x} y= #{@y} facing= #{@direction}"
end
def right
if @direction == 'S'
puts "the rover is facing West."
@direction = 'W'
elsif @direction == 'N'
puts "the rover is facting East"
@direction = 'E'
elsif @direction == 'W'
puts "the rover is facting North"
@direction = 'N'
else
puts "the rover is facing South"
@direction = 'S'
end
end
def left
if @direction == 'N'
puts "the rover is facing North."
@direction = 'W'
elsif @direction == 'W'
puts "the rover is facting West"
@direction = 'S'
elsif @direction == 'E'
puts "the rover is facting East"
@direction = 'N'
else
puts "the rover is facing South"
@direction = 'E'
end
end
def move
if @direction == 'N'
@y += 1
elsif @direction == 'E'
@x += 1
elsif @direction == 'S'
@y -= 1
else
@x -= 1
end
end
end
#instance of our rovers with direction x, y, direction facing N, E, S, or W
mars_rover_a = Rover.new(0,0,'N')
# mars_rover_a.move()
mars_rover_b = Rover.new(1,1,'E')
#call the instruction for each instance of the object rover
mars_rover_a.instruction(['L','M','R','M','L','M','R','R','M'])
mars_rover_b.instruction(['R','M','M','L','M','L','L','M'])
javascript ruby
New contributor
Kathy Toufighi is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
$endgroup$
I have written a simplified version of the Mars Rover challenge in Ruby. I would like to try and rewrite it in JavaScript. Before that, would you review it for best coding practices perhaps and provide any advice about how to recode it JavaScript.
Working Code
class Rover
def initialize(x, y, direction)
@x = x
@y = y
@direction = direction
end
def instruction(position)
position.each do |input|
if input == 'L'
left
elsif input == 'R'
right
else
move
end
end
puts "this is where the rover should be: x= #{@x} y= #{@y} facing= #{@direction}"
end
def right
if @direction == 'S'
puts "the rover is facing West."
@direction = 'W'
elsif @direction == 'N'
puts "the rover is facting East"
@direction = 'E'
elsif @direction == 'W'
puts "the rover is facting North"
@direction = 'N'
else
puts "the rover is facing South"
@direction = 'S'
end
end
def left
if @direction == 'N'
puts "the rover is facing North."
@direction = 'W'
elsif @direction == 'W'
puts "the rover is facting West"
@direction = 'S'
elsif @direction == 'E'
puts "the rover is facting East"
@direction = 'N'
else
puts "the rover is facing South"
@direction = 'E'
end
end
def move
if @direction == 'N'
@y += 1
elsif @direction == 'E'
@x += 1
elsif @direction == 'S'
@y -= 1
else
@x -= 1
end
end
end
#instance of our rovers with direction x, y, direction facing N, E, S, or W
mars_rover_a = Rover.new(0,0,'N')
# mars_rover_a.move()
mars_rover_b = Rover.new(1,1,'E')
#call the instruction for each instance of the object rover
mars_rover_a.instruction(['L','M','R','M','L','M','R','R','M'])
mars_rover_b.instruction(['R','M','M','L','M','L','L','M'])
javascript ruby
javascript ruby
New contributor
Kathy Toufighi is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
Kathy Toufighi is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
edited 4 mins ago


Emma
158213
158213
New contributor
Kathy Toufighi is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
asked 39 mins ago


Kathy ToufighiKathy Toufighi
42
42
New contributor
Kathy Toufighi is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
Kathy Toufighi is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
Kathy Toufighi is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
put on hold as off-topic by Jamal♦ 35 mins ago
This question appears to be off-topic. The users who voted to close gave this specific reason:
- "Code not implemented or not working as intended: Code Review is a community where programmers peer-review your working code to address issues such as security, maintainability, performance, and scalability. We require that the code be working correctly, to the best of the author's knowledge, before proceeding with a review." – Jamal
If this question can be reworded to fit the rules in the help center, please edit the question.
put on hold as off-topic by Jamal♦ 35 mins ago
This question appears to be off-topic. The users who voted to close gave this specific reason:
- "Code not implemented or not working as intended: Code Review is a community where programmers peer-review your working code to address issues such as security, maintainability, performance, and scalability. We require that the code be working correctly, to the best of the author's knowledge, before proceeding with a review." – Jamal
If this question can be reworded to fit the rules in the help center, please edit the question.
add a comment |
add a comment |
0
active
oldest
votes
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
4NXm,whH6FOj